You MUST KNOW These Traits in Rust
Summary
TLDRこの動画スクリプトでは、Rust言語の特徴的なトレイト「From」と「Into」、およびそれらのエラーハンドリングバージョン「TryFrom」と「TryInto」について解説しています。Rustにおけるデータの所有権と借用の概念を理解し、これらのトレイトを使用してAPIの使いやすさを向上させる方法を学ぶことができます。また、データの変換が失敗する可能性がある場合にどのように扱うか、そして参照と借用に関する「AsRef」と「AsMut」トレイトの使い方についても説明されています。この知識は、Rustの標準ライブラリや一般的なクレートのAPIをより理解し、より効率的にコードを書くための重要な基盤となります。
Takeaways
- 🌟 Rustの借用チェッカーを克服すると、コードがよりスムーズに流れるようになります。
- 📚 Rustにおけるidiomaticなコードを学ぶことの重要性と、特定のトレイト(from, into, try_from, try_into)を知ることがAPIの使いやすさに寄与します。
- 🔄 `from`と`into`は、Rustでの型変換の基本であり、`into`は`from`の逆演算です。
- 📊 Rustは所有データと借用データの2つのタイプがあり、`from`と`into`はこれらのデータの変換に関連しています。
- 🚀 `from`トレイトは、変換が失敗しないことを前提としており、計算コストが高い可能性があることを考慮する必要があります。
- 🔧 `try_from`と`try_into`は、失敗が可能な変換を扱うために存在し、関連型`Error`を定義することができます。
- 🛠️ 型変換を標準化することで、Rust開発者が検索しやすくなり、特定の型変換に必要なメソッドを簡単に見つけることができます。
- 📌 関数のジェネリクスと`Into`トレイトの使用により、APIのエルゴノミクスを向上させることができます。
- 🔄 `as_ref`と`as_mut`は、所有権を取得せずに値を借用するためのトレイトで、参照の変換がより簡単に行えます。
- 📝 標準ライブラリは`as_ref`と`as_mut`のブランケット実装を提供しており、異なるタイプのデータの借用を自動的に扱うことができます。
- 🔗 これらのトレイトの理解と使用は、Rustでのコードの改善と、既存のクレートやAPIの理解を深めることに役立ちます。
Q & A
Rustの借用チェッカーとは何ですか?
-Rustの借用チェッカーは、所有データと借用データの管理を担当し、安全なメモリアクセスを保証するコンパイラの機能です。
Rustにおける所有データと借用データの違いは何ですか?
-所有データは、コードブロックがメモリを解放する責任を持っており、借用データはそのメモリの解放を後で他のコードブロックが行うことになります。
FromトレイトとIntoトレイトの違いは何ですか?
-Fromトレイトは変換の所有権を取得する変換を定義し、Intoトレイトは変換の所有権を放棄する変換を定義します。IntoはFromの逆です。
Rustで標準的な変換を行うにはどうすればいいですか?
-Rustで標準的な変換を行うには、FromトレイトとIntoトレイトを実装し、それらを使用して変換を行うことが必要です。
TryFromとTryIntoはどのような状況で使用されるのでしょうか?
-TryFromとTryIntoは、変換が失敗する可能性がある場合に使用されます。これらのトレイトは、エラーを返すことができます。
Rustのトレイトに関連する型がある場合、どのようにエラーを定義するのですか?
-関連する型がある場合、Associated typeを使用してエラーの型を定義することができます。
Rustで借用参照を取得する方法は何ですか?
-Rustで借用参照を取得するには、AsRefトレイトとAsMutトレイトを使用することができます。これらは、参照を取得するためのcheapな方法を提供します。
Rustでファイルの名前を変更する方法は何ですか?
-Rustでファイルの名前を変更するには、std::fsモジュールのrename関数を使用することができます。この関数は、所有権を取得せずにパスを借用します。
Rustで標準ライブラリが提供するAsRefとAsMutの利点は何ですか?
-AsRefとAsMutは、関数に借用参照を渡す際に、コードの可読性と使いやすさを向上させます。また、標準ライブラリは、これらのトレイトのblanket実装を提供し、複数の参照を自動的にdereferenceします。
RustでAPIの使いやすさを向上させるためにどのようなアプローチがとることができますか?
-APIの使いやすさを向上させるために、関数をジェネリックにし、Intoトレイトを利用して、所有権を取得するデータを受け取ることができます。また、AsRefやAsMutを利用して借用参照を渡すこともできます。
Outlines
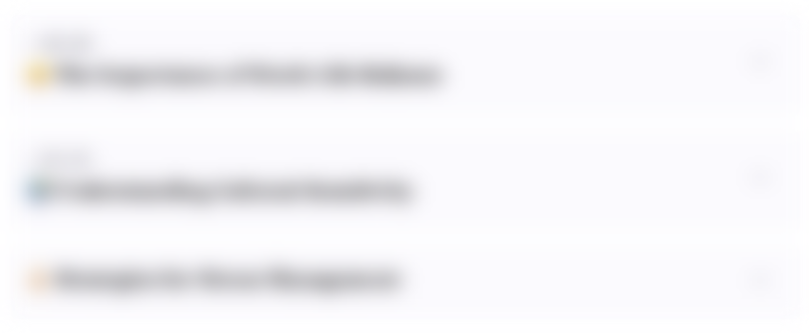
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
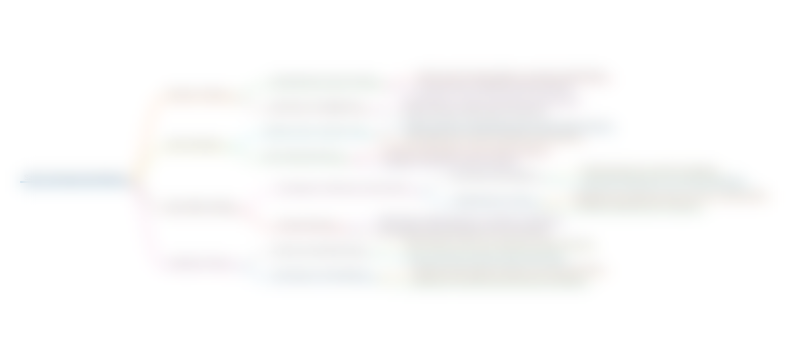
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
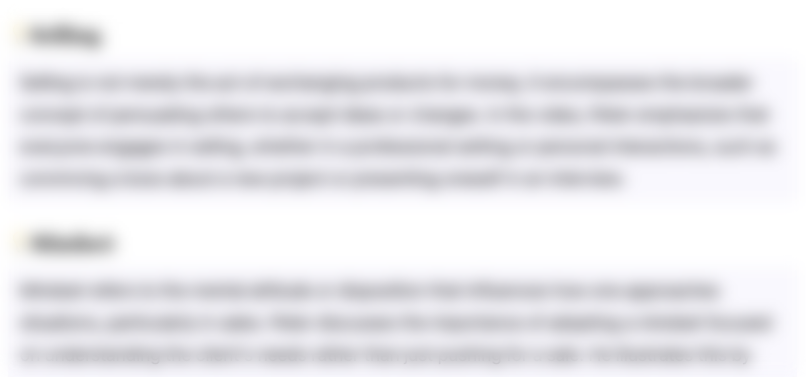
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
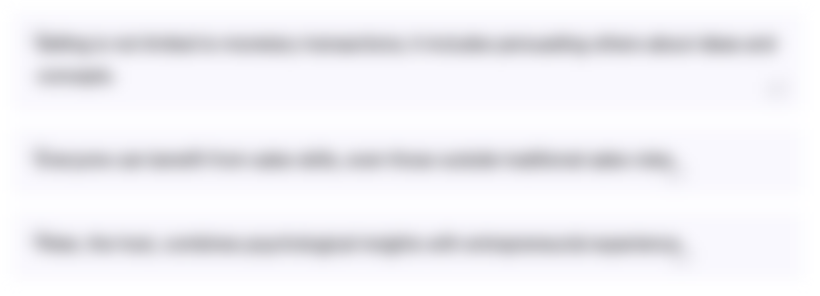
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
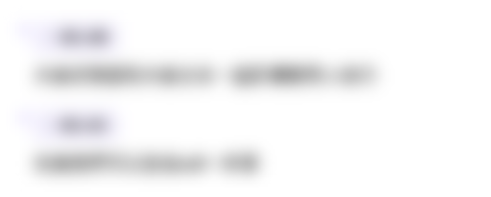
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)