Common Programming Concepts in Rust
Summary
TLDRこの動画はRustプログラミング言語についての教育チャンネル「Let's Get Rusty」の第3回目の動画です。ホストのBogdanは、基本的なプログラミング概念を紹介しています。変数の不変性と可変性、定数、型注釈、シャドウイング、スカラー型と複合型のデータ型、関数の定義と呼び出し、制御フローの構造(if文、ループ)、そしてコメントの使い方について学ぶことができます。特に、Rustにおける変数の不変性と定数の違い、配列の固定長性、関数の戻り値の指定方法、if-else文の使い方、そしてループの種類について詳しく解説しています。この動画はRustを学びたい人にとって、非常に役立つ内容となっています。
Takeaways
- 📌 Rust は変数の不変性をデフォルトでサポートしています。
- 🔄 変数を再代入するには、`let` キーワードの後に `mut` を追加する必要があります。
- 🔒 `const` キーワードを使用して定数を定義し、変更できない値を作成できます。
- 📈 Rust は整数、浮動小数点数、ブール値、文字の4つの主要なスカラーデータ型をサポートしています。
- 🔢 Rust の整数型には、符号付きと符号なし、および異なるビットサイズ(8, 16, 32, 64, 128ビット)があります。
- 🔄 Rust は整数オーバーフローを検出し、デバッグビルドではパニックを起こし、リリースビルドでは2の補数巻戻しを行います。
- 📊 Rust はタプル型と配列型を備えており、タプルは異なる型の固定サイズのデータグループを表し、配列は固定長のデータ構造です。
- 🔧 Rust の関数は `fn` キーワードを使用して宣言され、引数を受け取ることができます。
- 🔄 Rust の制御フローには if 文、while ループ、for ループがあります。
- 📝 Rust には1行コメントとブロックコメントがあり、コードの説明や無効化に使用されます。
- 🔄 Rust 関数は値を返すことができます。最後の式は省略可能で、関数の戻り値として使用されます。
Q & A
Rust言語における変数の初期値設定はどのように行われますか?
-Rustでは、変数は`let`キーワードを使って初期値を設定します。例えば、`let x = 5;`のように宣言と同時に初期値を設定できます。
Rustにおける不変変数と可変変数の違いは何ですか?
-Rustでは、変数はデフォルトで不変(immutable)です。不変変数は一度値が設定されると、その後変更することができません。可変変数を作り、値を再代入するには、`let mut`を使用します。
Rustで定数を定義する方法は何ですか?
-Rustで定数を定義するには`const`キーワードを使用します。例えば、`const SUBSCRIBER_COUNT: u32 = 100_000;`のように、型注釈も必要です。定数は変更できないため、安全にグローバルにアクセスできます。
Rustにおけるシャドウイングとは何ですか?
-シャドウイングは、既存の変数名を使って新しい変数を宣言する機能です。これにより、前の変数が新しい変数によって遮られます。シャドウイングされた変数は、前の変数とは型が異なる場合もあります。
Rustのスカラーデータ型にはどのようなものがありますか?
-Rustのスカラーデータ型には、整数(整数型)、浮動小数点数(浮動小数点型)、ブール値(boolean型)、文字(character型)の4つがあります。
Rustのタプル型はどのように定義されるか?タプルの値を取得する方法は?
-タプル型は、異なる型のデータを含む固定サイズの配列のようなものです。定義は`(データ1, データ2, ...)`の形式で行われます。タプルの値を取得には、解構(destructuring)またはドット記法(dot notation)を使用できます。
Rustの配列はどのように宣言され、アクセスされるか?
-配列は`[データ1, データ2, ...]`の形式で宣言され、固定長です。配列の要素にアクセスするには、ブラケット記法(`配列名[index]`)を使用します。ただし、範囲外のインデックスにアクセスしようとすると、境界外例外が発生します。
Rustの関数はどのように定義されるか?
-関数は`fn`キーワードを使って定義されます。関数名はスネークケース(snake_case)で記述し、引数は括弧内に型注釈付きの名前を指定します。
Rustの制御フローにおいて、if文はどのように使われますか?
-Rustのif文は、条件が真である場合にコードブロックを実行します。else ifとelseブロックも使用でき、条件は明示的にブール値でなければなりません。
Rustのループにはどのような種類がありますか?
-Rustのループには、`loop`、`while`、および`for`の3種類があります。`loop`は無条件にループし、`while`は条件が真である間ループし、`for`はコレクションを反復処理するために使用されます。
Rustのコメントにはどのような種類がありますか?
-Rustには、単一行コメント(`//`)とブロックコメント(`/* ... */`)の2種類があります。また、文書コメント(`///`)もありますが、これは別の用途に使われます。
Rustの関数から値を返す方法は?
-Rustの関数は、`return`文を使って値を返すことができます。また、関数の最後の式は暗黙的に返されます。関数の戻り値の型は、関数定義の後ろに指定することができます。
Outlines
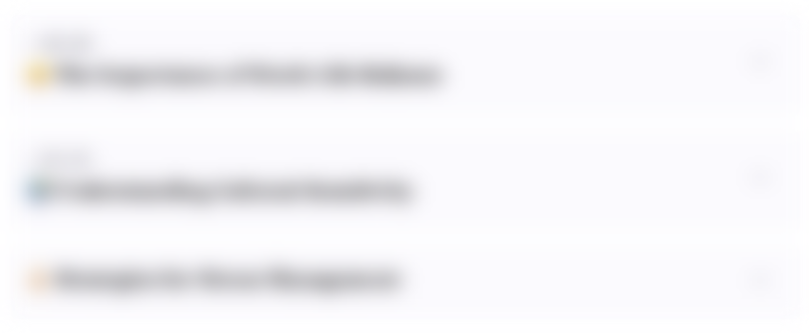
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
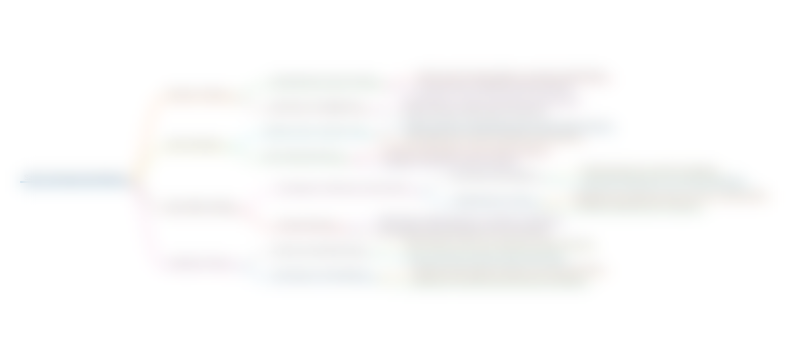
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
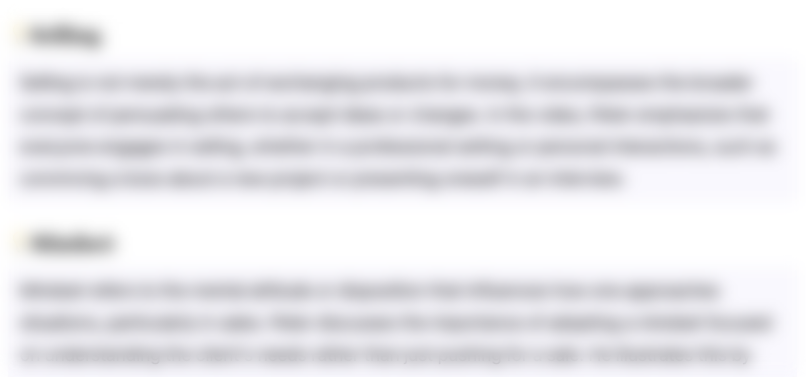
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
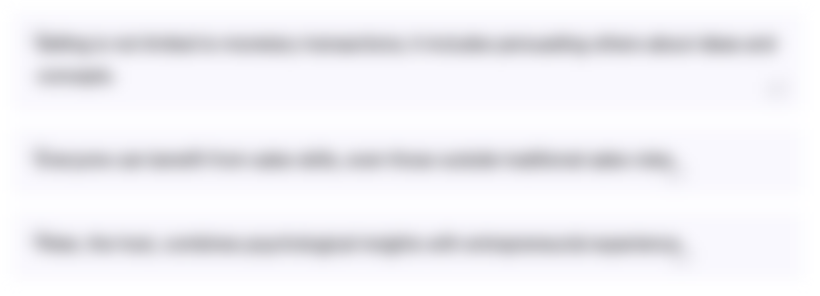
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
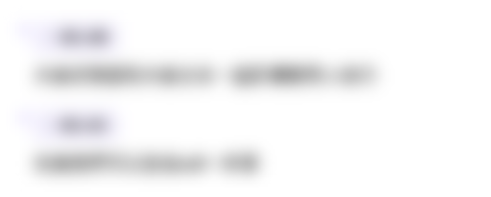
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)