The Key Prop | Lecture 128 | React.JS 🔥
Summary
TLDRThis video script offers an in-depth explanation of the key prop in React, a crucial concept for optimizing performance and managing state. It begins by introducing the key prop and its role in the diffing algorithm, enabling React to uniquely identify elements. The script then delves into the two main use cases: using stable keys in lists to preserve DOM elements across renders, and changing keys to reset component state when necessary. With clear examples and a logical progression, the script aims to provide viewers with a comprehensive understanding of when and why to use the key prop, reinforcing best practices for efficient React development.
Takeaways
- 🔑 The `key` prop is a special prop in React that allows the diffing algorithm to uniquely identify elements, both DOM elements and React elements.
- ✅ Using a stable `key` (one that stays the same across renders) for an element allows React to keep that element in the DOM even if its position in the tree changes, resulting in better performance.
- ♻️ When the `key` of an element changes between renders, React will destroy the old element and create a new one in its place, even if the position in the tree is the same, allowing for state reset.
- 📝 Use the `key` prop when rendering lists of elements of the same type, otherwise React will complain and give a warning.
- ⚡ Using `key` properly can greatly improve performance, especially when dealing with large lists with thousands of elements.
- 🔄 To reset the state of a component instance, change the `key` prop of that instance, forcing React to create a new instance with a fresh state.
- 🧠 React cannot inherently determine if an element is the same across renders, so using `key` provides that information to React.
- 🚫 Without using `key` in lists, React will remove and recreate DOM elements unnecessarily, leading to wasted work and decreased performance.
- 🔑 The two main use cases for the `key` prop are: 1) Using stable keys in lists, and 2) Changing keys to reset component instance state.
- 📖 The script provides a clear explanation of the `key` prop and its importance, along with practical examples and use cases.
Q & A
What is the purpose of the key prop in React?
-The key prop is a special prop that we can use to uniquely identify elements in React. It helps the diffing algorithm distinguish between multiple instances of the same component type, allowing React to keep track of which elements have been modified, added, or removed.
Why is it important to use keys when rendering lists in React?
-When rendering lists of components, it's crucial to provide a unique key for each item. This allows React to efficiently update the list when items are added, removed, or reordered. Without keys, React would have to re-render the entire list, which can lead to performance issues, especially for large lists.
What happens when an element has a stable key across renders?
-If an element has a stable key (a key that stays the same across renders), React will keep that element in the DOM, even if its position in the tree has changed. This behavior improves performance by avoiding unnecessary re-renders or recreations of the same DOM element.
What is the purpose of changing an element's key across renders?
-Changing an element's key across renders tells React to treat it as a different instance, even if the element's position in the tree is the same. This is useful when you need to reset the state of a component instance, as React will create a new DOM element and discard the previous state.
How does the key prop affect state preservation in React components?
-If an element's key is unchanged and its position in the tree is the same, React will preserve its DOM element and state across renders. However, if the key changes, React will create a new DOM element and reset the component's state.
Can you provide an example of when changing an element's key would be useful?
-One scenario where changing an element's key would be useful is when you have a component that displays a question, and the question changes. By changing the key, you can force React to create a new component instance with a fresh state, ensuring that the old answer doesn't persist and confuse the user.
What happens if you don't provide keys when rendering a list of components in React?
-If you don't provide keys when rendering a list of components, React will issue a warning in the console. Additionally, when items are added, removed, or reordered in the list, React will have to re-render the entire list, which can lead to performance issues, especially for large lists.
Is it necessary to use keys for all React components?
-No, it's not necessary to use keys for all React components. Keys are primarily used when rendering lists of components or when you need to reset the state of a component instance based on changes in props or other conditions.
Can keys be duplicated within the same list of components?
-No, keys must be unique within the same list of components. Duplicating keys can lead to unexpected behavior and potential bugs in your React application.
What data type can be used for keys in React?
-Keys in React can be strings or numbers, but it's recommended to use strings for keys when possible, as they provide a more reliable way of uniquely identifying components.
Outlines
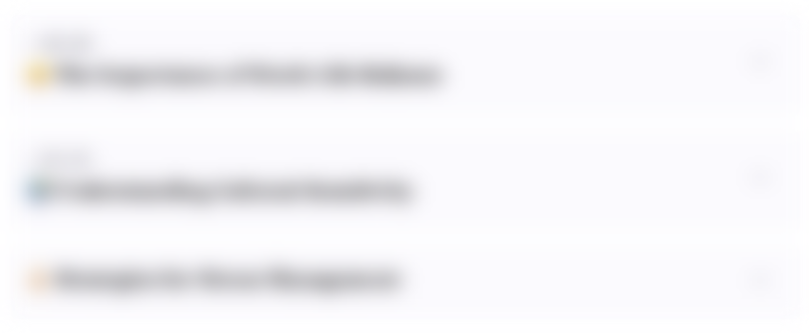
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
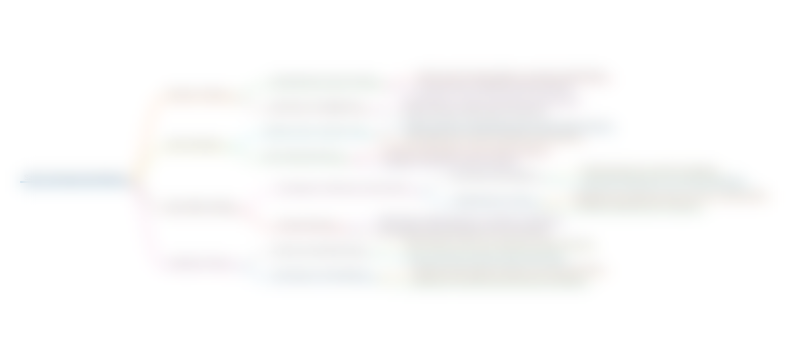
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
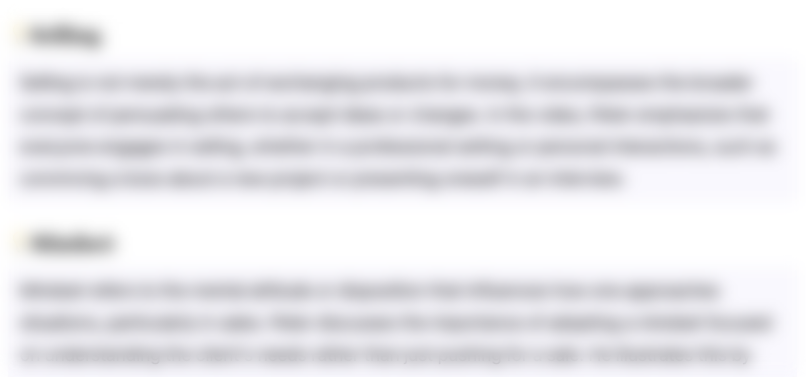
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
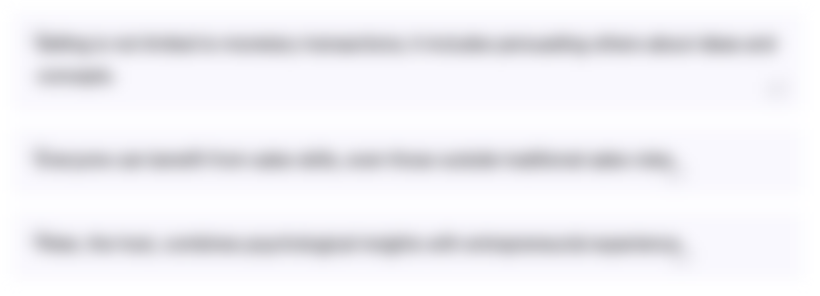
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
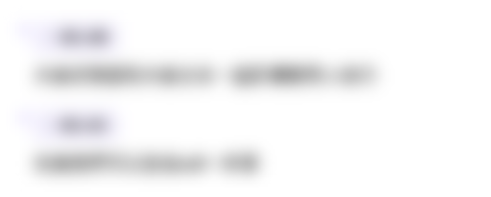
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Elements, Children and Re-renders - Advanced React course, Episode 2
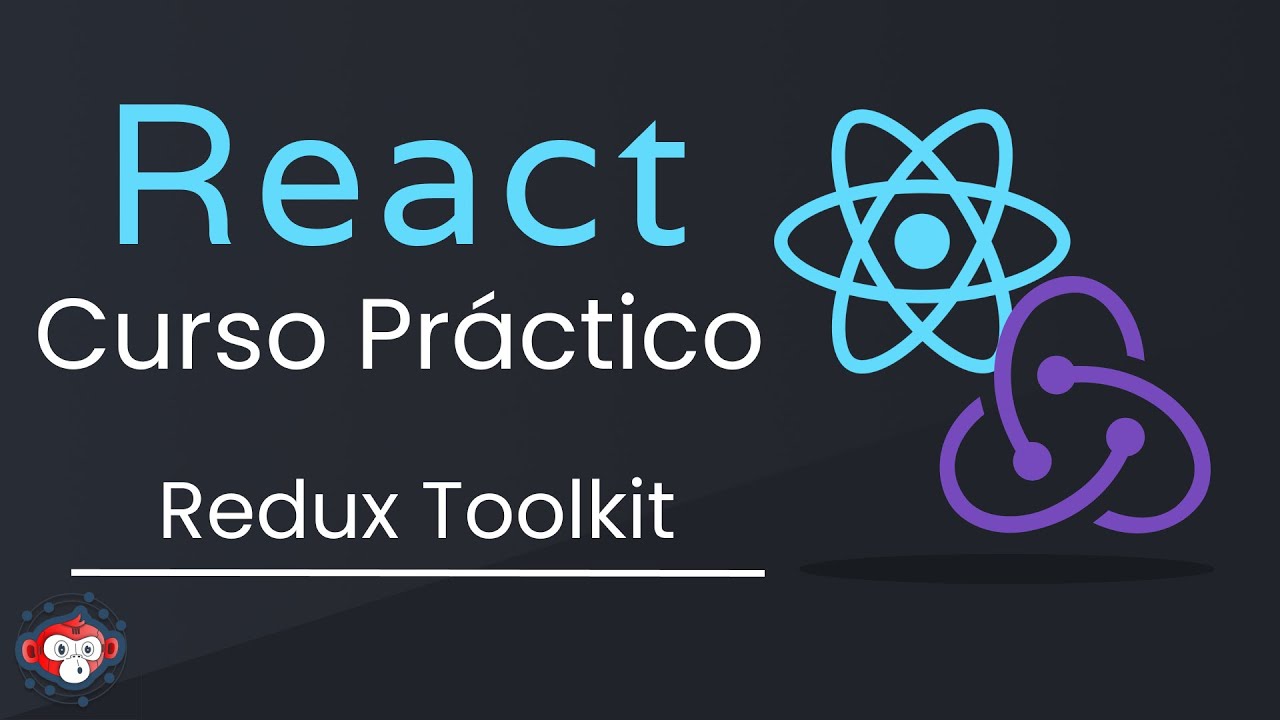
React & Redux Toolkit - Bases y proyecto práctico
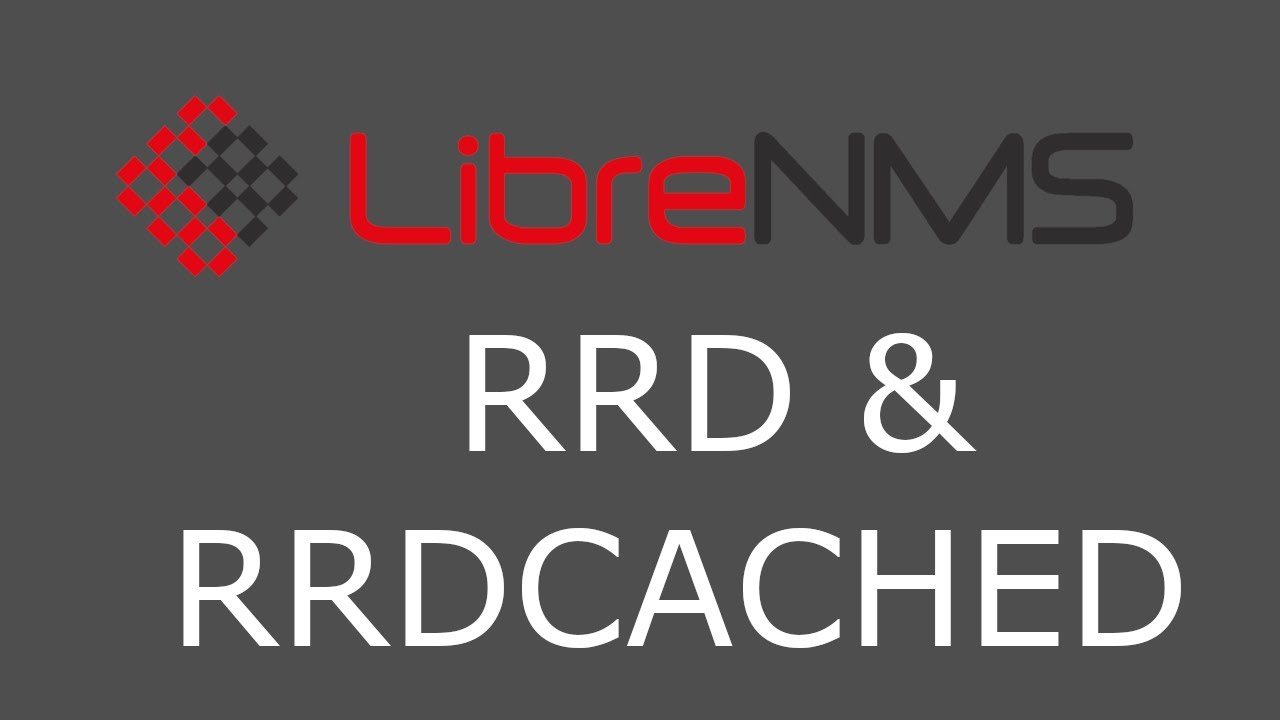
LibreNMS RRD & RRDCached
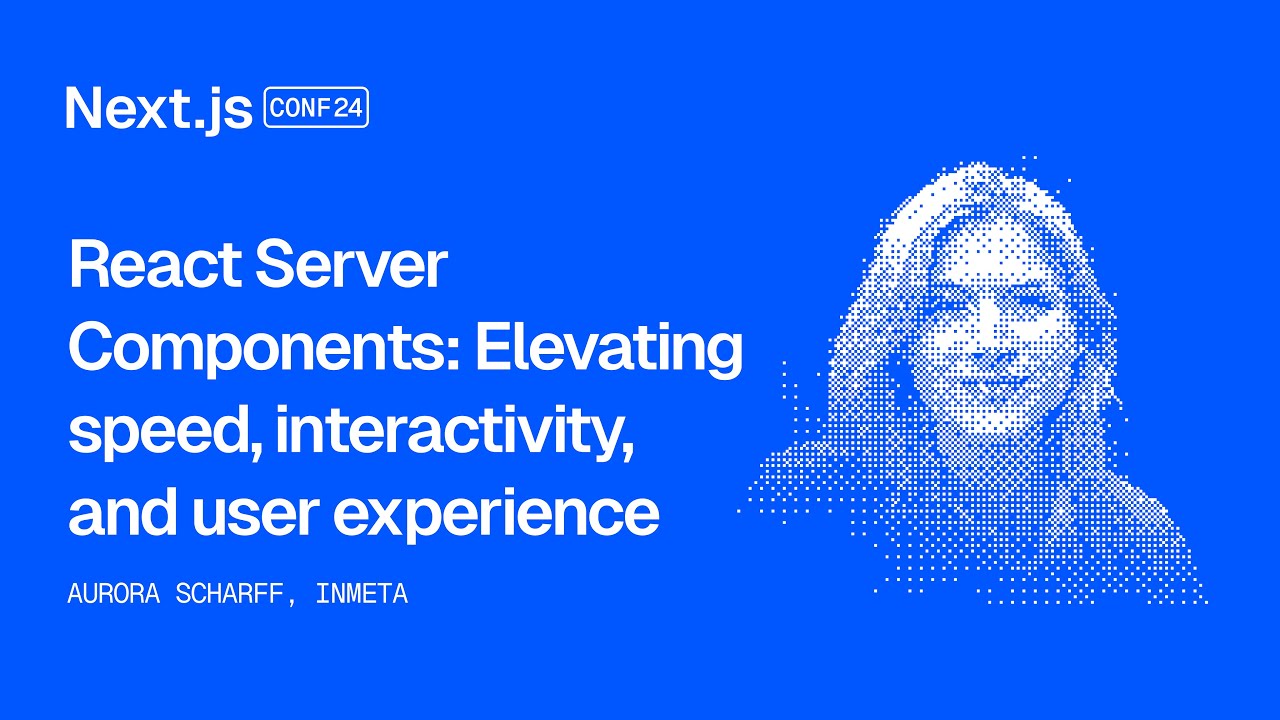
React Server Components: Elevating speed, interactivity, and user experience (Aurora Scharff)

Imperator rome: Гайд по экономике и старту. (без воды и по факту)

Dcuo gadgets dps omega loadout
5.0 / 5 (0 votes)