Default Parameters | JavaScript ๐ฅ | Lecture 119
Summary
TLDRThe video delves into the concept of default parameters in JavaScript functions, introduced in ES6. It starts by demonstrating the traditional ES5 approach, using logical OR operators and falsy values to set default parameter values. The instructor then introduces the more concise and intuitive ES6 syntax, where default values can be assigned directly in the parameter list. The video also explores the ability to use expressions and other parameter values to calculate dynamic defaults. Additionally, it covers the technique of passing `undefined` to skip a parameter and retain its default value. Throughout the tutorial, the instructor incorporates practical examples and explanations, making it an engaging educational resource on this JavaScript feature.
Takeaways
- ๐ Default parameters in JavaScript functions allow you to set a default value for a parameter in case it is not provided when calling the function.
- ๐ Default parameters in ES6 can be set by assigning a value to the parameter in the function declaration, e.g., `function(param = 'default')` .
- ๐ข Default parameter values can be any expression, including calculations based on other parameters defined before it.
- โ ๏ธ You cannot skip arguments when calling a function; if you want to use a default value for a parameter, you must pass `undefined` as the argument for that parameter.
- ๐ Default parameters make code more concise and readable compared to the old ES5 way of using logical OR operators to handle default values.
- ๐ The provided script uses an airline booking example to demonstrate the use of default parameters.
- ๐ The script assumes strict mode is enabled, and it uses enhanced object literal syntax and array methods like `push()`.
- ๐ The script suggests reloading the page in the browser to see the updated output after making changes.
- ๐ ES6 introduced improvements to JavaScript, including default parameters, which make code more expressive and easier to write and maintain.
- ๐งโ๐ป Understanding default parameters is essential for writing more robust and maintainable JavaScript functions.
Q & A
What is the purpose of this section in the video?
-The purpose of this section is to explain default parameters in JavaScript functions, which allow you to set default values for function parameters when they are not explicitly provided.
How were default parameters implemented before ES6?
-Before ES6, default parameters were implemented using logical operators like the OR operator (||) to check if a parameter was falsy (e.g., undefined) and then assign a default value if it was.
What is the ES6 syntax for setting default parameters?
-In ES6, you can set default parameters by assigning a value to the parameter in the function declaration, like so: `function(param = defaultValue) {...}`.
Can default parameters be dynamic expressions?
-Yes, default parameters can be dynamic expressions. You can use any valid JavaScript expression as the default value, including calculations based on other parameters.
Is it possible to skip a parameter and use the default value?
-Yes, you can skip a parameter and use its default value by passing `undefined` as the argument for that parameter when calling the function.
What is the advantage of using default parameters?
-Default parameters make it easier to handle optional function arguments, reducing the need for additional checks and assignments within the function body. They also improve code readability and maintainability.
Can default parameters reference parameters that are defined after them?
-No, default parameters can only reference parameters that are defined before them in the parameter list. This is because JavaScript evaluates parameters in order.
What is the purpose of the `createBooking` function in the example?
-The `createBooking` function is used to create an object representing a booking for an airline, with properties like flight number, number of passengers, and price. It demonstrates the use of default parameters.
How are the bookings stored in the example?
-The bookings are stored in an array called `bookings`. Each time the `createBooking` function is called, the resulting booking object is pushed into the `bookings` array.
What is the purpose of using `strict mode` in the example?
-The example uses `strict mode` in JavaScript, which enforces stricter parsing and error handling, and eliminates some silent errors that can occur in non-strict mode. It helps in writing more robust and secure code.
Outlines
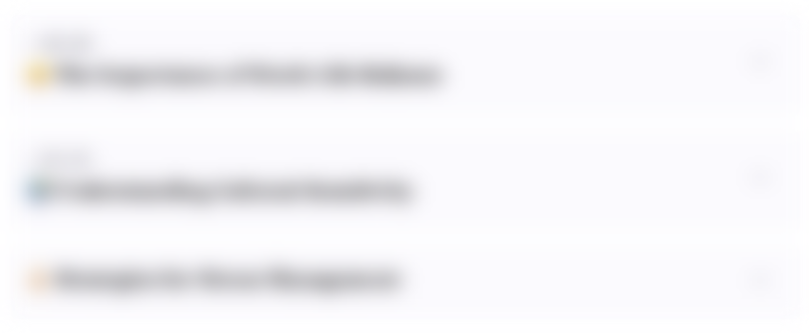
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
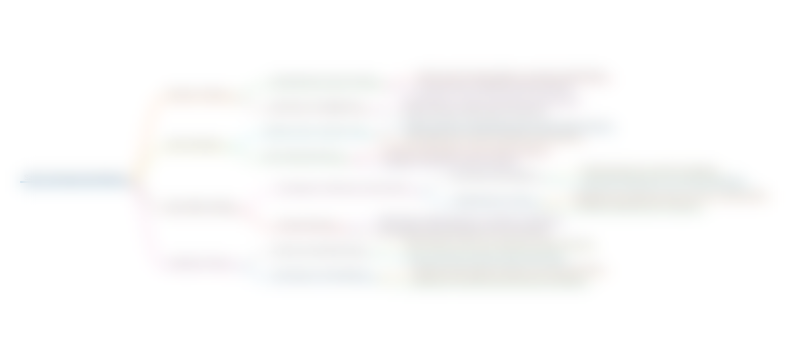
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
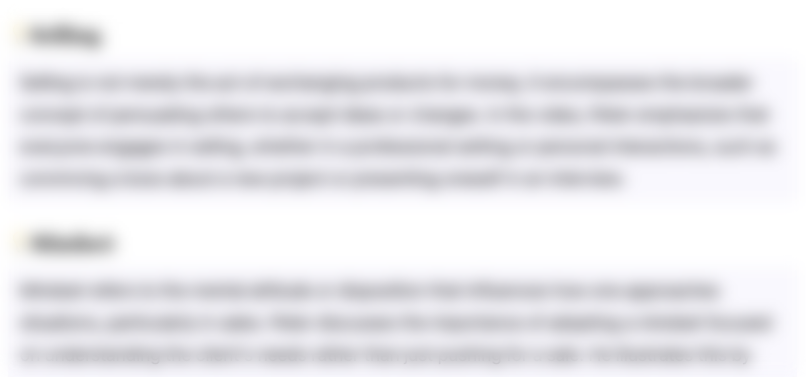
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
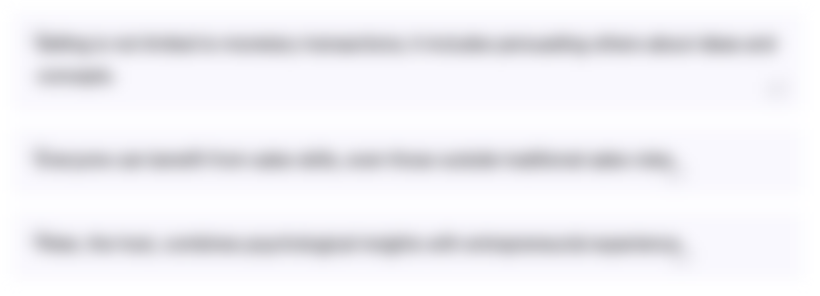
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
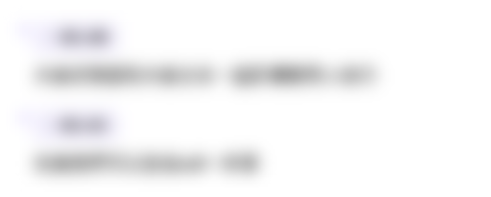
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
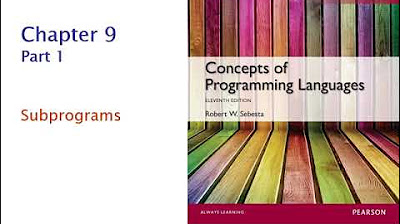
COS 333: Chapter 9, Part 1
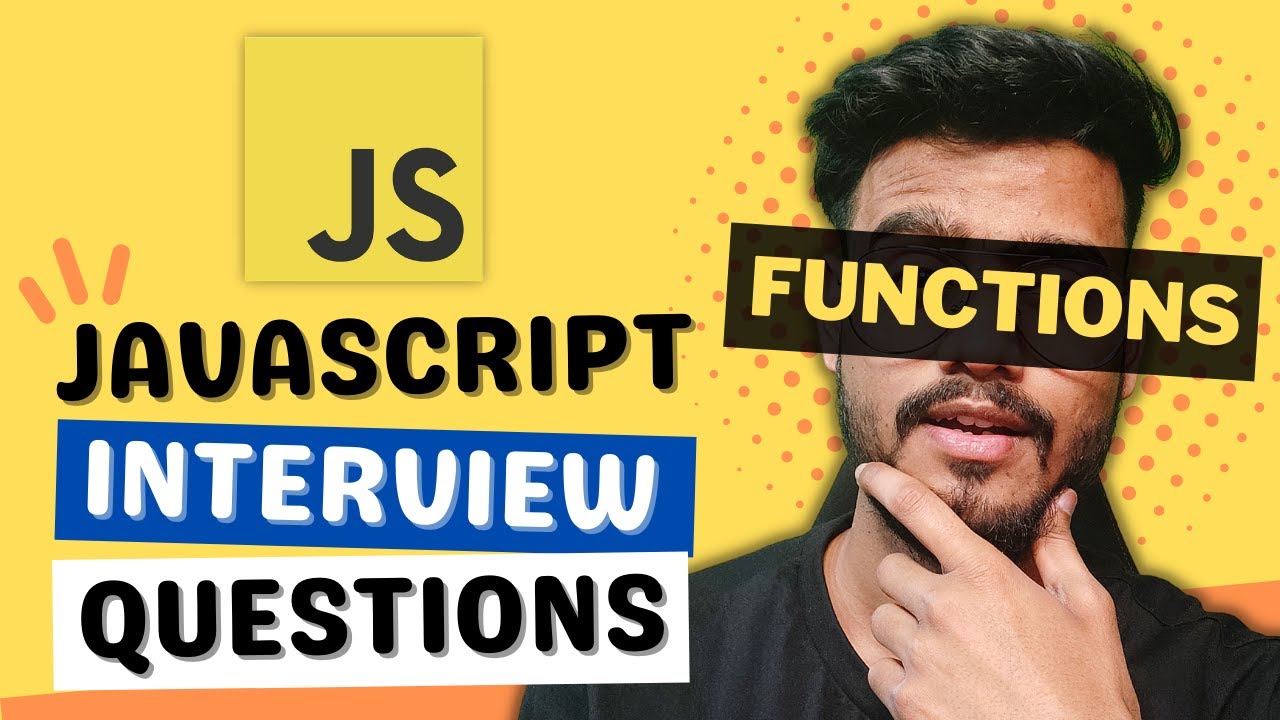
Javascript Interview Questions ( Functions ) - Hoisting, Scope, Callback, Arrow Functions etc
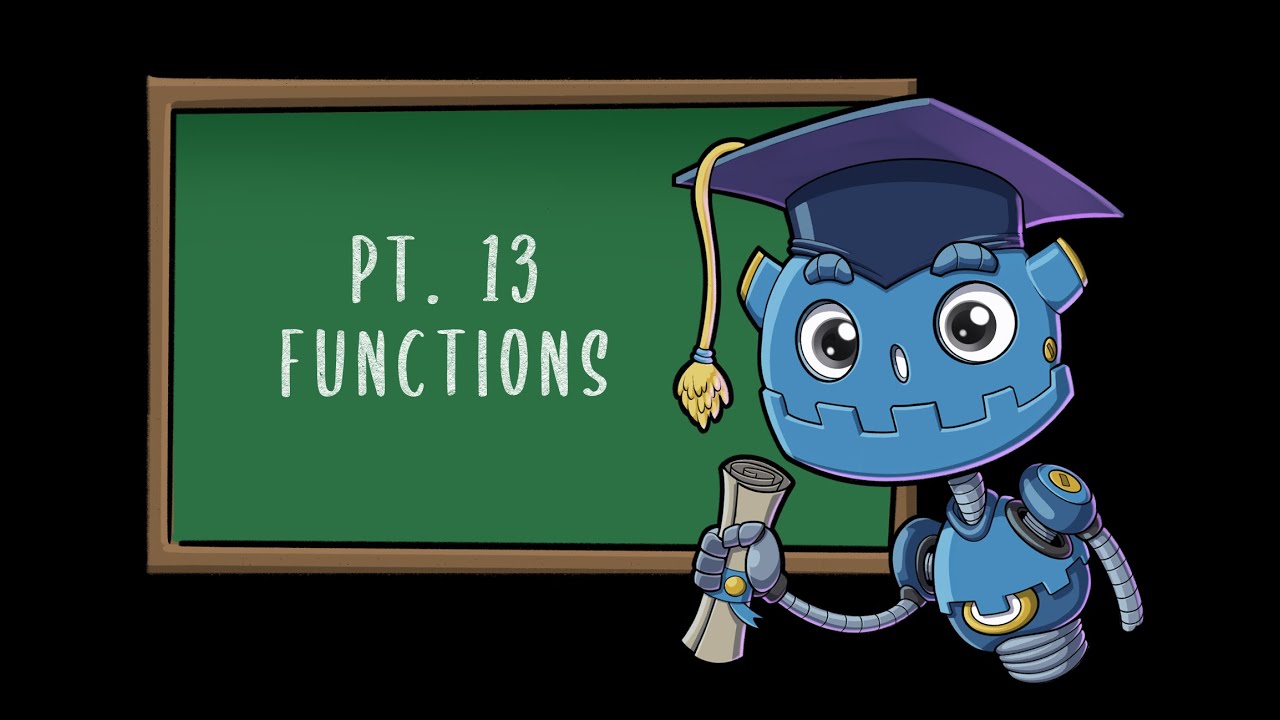
Functions | Godot GDScript Tutorial | Ep 13
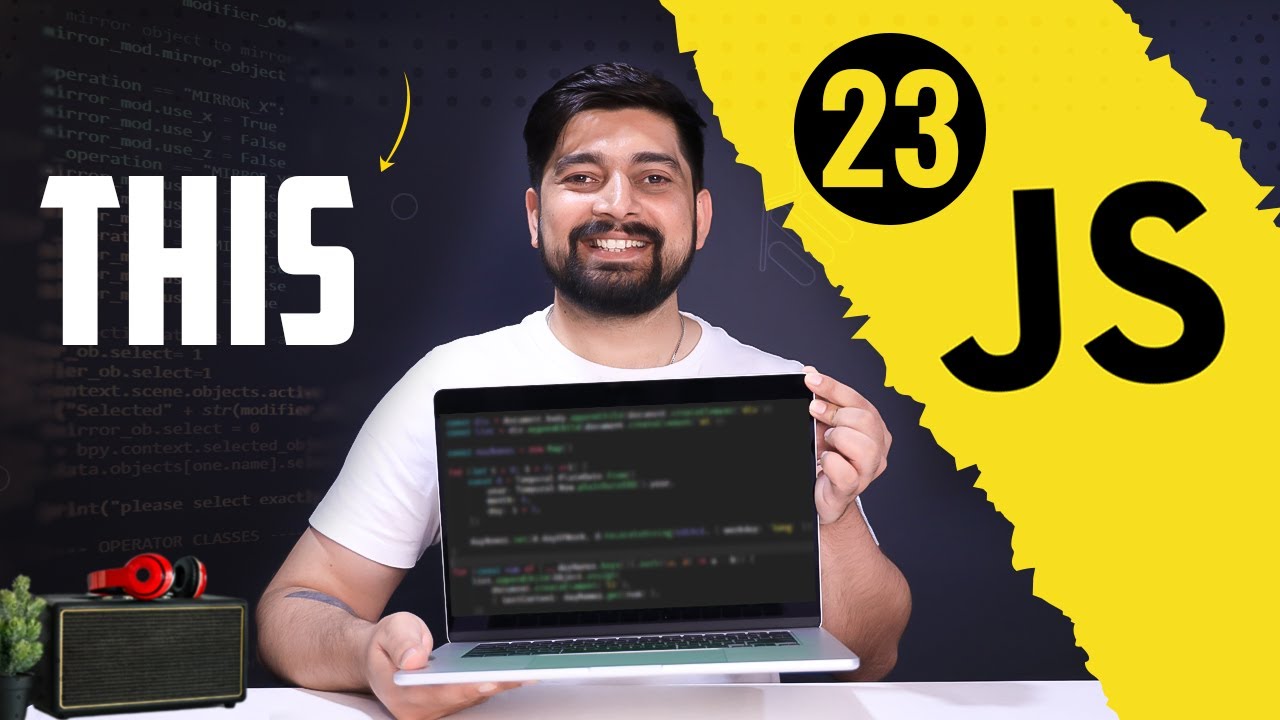
THIS and arrow function in javascript | chai aur #javascript
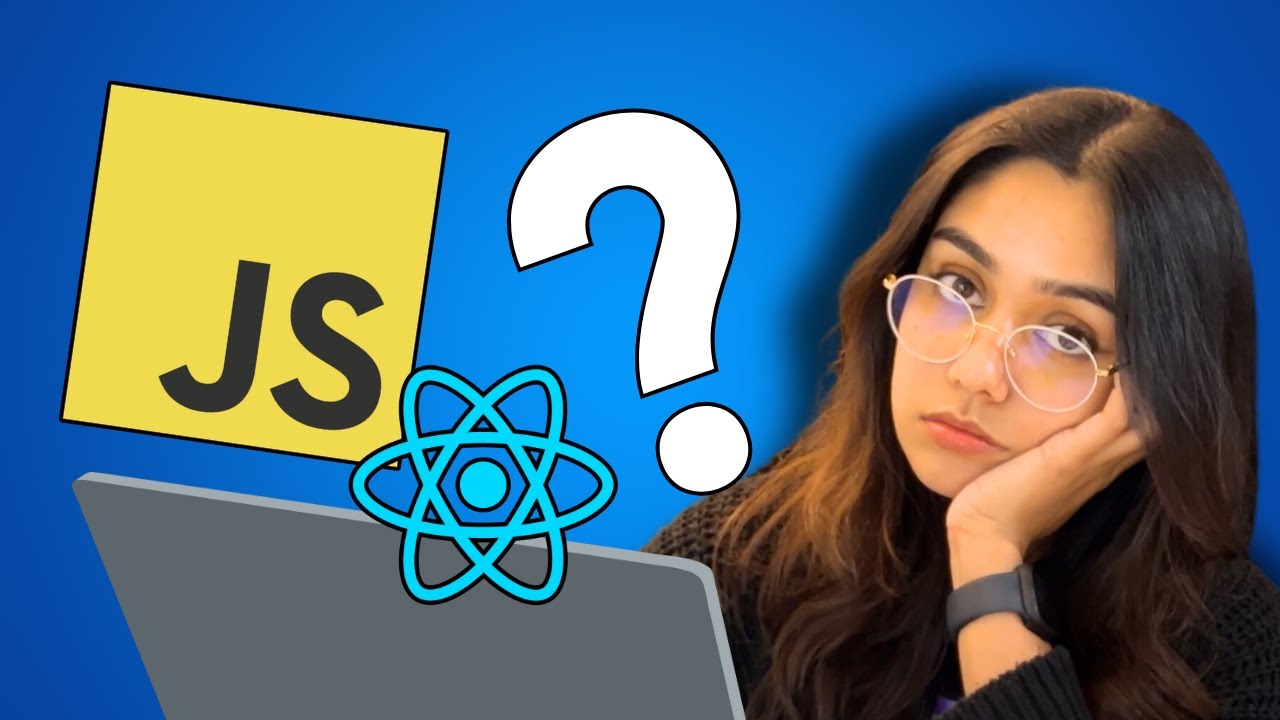
How much JavaScript do you need to learn React?

ES6 #1: Spread & Rest Operator in ES6 in JavaScript in Hindi | Technical Suneja
5.0 / 5 (0 votes)