Next.js App Router Authentication (Sessions, Cookies, JWTs)
Summary
TLDRIn this video, the presenter demonstrates how to implement basic session authentication in Next.js without additional libraries, focusing on creating, managing, and securing sessions using cookies and JWTs. The tutorial covers essential aspects such as login, logout, and session refresh, highlighting the use of Next.js functions and middleware. Additionally, the video explores using the NextAuth.js library for more advanced and abstracted authentication, supporting multiple providers like GitHub. The presenter emphasizes the importance of securing secret keys and touches on future updates and deeper topics like authorization and database sessions.
Takeaways
- 🔐 The video covers basic session authentication in Next.js without additional libraries, as well as other options if you prefer not to build your own authentication system.
- 🖥️ The example begins with a simple Next.js application setup, showing how to handle sessions in the root layout and index route.
- 📋 The session management involves setting up a login function that processes form data, creates a session object, and stores it as an HTTP-only cookie using Next.js's cookies function.
- 🔒 Encryption of the session data is handled with the Jose library, which signs the JWT (JSON Web Token) using a secret key. This key should be kept secure, ideally in an environment variable.
- 🕒 The session expiration is managed by updating the session cookie every time the user interacts with the application, ensuring it doesn't expire prematurely.
- 🛠️ Middleware is used to refresh the session on every request by decrypting the session data, updating the expiration time, and setting a new cookie.
- 📂 The video also demonstrates how to read the session information from the cookies using a getSession function, allowing the application to display user-specific data.
- 🚪 The logout function simply deletes the session cookie, effectively logging the user out.
- 🧩 The video contrasts the basic custom authentication setup with the more robust NextAuth.js library, which provides built-in support for various authentication providers like GitHub.
- 📚 The video concludes by mentioning additional resources and documentation on more advanced topics like authorization, protecting server actions, and integrating with other popular authentication libraries like Auth0, Supabase, and Clerk.
Q & A
What is the primary focus of the video?
-The primary focus of the video is to demonstrate how to implement basic session authentication in Next.js without using any additional libraries, followed by an introduction to using NextAuth.js for more complex authentication setups.
What is the purpose of the `login` function in the code example?
-The `login` function handles user authentication by processing form data, creating a session for the user, and setting it as an HTTP-only cookie. This session is encrypted using a JSON Web Token (JWT) before being stored.
Why is the session stored as an HTTP-only cookie?
-The session is stored as an HTTP-only cookie to ensure that it can only be accessed by the server, enhancing security by preventing client-side scripts from accessing the session data.
What role does the `encrypt` function play in the authentication process?
-The `encrypt` function is responsible for signing and encrypting the JWT, which contains the session data. This ensures that the session information is secure and can only be decrypted by the server.
How does the middleware in Next.js contribute to session management?
-The middleware runs before every request in the application. It updates the session expiration time by calling the `updateSession` function, which refreshes the session if a valid session cookie is present.
What happens when the `getSession` function is called?
-The `getSession` function reads the session cookie, decrypts it, and returns the session data. This allows the application to retrieve information about the logged-in user, such as their email address.
How does the logout process work in this authentication setup?
-The logout process involves calling the `logout` function, which deletes the session cookie using the `cookies` function in Next.js. This effectively logs the user out by removing their session data.
What is the advantage of using NextAuth.js over the custom authentication setup?
-NextAuth.js provides a higher level of abstraction, simplifying the implementation of authentication by offering built-in support for various providers like GitHub, Google, and others. It handles many aspects of authentication, including session management and route protection, without requiring the developer to write as much custom code.
How does NextAuth.js manage sessions differently from the custom setup?
-NextAuth.js abstracts the session management by automatically handling session tokens, CSRF protection, and session refreshes. It also offers a middleware with configurable options to control when and where the session management should run.
What are some key differences between cookie-based sessions and database sessions?
-Cookie-based sessions store session data directly in the client's cookies, often as an encrypted JWT. Database sessions, on the other hand, store session data on the server side in a database, with the client only holding a session identifier. This can provide additional security and scalability benefits, especially for larger applications.
Outlines
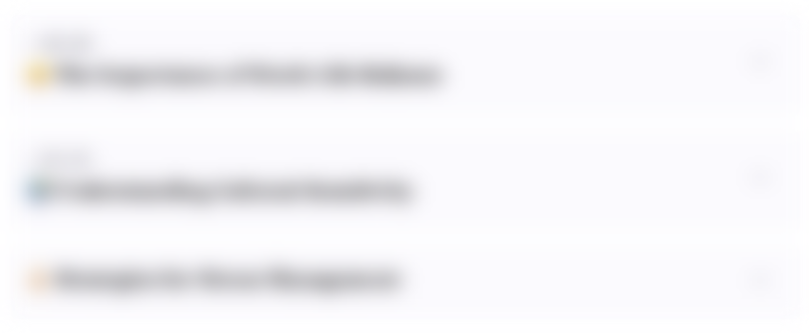
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
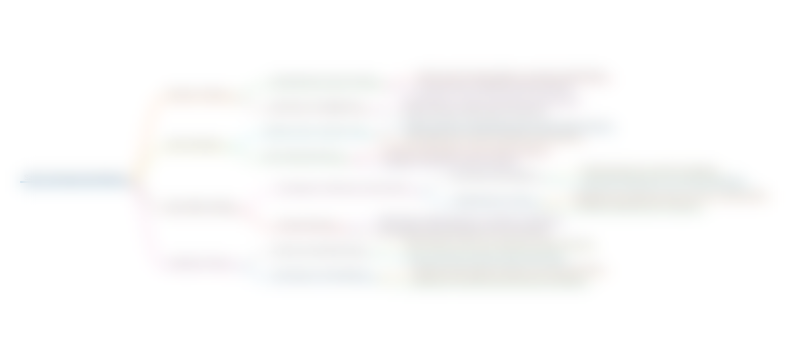
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
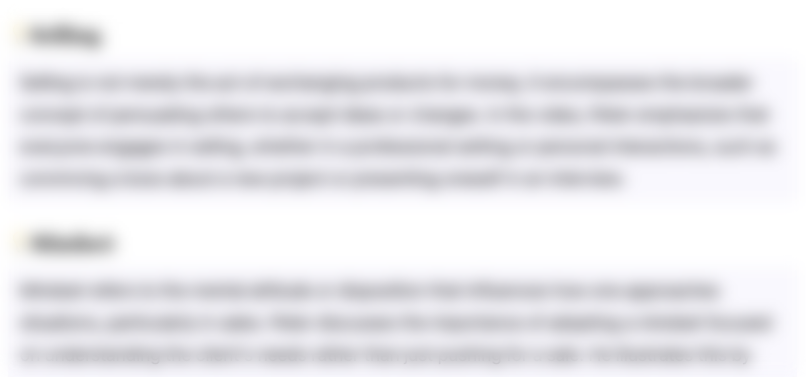
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
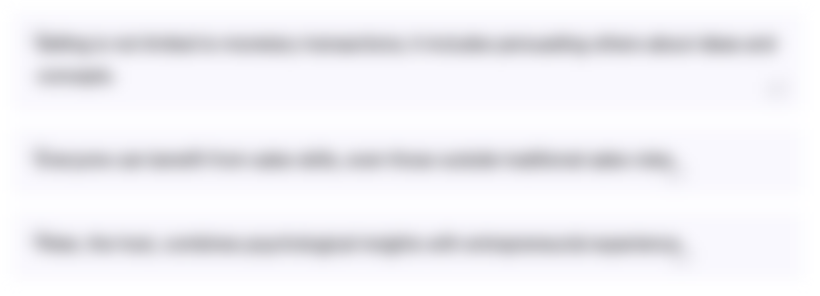
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
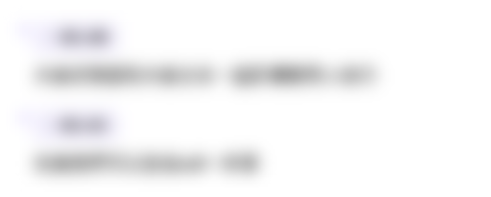
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
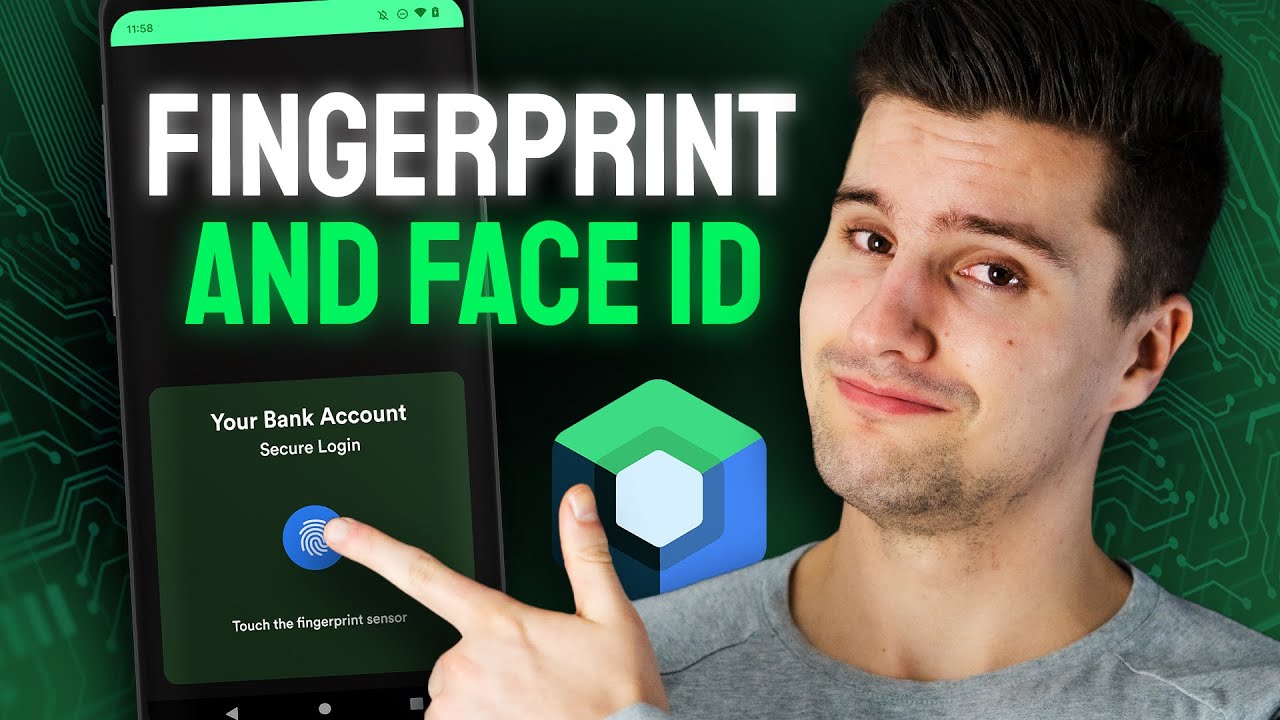
How to Implement Biometric Auth in Your Android App
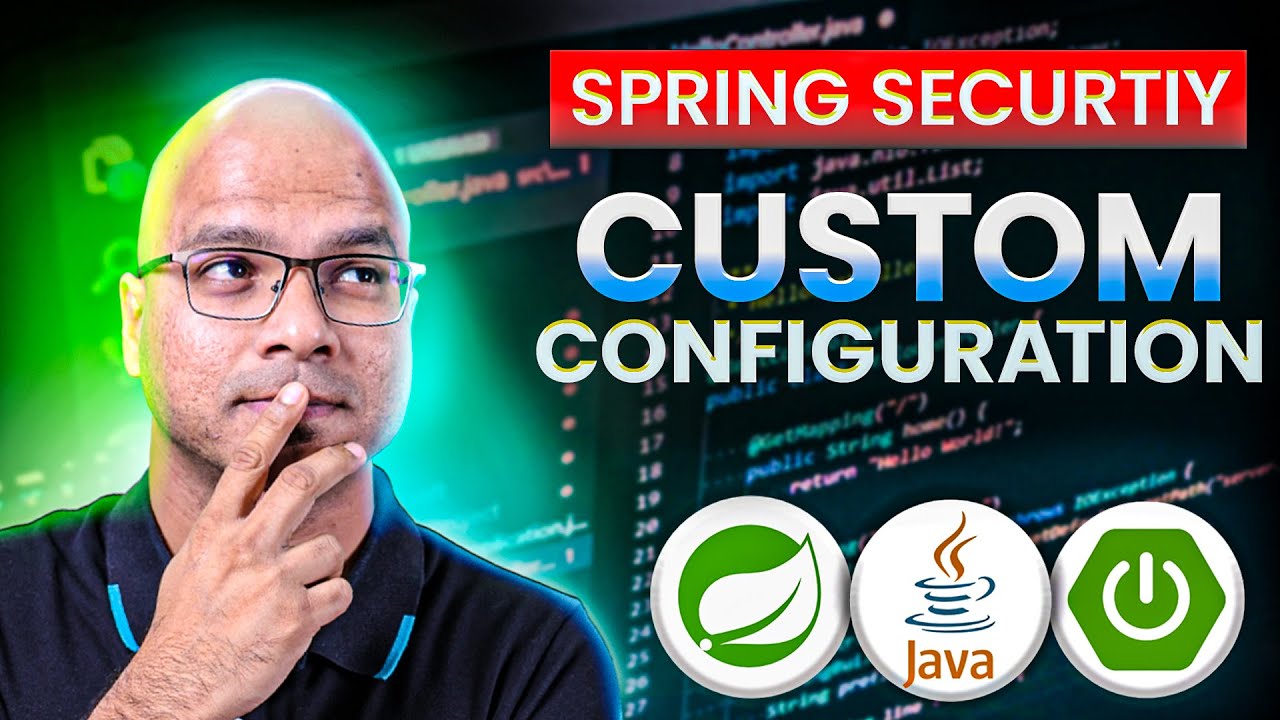
#32 Spring Security | Custom Configuration

#12 Creating Routes in Node JS | Fundamentals of NODE JS | A Complete NODE JS Course
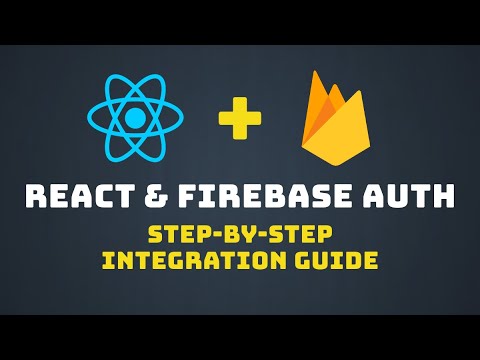
Setting Up Firebase Auth with React: Step-by-Step Tutorial
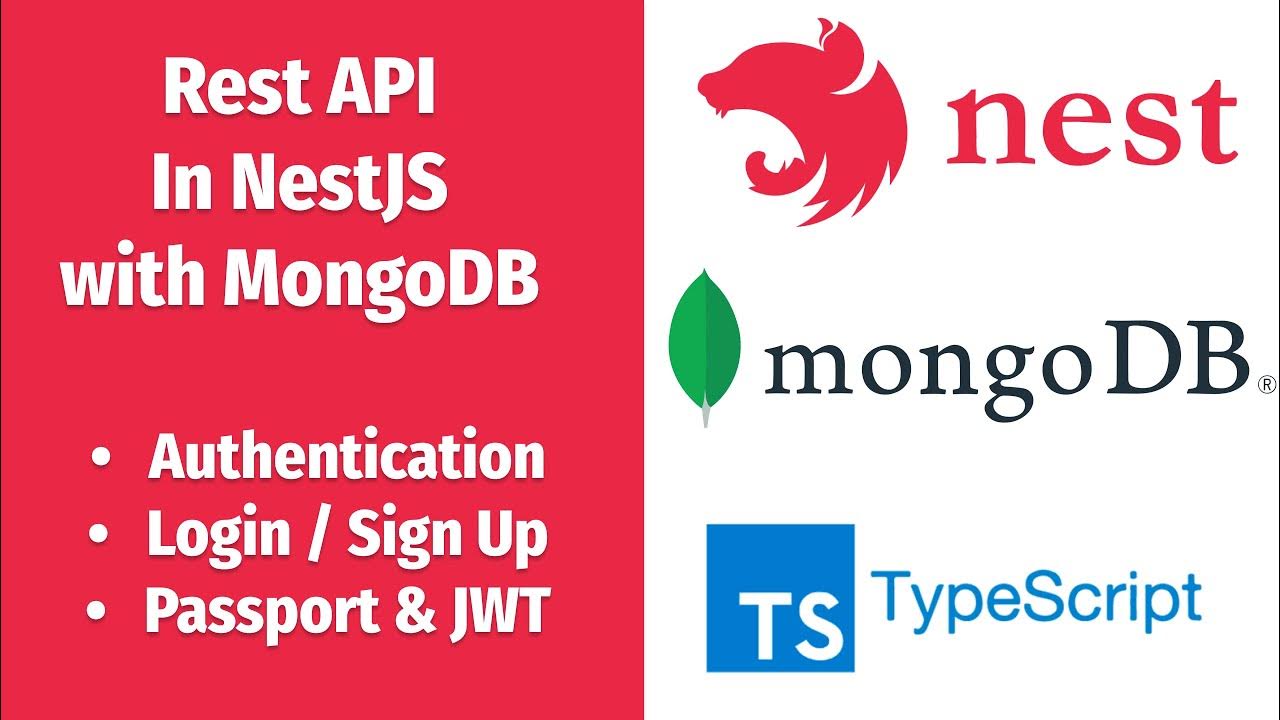
NestJs REST API with MongoDB #4 - Authentication, Login/Sign Up, assign JWT and more
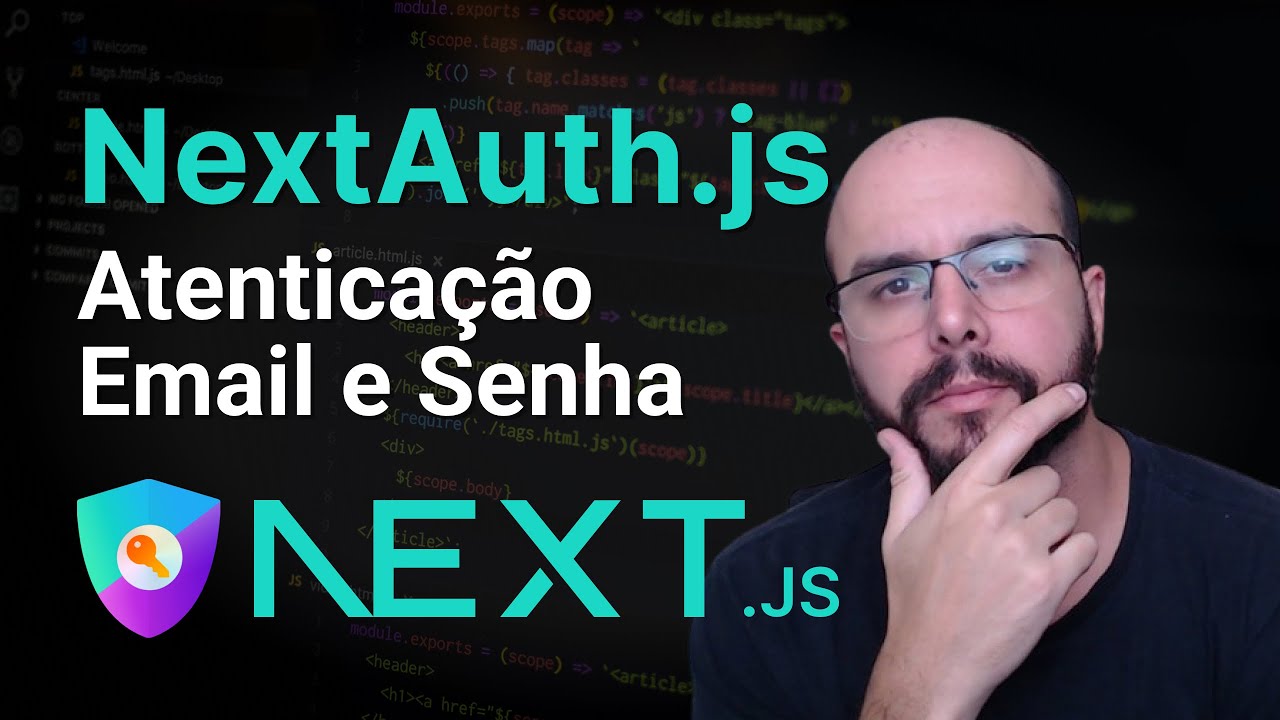
Autenticação com NextJS e Next Auth
5.0 / 5 (0 votes)