Karel Python - Control Structures
Summary
TLDRThis video explores control structures in programming through a practical example. It explains the use of if, if-else, for, and while loops to direct the flow of a program. The example involves a character named Carol who must collect tennis balls across a screen of unknown size. The script demonstrates a common issue called the 'fencepost problem,' where the last item is often overlooked. The video concludes by illustrating how to solve this problem using control structures, ensuring all balls are collected even in differently sized worlds.
Takeaways
- 📚 Control structures are programming constructs that direct the flow of a program, including if statements, if-else statements, for loops, and while loops.
- 🔍 The if statement and if-else statement are used to ask questions about the world and make decisions based on the answers.
- 🔁 The for loop is used to repeat code a fixed number of times, while the while loop repeats code as long as a condition is true.
- 🎯 The example in the video demonstrates using control structures to solve a problem where Carol needs to clean up tennis balls in an unknown environment.
- 👣 A while loop is chosen for the solution because the size of the world is unknown, allowing Carol to move across the screen until the front is no longer clear.
- 🔄 Before moving, the program checks if Carol is standing on a ball and, if so, picks it up using an if statement.
- 🛑 The script identifies a common mistake in loops known as the 'fencepost problem', where the last case is often forgotten at the end of the loop.
- 🔄 To solve the fencepost problem, an additional check is made outside the loop to ensure the last ball is picked up after all moves are completed.
- 🌐 The solution is adaptable to different world sizes, demonstrating the flexibility of using control structures in various scenarios.
- 🔧 The video script emphasizes the importance of properly structuring loops and control structures to avoid logical errors.
- 📝 The example serves as a practical demonstration of how to use programming control structures to solve real-world problems efficiently.
Q & A
What are control structures in programming?
-Control structures are programming constructs that determine the order in which the program's instructions are executed. They include if statements, if-else statements, for loops, and while loops.
What is the purpose of an if statement in programming?
-An if statement is used to execute a block of code only if a specified condition is true.
How does an if-else statement differ from an if statement?
-An if-else statement includes an additional block of code that is executed if the condition in the if statement is false.
What is a for loop used for in programming?
-A for loop is used to repeat a block of code for a fixed number of times.
What is the main purpose of a while loop?
-A while loop is used to repeat a block of code as long as a specified condition remains true.
In the video script, what is Carol's task in the world?
-Carol's task is to go across the row and clean up all the tennis balls, ending up on the other side.
Why is a while loop chosen for Carol's task in the script?
-A while loop is chosen because the size of Carol's world is unknown, and it allows the program to continue moving until the condition 'front is clear' is no longer met.
What is the issue that arises when Carol tries to clean up the last tennis ball in the script?
-The issue is that the while loop does not execute the 'if ball is present' check after the last move, leaving one ball uncollected.
What is the solution to the problem of the last tennis ball not being collected?
-The solution is to perform an additional check for a ball outside of the while loop after all moves have been executed.
What is the fencepost problem mentioned in the script?
-The fencepost problem refers to the common mistake of forgetting to handle the last case in a loop, similar to how a fence requires one more post than the number of spaces between posts.
How can the fencepost problem be resolved in programming?
-The fencepost problem can be resolved by adding an extra case outside of the loop to handle the last item or by adjusting the loop's condition to include the final iteration.
Outlines
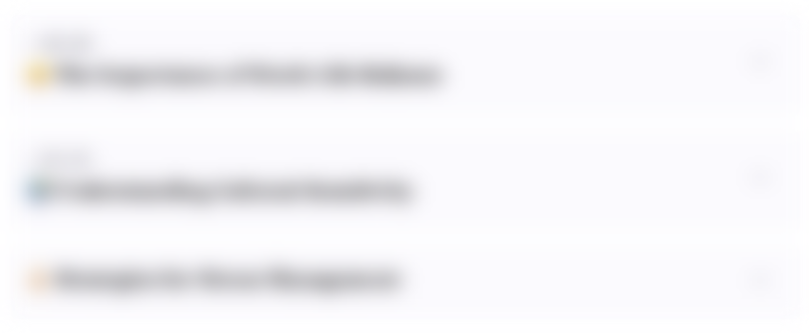
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
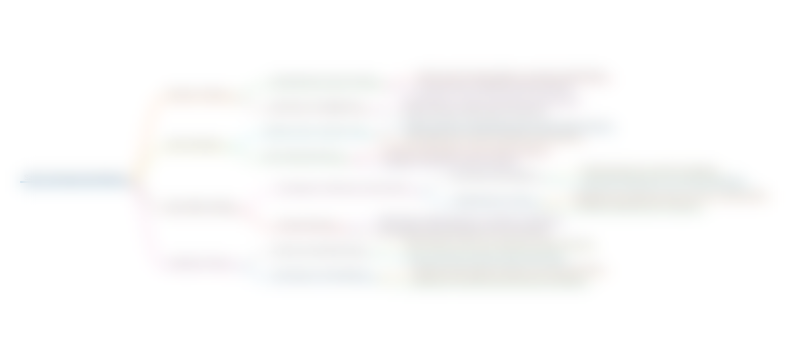
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
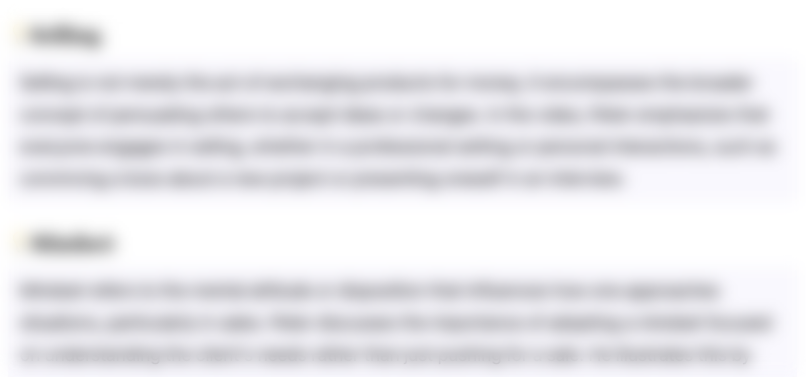
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
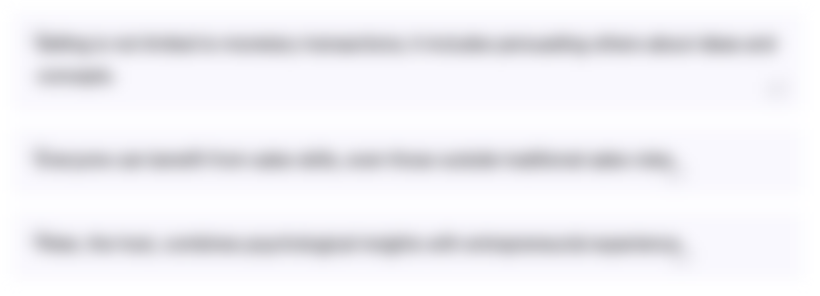
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
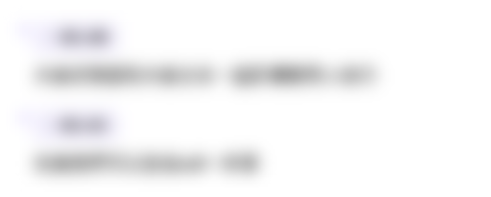
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)