Map, Filter & Reduce EXPLAINED in JavaScript - It's EASY!
Summary
TLDRIn this informative video, Dom introduces three essential JavaScript array methods: map, filter, and reduce. He demonstrates how map creates a new array by transforming each element, filter selectively includes elements based on a condition, and reduce consolidates the array into a single value. Using an array of person objects, Dom illustrates the practical applications of these methods, showing how they can be chained for complex operations and emphasizing their utility in web development.
Takeaways
- 🗺️ The video introduces three essential JavaScript array methods: map, filter, and reduce.
- 🔄 The map method transforms each item in an array into a new one based on the existing item, maintaining the array's original length.
- 🚫 The filter method allows for the creation of a new array with items that meet specific criteria, likely resulting in a shorter array.
- 🔢 The reduce method condenses an array into a single value, which is not an array but could be a number, string, or other data type.
- 👤 The examples in the video use an array of person objects with properties for name, age, and occupation.
- 📝 The map method is demonstrated by creating a new array containing only the names of the people from the original array.
- 🎯 The filter method is shown by filtering out individuals under the age of 30, resulting in an array of people over 30.
- 🔗 The video explains that the map and filter methods can be chained together to perform multiple transformations and filtering in sequence.
- 🧩 The reduce method is used to calculate the total age of all people in the array, demonstrating its capability to aggregate data.
- 📊 The reduce method's parameters include an accumulator (total) and the current array item (person), with the ability to set an initial value for the accumulator.
- 🌐 The video encourages viewers to subscribe for more web development tutorials and projects.
Q & A
What are the three JavaScript methods discussed in the script?
-The three JavaScript methods discussed in the script are map, filter, and reduce.
What does the map method do?
-The map method takes an array of items and converts each item into a new one based on the existing one, resulting in an array of the same length as the original.
How does the filter method differ from the map method?
-The filter method takes an array and removes items that do not meet a specified criteria, likely resulting in an array with a length shorter than the original.
What is the primary purpose of the reduce method?
-The reduce method is used to reduce an array to a single value, which is typically not an array but could be a number, string, or any other data type.
What is the purpose of the conversion function in the map method?
-The conversion function in the map method applies to every item in the array, allowing you to transform each item into a new form, such as extracting only the name from a person object.
Can you provide an example of how the filter method is used in the script?
-In the script, the filter method is used to create an array of people over the age of 30, excluding those who are younger.
What is the significance of chaining methods like map and filter together?
-Chaining methods allows for more complex data manipulations in a concise manner. For example, after filtering people over 30, you can chain a map method to extract their names.
How does the reduce method calculate the total age of all people in an array?
-The reduce method iteratively adds the age of each person to a running total, starting with an initial value (in this case, 0), and ultimately returns the sum of all ages.
What is the initial value set for the reduce method in the script?
-The initial value set for the reduce method in the script is 0, which is the starting point for the cumulative total of ages.
Can the reduce method return types other than numbers?
-Yes, the reduce method is not limited to numbers and can return any data type, such as arrays, strings, or booleans, depending on the logic implemented in the function.
Outlines
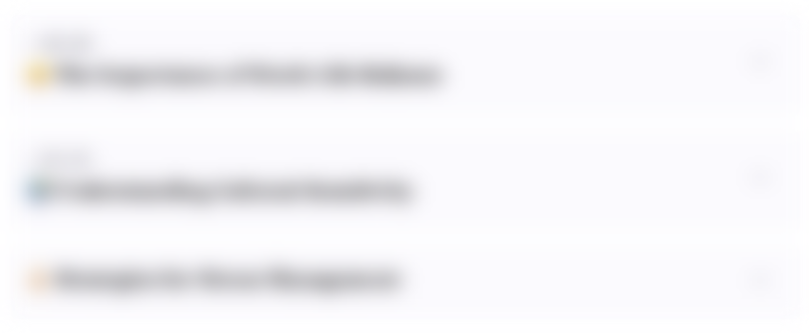
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
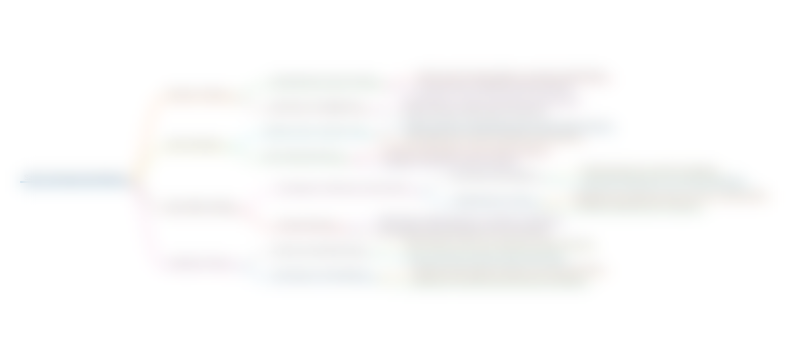
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
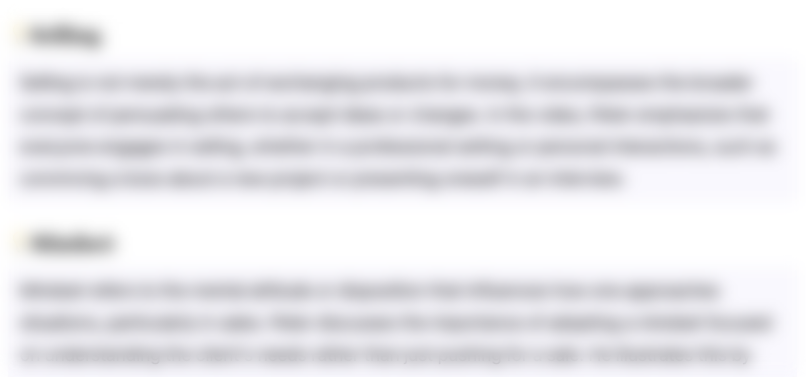
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
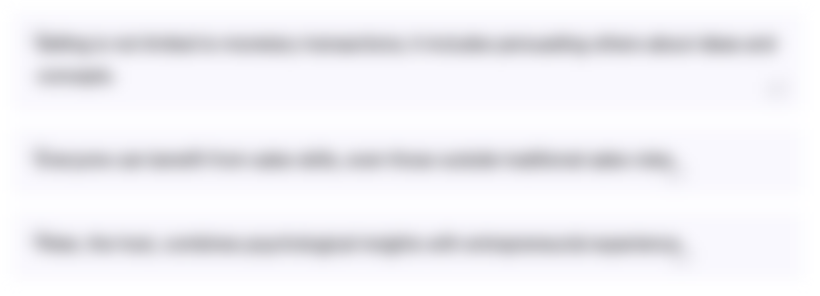
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
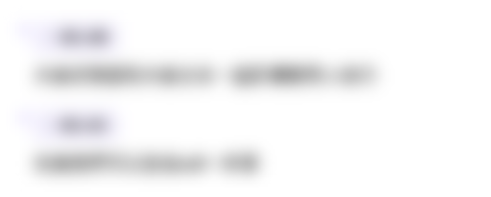
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

JavaScript forEach vs. map — When To Use Each and Why
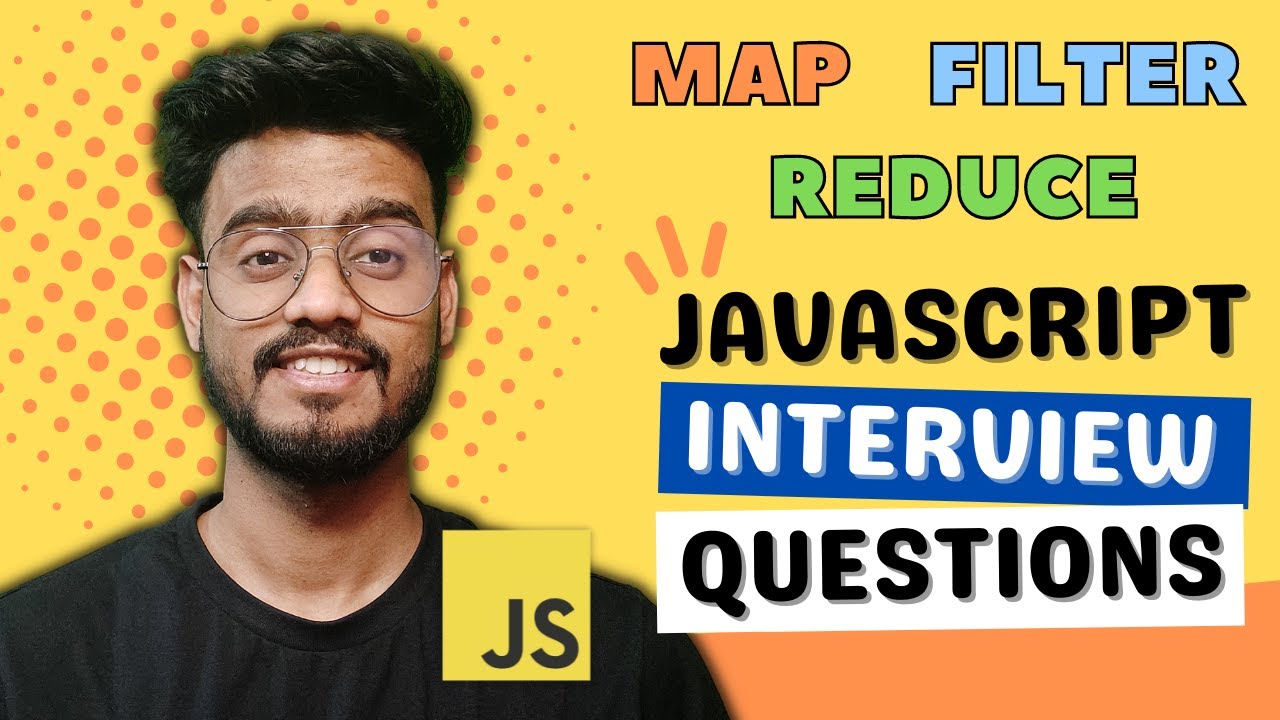
Javascript Interview Questions ( map, filter and reduce ) - Polyfills and Output Based Questions
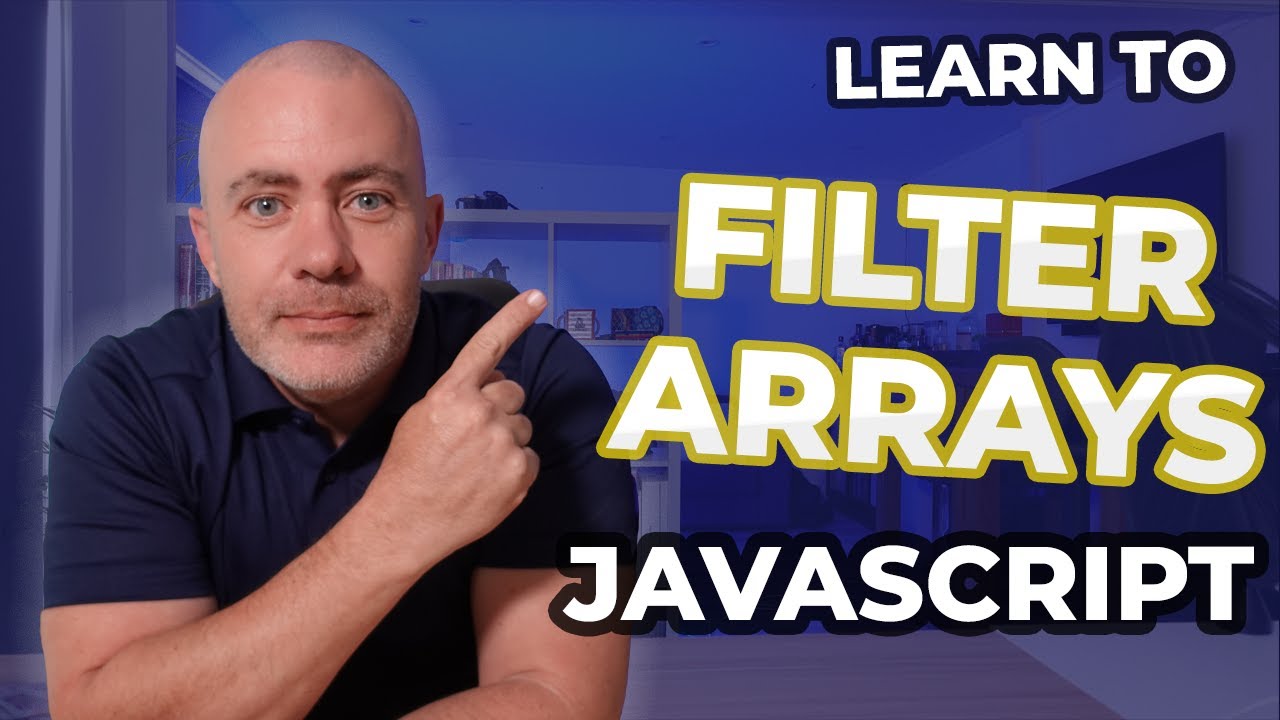
How to Filter Array of Objects in Javascript - ReactJS Edition
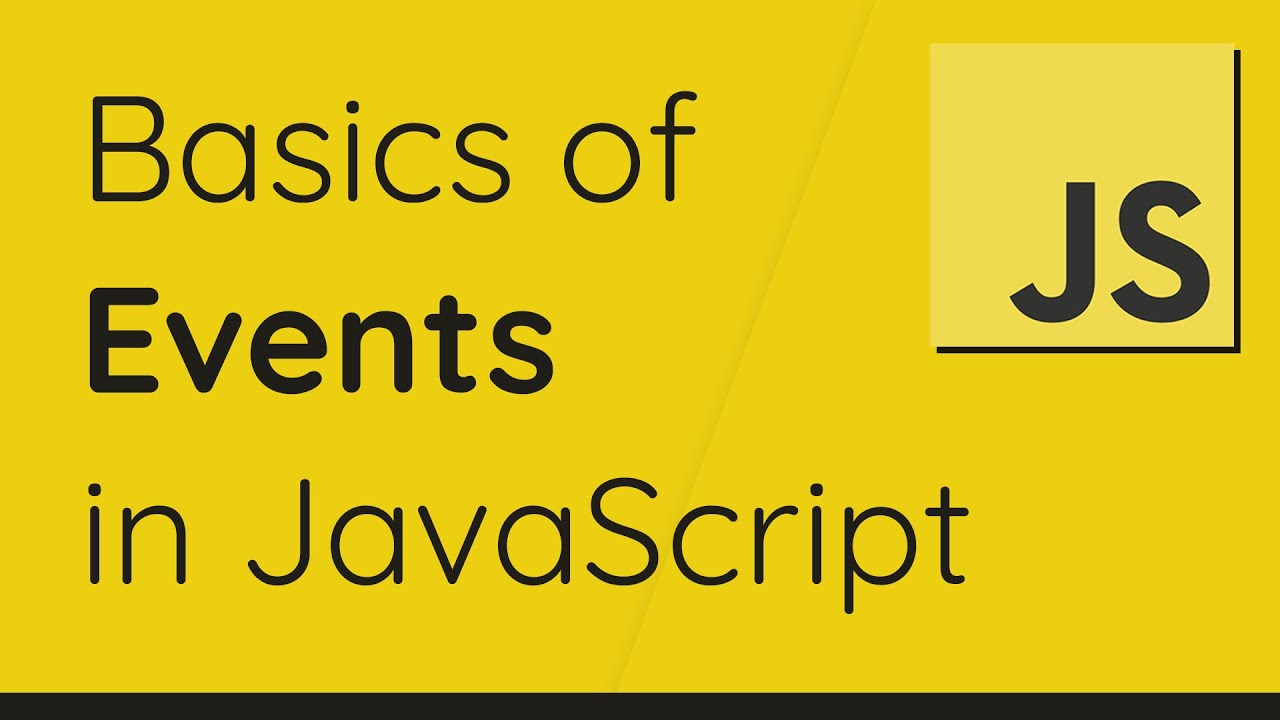
A Complete Overview of JavaScript Events - All You Need To Know
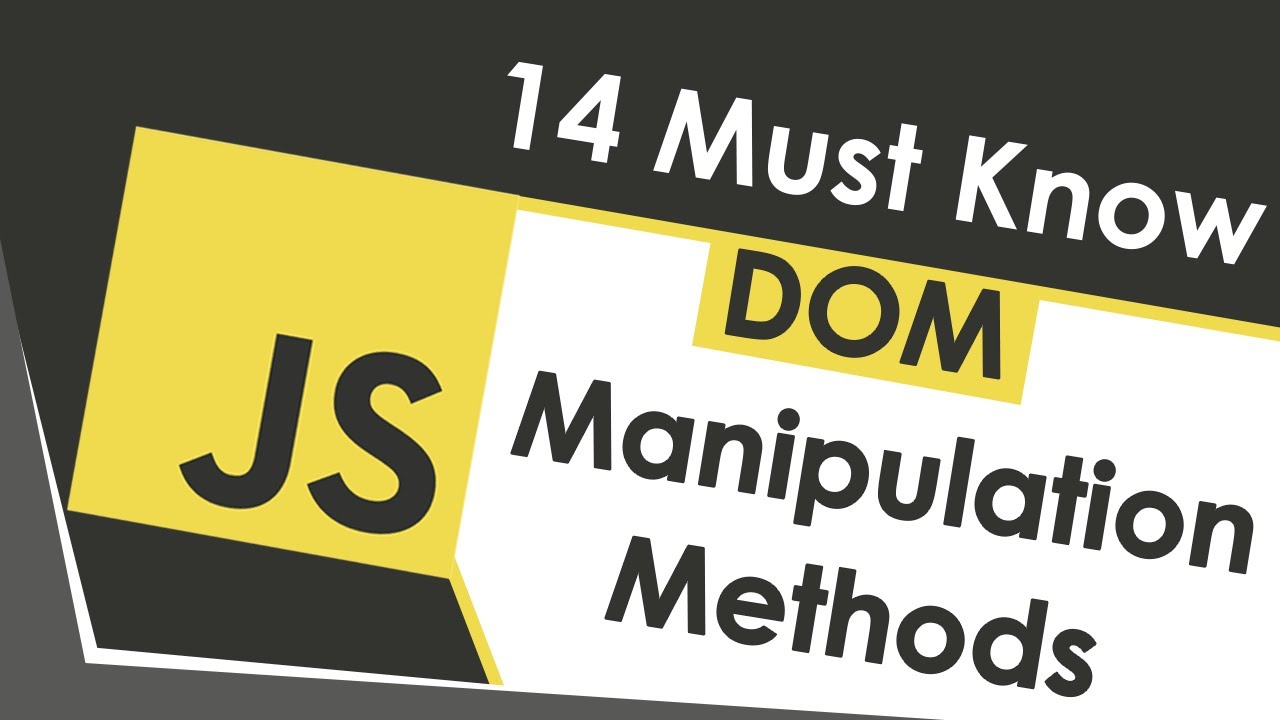
Learn DOM Manipulation In 18 Minutes

La diferencia de usar forEach() y map() en javascript #es6
5.0 / 5 (0 votes)