A Complete Overview of JavaScript Events - All You Need To Know
Summary
TLDRIn this informative video, Dom introduces the fundamentals of JavaScript events, ideal for beginners and those seeking a refresher. He covers HTML inline events, the crucial 'addEventListener' function, the JavaScript event object for capturing event details, and the distinction between standard functions and arrow functions in event handling. With practical examples, Dom demonstrates how to respond to user interactions like clicks and provides insights on when to use each function type for clear and effective coding practices.
Takeaways
- 😀 JavaScript events allow you to react to user interactions on a webpage, such as clicks or form submissions.
- 📌 The script introduces four main topics for understanding JavaScript events: HTML inline events, the 'addEventListener' function, the JavaScript event object, and the difference between standard and arrow functions in event handling.
- 🔗 HTML inline events are the simplest form of event handling but can be limiting due to the lack of complexity they can manage within the HTML attributes.
- 🔧 The 'addEventListener' method is a preferred approach for attaching events to elements because it keeps JavaScript separate from HTML, making the code cleaner and more manageable.
- 📚 The JavaScript event object provides detailed information about the event that occurred, such as mouse coordinates and whether modifier keys were pressed during the event.
- 👉 Inline event handlers can quickly become messy and are not recommended for complex projects, where separating JavaScript from HTML is advisable.
- 🛠️ Functions like 'onClick' and 'onMouseOver' can be attached to elements to define actions that should occur when specific events are triggered.
- 🔄 When using 'addEventListener', you can specify the event type and a function to be executed when the event occurs, promoting better organization of code.
- 🎯 The 'this' keyword behaves differently in standard functions and arrow functions within event handlers, with the former referring to the element that triggered the event and the latter to the global object.
- 👨🏫 The video script is aimed at beginners and those with some experience who want to review JavaScript events, emphasizing the importance of understanding the basics for effective web development.
- 🔍 The script suggests that developers should research and understand the various types of events available in JavaScript to effectively use them in their projects.
Q & A
What are JavaScript events, and why are they important?
-JavaScript events allow you to react to specific interactions or occurrences on a web page, such as clicks, form submissions, or mouse movements. They are important because they enable dynamic and interactive user experiences by triggering actions when certain events happen.
What is an inline HTML event in JavaScript, and when might you use it?
-An inline HTML event in JavaScript is an event handler directly placed within the HTML element, such as `onclick="alert('Hello!')"`. It's typically used in beginner tutorials for its simplicity but is not recommended for complex projects due to its limitations in maintainability and functionality.
What is the `addEventListener` function in JavaScript, and why is it preferred over inline events?
-The `addEventListener` function is used to attach an event handler to an element separately from the HTML markup. It is preferred over inline events because it keeps the HTML clean, allows multiple events to be attached to a single element, and provides more flexibility in handling events within the JavaScript code.
How does the `getElementById` function relate to event handling in JavaScript?
-The `getElementById` function is used to select an HTML element by its ID, allowing you to reference that element in your JavaScript code. Once selected, you can attach event handlers, such as using `addEventListener`, to respond to user interactions with that element.
What is the JavaScript event object, and what information does it provide?
-The JavaScript event object, often referred to as `e` or `event`, contains information about the event that just occurred, such as the type of event (e.g., click, mouseover), the position of the mouse, and whether modifier keys like Alt or Ctrl were pressed. This information can be used to make the event handling more dynamic and responsive to user actions.
Why might you use an external function for handling events in JavaScript?
-Using an external function for handling events in JavaScript, rather than defining the function inline, helps keep the code organized and reusable. It allows you to separate the logic from the event attachment, making the code easier to maintain, debug, and extend.
What is the difference between a standard JavaScript function and an arrow function in the context of event handling?
-The main difference between standard JavaScript functions and arrow functions in event handling is how they treat the `this` keyword. In standard functions, `this` refers to the element that triggered the event. In arrow functions, `this` is inherited from the surrounding context, often leading to it referring to the global window object instead of the element.
Why might the `this` keyword behave differently in arrow functions compared to standard functions?
-In arrow functions, the `this` keyword does not bind to the element that triggered the event but instead inherits `this` from the surrounding lexical context. This can lead to unexpected behavior, especially in event handlers, where you might expect `this` to refer to the element the event is bound to.
How can you log the event object to the console in JavaScript, and why would you do this?
-You can log the event object to the console using `console.log(e)` within the event handler function. This is useful for debugging and understanding the properties of the event, such as the mouse coordinates or whether modifier keys were pressed, allowing you to tailor your event handling logic accordingly.
What are some common use cases for the information provided by the event object in JavaScript?
-Common use cases for the event object include determining the position of the mouse during a click event, checking if modifier keys (like Ctrl or Alt) were held down, and accessing the target element that triggered the event. This information can be used to create more responsive and interactive user interfaces.
Outlines
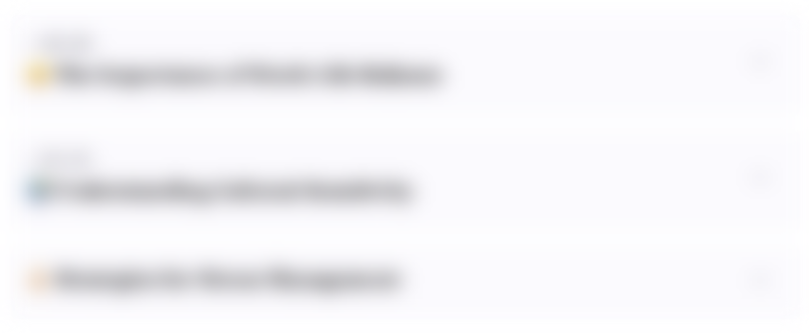
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
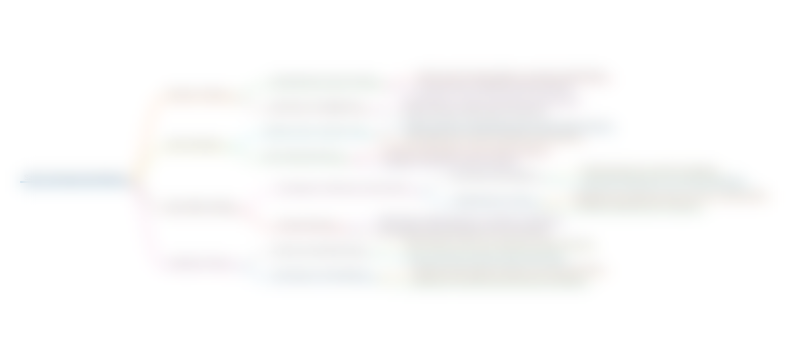
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
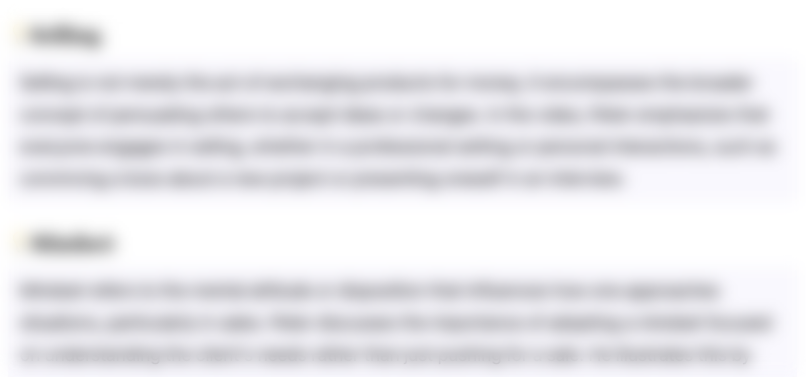
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
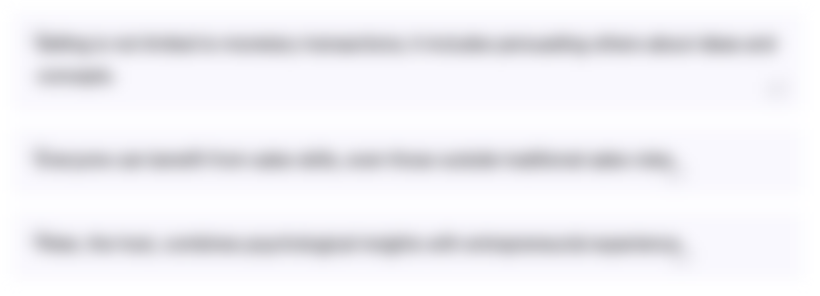
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
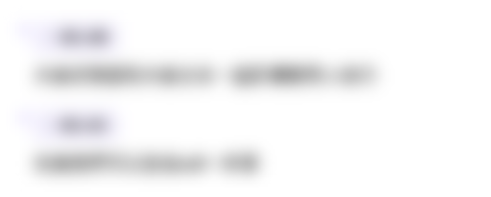
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
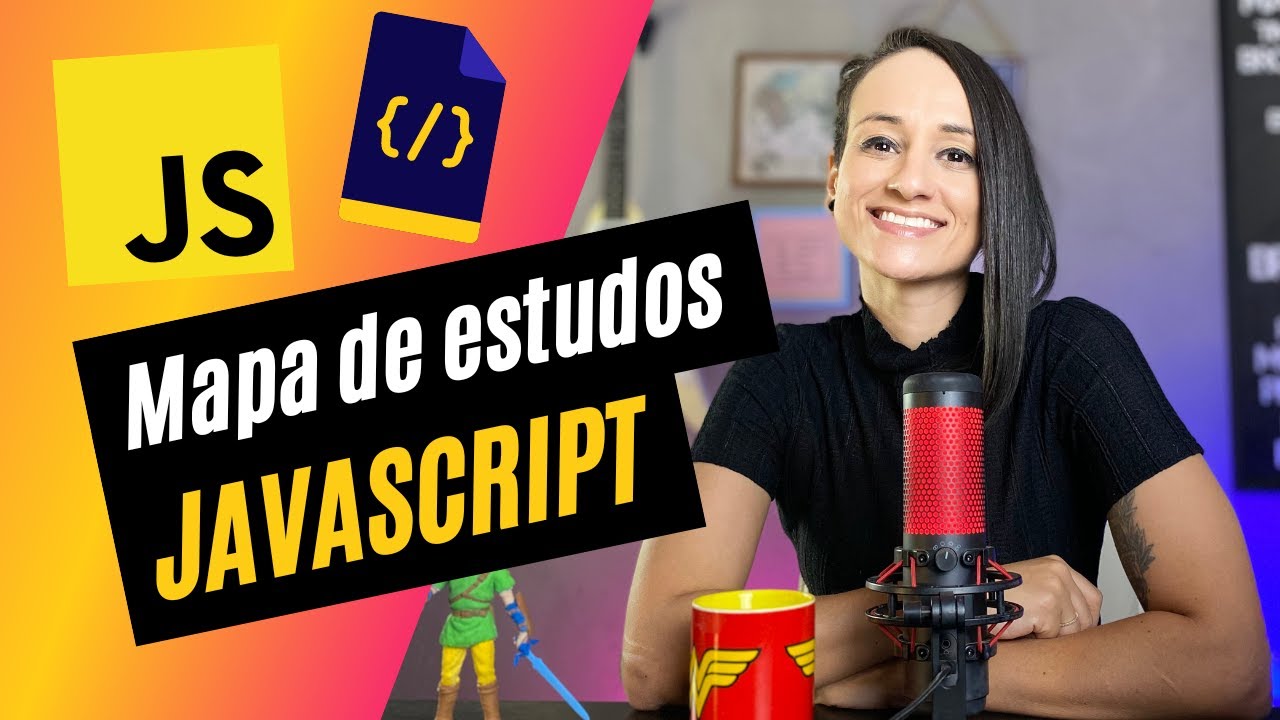
JAVASCRIPT do básico ao avançado ( Mapa de estudos / Roadmap )

NUTRITION 101 | Beginner's Guide to Healthy Eating
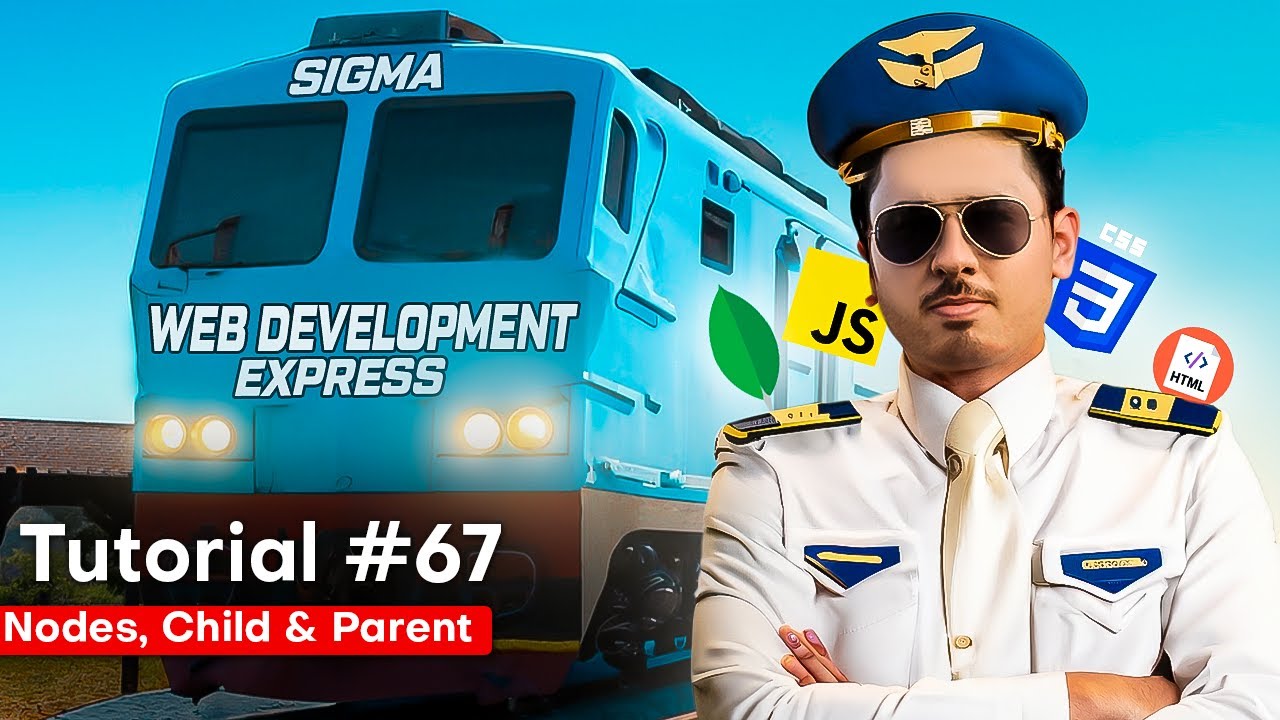
JavaScript DOM - Children, Parent & Sibling Nodes | Sigma Web Development Course - Tutorial #67
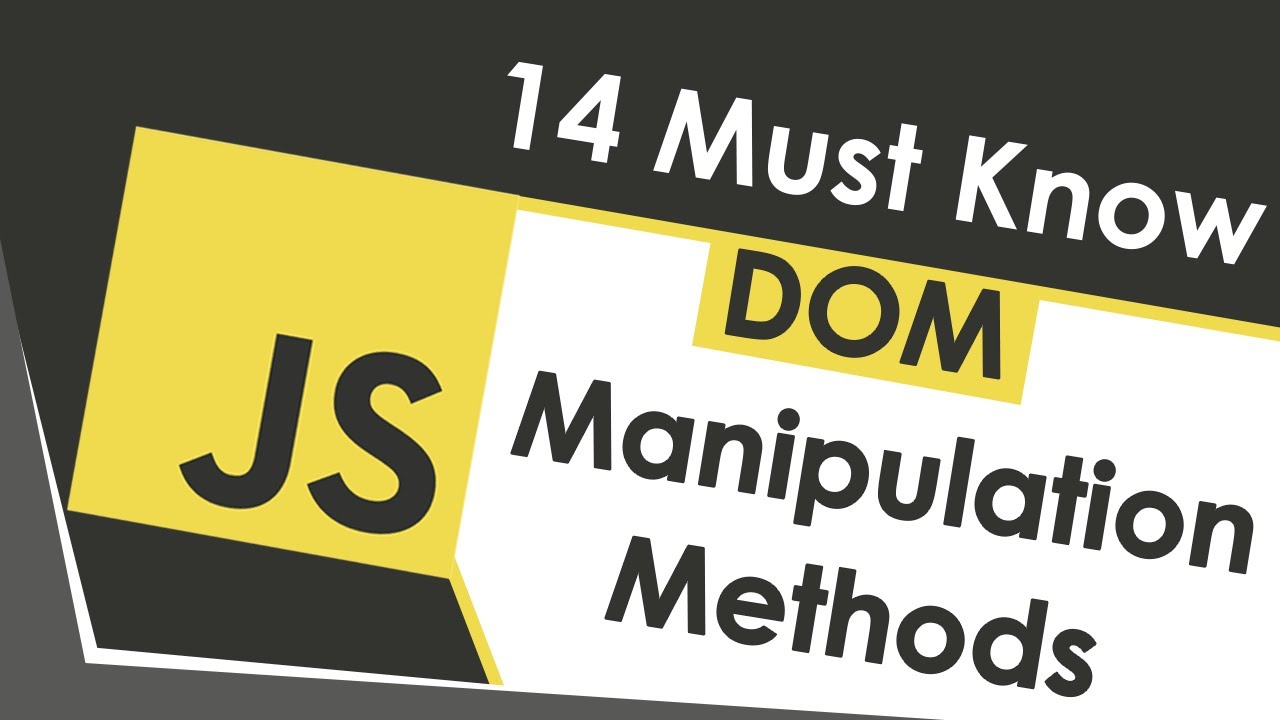
Learn DOM Manipulation In 18 Minutes

JavaScript forEach vs. map — When To Use Each and Why
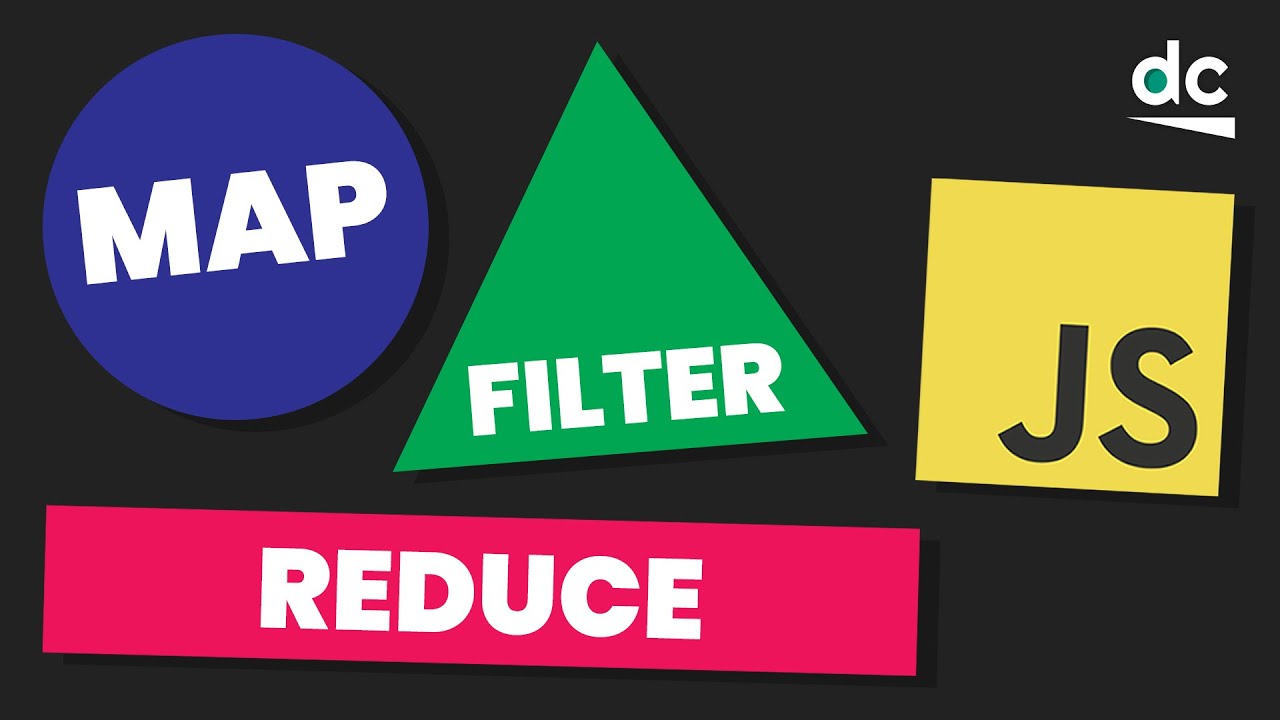
Map, Filter & Reduce EXPLAINED in JavaScript - It's EASY!
5.0 / 5 (0 votes)