Generics In Java - Full Simple Tutorial
Summary
TLDRIn this Java tutorial video, the presenter, John, a lead Java software engineer, dives into the concept of generics in Java. He explains that generics can be confusing at first glance due to the use of various symbols and conventions. However, he assures viewers that by the end of the video, they will understand what generics are, why they are essential, and how to implement them in their code. John begins by illustrating the problem generics solve, which is the issue of code duplication when creating classes that handle different data types. He then demonstrates how to create a generic class, using a 'Printer' class as an example, and how to specify the type of object the class will handle. The video also covers the use of generics with Java's Collections Framework, the importance of type safety, and advanced topics such as bounded generics, multiple bounds, generic methods, and wildcards. By the end, John has shown how generics allow for flexible and type-safe programming in Java, ultimately reducing code duplication and increasing maintainability.
Takeaways
- 📚 **Generics in Java**: Generics allow for the creation of classes, interfaces, and methods that can operate on any data type while providing compile-time type safety.
- 🔄 **Elimination of Code Duplication**: Before generics, developers had to create separate classes for each data type they wanted to handle, leading to code duplication. Generics solve this by allowing one class to handle multiple types.
- 🔑 **Type Parameters**: Classes and methods that use generics define a type parameter (commonly denoted as 'T') which stands for 'type' and can be used to specify the type of object the class or method will work with.
- 🚫 **Type Safety**: Generics enforce type safety, meaning that if you create a `Printer<Integer>`, you can't accidentally pass a `String` to it, which would cause a compile-time error.
- 🔄 **Wrapper Classes**: Generics do not work with primitive types like `int` or `long`. Instead, wrapper classes like `Integer` and `Long` are used to maintain type safety.
- 📦 **Collections Framework**: Java's Collections Framework widely uses generics, such as `ArrayList<Cat>`, which ensures that only objects of type `Cat` can be added to the list.
- 🚀 **Advanced Generics**: You can create bounded generics by specifying that the type parameter extends a particular class or implements an interface, allowing for more specific behavior.
- ➡️ **Multiple Bounds**: It's possible to specify that a type parameter extends multiple classes or interfaces, although only one class is allowed, followed by any number of interfaces.
- 📢 **Generic Methods**: Methods can also be generic, allowing them to work with different types, which can be specified when the method is called.
- 🔄 **Generic Wildcards**: Wildcards, denoted by a `?`, can be used when you want a generic type to accept any type, without knowing the exact type at compile time.
- 📋 **Bounded Wildcards**: Similar to bounded generics, bounded wildcards can specify that the unknown type must extend a particular class or implement an interface, providing a level of type safety.
Q & A
What is the main purpose of using generics in Java?
-Generics in Java are used to create classes, interfaces, and methods that can work with different types of objects without losing type safety. They help to avoid code duplication and ensure that the correct type of object is used throughout the program.
What problem did Java developers face before the introduction of generics?
-Before generics, developers had to create separate classes for different data types, which led to code duplication and maintenance issues. For example, if a class was designed to hold integers, a separate class would be needed to handle doubles or strings.
How does a generic class declaration look like in Java?
-A generic class in Java is declared by placing a type parameter in angle brackets after the class name and before the opening curly brace. By convention, this type parameter is often referred to as 'T', which stands for 'type'. For example: `public class Printer<T> { ... }`.
What is the issue with using raw types in Java?
-Raw types refer to using generic types without specifying the type parameter. This can lead to a loss of type safety, as the compiler cannot verify the type of objects being used with the generic class, potentially resulting in runtime errors like `ClassCastException`.
How do generics ensure type safety in Java?
-Generics ensure type safety by enforcing that only objects of the specified type can be used with a generic class or method. This prevents runtime errors that would occur if an object of an incompatible type were to be used.
What are bounded generics in Java?
-Bounded generics are a way to limit the type parameter to a specific class or interface. This is done by using the 'extends' keyword followed by the class or interface name. For example, `<T extends Animal>` ensures that T can only be a subclass of `Animal`.
How can you specify that a generic type must implement a certain interface?
-To specify that a generic type must implement a certain interface, you use the 'extends' keyword followed by the interface name within the type parameter. For example, `<T extends Serializable>` indicates that T must implement the `Serializable` interface.
What are wildcards in the context of Java generics?
-Wildcards in Java generics are used when you want to specify that a generic type can accept any type. They are represented by a question mark (?) and can be used to define a method or class that operates with any object type without specifying it.
How do you create a generic method in Java?
-A generic method in Java is created by declaring a type parameter within angle brackets in the method signature. For example: `public static <T> void shout(T thingToShout) { ... }`. This method can then be used with any type of object.
What is the limitation when using multiple bounds in generics?
-When using multiple bounds in generics, you can only have one class and it must be listed first, followed by interfaces (if any). Java does not support multiple inheritance, so the class name must precede any interface names in the bounds list.
Why can't a list of integers be considered a subtype of a list of objects in Java?
-In Java, the type system is invariant, meaning that even though `Integer` is a subclass of `Object`, a `List<Integer>` is not a subtype of `List<Object>`. This is because lists are covariant in their element types, and allowing such a subtype would break type safety.
Outlines
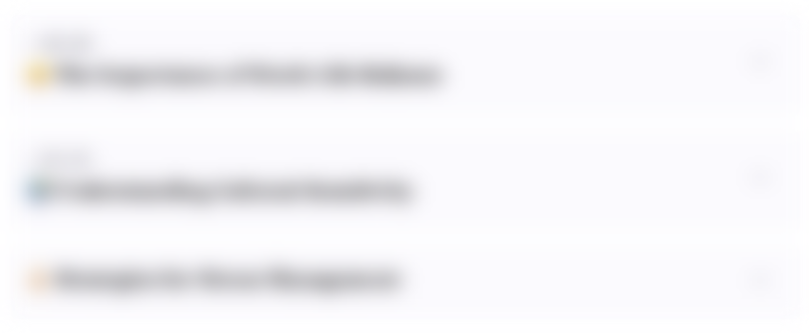
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
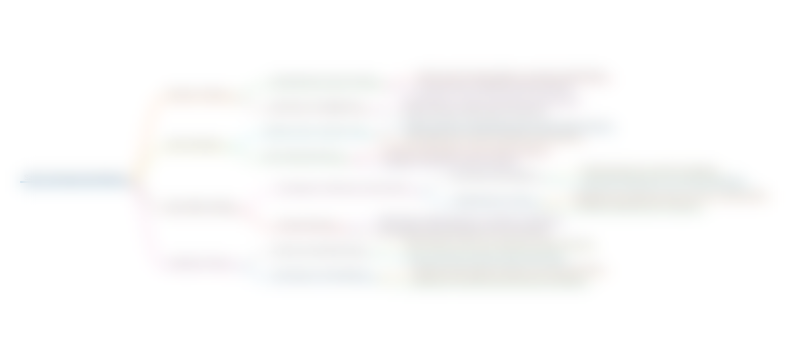
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
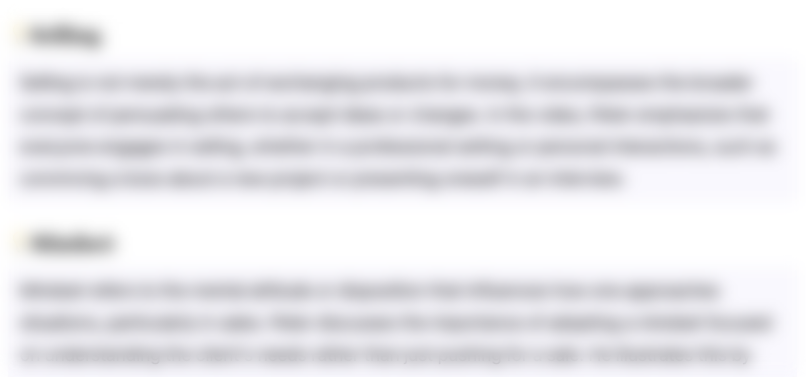
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
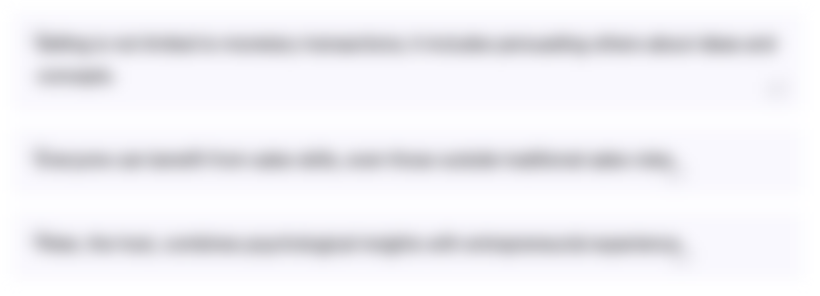
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
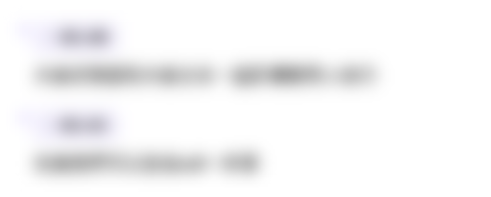
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)