Fuzzy Logic (2/7)
Summary
TLDRThis tutorial covers the implementation of a simple fuzzy system in Python. It begins by generating a random array of numbers and proceeds with classifying them into categories based on conditions (e.g., 'Lulus' for pass, 'Tidak Lulus' for fail). The script then removes duplicates, sorts the list, and selects two random values. The video demonstrates key concepts such as data classification, random number generation, sorting, and array manipulation, all while introducing fundamental principles of fuzzy logic in a simple yet practical way.
Takeaways
- 😀 Introduction to implementing simple fuzzy logic in Python using random numbers.
- 😀 Step 1: Importing necessary libraries such as `numpy` for array handling and `random` for number generation.
- 😀 Step 2: Generating random integers using `numpy.random.randint()`, specifying low, high, and size parameters.
- 😀 Step 3: Converting generated array into a list for easier manipulation and displaying its type.
- 😀 Step 4: Classifying numbers based on conditions: 'Fail' if less than 6, 'Pass' if 6 or above.
- 😀 Step 5: Displaying the index, value, and classification result for each number.
- 😀 Step 6: Sorting the list in ascending order and removing duplicates using `set()`.
- 😀 Step 7: Selecting two random numbers from the sorted list using `random.sample()` and displaying the results.
- 😀 Step 8: Demonstrating a simple fuzzy logic system with classification rules for 'Pass' and 'Fail'.
- 😀 Step 9: Potential extension of the example into more complex fuzzy systems with detailed classifications, e.g., 'Good Pass' or 'Poor Fail'.
- 😀 Conclusion: The script provides a basic framework for working with fuzzy logic in Python, with steps that can be expanded for more complex systems.
Q & A
What is the main goal of the tutorial presented in the video?
-The main goal of the tutorial is to demonstrate how to implement a simple fuzzy logic system in Python, including generating random data, classifying values based on specific conditions, removing duplicates, sorting, and selecting random elements.
Which Python libraries are used in the tutorial for data manipulation?
-The tutorial uses the `numpy` library for array manipulation and the `random` library for generating random numbers.
How is the random data generated in the tutorial?
-The random data is generated using the `numpy.random.randint` function, which creates an array of random integers between a specified lower and upper bound, and the size of the array is also defined.
What does the script do after generating the random data?
-After generating the random data, the script converts the data from a numpy array to a Python list using the `tolist()` function and prints the data type to confirm the conversion.
How are the values classified in the tutorial?
-Values are classified based on their size: values less than 6 are labeled as 'not passing,' and values 6 or greater are labeled as 'passing.' This classification follows a basic fuzzy logic approach where conditions are applied to categorize the data.
What happens if the data contains duplicate values?
-The script removes any duplicate values from the generated data by using the `set()` function, which automatically eliminates duplicates and then sorts the remaining values.
How does the script handle sorting the values?
-The script sorts the list of values using the `sorted()` function, which arranges the values in ascending order after removing duplicates.
How are random elements selected in the script?
-Two random elements are selected from the list using the `random.sample()` function, which allows for random sampling without repetition.
What happens to the elements that are not selected randomly?
-The elements that are not selected randomly are displayed separately, so the user can see which elements were picked and which were not.
How does this example relate to fuzzy logic systems in more complex scenarios?
-While the example in the tutorial is simple, the same basic concepts of classification, sorting, and random selection can be applied in more complex fuzzy logic systems where rules are more detailed, such as classifying based on multiple conditions or using more advanced fuzzy rules.
Outlines
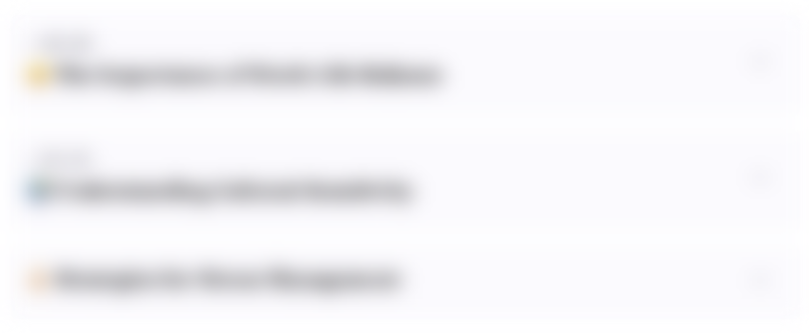
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
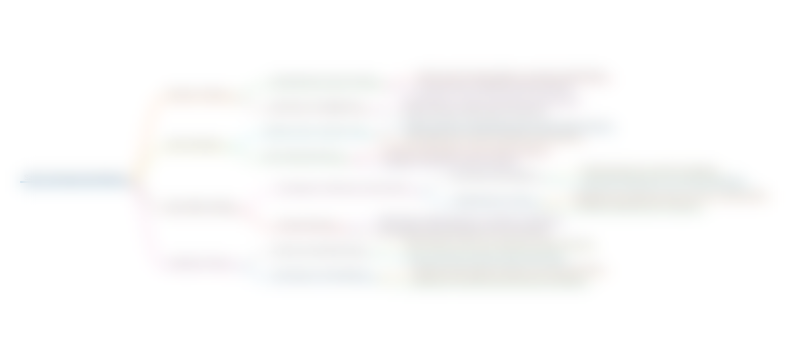
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
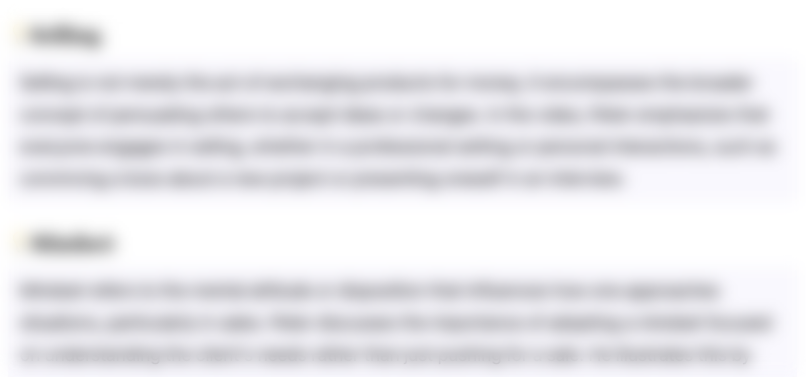
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
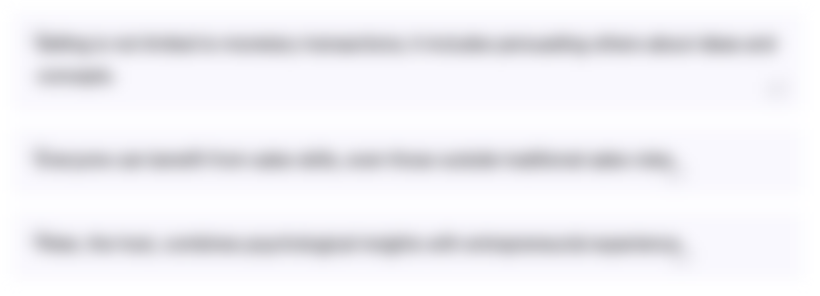
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
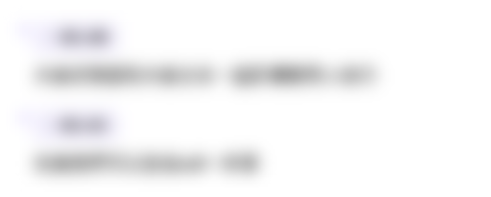
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
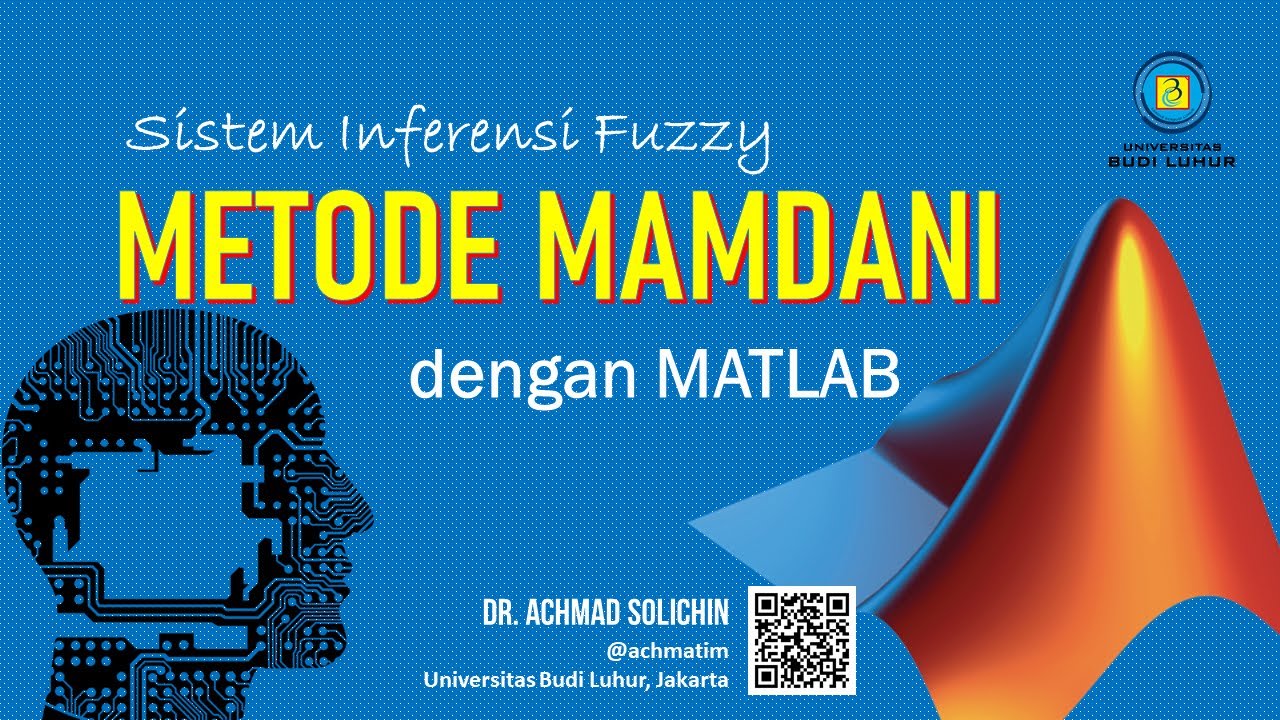
Logika Fuzzy MAMDANI dengan MATLAB | Fuzzy Logic Designer Toolbox
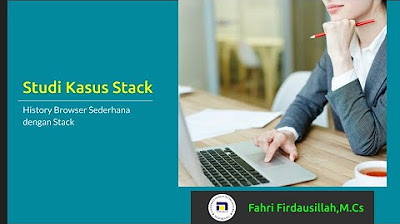
Studi Kasus Stack - Histori Browser
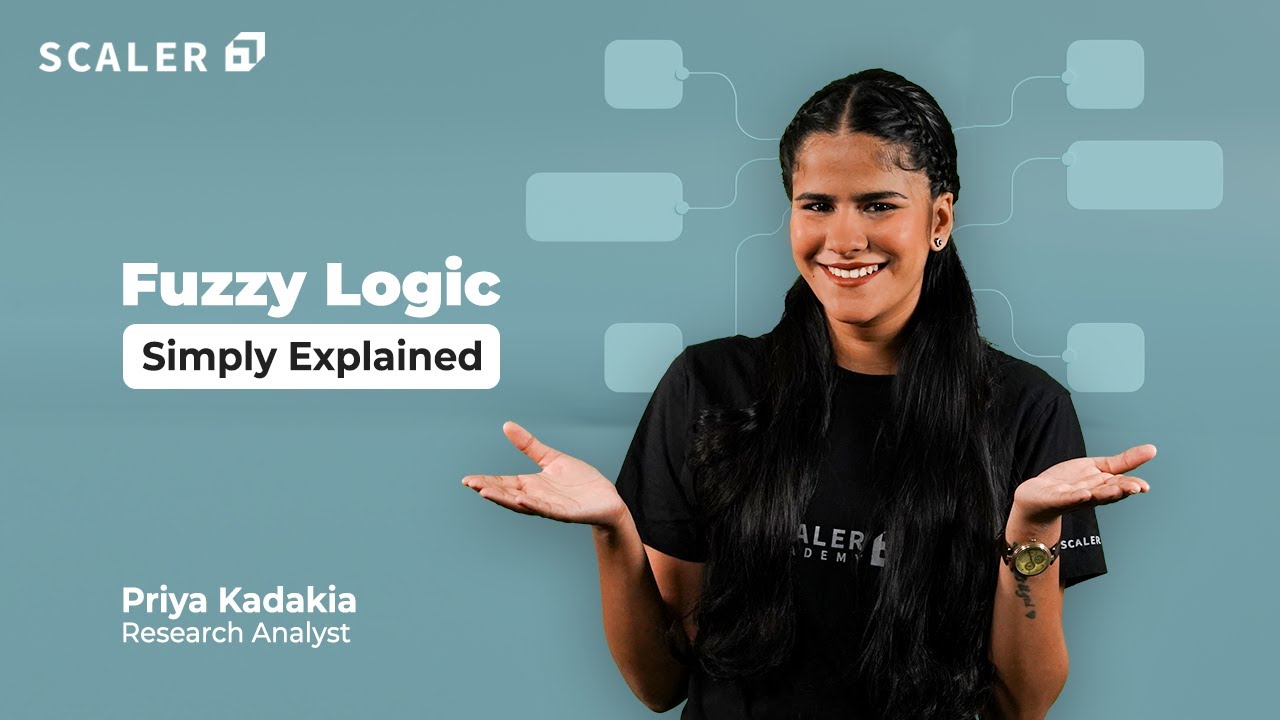
Fuzzy Logic in AI Explained for Beginners | Fuzzy Logic in Artificial Intelligence | Scaler
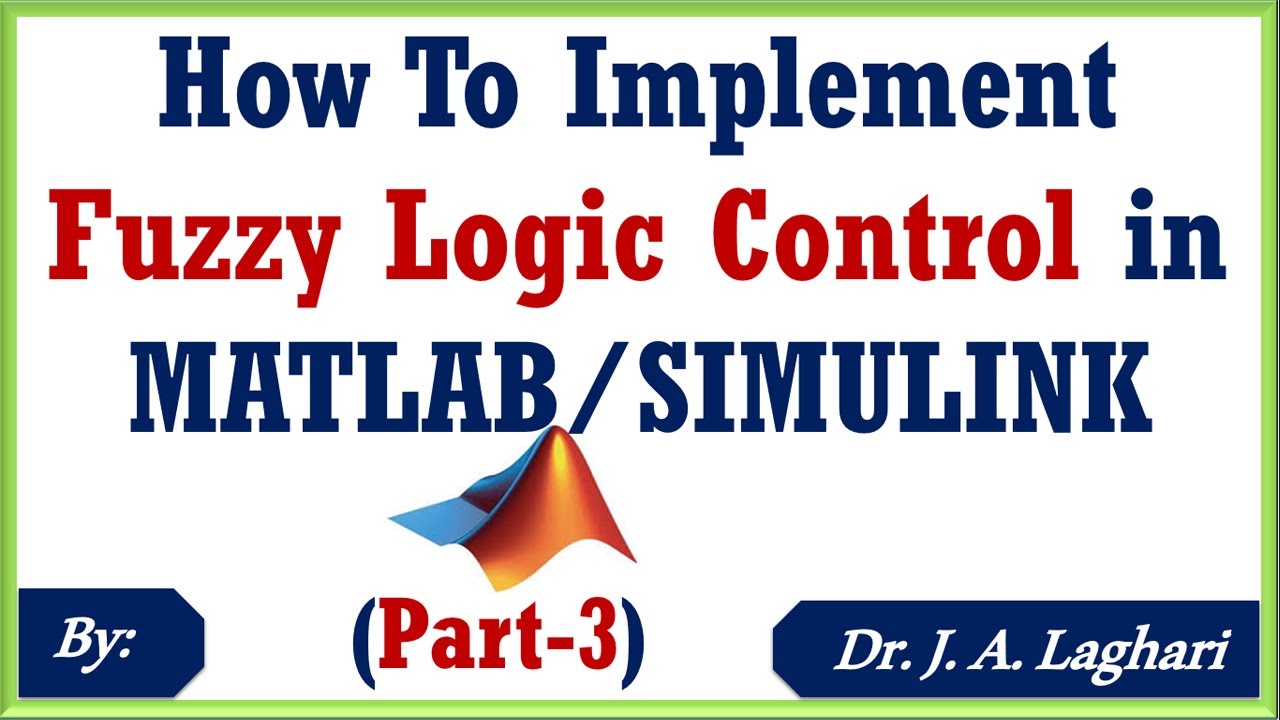
How To Implement Fuzzy Logic Control in MATLAB/SIMULINK ? (Part-3) | Dr. J. A. Laghari
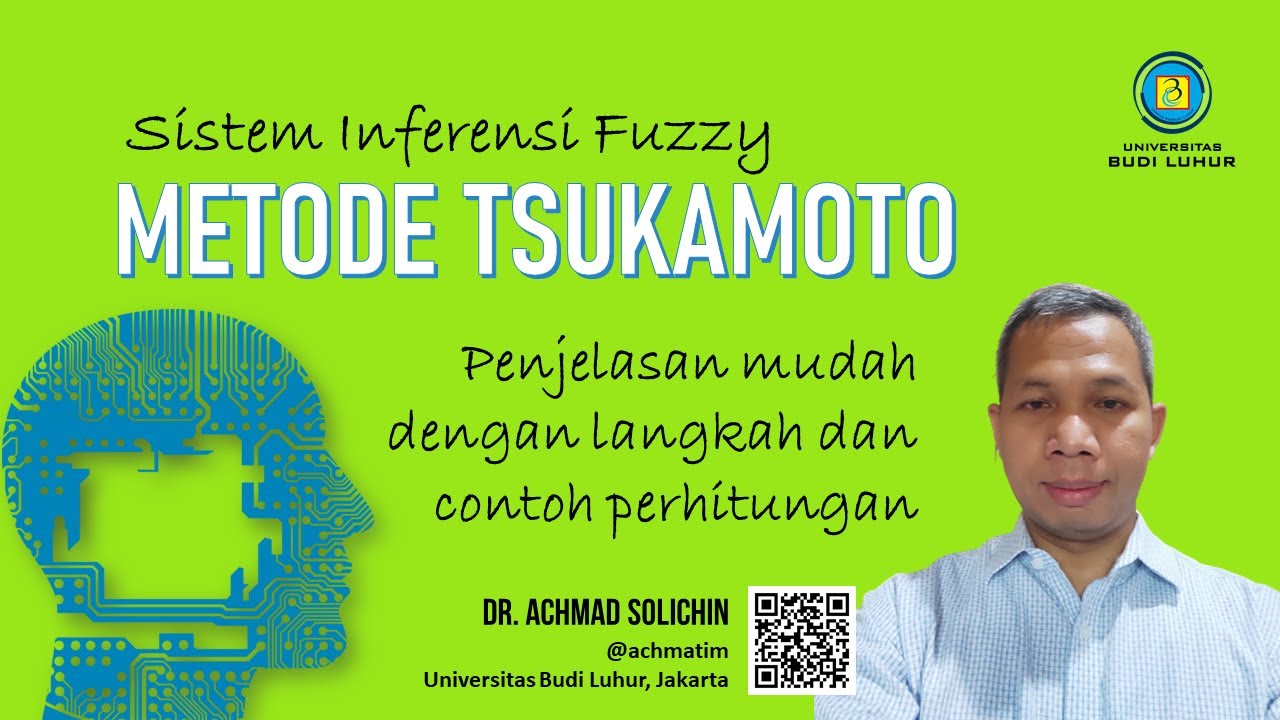
Fuzzy TSUKAMOTO | Sistem Inferensi Fuzzy | Contoh Studi Kasus dan Langkah Penyelesaiannya
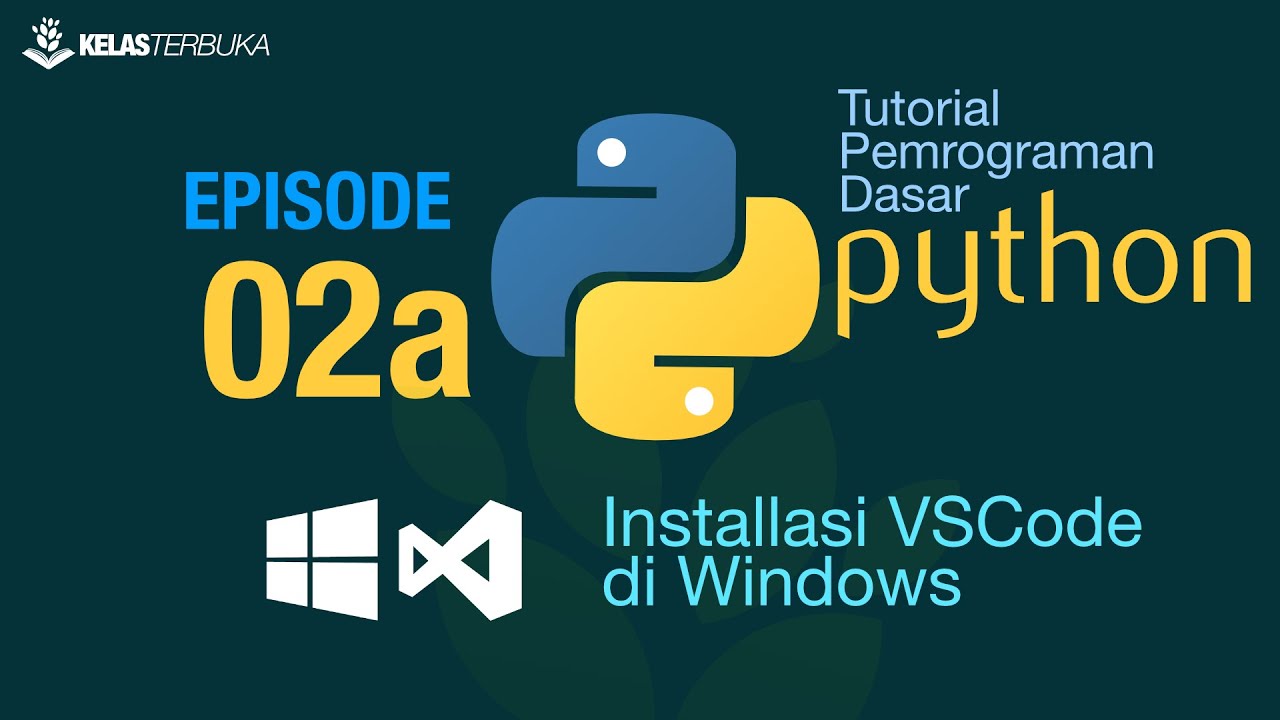
Belajar Python [Dasar] - 02a - Installasi Python dan VS Code di Windows
5.0 / 5 (0 votes)