Spring boot @Async Annotation - Part2 | Async Annotation Important Interview questions
Summary
TLDRIn this Concept and Coding video, Shan discusses the proper use of the @Async annotation in Spring Boot, emphasizing two key conditions: the method must be public and in a different class from the caller. He addresses common interview questions, such as how @Async interacts with transaction management and the challenges that arise, highlighting the importance of understanding transactional context when using async methods. Shan also covers @Async method return types, particularly the use of Future and CompletableFuture for handling method outputs and exceptions. Lastly, he explains how to handle exceptions in async methods, suggesting implementing a custom AsyncUncaughtExceptionHandler for better control over exception handling.
Takeaways
- 📌 The `@Async` annotation in Spring requires two conditions to work properly: the method must be in a different class from where it's called, and it must be a public method.
- 🔍 If the `@Async` method is in the same class as the caller, it won't execute in a separate thread as expected because AOP (Aspect-Oriented Programming) doesn't intercept calls within the same class.
- 🛠 AOP is used in the background for `@Async`, and it only intercepts public methods and calls between different classes.
- 🚫 Mixing `@Async` with `@Transactional` can lead to issues as the transaction context isn't propagated to the new thread created by `@Async`.
- ⚠️ When `@Transactional` is used within an `@Async` method, it starts a new transaction instead of using the existing one, leading to potential transaction management problems.
- 🔄 Use case 3 demonstrates the correct way to use `@Async` with `@Transactional`: run the transactional work in a separate thread while keeping the main thread free to continue processing.
- 📚 For understanding `@Async` method return types, refer to the Java playlist on Future, Callable, and Completable Future.
- 🔧 When using `@Async` with a return type, you can use `Future` or `CompletableFuture` to handle the result and exceptions.
- 💡 Exception handling in `@Async` methods that don't return anything can be managed by implementing a custom `AsyncUncaughtExceptionHandler`.
- 👨💻 Spring Boot provides a default `AsyncUncaughtExceptionHandler` that logs uncaught exceptions from `@Async` methods.
Q & A
What are the two conditions required for the @Async annotation to work properly?
-The two conditions required for the @Async annotation to work properly are: 1) The method with the @Async annotation should be in a different class from where it is being called. 2) The method should be a public method.
Why does the @Async annotation not work as expected when used within the same class?
-The @Async annotation does not work as expected when used within the same class because the AOP (Aspect-Oriented Programming) framework requires interception to work across different classes. Interception does not occur for private or protected methods, and it also doesn't work when a method is called within the same class from where it is defined.
How does the transactional context behave when an @Async method is called within a @Transactional method?
-The transactional context does not carry forward to a new thread created by an @Async method. This means that any transactional operations performed in the new thread will not be part of the transaction defined in the @Transactional method that invoked the @Async method.
What is the recommended way to use @Async with @Transactional annotations?
-The recommended way to use @Async with @Transactional annotations is to ensure that the @Transactional method is called in a separate thread initiated by @Async. This way, the main thread remains free to continue its work, and any transactional operations within the @Transactional method are handled in the new thread, with proper rollbacks in case of failures.
Why is it important to understand the return type of an @Async method?
-Understanding the return type of an @Async method is important because it determines how you can handle the result or exception from the asynchronous operation. Methods returning a Future or a CompletableFuture allow you to retrieve the result or handle exceptions using the Future's methods like get(), while methods returning void require different exception handling strategies.
How can you handle exceptions in an @Async method that returns void?
-Handling exceptions in an @Async method that returns void can be done by implementing a custom AsyncUncaughtExceptionHandler. You can create a class that implements the AsyncUncaughtExceptionHandler interface and override the handleUncaughtException method to define your custom exception handling logic.
What is the role of the AsyncUncaughtExceptionHandler in Spring Boot?
-The AsyncUncaughtExceptionHandler in Spring Boot is responsible for handling any uncaught exceptions thrown by @Async methods. By default, Spring Boot provides a SimpleAsyncUncaughtExceptionHandler that logs the exceptions. However, you can implement your own handler to customize the exception handling behavior.
How can you ensure that your custom AsyncUncaughtExceptionHandler is used in a Spring Boot application?
-To ensure that your custom AsyncUncaughtExceptionHandler is used, you need to create a configuration class annotated with @AsyncConfigurer and override the getAsyncUncaughtExceptionHandler method to return an instance of your custom handler. This configuration class should be included in your application context.
What is the difference between using Future and CompletableFuture in @Async methods?
-Both Future and CompletableFuture can be used to handle the result of an @Async method, but CompletableFuture is an enhanced version introduced in Java 8. It provides more functionality and is more commonly used in the industry. CompletableFuture supports asynchronous computation and allows for more complex operations like composition and transformation through its various methods.
Why is it important to understand the propagation levels of transactions when using @Async?
-Understanding the propagation levels of transactions is important when using @Async because it determines how transactions should behave when a new transaction is created or an existing one is used. This is particularly relevant when an @Async method is called within a @Transactional method, as the transactional context might not carry over to the new thread, affecting how rollbacks and commits are handled.
Outlines
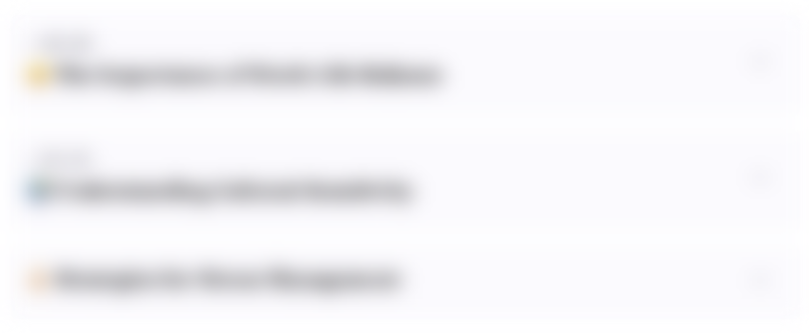
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
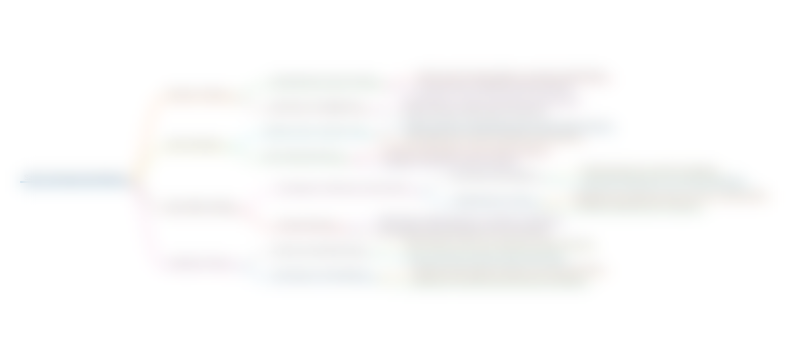
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
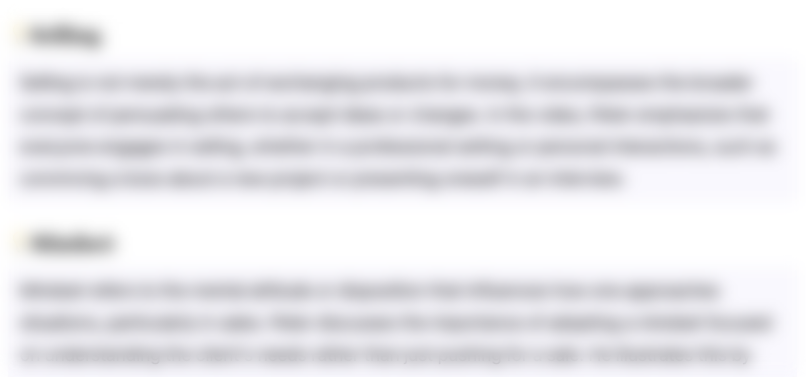
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
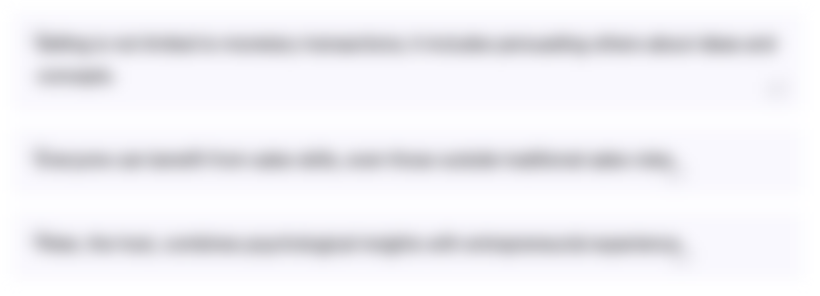
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
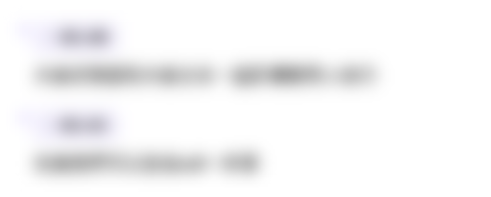
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)