Managing State With useReducer | Lecture 185 | React.JS 🔥
Summary
TLDRThe video script discusses the useReducer hook in React, which centralizes state updating logic in a reducer function, ideal for complex state management. It contrasts useReducer with useState, highlighting that useReducer allows for related state pieces to be managed together, resulting in cleaner, more readable components. The script explains that a reducer function takes the current state and an action to return the next state, emphasizing immutability and the lack of side effects. It also describes the dispatch function's role in triggering state updates based on actions. An analogy of withdrawing money from a bank illustrates the concept, clarifying the roles of dispatcher, reducer, action, and state in the useReducer mechanism.
Takeaways
- 🧠 UseReducer Hook: Centralizes state updating logic in a reducer function.
- 📦 Complex State Management: useReducer is ideal for managing complex state and related pieces of state.
- 🔄 useState Limitations: In complex scenarios with multiple state variables and updates, useState can become overwhelming.
- 🎯 Synchronous State Updates: useReducer allows for multiple state updates as a reaction to the same event.
- ⚙️ Reducer Function: A pure function that takes current state and action to return the next state without mutation.
- 🚫 Immutable State: React's state must not be mutated directly; reducers must return new state objects.
- 📜 Action Object: Describes state updates with an action type and payload, which is input data.
- 🔧 Dispatch Function: Triggers state updates by sending actions to the reducer from event handlers.
- 🔄 State Update Cycle: Dispatching an action leads to state computation by the reducer and subsequent component re-render.
- 💡 Reducer Analogy: Like the array reduce method, React reducers accumulate actions into a single state over time.
- 📈 useState vs useReducer: useState is simpler but useReducer solves complex state management problems and keeps components clean.
Q & A
What is the primary purpose of the useReducer hook?
-The useReducer hook is used to centralize state updating logic in a reducer function, making it an ideal solution for managing complex state and related pieces of state in a React application.
Why might using useState be insufficient for managing state in certain situations?
-In situations where components have many state variables and updates spread across multiple event handlers, or when multiple state updates need to occur simultaneously, using useState can become overwhelming and difficult to manage.
How does useReducer help with the challenges of managing complex state?
-useReducer allows for the decoupling of state logic from the component itself by moving all state updating logic into a single reducer function, resulting in cleaner and more readable components.
What does a reducer function typically take as inputs?
-A reducer function typically takes the current state and an action as inputs, and based on these values, it returns the next state (the updated state).
What is the significance of immutability in the context of React state management?
-In React, state is immutable, meaning the reducer function is not allowed to mutate the state directly. Instead, it must always return a new state object based on the current state and the received action.
What is the role of the dispatch function in useReducer?
-The dispatch function, returned by useReducer, is used to trigger state updates by sending an action to the reducer, which then computes the next state based on the current state and the action.
How does the useReducer mechanism differ from useState in terms of updating state?
-With useState, you directly call setState with the new state value to update the state, whereas with useReducer, you use the dispatch function to send an action to the reducer, which then determines how to update the state.
What is the analogy used in the script to help understand the useReducer mechanism?
-The analogy used is that of withdrawing money from a bank. The customer (dispatcher) goes to the bank (reducer) to request money (state update), and the bank employee (reducer function) handles the withdrawal from the vault (state object) based on the customer's request (action).
What does the action object represent in the useReducer mechanism?
-The action object represents a request to update the state. It usually contains an action type and a payload, which provides the necessary information for the reducer to determine how to update the state.
How does the useReducer hook manage related pieces of state?
-useReducer manages related pieces of state by storing them in a state object that is returned from the hook. This allows for a more organized and efficient way of handling complex state updates.
What is the benefit of using useReducer over useState in complex scenarios?
-useReducer provides a more structured and scalable approach to state management, especially for complex scenarios with multiple related state variables and updates. It helps maintain a clear separation of concerns, making the codebase easier to understand and maintain.
Outlines
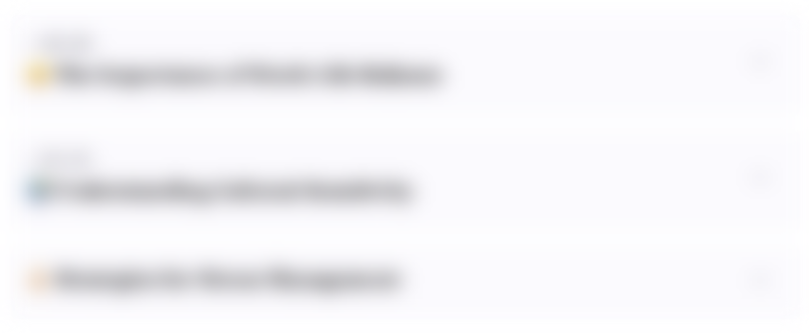
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
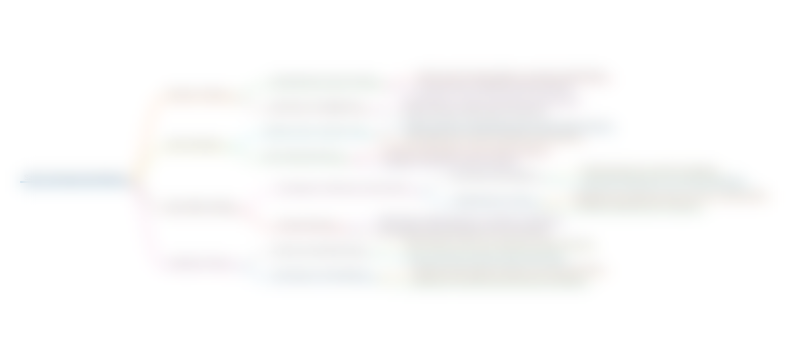
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
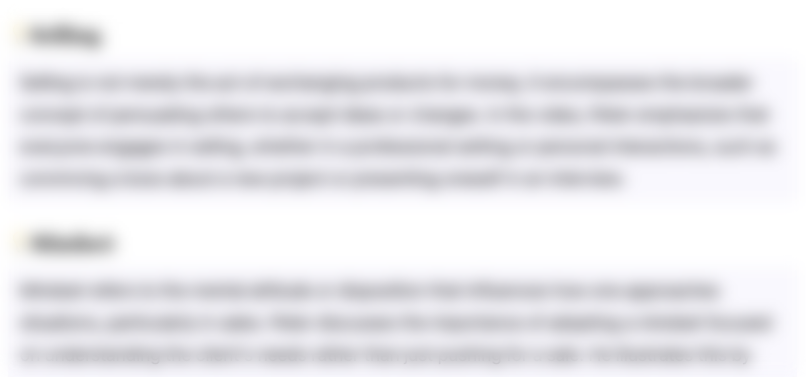
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
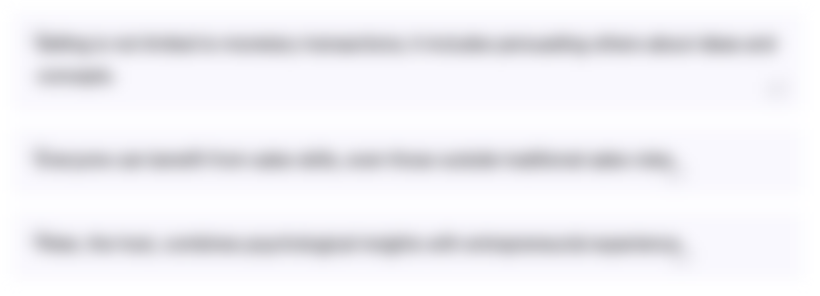
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
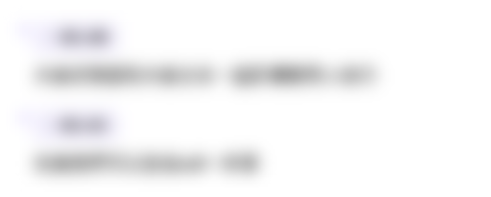
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Introduction to Redux | Lecture 257 | React.JS 🔥

How NOT to Fetch Data in React | Lecture 139 | React.JS 🔥
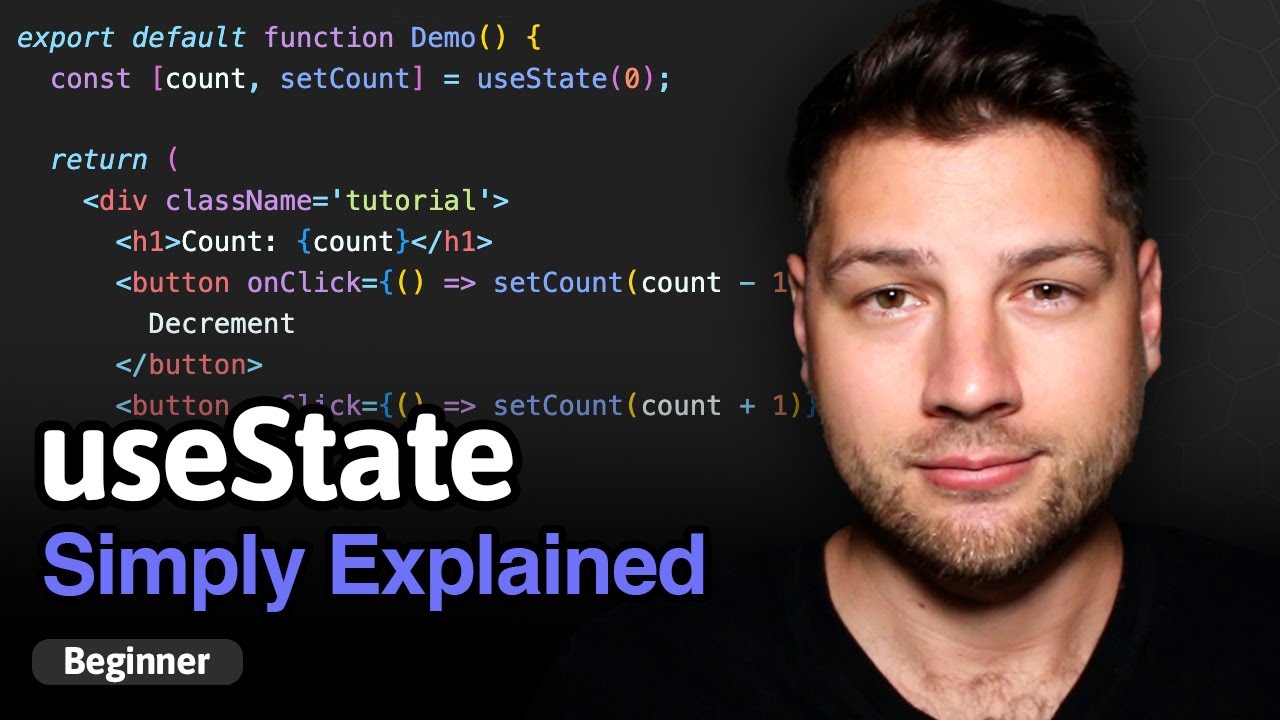
Learn React Hooks: useState - Simply Explained!

useState Hook | Mastering React: An In-Depth Zero to Hero Video Series

useEffect to the Rescue | Lecture 140 | React.JS 🔥

Rules for Render Logic: Pure Components | Lecture 131 | React.JS 🔥
5.0 / 5 (0 votes)