C# objects as arguments 🧳
Summary
TLDRThis video tutorial demonstrates how to pass objects as arguments in programming. It uses a 'Car' class example with 'model' and 'color' properties. The instructor shows how to create a method to change a car's color by passing a car object and a new color. Additionally, it covers creating a method to copy a car object, returning a new car with the same properties. The video is aimed at helping viewers understand object passing and returning in methods, and encourages engagement through likes and subscriptions.
Takeaways
- 🚗 The video demonstrates how to pass an object as an argument in a method.
- 🔑 A class 'Car' is introduced with two fields: 'model' and 'color', and a constructor to assign values to these fields.
- 🎨 A method 'changeColor' is created to alter the color of a 'Car' object, requiring the object and a new color as parameters.
- 🔄 The method 'changeColor' is static, void, and uses direct object field manipulation to change the car's color.
- 📝 The script shows how to invoke the 'changeColor' method by passing a 'Car' object and a new color string.
- 🔍 The video explains the importance of matching the parameter type with the object being passed.
- 🔄 Another method 'copy' is introduced to return a new 'Car' object, demonstrating how to return objects from methods.
- 🆕 The 'copy' method returns a new 'Car' object by utilizing the passed 'Car' object's properties to create a duplicate.
- 💾 The script illustrates object copying by invoking the 'copy' method and passing an existing 'Car' object as an argument.
- 📢 The video concludes with a call to action for viewers to like, comment, and subscribe for more content.
Q & A
What is the purpose of the 'changeColor' method in the video?
-The 'changeColor' method is used to alter the color of a car object by accepting a car object and a new color as parameters.
How many fields does the 'Car' class have according to the video?
-The 'Car' class has two fields: 'model' and 'color'.
What is the data type of the 'color' field in the 'Car' class?
-The 'color' field in the 'Car' class is of the data type 'string'.
What is the name of the method created to copy a car object?
-The name of the method created to copy a car object is 'copy'.
How does the 'copy' method return a new 'Car' object?
-The 'copy' method returns a new 'Car' object by creating a new instance of 'Car' using the 'model' and 'color' from the passed car object.
What is the signature of the 'changeColor' method as described in the video?
-The 'changeColor' method signature is 'public static void changeColor(Car car, string color)'.
What is the signature of the 'copy' method as described in the video?
-The 'copy' method signature is 'public static Car copy(Car car)'.
How does the video demonstrate changing the color of 'car1'?
-The video demonstrates changing the color of 'car1' by invoking the 'changeColor' method with 'car1' and the new color 'silver' as arguments.
What is the output of the console statement after changing the color of 'car1'?
-The output of the console statement after changing the color of 'car1' is 'Silver Mustang'.
How does the video explain passing an object as an argument?
-The video explains that to pass an object as an argument, you need to have the correct parameter setup, which includes the data type of the object followed by a name for the parameter.
What is the significance of the 'car.color = color' line in the 'changeColor' method?
-The 'car.color = color' line in the 'changeColor' method assigns the new color to the 'color' field of the passed 'car' object.
Outlines
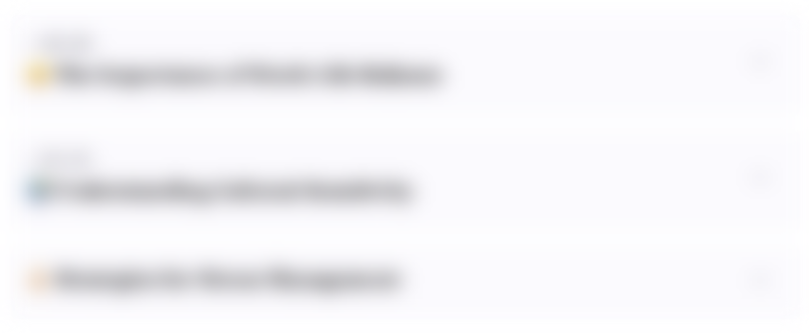
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
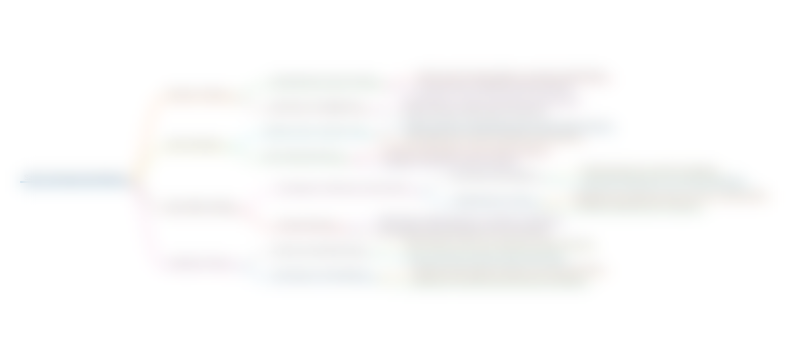
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
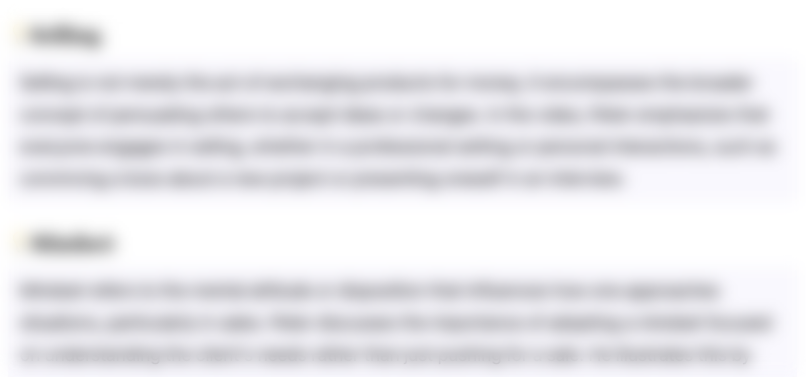
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
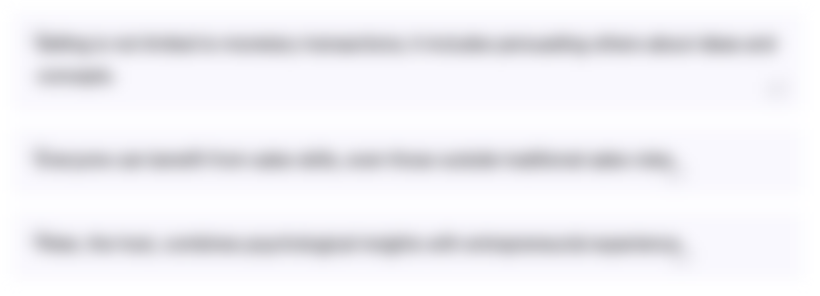
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
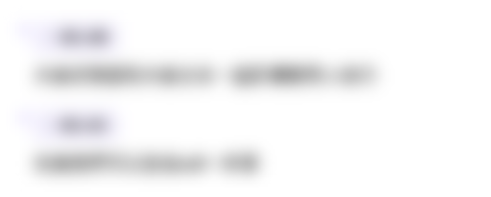
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
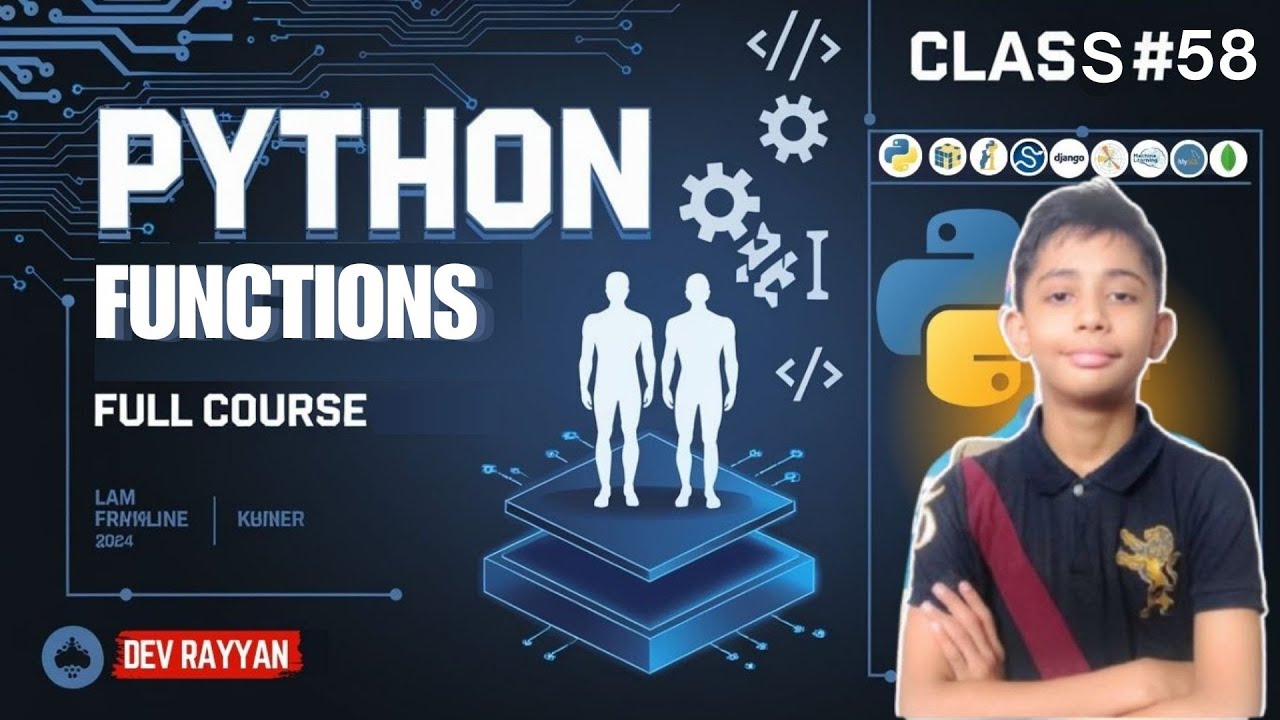
PYTHON FUNCTIONS | Python Tutorial - Lesson #58
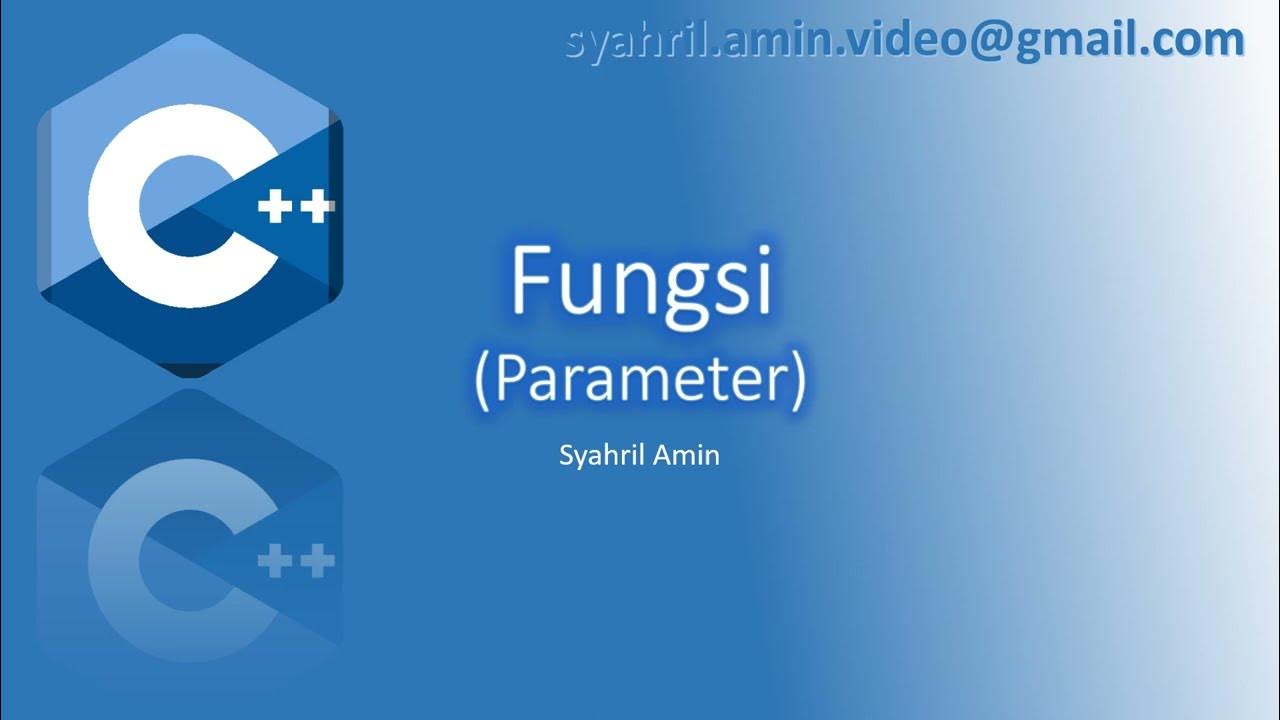
Fungsi (Parameter)

Class Objects & Constructors | Godot GDScript Tutorial | Ep 16
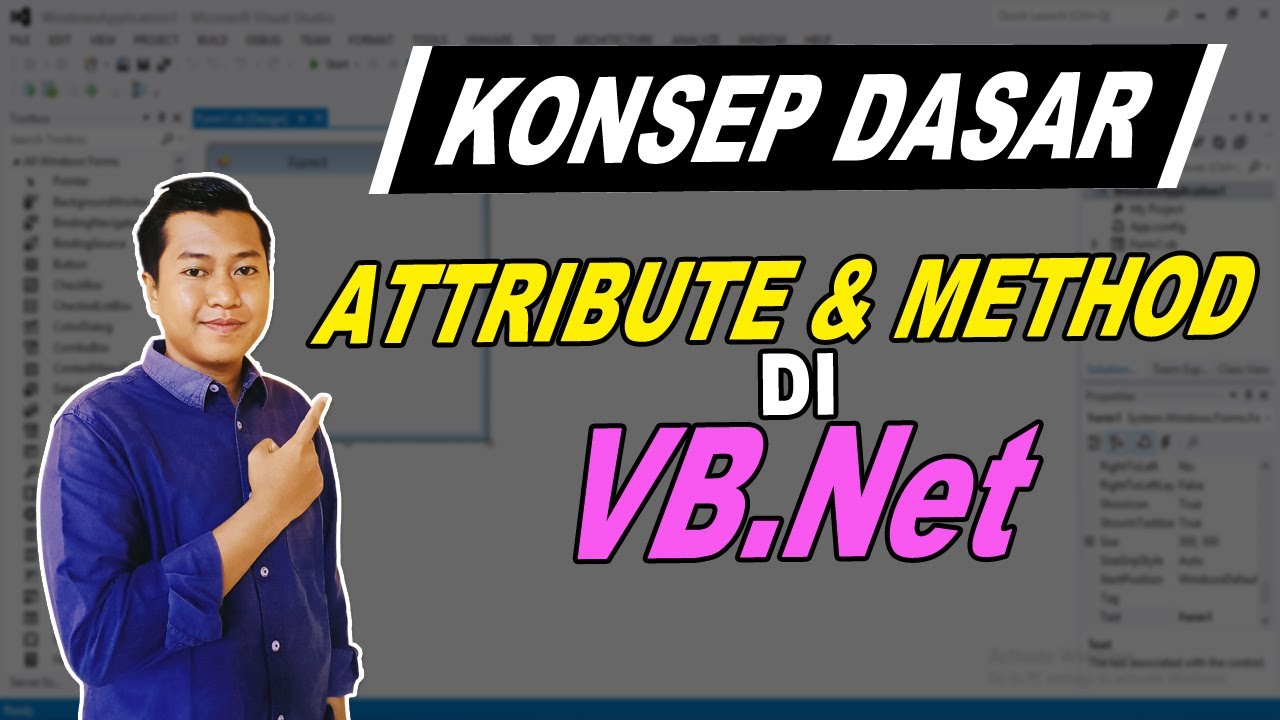
Memahami Konsep OOP di VB.Net: Penjelasan dan Contoh Attribute / Property & Method / Behavior
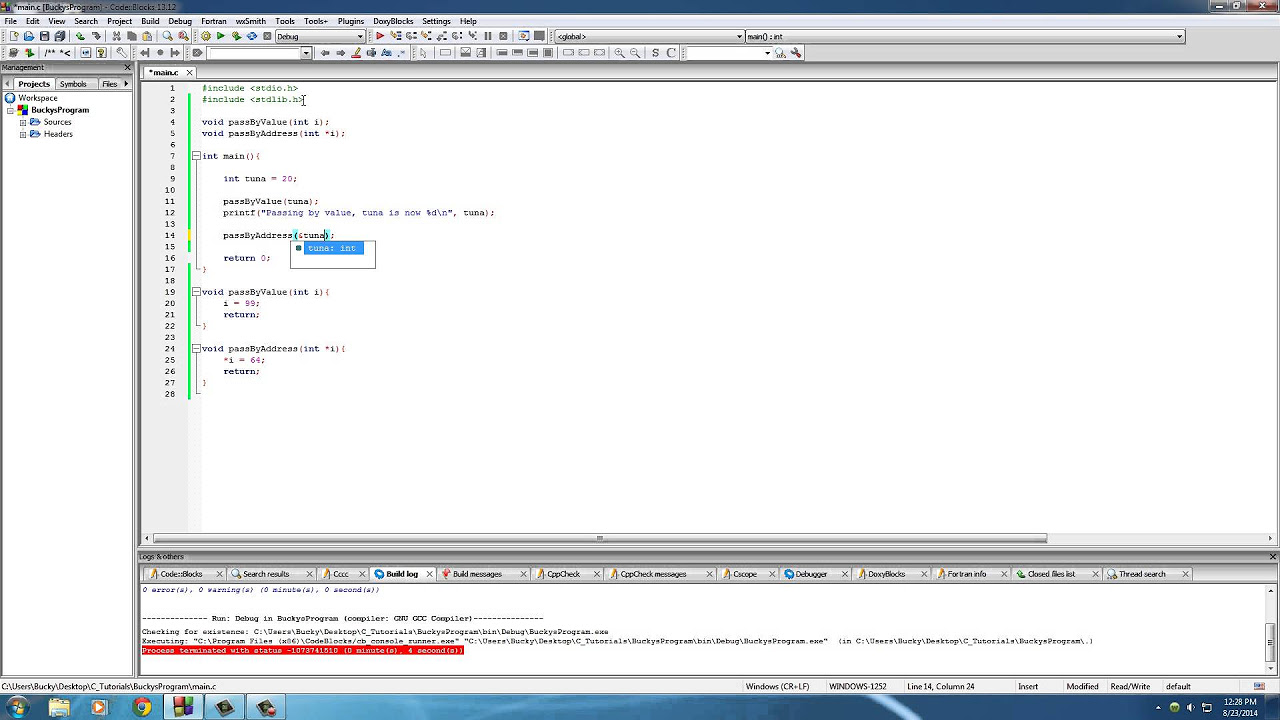
C Programming Tutorial - 58 - Pass by Reference vs Pass by Value

6. Explicit Intent With Data
5.0 / 5 (0 votes)