C Programming Tutorial - 58 - Pass by Reference vs Pass by Value
Summary
TLDRIn this concluding tutorial on C programming, the instructor explains the critical concepts of passing variables to functions: pass by value and pass by address. Using clear examples, the video illustrates how pass by value creates a copy of the variable, leaving the original unchanged, while pass by address allows direct manipulation of the variable's value in memory. The instructor emphasizes the importance of understanding these methods for effective programming and encourages viewers to practice these concepts for mastery. With a friendly tone, he invites users to subscribe for future Python programming tutorials.
Takeaways
- 🎉 The tutorial is the final installment in a C programming series, emphasizing the importance of understanding variable passing methods.
- 🔍 There are two primary ways to pass variables to functions in C: 'pass by value' and 'pass by address' (or 'pass by reference').
- 📥 'Pass by Value' means that a copy of the variable's value is passed to the function, leaving the original variable unchanged.
- 📫 'Pass by Address' passes the memory address of the variable, allowing the function to modify the original variable directly.
- 🔧 The example used involves an integer variable named 'tuna' which is manipulated through both methods to illustrate their differences.
- 👀 After calling the 'pass by value' function, the variable 'tuna' retains its original value, demonstrating no change.
- 📝 In contrast, after calling the 'pass by address' function, the variable 'tuna' is updated, showing how this method modifies the original data.
- 📊 Understanding these concepts is crucial for effective programming, particularly for functions that need to alter data.
- 🤔 The speaker encourages practice with these concepts to ensure they become second nature for the viewer.
- 📹 The tutorial concludes with an invitation to subscribe for more content, specifically mentioning upcoming lessons in Python programming.
Q & A
What are the two ways to pass variables into functions in C?
-The two ways to pass variables into functions in C are 'pass by value' and 'pass by reference' (or pass by address).
What happens when a variable is passed by value?
-When a variable is passed by value, a copy of its value is sent to the function. Changes made to this copy do not affect the original variable.
Can you provide an example of pass by value?
-Sure! If you have an integer variable `tuna` set to 20 and you pass it to a function by value, any changes made to the parameter inside the function will not change the value of `tuna`. It will remain 20 after the function call.
What is meant by passing a variable by reference?
-Passing a variable by reference means that the function receives the memory address of the variable. This allows the function to modify the original variable directly.
What is the effect of passing a variable by reference in a function?
-When a variable is passed by reference, any changes made to the parameter inside the function will directly affect the original variable.
Can you illustrate pass by reference with an example?
-Yes! If `tuna` is passed by reference and the function changes it to 64, then after the function call, `tuna` will be 64, reflecting the change made inside the function.
Why might a programmer choose to use pass by value?
-A programmer might choose to use pass by value to preserve the original variable's state while still performing calculations or operations in the function.
What are pointers in C, and how do they relate to passing by reference?
-Pointers are variables that store the memory address of another variable. In passing by reference, a pointer is used to provide the function with the address of the variable, enabling direct modifications.
How does understanding pass by value and pass by reference benefit a C programmer?
-Understanding these concepts helps a C programmer control how data is manipulated and managed in functions, leading to more efficient and effective coding practices.
What might be some common pitfalls when using pass by reference?
-Common pitfalls include unintentionally modifying variables, leading to bugs, and misunderstanding the implications of memory management, especially with pointers.
Outlines
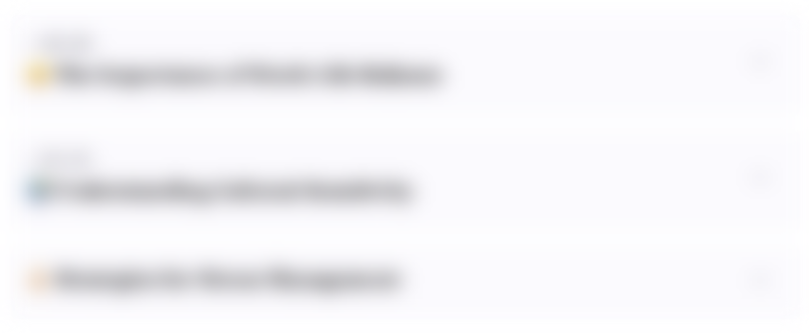
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
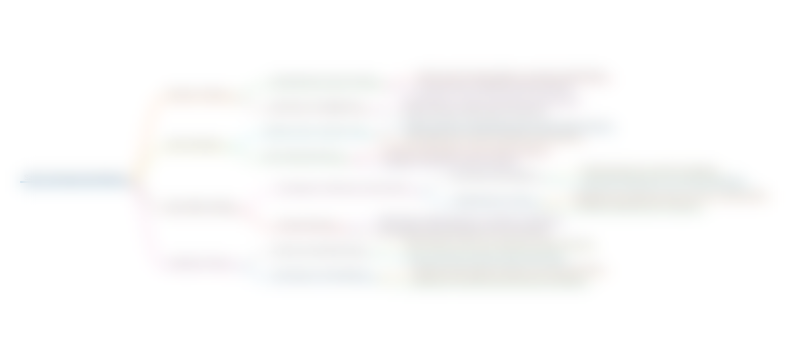
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
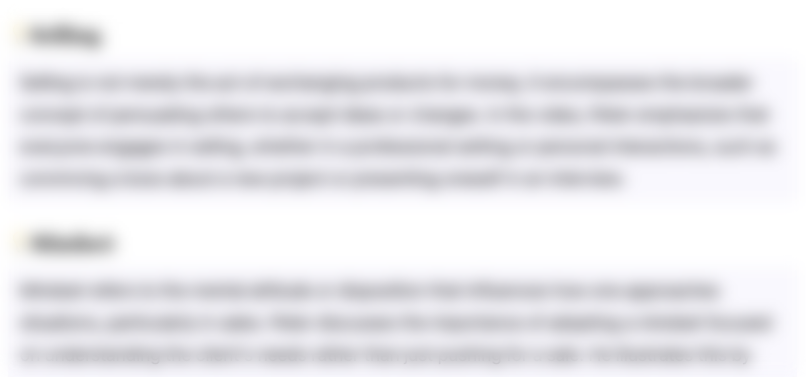
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
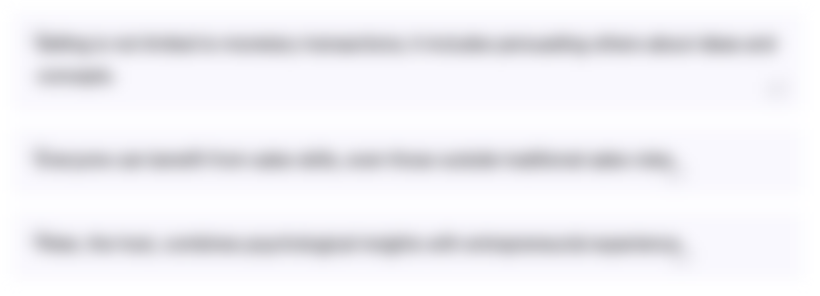
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
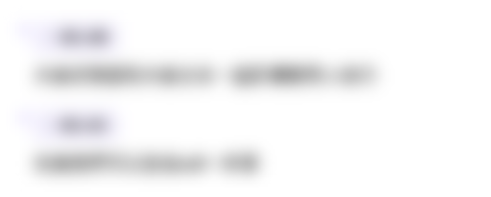
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
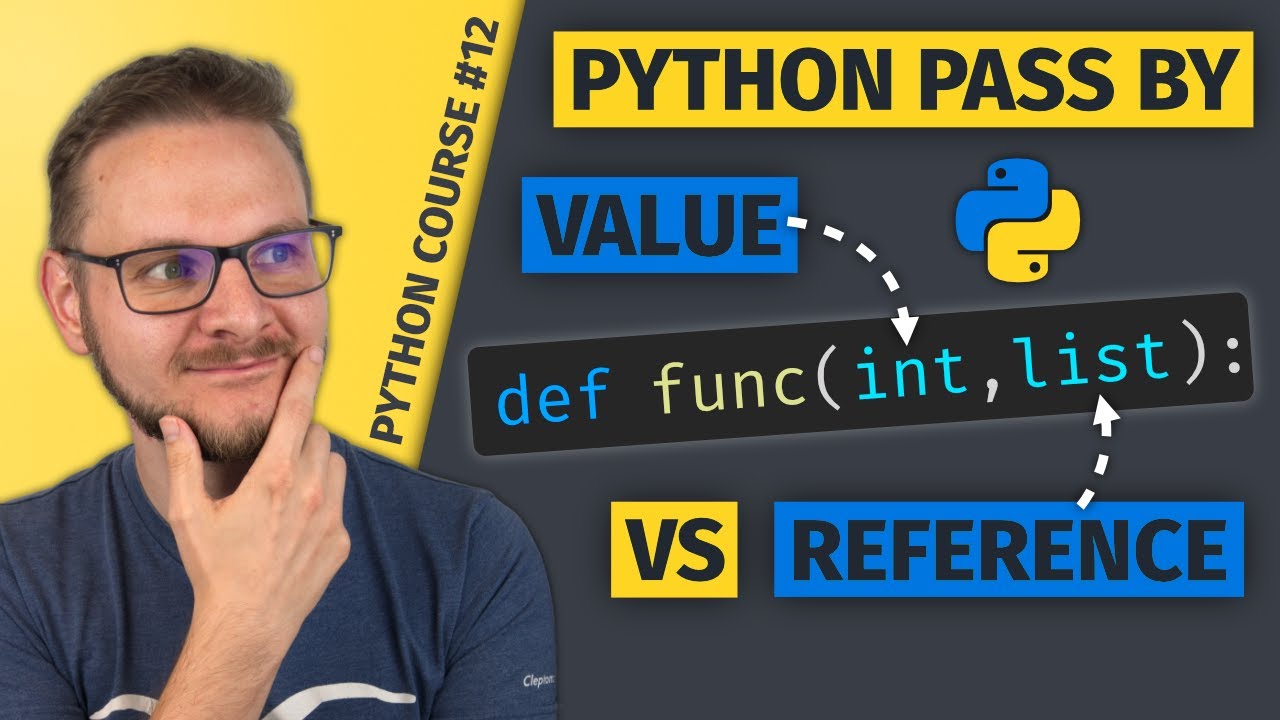
Pass by Value vs. Pass by Reference | Python Course #12
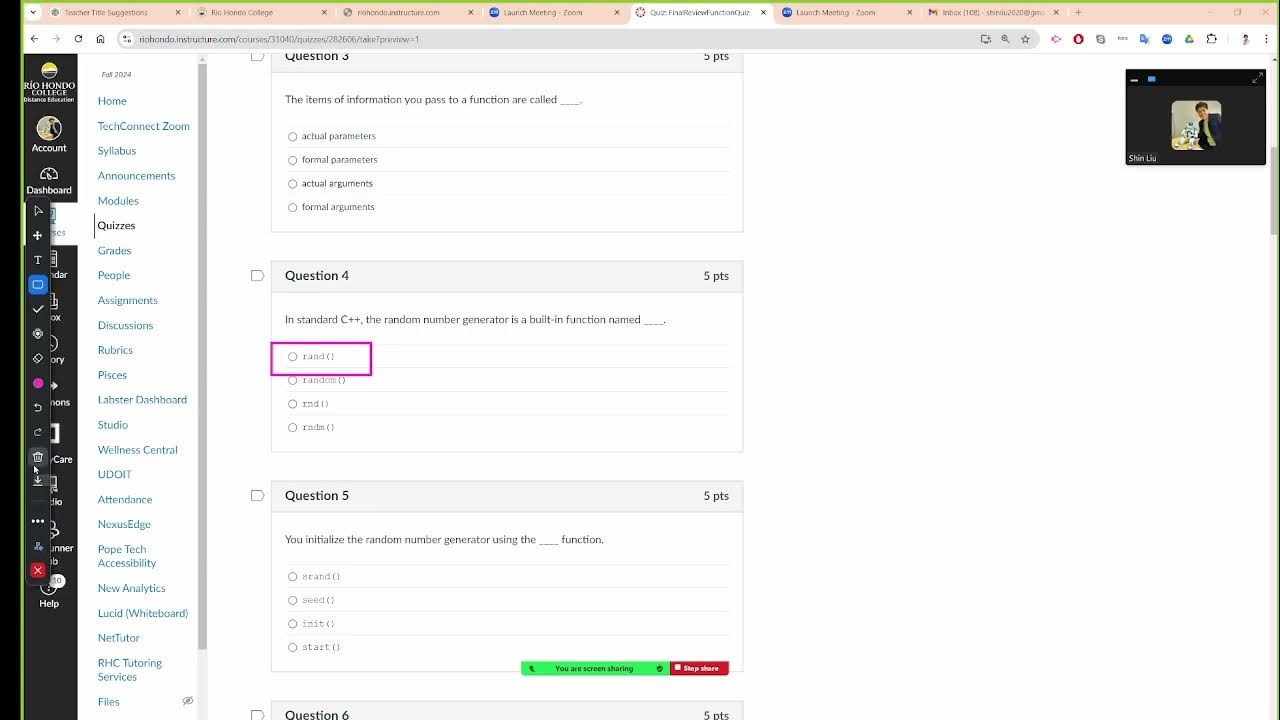
C++ Final Review Function Quiz 10 questions
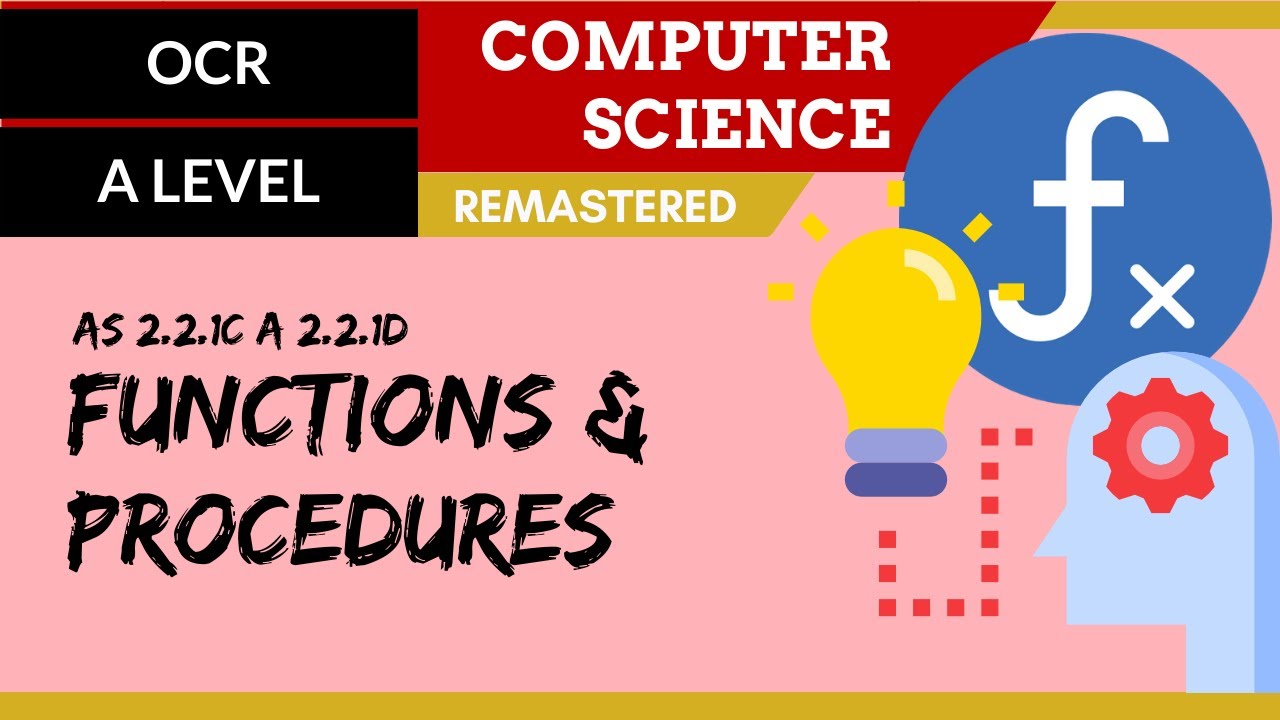
136. OCR A Level (H046-H446) SLR23 - 2.2 Functions & procedures
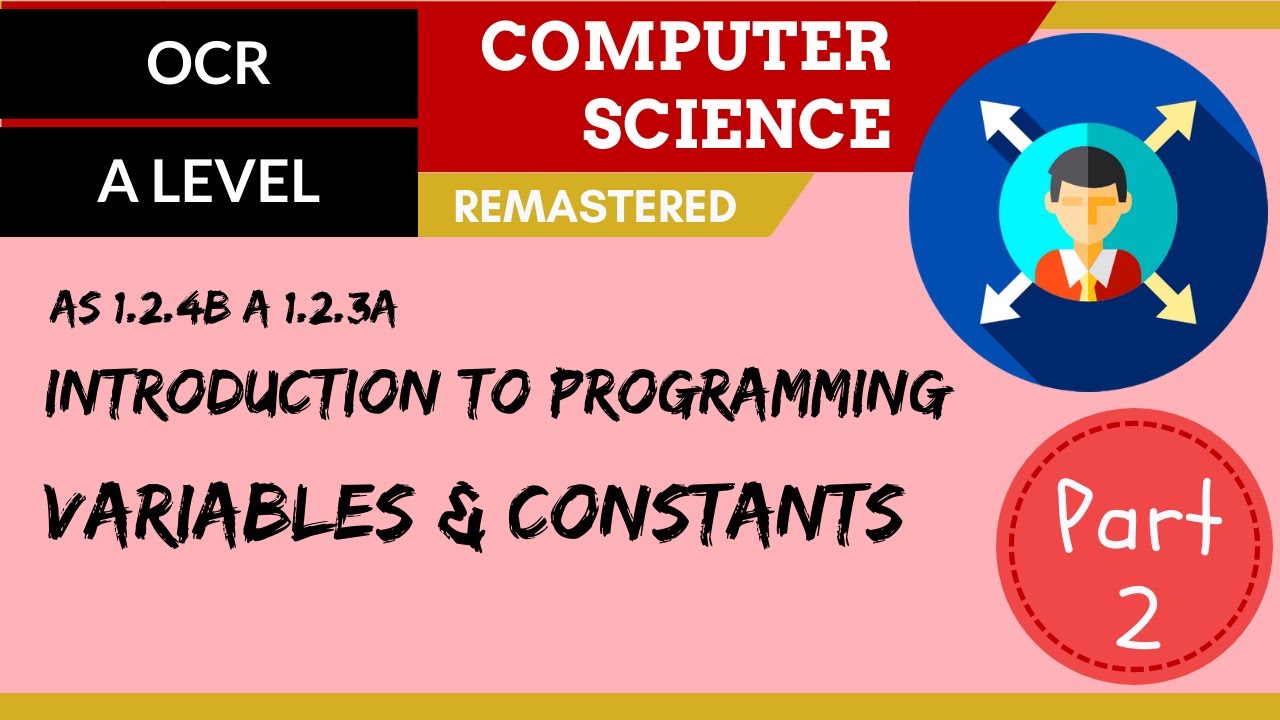
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants

ССЫЛОЧНЫЕ И ЗНАЧИМЫЕ ТИПЫ C# | СТЕК И КУЧА C# | REFERENCE AND VALUE TYPES C# | C# Уроки | # 38

COS 333: Chapter 9, Part 2
5.0 / 5 (0 votes)