Lec-6: Typecasting in Python 🐍 with Execution | Python Programming 💻
Summary
TLDRThe video script is an educational tutorial focused on Python programming, specifically discussing the concept of type casting. It begins with a simple program where the instructor demonstrates how to take two numbers from the user and print their sum. The script highlights the importance of type casting when performing operations between different data types, such as converting strings to integers or floats. The instructor uses examples to explain implicit type casting, where Python automatically converts data types during operations, and explicit type casting, where the user must manually convert data types. The tutorial aims to help students understand the nuances of type casting in Python to avoid errors and ensure accurate program execution.
Takeaways
- 😀 The video is a tutorial for students on a simple program to print the sum of two numbers.
- 🔢 The program takes two numbers as input from the user at runtime.
- 💡 The issue of incorrect output (12 14 instead of 26) is due to the default string concatenation instead of addition.
- 🔄 The concept of 'type casting' is introduced to correct the output by converting string inputs to integer.
- 🛠️ The video demonstrates explicit type casting using the `int()` function to ensure the numbers are treated as integers and not strings.
- 📚 The importance of understanding type casting is emphasized for programming, especially in Python.
- 💬 The video also covers implicit type casting, where Python automatically converts data types during operations, such as converting an integer to a float.
- 📈 An example is given where multiplying an integer by a float results in a float output, avoiding data loss.
- 🔢 The tutorial includes additional examples of converting data types, such as converting integers to hexadecimal, octal, and binary.
- 🔤 The video concludes with a demonstration of converting a character's Unicode value to its character representation.
- 💼 The takeaways are intended to prepare students for interviews and real-world programming scenarios where type casting is crucial.
Q & A
What is the main issue the video script is addressing?
-The video script is addressing the issue of type casting in programming, specifically how data types are automatically converted in Python, which can lead to unexpected results if not handled properly.
Why does the script mention 'input()' function with a default string return type?
-The 'input()' function in Python returns a string by default, even if the user inputs a number. This is important to understand because attempting arithmetic operations with these string values without converting them to integers or floats will result in string concatenation instead of the expected mathematical operations.
What is the concept of 'type casting' explained in the script?
-Type casting is the process of converting data from one type to another. The script explains explicit type casting, where the programmer explicitly converts a value from one data type to another using functions like `int()`, `float()`, `str()`, etc.
How does implicit type casting work in Python?
-In Python, implicit type casting occurs when the language automatically converts a value from one data type to another based on the context in which it is used. Python tends to convert lower data types (like integers) to higher data types (like floats) to prevent data loss.
What is the significance of the output '12 14' in the script?
-The output '12 14' signifies that the script attempted to add two numbers but treated them as strings due to the default behavior of the 'input()' function. This led to string concatenation instead of addition, resulting in the output being the concatenated string '12 14' instead of the sum '26'.
Why is it important to understand type casting when programming?
-Understanding type casting is crucial because it affects how data is processed and manipulated in a program. Without proper type casting, programmers may encounter bugs and unexpected behavior, such as the difference between performing arithmetic operations on numbers versus concatenating strings.
What is the example given in the script to demonstrate explicit type casting?
-The script demonstrates explicit type casting by converting a string input '12' to an integer using the `int()` function and then performing arithmetic operations to get the correct sum of 26.
How does the script handle the scenario where a float is implicitly converted to an integer?
-The script shows that when an integer is added to a float, Python implicitly converts the integer to a float to perform the operation, preventing data loss and ensuring the correct result is obtained.
What are the different data type conversion functions mentioned in the script?
-The script mentions several data type conversion functions, including `int()` for converting to integers, `float()` for converting to floats, `str()` for converting to strings, `hex()` for hexadecimal conversion, `oct()` for octal conversion, and `bin()` for binary conversion.
What is the purpose of the 'ord()' and 'chr()' functions in the context of the script?
-The 'ord()' function is used to get the Unicode code point of a character, and 'chr()' is used to convert a Unicode code point to its corresponding character. In the script, these functions are used to demonstrate how to convert between character values and their Unicode representations.
What is the key takeaway from the script regarding type casting in programming?
-The key takeaway is that understanding and correctly applying type casting is essential for accurate data manipulation and to avoid common bugs in programming, such as unintended string concatenation instead of arithmetic operations.
Outlines
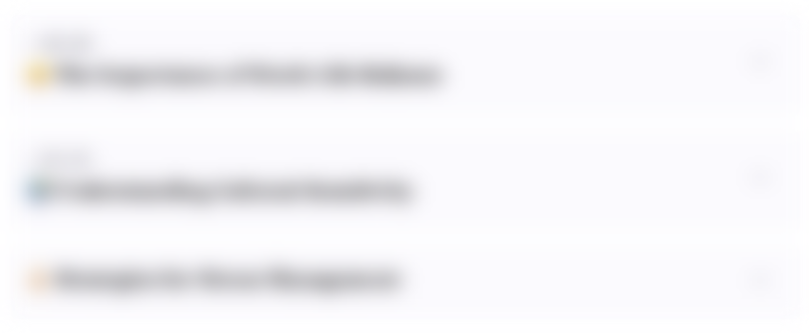
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
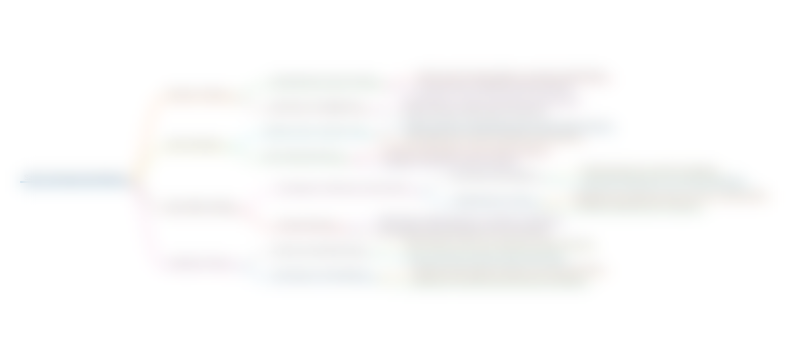
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
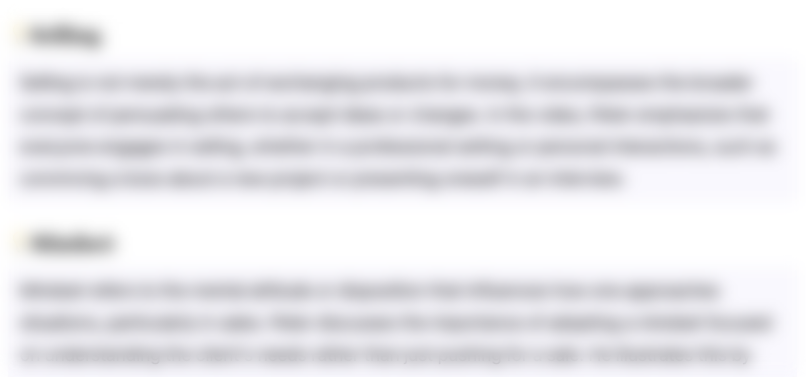
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
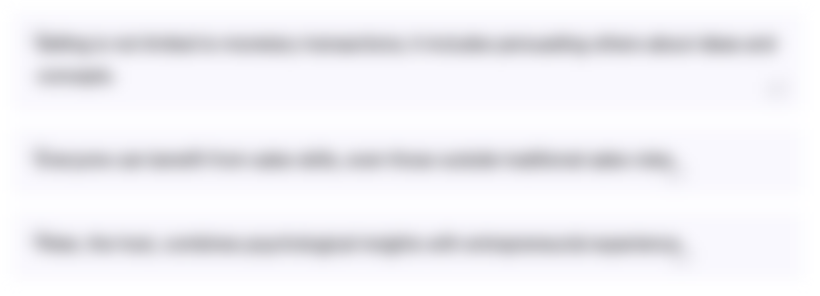
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
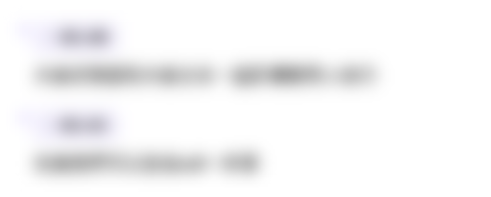
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
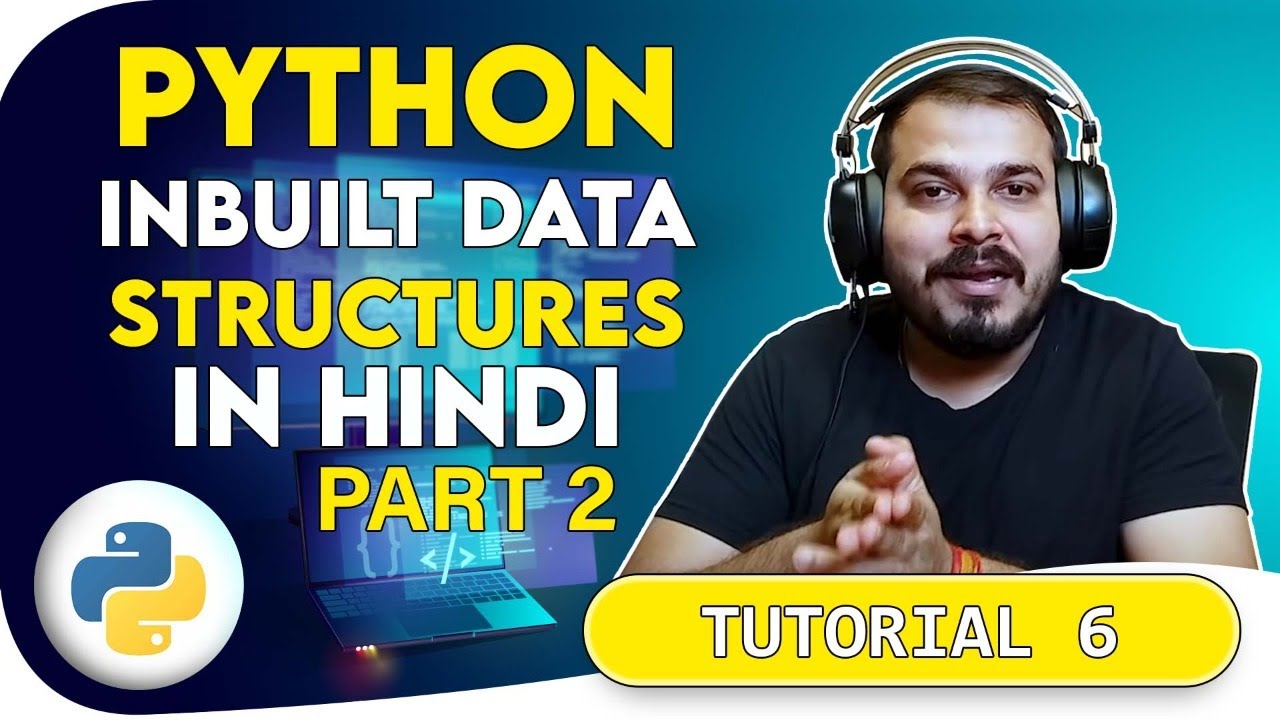
Tutorial 6- Python List And Its Inbuilt Function In Hindi
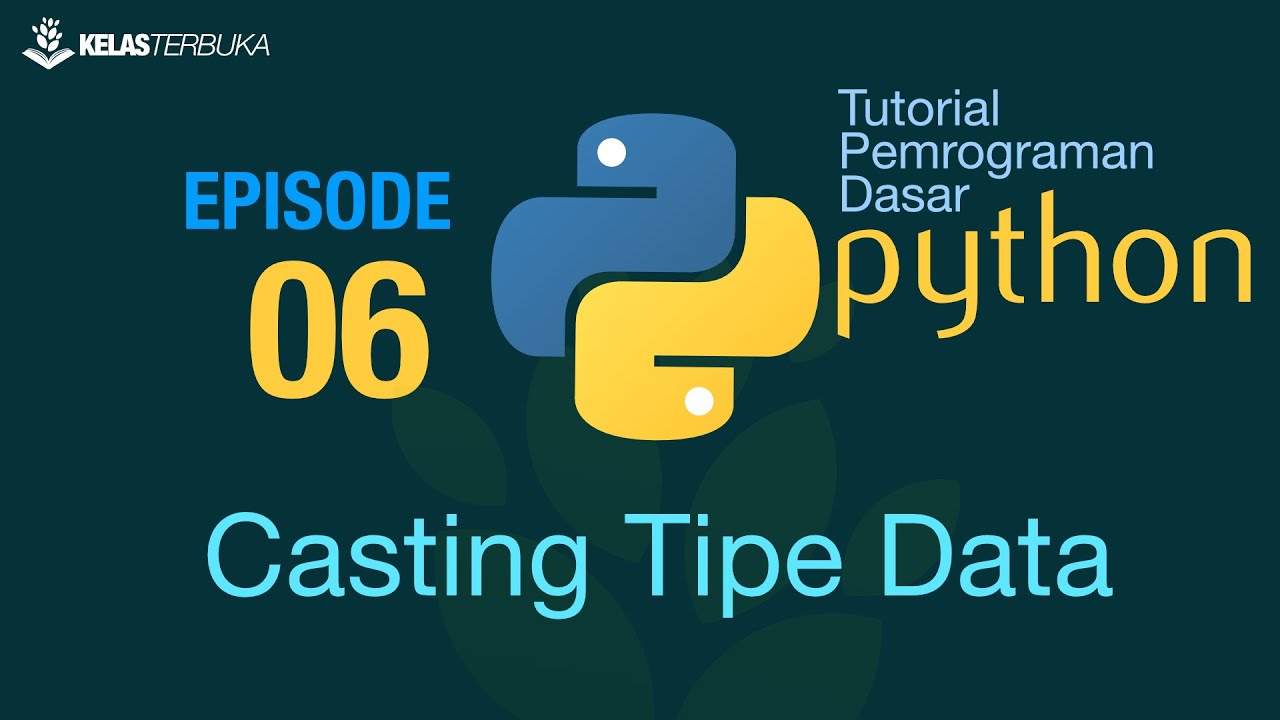
Belajar Python [Dasar] - 06 - Casting Tipe Data
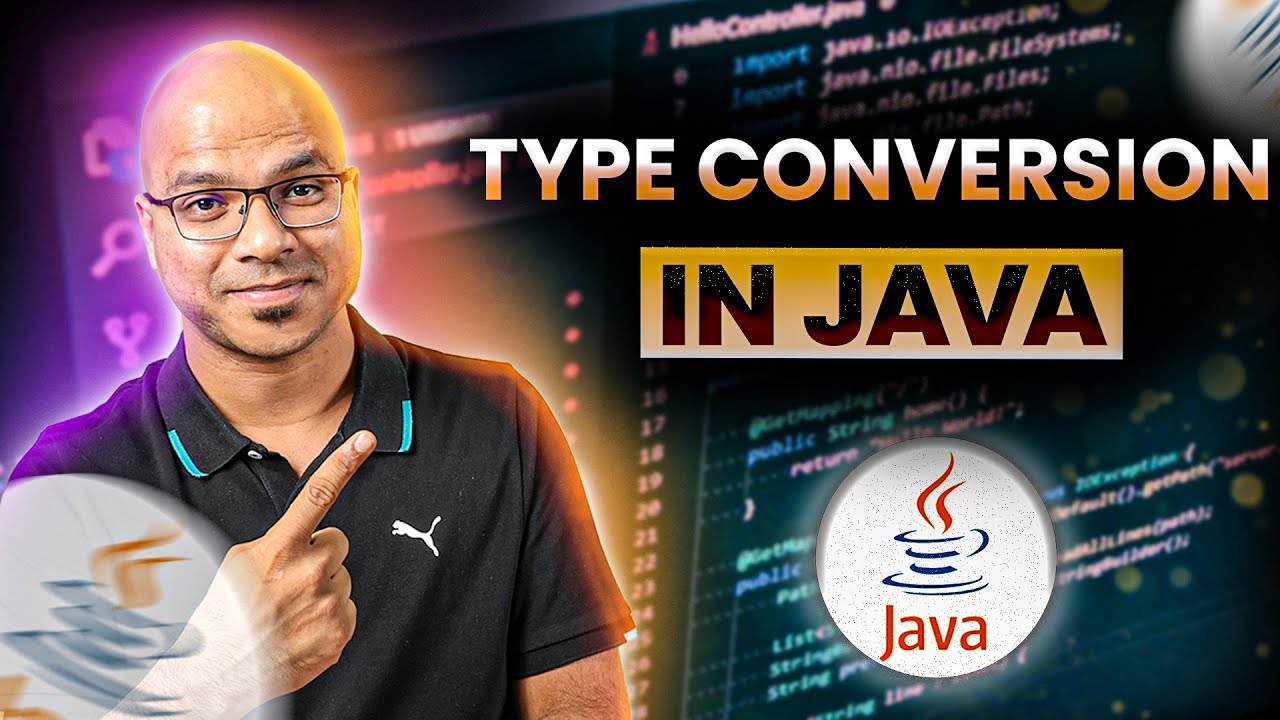
#8 Type Conversion in Java
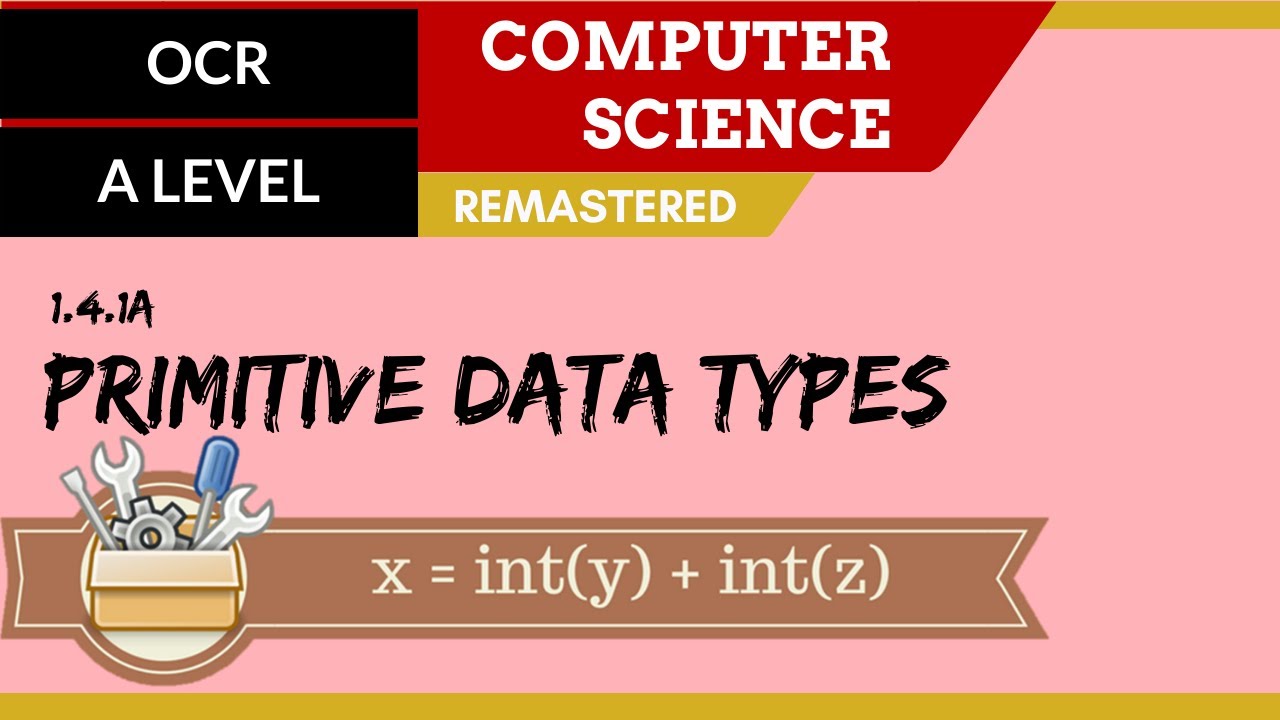
72. OCR A Level (H046-H446) SLR13 - 1.4 Primitive data types
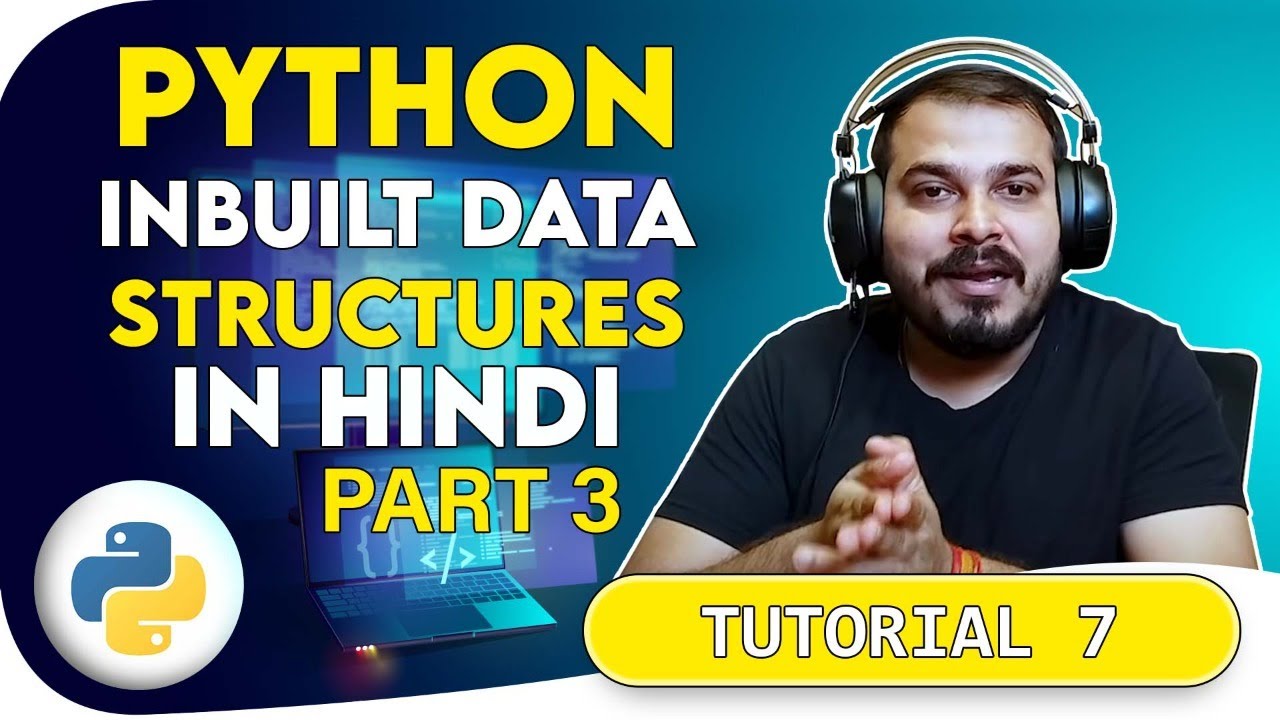
Tutorial 7- Python Sets, Dictionaries And Tuples And Its Inbuilt Functions In Hindi
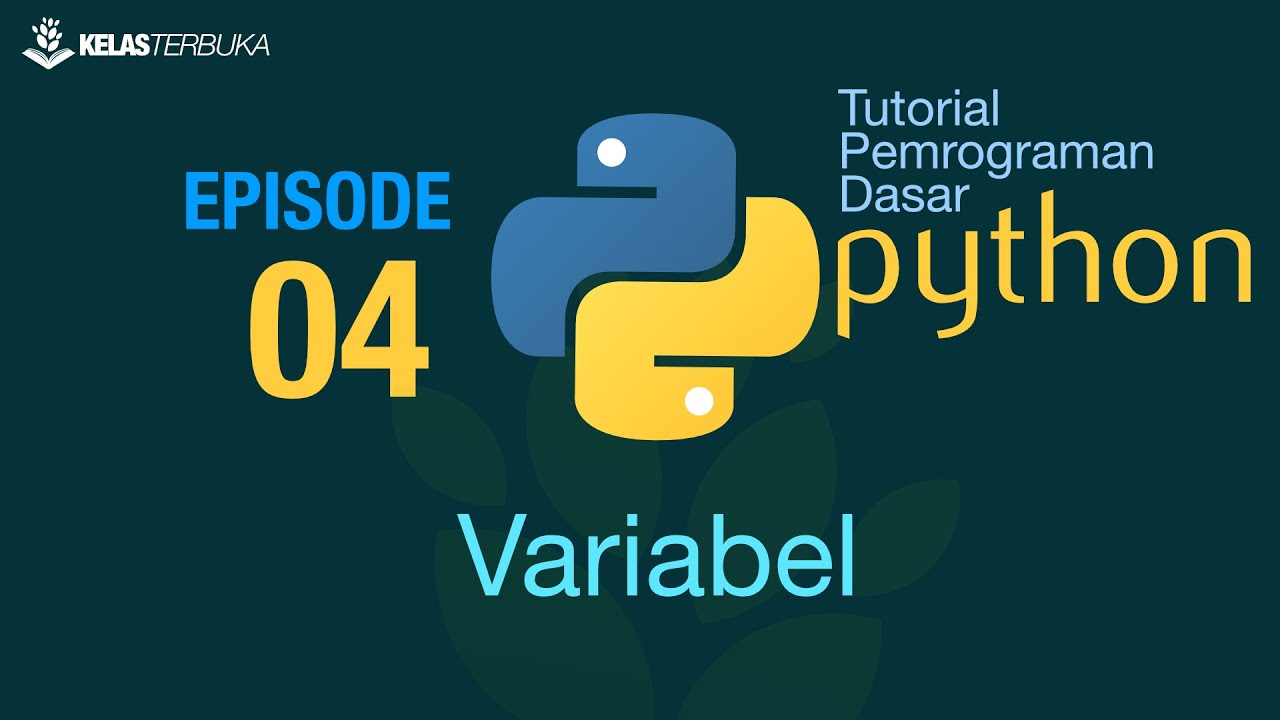
Belajar Python [Dasar] - 04 - Mengenal Variabel
5.0 / 5 (0 votes)