Subtree of Another Tree - Leetcode 572 - Python
Summary
TLDRIn this coding tutorial, the presenter tackles the 'Subtree of Another Tree' problem from LeetCode's Blind 75 list. They explain what a subtree is and propose a brute force solution to check if one binary tree is a subtree of another. The presenter then suggests a recursive approach, introducing a 'same tree' helper function to simplify comparisons. They also discuss edge cases and provide a step-by-step guide to implementing the solution, emphasizing the importance of understanding tree problems recursively.
Takeaways
- 😀 The video discusses solving the 'Subtree of Another Tree' problem from the LeetCode Blind 75 list.
- 📈 The presenter has a spreadsheet tracking all 75 problems, with notes and video solutions for most of them.
- 🔍 A subtree is defined as any tree that can be found by starting at any node in a given tree and including all nodes in that subtree.
- 🌳 The problem involves checking if one binary tree (subRoot) is a subtree of another binary tree (root).
- 🤖 A brute force approach is suggested, which involves checking every node in the root tree to see if the subtree matches the subRoot tree.
- 🔄 The presenter recommends solving the 'Same Tree' problem on LeetCode before tackling this one, as it involves recursive tree comparison.
- 🔢 The time complexity of the brute force solution is O(s * t), where s and t are the sizes of the two trees.
- 🛠️ The implementation of the solution is considered tricky, and the presenter suggests thinking recursively to understand tree problems better.
- 📝 Two functions are highlighted: a helper function 'sameTree' to compare two trees, and the main function 'isSubtree' to determine if one tree is a subtree of another.
- 🏁 The video emphasizes the importance of considering edge cases when comparing trees, such as when one or both trees are null.
- 💻 The presenter provides a step-by-step explanation of the code implementation, including base cases and recursive logic.
Q & A
What is the main problem discussed in the video?
-The main problem discussed in the video is determining whether one binary tree is a subtree of another, which is a problem from the LeetCode Blind 75 list.
What is a subtree in the context of binary trees?
-A subtree is a tree that can be found within another tree, starting from any node in the tree and including all the nodes connected to it.
What is the brute force approach to solving the subtree problem?
-The brute force approach involves visiting every node in the root tree and checking if the subtree rooted at that node matches the subroot tree by recursively comparing them.
Why is the brute force solution considered inefficient?
-The brute force solution is inefficient because it has a time complexity of O(s*t), where s and t are the sizes of the two trees, which can be computationally expensive.
What is the 'same tree' problem mentioned in the video?
-The 'same tree' problem is a LeetCode problem where you need to determine if two binary trees are exactly the same, which is a prerequisite for solving the subtree problem.
What is the role of the 'same tree' helper function in the subtree problem?
-The 'same tree' helper function is used to compare two trees recursively and determine if they are identical, which is a part of checking if one tree is a subtree of another.
What are the base cases for the 'is subtree' recursive function?
-The base cases for the 'is subtree' function are when both trees are null (return true), when one tree is null and the other is not (return false), and when the root values of the trees are not the same (also return false).
How does the video suggest handling edge cases when comparing trees?
-The video suggests handling edge cases by considering scenarios where both trees are null, one tree is null while the other is not, and when the trees have different root values, ensuring that these conditions are checked before proceeding with the recursive comparison.
What is the time complexity of the final solution provided in the video?
-The time complexity of the final solution is still O(s*t), as it involves potentially comparing every node in one tree to every node in the other tree.
How can one further support the channel mentioned in the video?
-Viewers can support the channel by liking and subscribing, as well as checking out the Patreon page where they can offer additional support.
Outlines
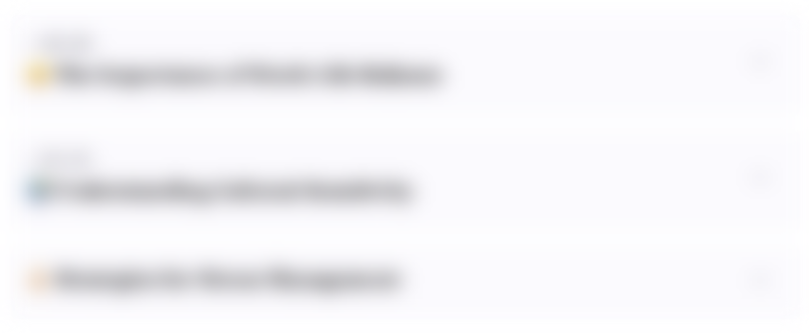
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
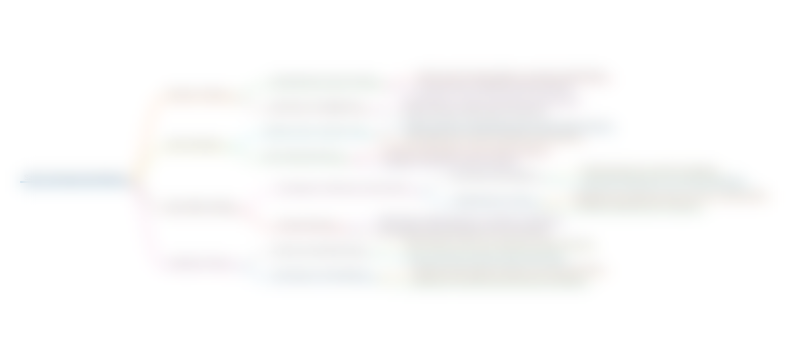
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
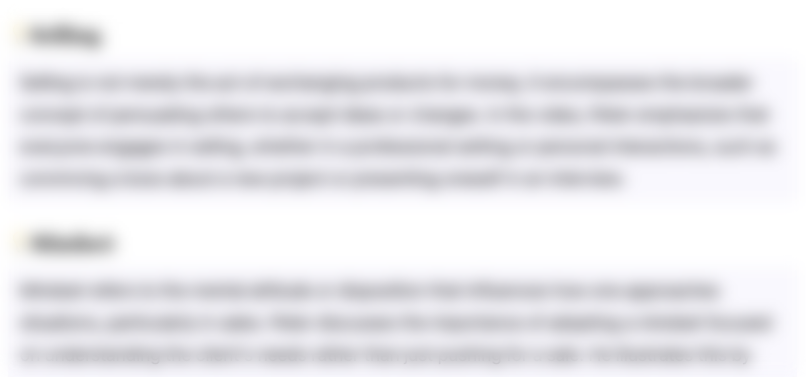
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
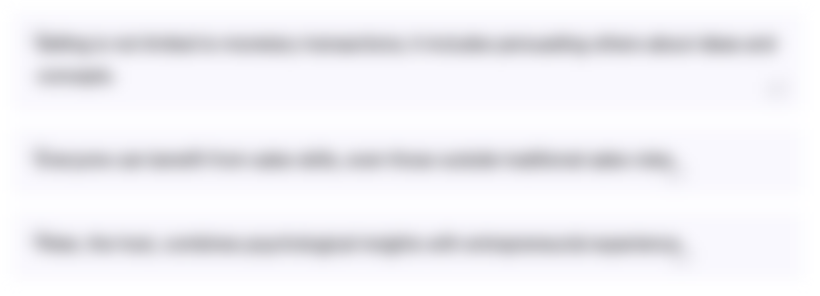
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
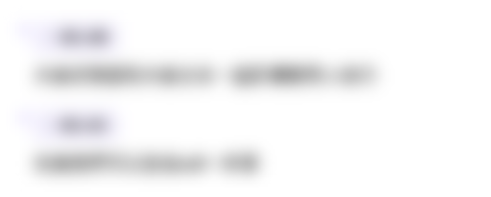
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
5.0 / 5 (0 votes)