JavaScript Strings
Summary
TLDRThis video script explores the intricacies of the String object in JavaScript, highlighting the difference between string primitives and string objects. It demonstrates properties like 'length' and methods such as 'includes', 'startsWith', 'endsWith', 'indexOf', 'replace', 'toUpperCase', 'toLowerCase', 'trim', and 'split'. The tutorial also covers escape notation and introduces template literals, offering a comprehensive guide for both beginners and experienced developers to master string manipulation in JavaScript.
Takeaways
- 📝 JavaScript has two types of strings: primitive strings and string objects. Primitive strings don't have properties or methods, but string objects do.
- 🔍 The JavaScript engine automatically wraps primitive strings with a string object when using dot notation, allowing access to string methods.
- 🔎 To learn about string methods, it's recommended to refer to the official documentation on developer.mozilla.org.
- 📊 The 'length' property of a string returns the number of characters, which is useful for input validation and character limits.
- 👉 Accessing a character at a specific index in a string can be done using square brackets, like 'message[0]'.
- 🔍 The 'includes' method checks if a string contains a specific word or character, and is case-sensitive.
- 📚 The 'startsWith' and 'endsWith' methods determine if a string begins or ends with a specified sequence, also case-sensitive.
- 🔑 The 'indexOf' method finds the index of a given character or string within a string.
- ✂️ The 'replace' method is used to replace parts of a string, returning a new string without modifying the original.
- 🔠 The 'toUpperCase' and 'toLowerCase' methods convert all characters in a string to uppercase or lowercase, respectively.
- 📝 The 'trim' method removes whitespace from both ends of a string, with variations like 'trimLeft' and 'trimRight'.
- 🚫 Escape notation is necessary for special characters in strings, such as using a backslash to escape a single quote.
- 📑 The 'split' method breaks a string into an array of words or substrings based on a specified delimiter.
- 📚 Template literals are a feature in JavaScript that allow for multiline strings and embedded expressions.
Q & A
What is the difference between a string primitive and a string object in JavaScript?
-In JavaScript, a string primitive is a basic data type that doesn't have properties or methods, while a string object is a more complex data structure that includes properties and methods. When a string primitive is accessed using dot notation, the JavaScript engine internally wraps it with a string object, allowing the use of string methods.
How can you create a string object in JavaScript?
-You can create a string object in JavaScript by using the String constructor function with the 'new' operator. For example, `let anotherString = new String('my string');`.
What is the purpose of the 'length' property in strings?
-The 'length' property returns the number of characters in a string, which is useful for validating user input length, such as ensuring a user types a minimum number of characters or not exceeding a certain limit.
How do you access a character at a specific index in a string?
-You can access a character at a specific index in a string using square brackets and the index number. For example, `message[0]` would return the first character of the string 'message'.
What does the 'includes' method do in strings?
-The 'includes' method checks if a string contains a specified sequence of characters, returning true or false. It is case-sensitive.
How can you determine if a string starts with a specific character or string?
-You can use the 'startsWith' method to determine if a string begins with a specified character or string. It is also case-sensitive.
What is the 'endsWith' method used for in strings?
-The 'endsWith' method checks if a string ends with a specified character or string and returns true or false.
How can you find the index of a character or substring within a string?
-You can use the 'indexOf' method to find the index of a character or substring within a string.
What happens when you use the 'replace' method in strings?
-The 'replace' method is used to replace a part of a string with another string. It returns a new string and does not modify the original string.
What does the 'toUpperCase' method do to a string?
-The 'toUpperCase' method converts all the characters in a string to uppercase letters and returns a new string.
What is the purpose of the 'trim' method in strings?
-The 'trim' method removes whitespace from both ends of a string. It is useful for cleaning up user input or when working with strings that have unwanted whitespace.
Can you explain the concept of escape notation in strings?
-Escape notation is used to encode special characters in strings. For example, to include a single quote in a string, you would use '\' followed by the single quote to escape it, preventing the JavaScript engine from interpreting it as the end of the string.
What is the 'split' method used for in strings?
-The 'split' method is used to split a string into an array of substrings based on a specified separator character. Each element in the resulting array is a word from the original string.
Outlines
📝 Understanding JavaScript String Primitives and Objects
This paragraph delves into the intricacies of JavaScript's handling of strings. It clarifies the distinction between string primitives and string objects, explaining how JavaScript engines internally treat string primitives as string objects when accessed with dot notation. The paragraph introduces the concept of string properties and methods, such as length, includes, startsWith, endsWith, indexOf, and replace, which are available through the String object. It also covers methods like toUpperCase, toLowerCase, and trim, emphasizing their non-destructive nature. Additionally, it touches on escape notations for special characters, illustrating how to include them in strings.
📚 Exploring String Methods and Template Literals in JavaScript
The second paragraph continues the discussion on strings by focusing on escape characters and the split method, which allows for the division of strings into arrays based on specified delimiters. It also introduces template literals, a feature that enhances string handling in JavaScript, allowing for embedded expressions and multi-line strings. The paragraph concludes with a promotional message about a JavaScript course aimed at web and mobile application developers, highlighting its comprehensive curriculum, practical exercises, and relevance to job seekers in the tech industry, with a special mention of a limited-time discount available through a link in the video description.
Mindmap
Keywords
💡String Object
💡Primitive Type
💡Dot Notation
💡Type of Operator
💡Length Property
💡Indexing
💡Includes Method
💡Starts With Method
💡Ends With Method
💡Index Of Method
💡Replace Method
💡Trim Method
💡Escape Notation
💡Split Method
💡Template Literals
Highlights
Introduction to the string object in JavaScript and its properties and methods.
Explanation of the difference between string primitives and string objects in JavaScript.
String primitives are automatically wrapped with a string object by the JavaScript engine when accessing methods or properties.
Demonstration of the length property to count the number of characters in a string.
Using square brackets to access characters at specific indices within a string.
Usage of the includes method to check if a string contains a specific word or substring.
Introduction to the startsWith and endsWith methods to check the beginning or end of a string.
Demonstration of the indexOf method to find the position of a substring within a string.
Explanation of the replace method to substitute parts of a string with a new substring.
Introduction to the toUpperCase and toLowerCase methods for changing the case of string characters.
Explanation of the trim, trimLeft, and trimRight methods to remove whitespace from strings.
Overview of escape notation for special characters within strings, such as single quotes and newlines.
Demonstration of the split method to break a string into an array based on a specified delimiter.
Introduction to template literals in JavaScript for more dynamic string creation.
Promotion of a JavaScript course designed for beginners and intermediate learners, including practical exercises and interview preparation.
Transcripts
the second built-in object we're gonna
look at is the string object so I'm
gonna define a constant message and set
it to a string now look at this message
dot what's going on here
it looks like we have a bunch of
properties and methods but earlier in
the course I told you that string is a
primitive type primitive types don't
have properties and methods only objects
do so why is it that we see these
properties and methods in this string
well the reason for this is because in
JavaScript we have two kinds of strings
so this is what we call a string
primitive but we also have a string
object so we have this constructor
function string and we can use that to
create a new string object so we can
pass the same string here now because
this is a constructor function we need
to apply the new operator and now we
have another string now let's take a
look at the type of each of these
constants so type of message that's a
string but type of another is an object
so the first constant is a string
primitive the second one is an object
however when we use the dot notation
with a string primitive JavaScript
engine internally wraps this with a
string object we don't see that but we
can work with this like a string object
now just like the math object if you
want to learn about all these methods
it's best to look at the documentation
so simply search for a JavaScript string
once again on developer mozilla.org
on this page you can see all the
properties and methods of the string
object in this lecture I'm going to show
you a few of these methods but I
strongly recommend you to look at the
documentation once just have a quick
look to see what methods are there in
case you need them so back to our code
let's change this string to this is my
first message here we have the length
property which returns the number of
characters in a string this is
particularly useful in situations where
you want to make sure the user types at
least certain number of characters in an
input field or maybe you want to put a
limit you don't want the user to type in
more than 100 characters now if you want
to access a character and a given index
you can use square brackets so message
of 0 returns T message of 1 returns H if
you want to see this string has a
special word you can use the includes
method so does the string have my yes it
does but it doesn't have not we also
have another method starts with so this
string starts with this but if you pass
a capital T here we get false so note
that these Searchers are case sensitive
we have a similar method ends with so
message that ends with E so you can see
the last character here is e if you want
to find the index of a given character
or a given string inside this string you
can use the index of method so let's see
what is the index of my so my start at
index 8 we can also replace a part of a
string so replace let's say you want to
replace first ways second pretty easy
note that this returns a new string and
does not modify the original one so if
you log the original one we still have
this is my first message we also have
a couple useful methods to uppercase
once again this returns a new string
where all characters are uppercase
similar to this method we have two lower
case and another useful method is trim
so let me have a couple of white spaces
here before and after our message now if
we call the trim method it gets rid of
all the white space before and after our
message and of course this method has
variations for example we have trim left
which only removes the whitespace at the
beginning of the string we have trim
bright and so on another important
concept you need to know in JavaScript
is escape notation so if you look at the
documentation for the string object you
can see in this table under escape
notation we've got these special
characters so if you want to use these
you need to encode them using the escape
notation for example let's say you want
to have a single quote in your string
now in this example we have defined this
string with a single code so if you want
to have a single code inside of the
string
look our JavaScript engine gets confused
because it thinks this second single
code represents the end of the string to
fix this we need to prefix this with a
backslash and now this character is
escaped it's encoded so when we log the
message you can see the single code is
actually part of the string another
useful escape character is backslash n
which represents a newline so back here
if we add a backslash n after my this
will add a new line so save the changes
let's look at message on the console you
can see we have a new line here another
very useful method is the split method
so message dot split with this we can
split a string based on a given
character so here I'm gonna pass a
whitespace and see what we get we get an
array of 5 items and each either
in this array is a word in our message
next we're going to look at template
literals
hi guys thank you for watching my
javascript tutorial this tutorial is
part of my JavaScript course where you
learn all the essential JavaScript
features that every web and mobile
application developer must know if
you're an absolute beginner or have some
experience in JavaScript and are looking
for a fun and in-depth course that
teaches you the fundamentals of
JavaScript this course is for you this
course is also packed with tons of
exercises that help you master what you
learned in the course in fact many of
these exercises are questions that come
up in technical programming interviews
so if you're pursuing a job as a
front-end or a back-end developer or if
you simply want to have a more in-depth
understanding of JavaScript I highly
encourage you to enroll in the course
for a limited time you can get this
course with a discount using the link in
the video description click the link to
find out more about the course and
enroll
関連動画をさらに表示
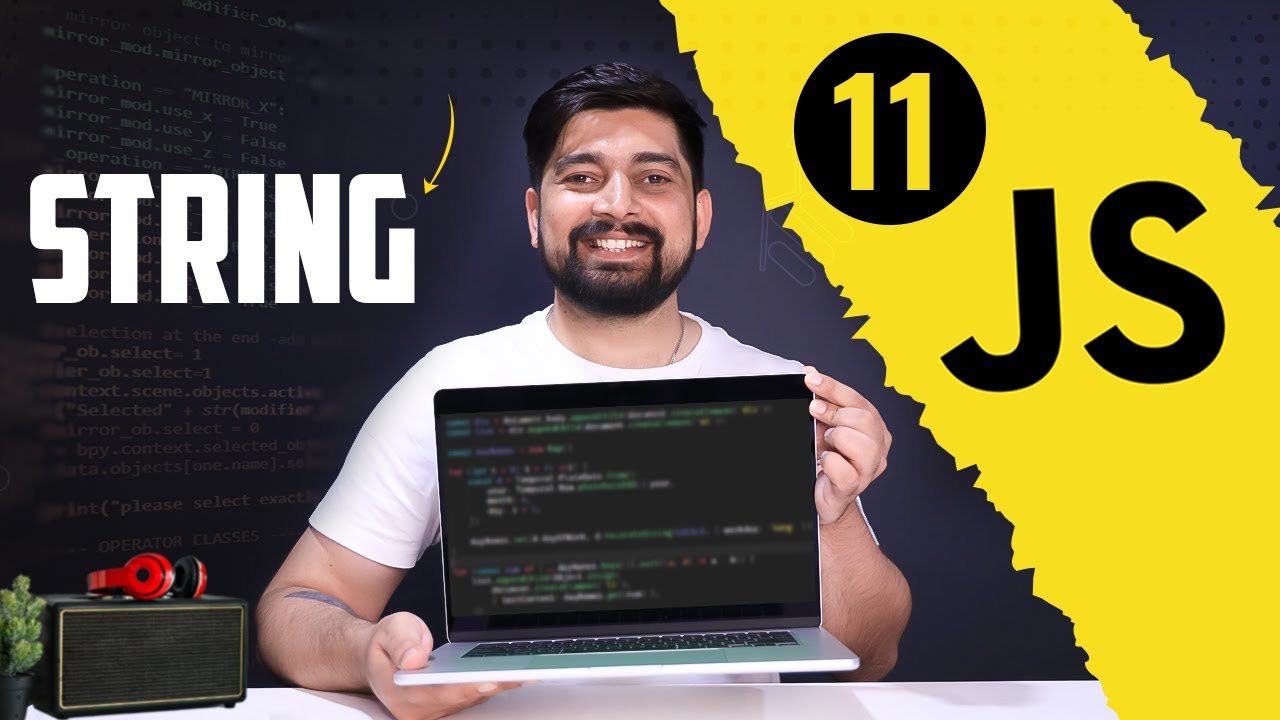
Strings in Javascript | chai aur #javascript
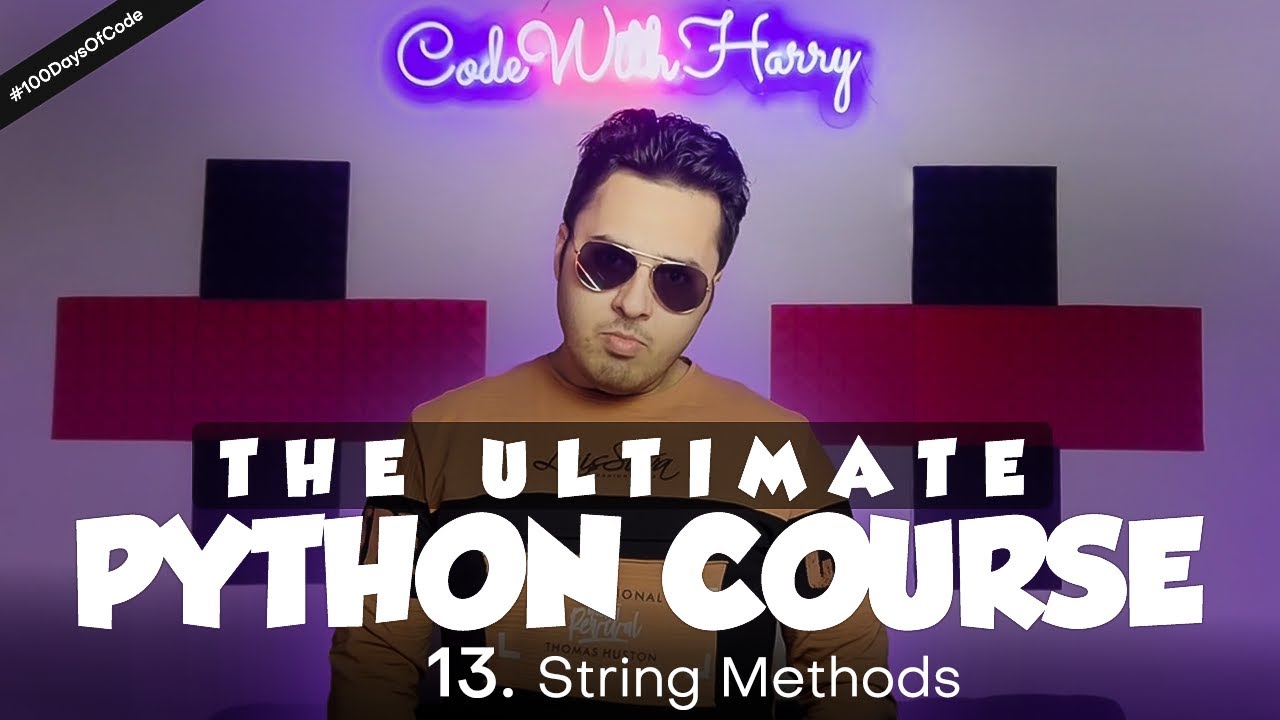
String Methods in Python | Python Tutorial - Day #13

C++ Strings | What is String? full Explanation
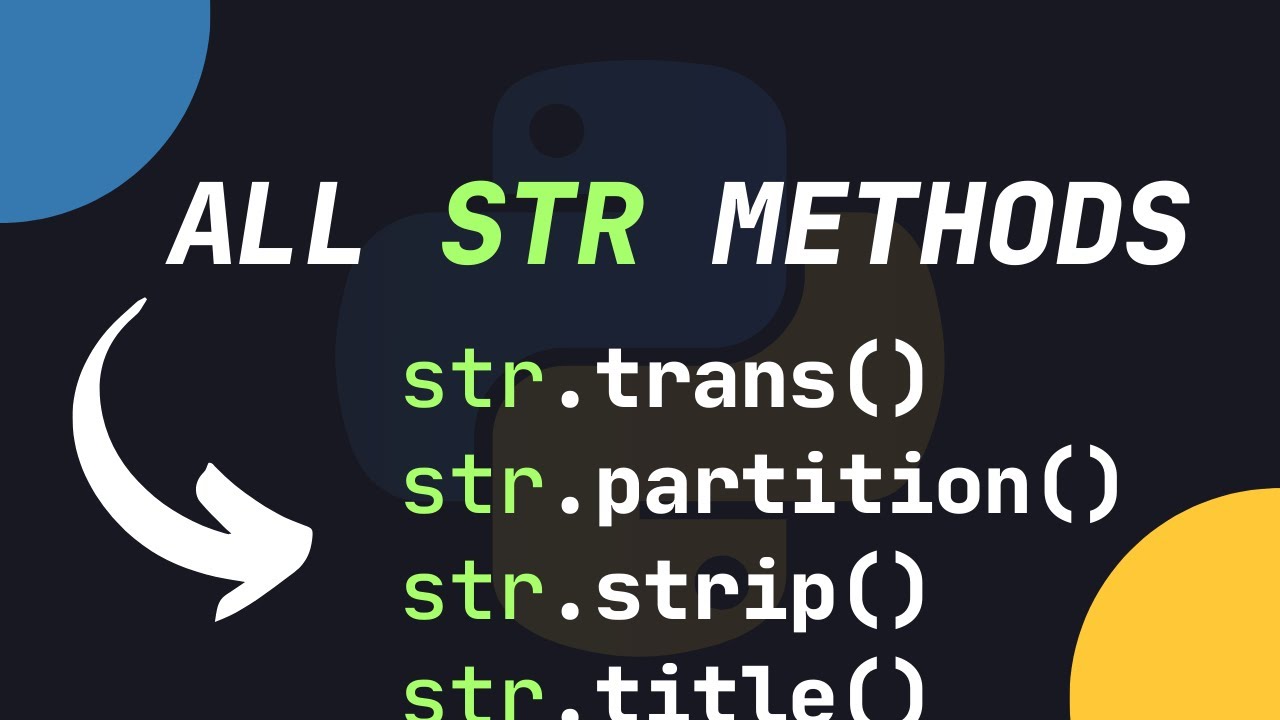
ALL 47 STRING METHODS IN PYTHON EXPLAINED
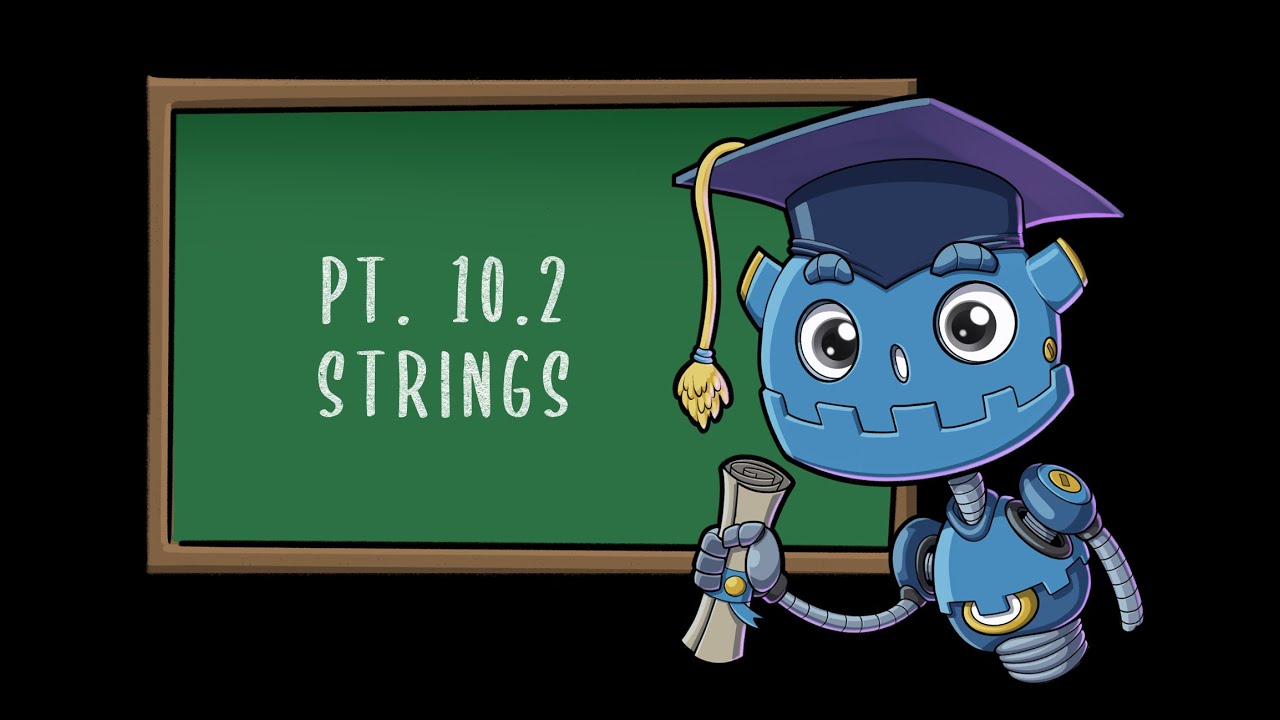
Strings (Basics of Memory) | Godot GDScript Tutorial | Ep 10.2
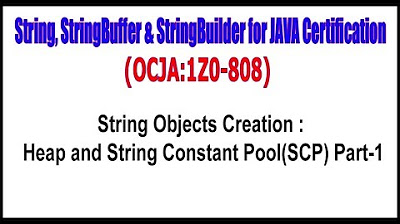
OCJA(1Z0 - 808) || String Objects Creation Heap and String Constant Pool (SCP) Part - 1
5.0 / 5 (0 votes)