#7 Arrays and Pointer Arithmetic
Summary
TLDRIn this lesson on embedded systems programming, Miro Samek introduces arrays and basic pointer arithmetic in C, focusing on how to optimize GPIO register manipulation for the Stellaris microcontroller. The lesson explains how to bypass the read-modify-write sequence with atomic write operations using pointers and arrays, ensuring faster and interrupt-safe GPIO control. Samek also covers the use of advanced peripheral buses (APB and AHB) to further enhance performance. Through practical examples, students learn to manipulate GPIO bits efficiently, using array indexing and pointer arithmetic for clean, effective code. The lesson concludes with an exploration of using the AHB bus for even faster operations.
Takeaways
- 😀 Introduction to arrays and basic pointer arithmetic in C.
- 😀 Learn how to use GPIO DATA registers in Stellaris microcontroller for bit manipulation.
- 😀 Understanding read-modify-write sequences in GPIO registers and their limitations with interrupts.
- 😀 The read-modify-write sequence can be problematic when interrupts change GPIO values during the cycle.
- 😀 Stellaris GPIO hardware design allows atomic writes to specific bits, avoiding the read-modify-write problem.
- 😀 Explanation of how GPIO bits are connected to a dedicated data and address line, enabling isolated bit manipulation.
- 😀 Introduction to using unique addresses for different combinations of GPIO bits in Stellaris, with 256 DATA registers.
- 😀 Hard-coding the GPIO register addresses directly in C can work but is not efficient or scalable.
- 😀 Using arrays in C simplifies managing GPIO registers and improves code readability.
- 😀 C arrays are closely related to pointers, allowing for efficient manipulation and access of GPIO registers using pointer arithmetic.
- 😀 Demonstration of pointer arithmetic and array indexing in accessing GPIO registers, with all approaches producing the same machine code.
- 😀 Difference between address arithmetic and pointer arithmetic: address arithmetic requires scaling, while pointer arithmetic handles it automatically.
- 😀 Code refactor to use array indexing for clarity and efficiency in manipulating GPIO bits.
- 😀 A faster and more efficient AHB peripheral bus is available on the Stellaris microcontroller, allowing for improved performance.
- 😀 Final steps involve switching from the default APB bus to AHB and updating GPIO register addresses accordingly.
Q & A
What is the main focus of this lesson?
-The main focus of this lesson is to introduce arrays and basic pointer arithmetic in C, specifically how these concepts can be applied to manipulate GPIO registers on the Stellaris microcontroller.
Why is the read-modify-write sequence used in manipulating GPIO registers?
-The read-modify-write sequence is used to change individual bits of the GPIO register without affecting other bits. It reads the current value of the register, modifies the relevant bit, and then writes the modified value back.
What issue does the read-modify-write sequence face when interrupts are involved?
-When an interrupt occurs during the read-modify-write sequence, changes made in the interrupt service routine can be lost, as the main code uses the GPIO register's previous value instead of the updated one.
How does the Stellaris GPIO hardware avoid the read-modify-write sequence?
-The Stellaris GPIO hardware allows for atomic write operations to individual GPIO bits by providing a unique address for each possible bit combination, which eliminates the need for the read-modify-write sequence.
How do GPIO bits communicate with the CPU in Stellaris?
-GPIO bits are connected to the CPU through a bus. Each bit is connected to both a dedicated data line and an address line, allowing the bit to be modified when the associated address line is set to 1.
What is the significance of the address ending in '0000111000' for GPIO manipulation?
-The address '0000111000' corresponds to the three GPIO bits connected to the LEDs. The address must be divisible by 4 due to hardware requirements, and this specific address allows direct access to the GPIO bits controlling the LEDs.
What role do arrays play in simplifying GPIO register manipulation?
-Arrays in C allow you to group multiple GPIO registers, making the code more elegant and readable. Instead of manually addressing each GPIO bit, arrays allow for easier indexing and access to registers.
How does pointer arithmetic relate to arrays in this context?
-Pointer arithmetic in C allows you to treat arrays as pointers. By adding an index to a pointer, you can directly access array elements, which is equivalent to using array indexing. This simplifies accessing GPIO registers in a more compact and efficient manner.
Why is array indexing considered the cleanest approach in the lesson?
-Array indexing is considered the cleanest approach because it is straightforward and easy to understand. It directly expresses the intention to access a specific GPIO register without additional complexity from pointer arithmetic or address calculations.
What is the difference between address arithmetic and pointer arithmetic in this context?
-Address arithmetic involves manually computing addresses and then casting them to pointers, requiring you to scale the offset based on the size of the data. In contrast, pointer arithmetic automatically handles the scaling of offsets when accessing array elements, making it simpler and more elegant.
What is the advantage of using the AHB bus over the APB bus for GPIO access?
-The AHB (Advanced High-Performance Bus) is faster and more efficient than the APB (Advanced Peripheral Bus), which is older and slower. By switching to AHB, you can achieve faster GPIO access and improved performance.
How do you switch from the APB bus to the AHB bus for GPIO in Stellaris?
-To switch from the APB bus to the AHB bus, you need to modify the GPIOHBCTL register by setting the bit corresponding to PortF. Additionally, you must update the GPIO register addresses in the code to use the AHB address range, denoted by the '_AHB' suffix.
Outlines
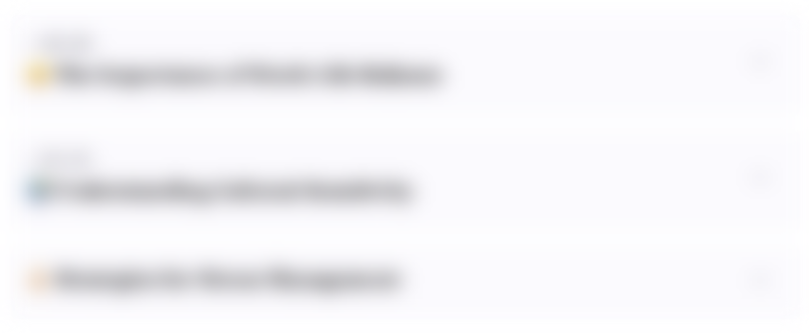
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
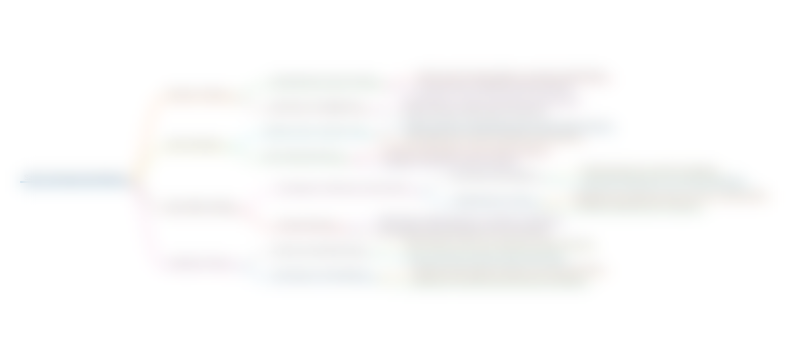
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
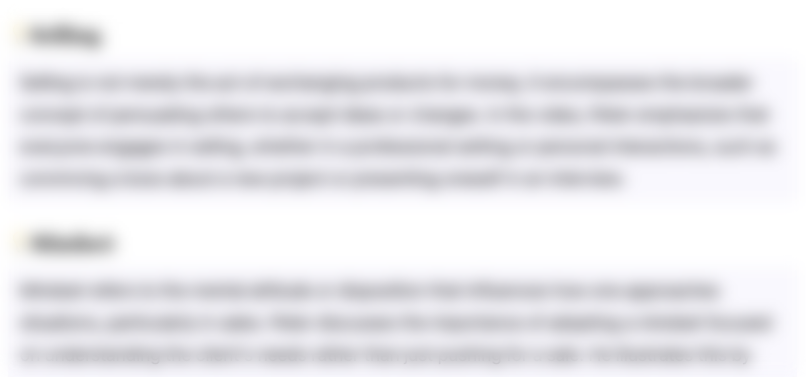
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
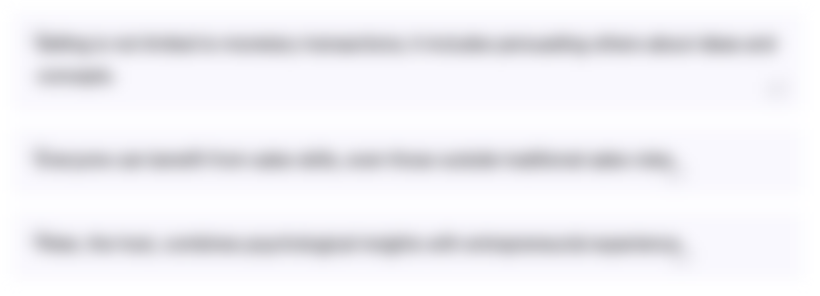
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
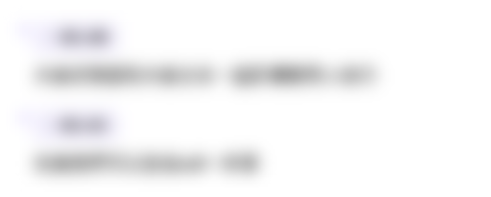
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)