Python Tutorial: File Objects - Reading and Writing to Files
Summary
TLDRThis tutorial covers essential Python file handling techniques, specifically how to work with binary files like images. The video demonstrates opening files in binary mode, reading and writing files in chunks, and copying files using efficient methods. Key concepts include managing chunk sizes for reading and writing and using loops for file operations. The tutorial also hints at future content on temporary and in-memory files, offering a comprehensive introduction to file handling in Python for users looking to expand their coding skills.
Takeaways
- 😀 Files can be opened in binary mode using 'rb' and 'wb' for reading and writing binary data like images.
- 😀 A basic method to copy files is to open the source file in binary mode, read the contents, and write them to a new file.
- 😀 The 'with' statement is used to manage files, ensuring they are properly closed after use.
- 😀 Reading and writing files line by line works well for text files, but for binary files, you should use binary read and write modes.
- 😀 Instead of processing files line-by-line, larger chunks of data can be read and written for more control over file operations.
- 😀 Using chunks to copy files allows for efficient memory usage and prevents potential memory overload when dealing with large files.
- 😀 A typical approach for reading and writing in chunks involves specifying the chunk size (e.g., 496 bytes) and handling each chunk until the end of the file.
- 😀 A while loop checks if the chunk read is not empty and continues to write the chunk to the destination file until the whole file is copied.
- 😀 The use of the 'read(size)' function allows reading a specific amount of data at a time, improving performance for large file transfers.
- 😀 The example code demonstrates how to make an exact copy of an image file, showing the binary data being transferred without any loss of quality or format.
- 😀 The presenter plans to offer more tutorials in the future on working with temporary files, in-memory files, and more advanced file operations.
Q & A
Why is it important to open files in binary mode when copying image files?
-Opening files in binary mode ('rb' for reading and 'wb' for writing) ensures that the file is treated as raw data rather than text. This is essential for binary files like images, which may contain special characters or encoding that could be corrupted if treated as text.
What is the purpose of adding the 'b' when opening files for reading and writing?
-The 'b' added to the file mode ('rb' and 'wb') specifies that the file should be opened in binary mode. This is crucial for non-text files such as images, audio, or video, which need to be processed byte-by-byte without any automatic encoding or decoding.
What changes were made in the script to allow the image to be copied correctly?
-The script was modified by opening the files in binary mode ('rb' and 'wb'). This change ensures that the raw byte data from the original file is correctly copied to the new file without any data loss or corruption.
How does the chunk size affect file reading and writing in this script?
-The chunk size defines how much data is read from the original file at once. In this script, a chunk size of 496 bytes is used. This allows for more efficient memory usage and faster processing, especially when working with large files.
Why is a while loop used in the script for copying files in chunks?
-The while loop is used to keep reading and writing chunks of the file until the entire file has been processed. The loop runs as long as there is data left to read (i.e., when the length of the chunk is greater than zero), ensuring that the entire file is copied.
What would happen if the chunk size was set to a smaller or larger value?
-If the chunk size is smaller, the script will read and write more frequently, which could lead to increased overhead and slower performance. A larger chunk size may reduce the number of reads/writes but could consume more memory, depending on the size of the file.
How does the script ensure that no data is skipped when copying the file?
-The script reads the file in chunks and writes each chunk sequentially to the new file. The while loop continues until all data has been read, ensuring that no part of the original file is skipped during the copy process.
What does the 'length of the chunk' in the while loop check for?
-The 'length of the chunk' checks if there is any data remaining to be read. If the chunk is empty (i.e., its length is zero), it means the end of the file has been reached, and the loop stops to prevent an infinite loop.
What role does the 'read' and 'write' functions play in this script?
-The 'read' function reads a specified amount of data (a chunk) from the original file, and the 'write' function writes that data to the new file. These functions are essential for transferring the data from the source file to the destination file.
What is the significance of deleting the copied file before running the script again?
-Deleting the copied file ensures that the script creates a fresh copy each time it is run, allowing for verification that the file copying process works correctly. This prevents errors caused by overwriting or reading from an outdated copy.
Outlines
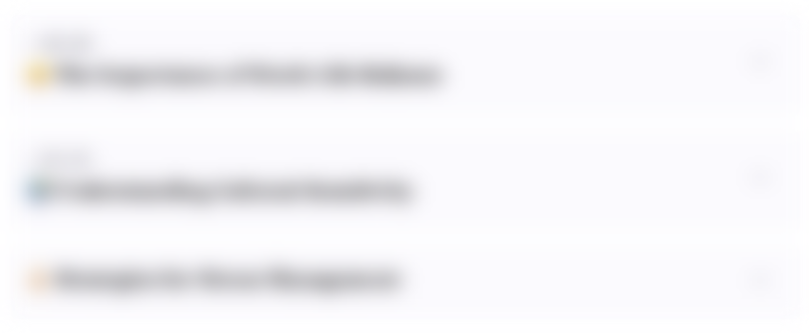
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
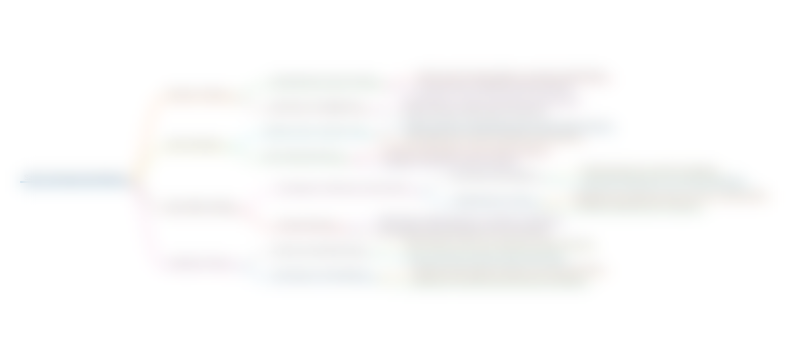
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
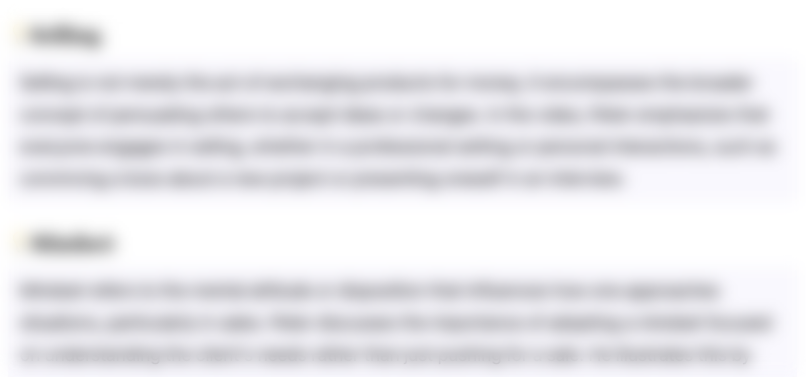
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
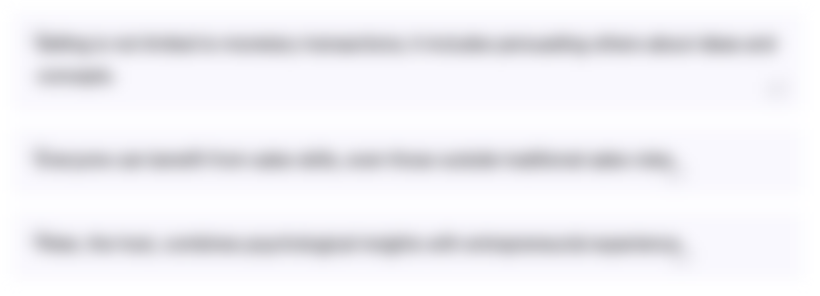
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
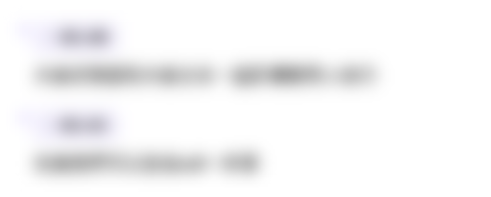
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
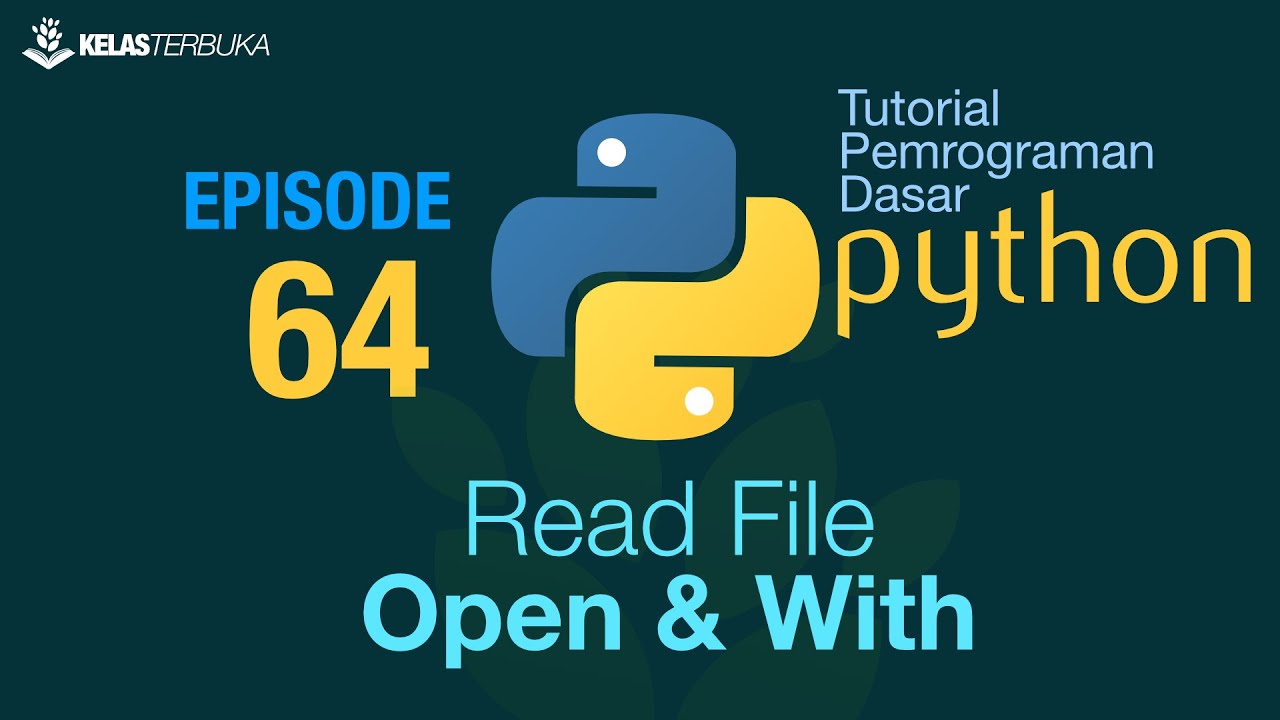
Belajar Python [Dasar] - 64 - Read external file - Open dan With
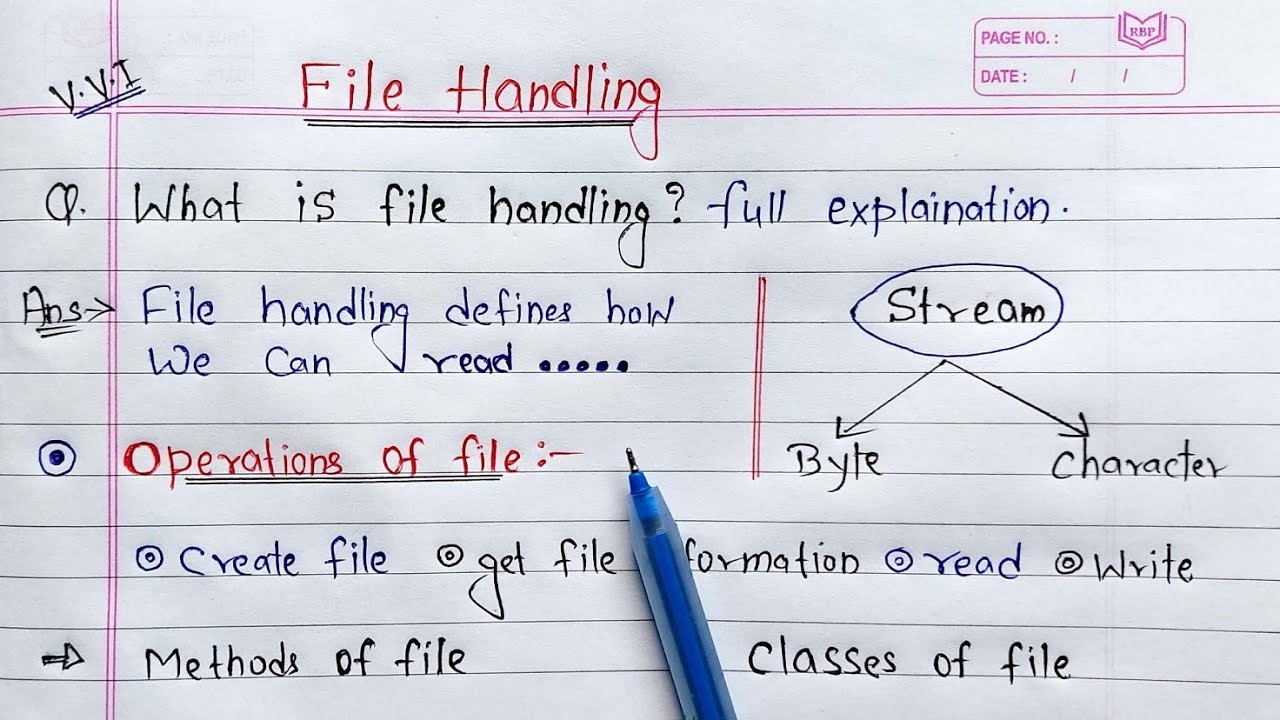
File Handling in Java | Java program to create a File
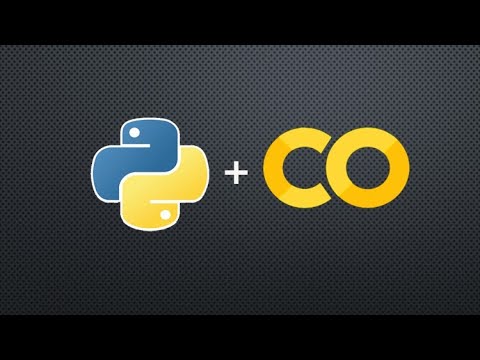
OpenCV on Google Colab - Working with Gray Image

BELAJAR PHP : mengimplementasikan Upload File berupa Gambar/Foto ke dalam database MySQL melalui WEB

C++ File Handling | Learn Coding
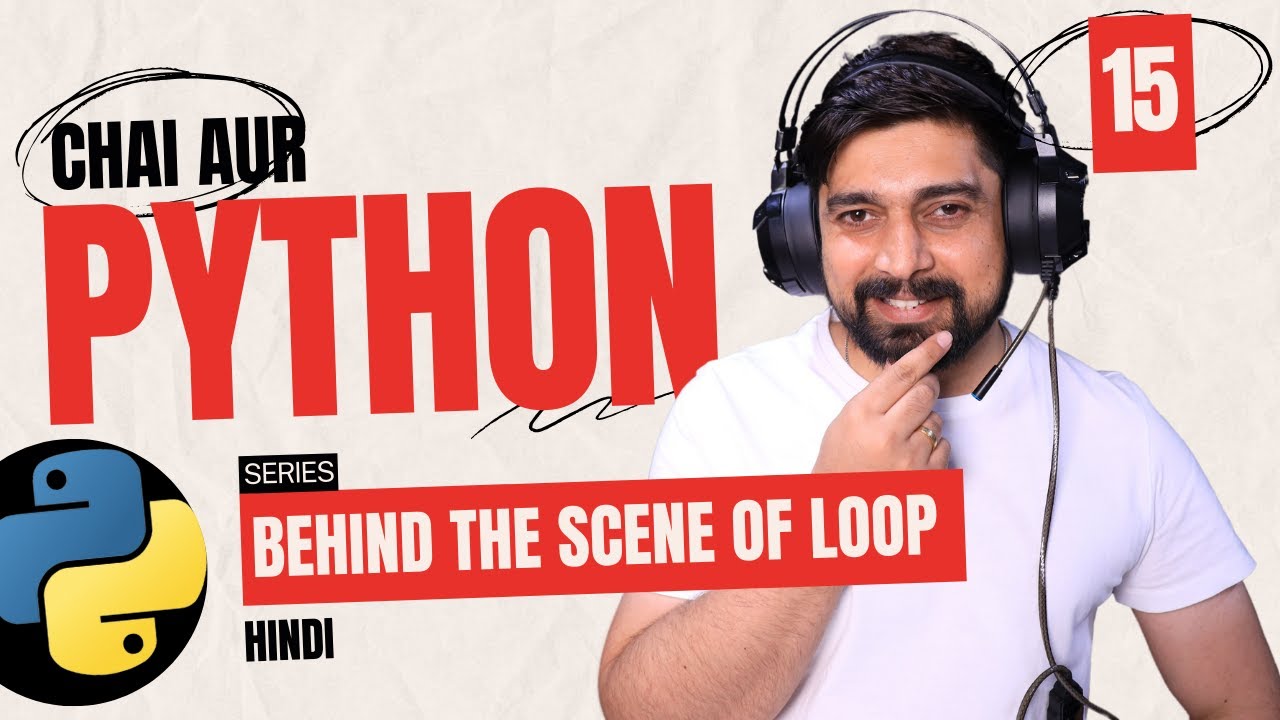
Behind the scene of loops in python
5.0 / 5 (0 votes)