Thread & Multithreading #JAVA
Summary
TLDRThis video explains the concept of multi-threading in programming, specifically its application in simulating the travel distance of two cars asynchronously. The speaker walks through creating a Java application that uses multi-threading to run two processes simultaneously, enhancing CPU utilization. The task involves implementing classes with Runnable interfaces to simulate the movement of cars, and the output is displayed as a dynamic process. The speaker also covers aspects like loop iteration, class implementation, and how the asynchronous nature of the program results in random outputs, mimicking real-time car movement simulations.
Takeaways
- 😀 The script introduces a topic about trading and how multithreading can improve the efficiency of program execution by allowing multiple tasks to run simultaneously.
- 😀 Multithreading is defined as the process of executing two or more threads concurrently to maximize the CPU's utility.
- 😀 The task involves creating a simulation application to model the travel distance of two cars asynchronously.
- 😀 The logo design for the simulation must represent two cars, A and B, to demonstrate their respective travel distances.
- 😀 The program needs to simulate the travel of two cars with one implementing the `Runnable` interface and the other using the `Thread` class.
- 😀 The travel simulation involves using a loop that multiplies a counter by 50 at each iteration, calculating the travel distance of the cars.
- 😀 The simulation will run for 10 iterations, incrementing the distance by 50 meters in each iteration, producing a growing output value.
- 😀 The cars' travel distances are calculated and displayed during each loop iteration, with distances increasing in multiples of 50 meters.
- 😀 There is an emphasis on using multithreading to allow the program to simulate car movements simultaneously, enhancing efficiency.
- 😀 The program's output can vary each time due to the random nature of the threads' execution order, with the cars' distances being output in a non-sequential, unpredictable order.
- 😀 The script concludes with a reminder that the program is functioning correctly, producing output based on the threading logic, and the final output will reflect the concurrent behavior of the two cars.
Q & A
What is multithreading and how does it improve program performance?
-Multithreading allows a program to execute multiple tasks simultaneously. This increases the efficiency of the program by making better use of CPU resources, enabling parallel processing and reducing the time required for task completion.
What is the difference between implementing `Runnable` and extending `Thread` in Java?
-When a class implements the `Runnable` interface, it allows for concurrent execution by providing a `run()` method that can be passed to a thread. Extending the `Thread` class, on the other hand, requires directly creating a thread subclass, but `Runnable` is more flexible because a class can implement multiple interfaces, whereas it can only extend one class.
How does the car distance simulation work in the provided program?
-The program simulates the distance traveled by two cars (Car A and Car B) using multithreading. Each car's progress is updated every iteration by multiplying the iteration number by 50 to calculate the distance traveled, and the process is executed asynchronously for both cars.
What does the `run()` method do in the car classes?
-The `run()` method in each car class contains the logic for the car’s travel simulation. It loops through 10 iterations, calculating and printing the distance traveled by the car during each iteration.
Why is multithreading used in the simulation of the two cars?
-Multithreading is used to run the two cars' simulations concurrently, allowing both cars to travel asynchronously and maximizing the program's efficiency. This approach mimics real-world scenarios where multiple tasks can happen at the same time.
How are the threads for Car A and Car B created and executed in the main method?
-In the main method, two `Thread` objects are created, one for each car. Each thread is assigned a `Runnable` task (the car's respective class). The threads are then started using the `start()` method, which initiates the execution of the `run()` method for both cars.
What is the significance of the `for` loop in the `run()` method?
-The `for` loop in the `run()` method controls the number of iterations (10 in this case) during which the distance traveled by each car is calculated. Each loop iteration updates the distance and prints it, simulating the car's progress.
What output can be expected when the program is run?
-The output will display the distances traveled by Car A and Car B, each printed during each iteration. The output may vary in order because the threads run asynchronously, causing the car distances to be printed in a random order.
How does the program handle asynchronous execution in terms of output display?
-Since both threads are running independently, the output is interwoven randomly. For example, Car A’s distance may be printed first, followed by Car B’s, or the print statements may alternate, depending on the timing of thread execution.
What would happen if the loop condition in the `run()` method was changed to `i <= 5`?
-If the loop condition was changed to `i <= 5`, the program would only simulate 5 iterations of distance traveled instead of 10. This would result in fewer output lines, with each car’s travel distance calculated and printed for only 5 iterations.
Outlines
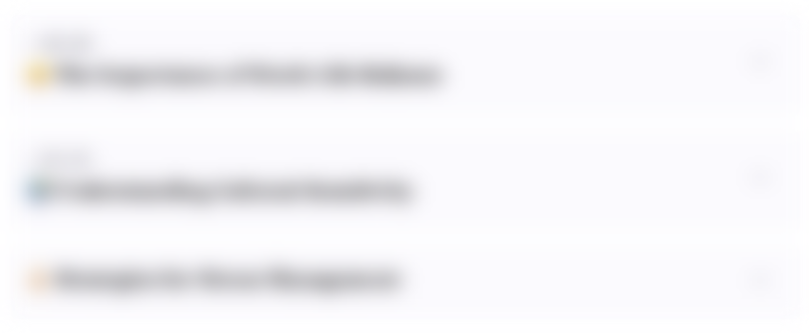
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
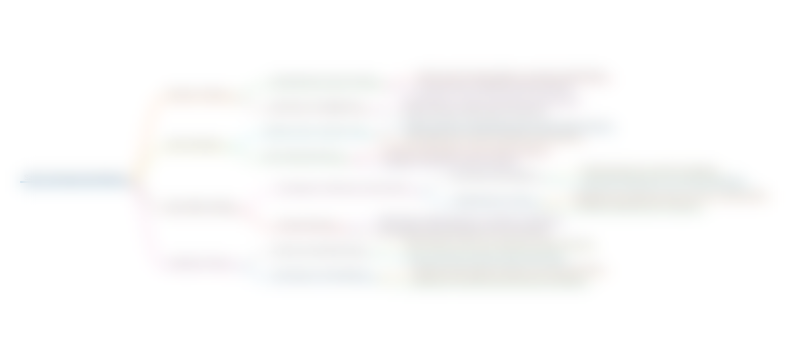
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
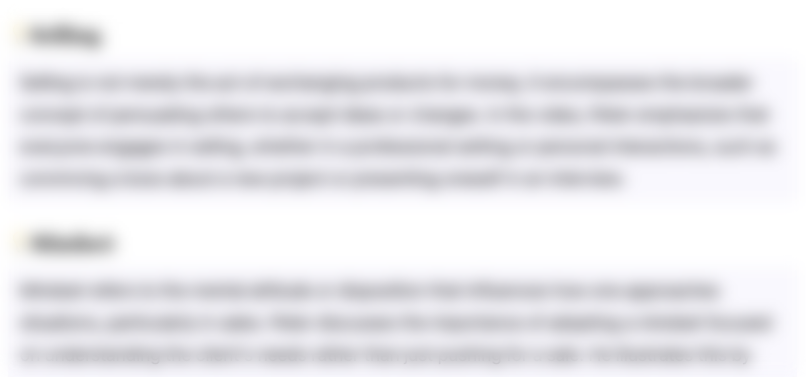
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
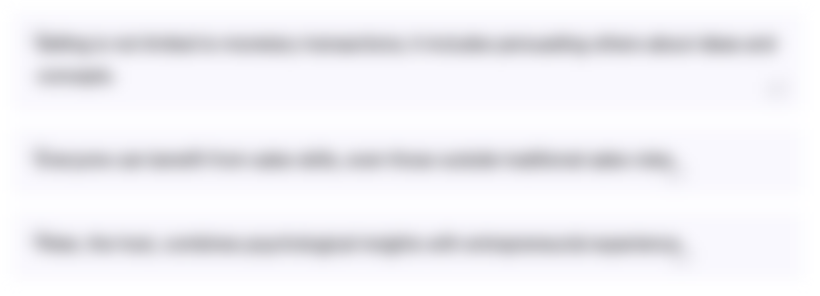
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
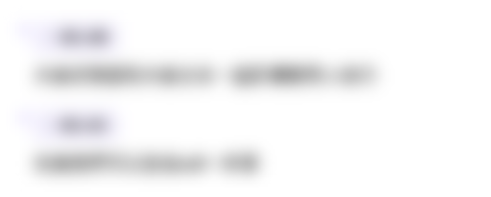
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)