L24. Right/Left View of Binary Tree | C++ | Java
Summary
TLDRIn this video, the presenter discusses how to find the right side view of a binary tree using recursive traversal techniques. Sponsored by Reeval, the segment emphasizes the importance of understanding the last node visible at each level of the tree. By employing a reverse pre-order traversal approach, viewers learn how to capture the rightmost nodes efficiently. The presenter also contrasts this method with level order traversal and highlights time and space complexities. Additionally, the video encourages viewers to attempt the left side view problem independently, reinforcing the concepts through practical coding examples in C++ and Java.
Takeaways
- 😀 Reeval is sponsoring the tree series and offers free registration for a test that opens job opportunities at top startups in India.
- 😀 The right side view of a binary tree includes the nodes visible when viewed from the right side.
- 😀 The nodes visible in the right view of the example tree are 1, 3, 7, and 6.
- 😀 To find the right side view, we need to capture the last node at each level during tree traversal.
- 😀 A reverse pre-order traversal (root, right, left) is an effective method for obtaining the right view.
- 😀 Recursive solutions are preferred in interviews due to their clean and concise code compared to iterative solutions.
- 😀 The recursive function checks if the current level is being visited for the first time to determine if a node should be added to the right view.
- 😀 A dry run illustrates how to traverse the tree and build the right side view effectively.
- 😀 The code implementation in C++ and Java demonstrates how to structure the recursive function for the right side view.
- 😀 The time complexity of the algorithm is O(N) and the space complexity is O(H), where H is the height of the tree.
Q & A
What is the main focus of the video?
-The video focuses on teaching viewers how to find the right side view and left side view of a binary tree, along with the methods for implementing these solutions.
What is the right side view of a binary tree?
-The right side view consists of the nodes that are visible when the tree is viewed from the right. For example, in the provided tree, the right side view includes nodes 1, 3, 7, and 6.
How can we determine which nodes to include in the right side view?
-To determine the right side view, we traverse the binary tree and identify the last node at each level, as these nodes represent what would be visible from the right.
What traversal technique is suggested for finding the right side view?
-The video suggests using reverse pre-order traversal, which visits the nodes in the order of root, right, and then left.
Why is recursive traversal preferred over iterative traversal for this problem?
-Recursive traversal is preferred because it results in shorter and cleaner code, which can be advantageous during interviews.
What are the time and space complexities associated with the recursive solution?
-The time complexity of the recursive solution is O(N), where N is the number of nodes, and the space complexity is O(H), where H is the height of the tree.
How is the data structure used in the recursive function for storing the right view?
-A data structure, such as a vector, is initialized to store the right view nodes. Each time a node is visited at a new level for the first time, it is added to this structure.
What adjustment needs to be made to find the left side view of a binary tree?
-To find the left side view, the traversal order should be changed to left, right instead of right, left.
Can you explain the dry run example provided in the video?
-In the dry run, the traversal starts at the root node (1) and proceeds right (to node 3), adding nodes to the right view as they are the first encountered at each level until reaching node 6.
What should viewers do if they have questions or want to engage with the content?
-Viewers are encouraged to leave comments with their questions or thoughts, especially regarding the implementation of the left side view.
Outlines
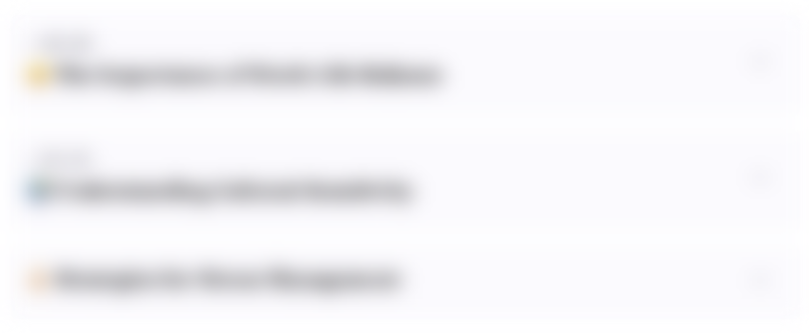
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
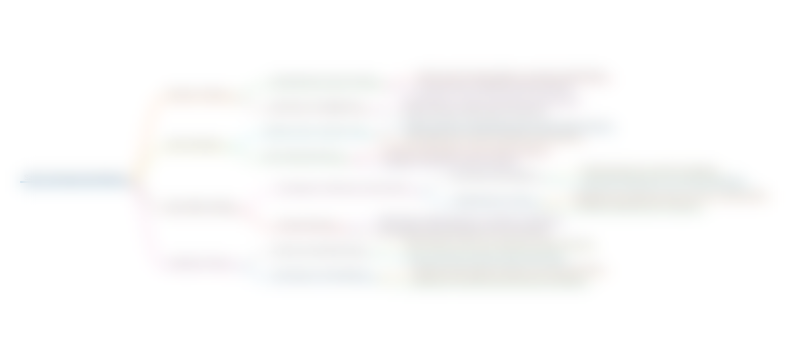
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
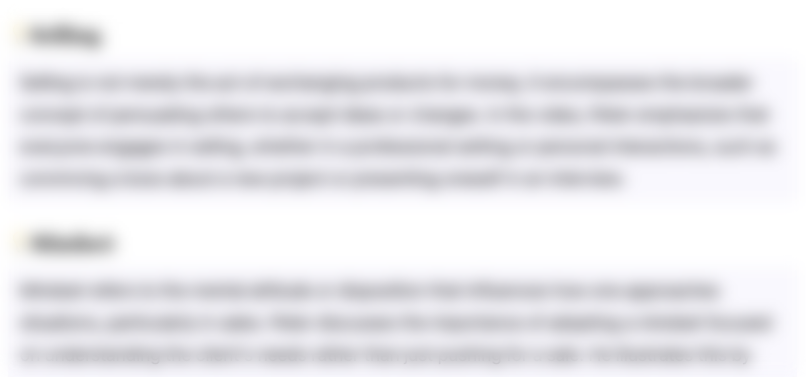
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
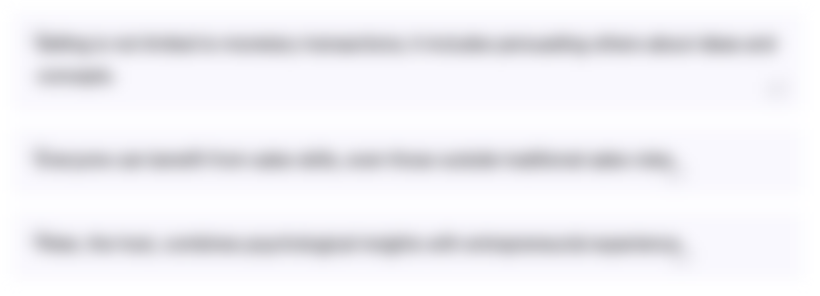
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
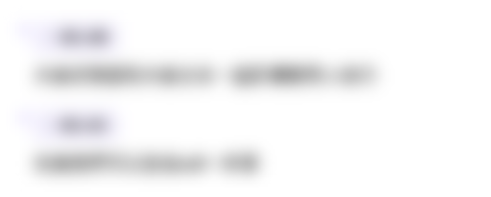
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)