LeetCode Problem: 226. Invert Binary Tree | Java Solution
Summary
TLDRThe video script discusses the concept of an inverted binary tree, explaining the basic structure and properties of binary trees where each node can have at most two children, referred to as left and right. It delves into the process of inverting a binary tree, which involves swapping the left and right children of all nodes. The script provides a step-by-step guide on how to perform the inversion, emphasizing the importance of understanding the tree's depth and structure. It also touches on the coding aspect, suggesting a recursive approach to inverting the tree, and concludes with an example to illustrate the process, aiming to clarify the concept for viewers.
Takeaways
- 🌟 The video discusses the concept of an Inverted Binary Tree, a task where the tree is flipped.
- 📚 A binary tree is defined as a type of tree where each node can have at most two children.
- 🔄 The process of inverting a binary tree involves swapping the left and right children of each node.
- 👀 The video explains that if a tree has a node with three children, it is not a binary tree.
- 💡 The concept of 'left' and 'right' does not apply to nodes with more than two children.
- 🤔 The task is described as being at an 'IQ level difficulty', indicating it is quite challenging.
- 👶 The video starts with the basics, explaining what a binary tree is and its properties.
- 🛠️ The video provides a step-by-step guide on how to invert a binary tree, emphasizing the importance of understanding the structure.
- 👨🏫 The script includes a detailed explanation of the algorithm used to invert the tree, including code snippets.
- 🔧 The video mentions that the root of the tree remains the same during the inversion process.
- 🎓 The video concludes with a demonstration of the complete inverting process, using a specific example to illustrate the steps.
Q & A
What is the main topic of the video script?
-The main topic of the video script is about inverting a binary tree. It explains the concept of a binary tree, how it works, and provides a step-by-step guide on how to invert it.
What is a binary tree as mentioned in the script?
-A binary tree is a type of tree data structure where each node has at most two children, referred to as the left and right child.
Why is the task of inverting a binary tree considered difficult in the script?
-The task of inverting a binary tree is considered difficult because it involves understanding the structure of the tree and recursively swapping the left and right children of each node, which can be complex for beginners.
What does the script mean by 'inverting' a binary tree?
-In the context of the script, 'inverting' a binary tree means to reverse the tree structure by swapping the left and right children of each node, effectively creating a mirror image of the original tree.
How does the script describe the process of inverting a binary tree?
-The script describes the process of inverting a binary tree by first explaining the basic structure of a binary tree, then it walks through a recursive function that swaps the left and right children of each node in the tree.
What is the significance of the 'root' in the context of the binary tree discussed in the script?
-In the context of the binary tree, the 'root' is the starting point of the tree. The script explains that during the inversion process, the root's left and right children are swapped, and this process is recursively applied to all nodes.
What is the role of the 'left' and 'right' children in the inversion process as described in the script?
-The 'left' and 'right' children of each node in the binary tree are crucial in the inversion process. The script explains that during the inversion, the roles of the left and right children are swapped, which is the core operation of inverting the tree.
How does the script handle the case where a node in the binary tree does not have any children?
-The script mentions that if a node does not have any children (referred to as 'null' or 'nil'), then no action is needed for that node during the inversion process, and it is simply returned as is.
What does the script imply by 'swapping' in the context of inverting a binary tree?
-In the context of inverting a binary tree, 'swapping' refers to the process of exchanging the positions of the left and right children of each node in the tree, which is a key step in inverting the tree structure.
What is the final outcome of the inversion process as described in the script?
-The final outcome of the inversion process, as described in the script, is a new binary tree structure that is the mirror image of the original tree, with all left and right children of the nodes swapped.
Does the script provide any tips or tricks to make the inversion process easier?
-The script suggests understanding the basic structure of a binary tree and following a recursive approach to invert the tree. It also emphasizes the importance of correctly identifying and swapping the left and right children at each node.
Outlines
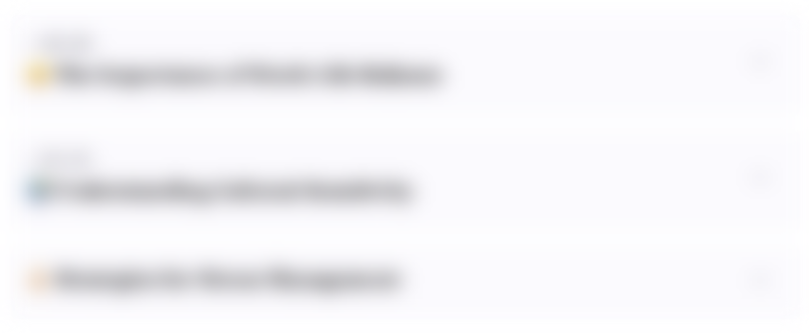
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
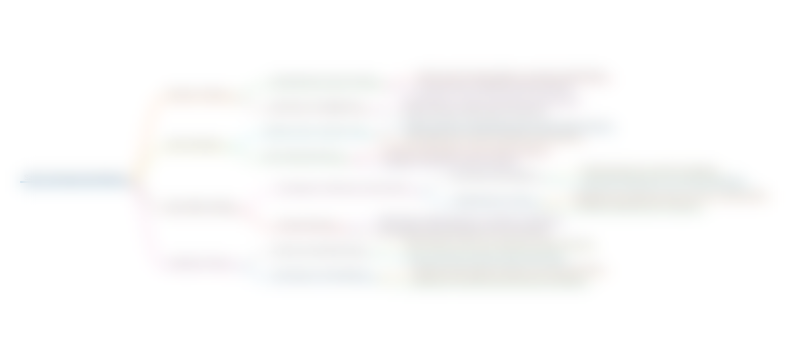
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
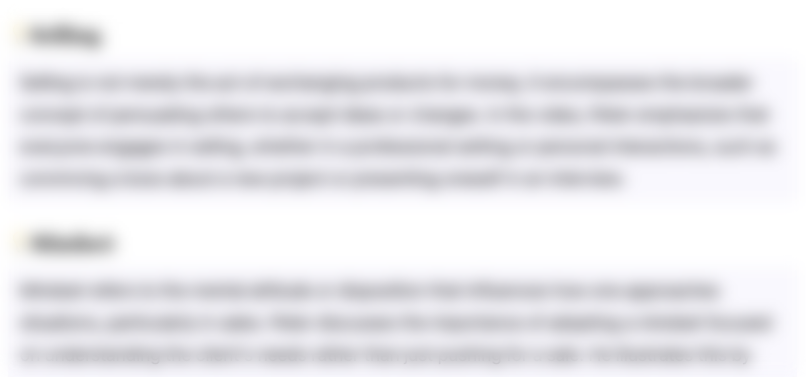
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
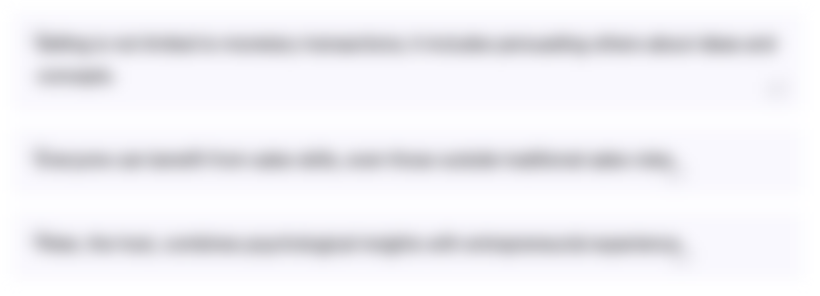
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
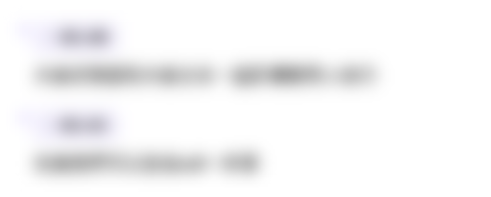
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
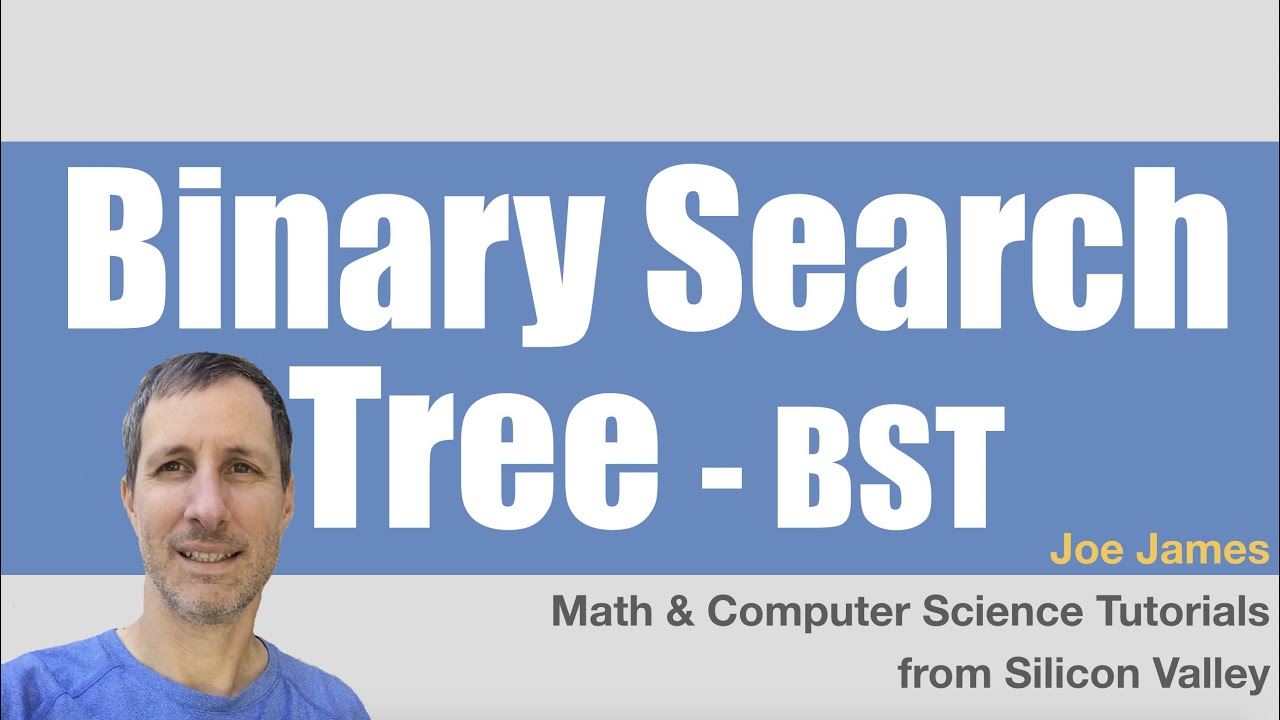
Binary Search Trees (BST) Explained in Animated Demo

90. OCR A Level (H446) SLR14 - 1.4 Data structures part 4 - Trees
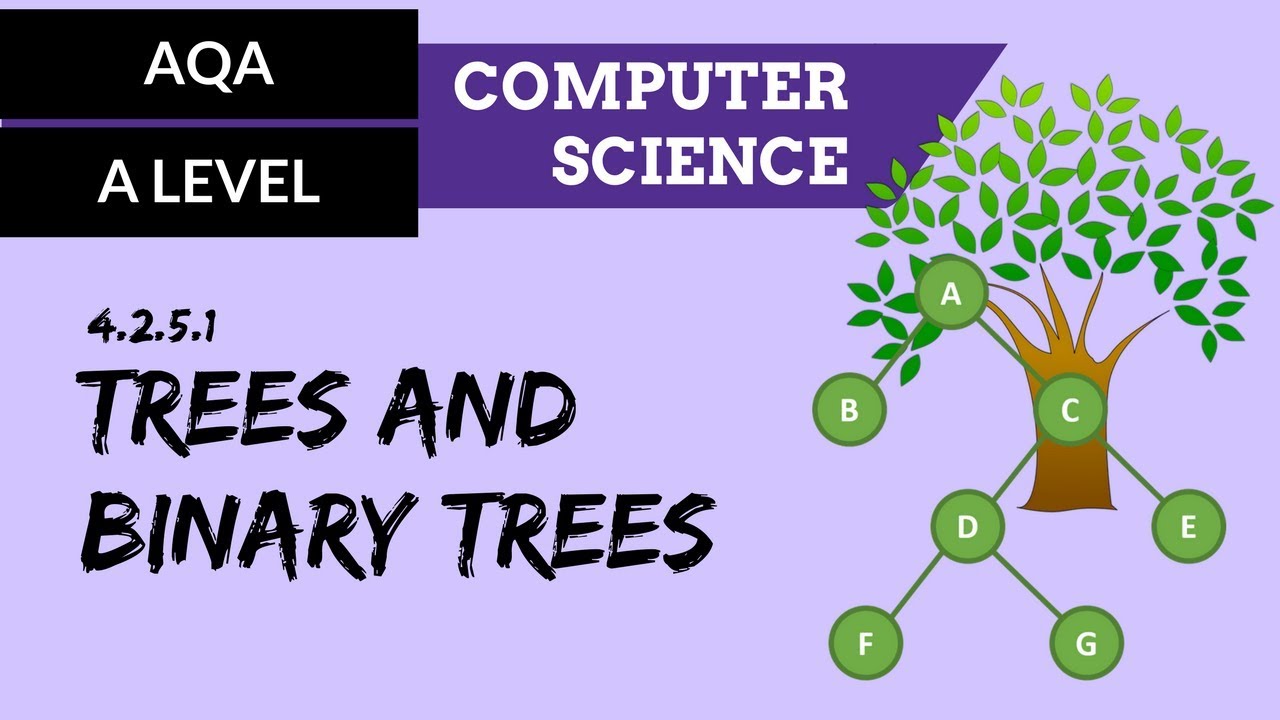
AQA A’Level Trees & Binary trees

L-3.7: Introduction to Trees (Binary Tree, Almost Complete Binary Tree, Full BT, Complete BT, BST)

Balanced Binary Tree - Leetcode 110 - Python
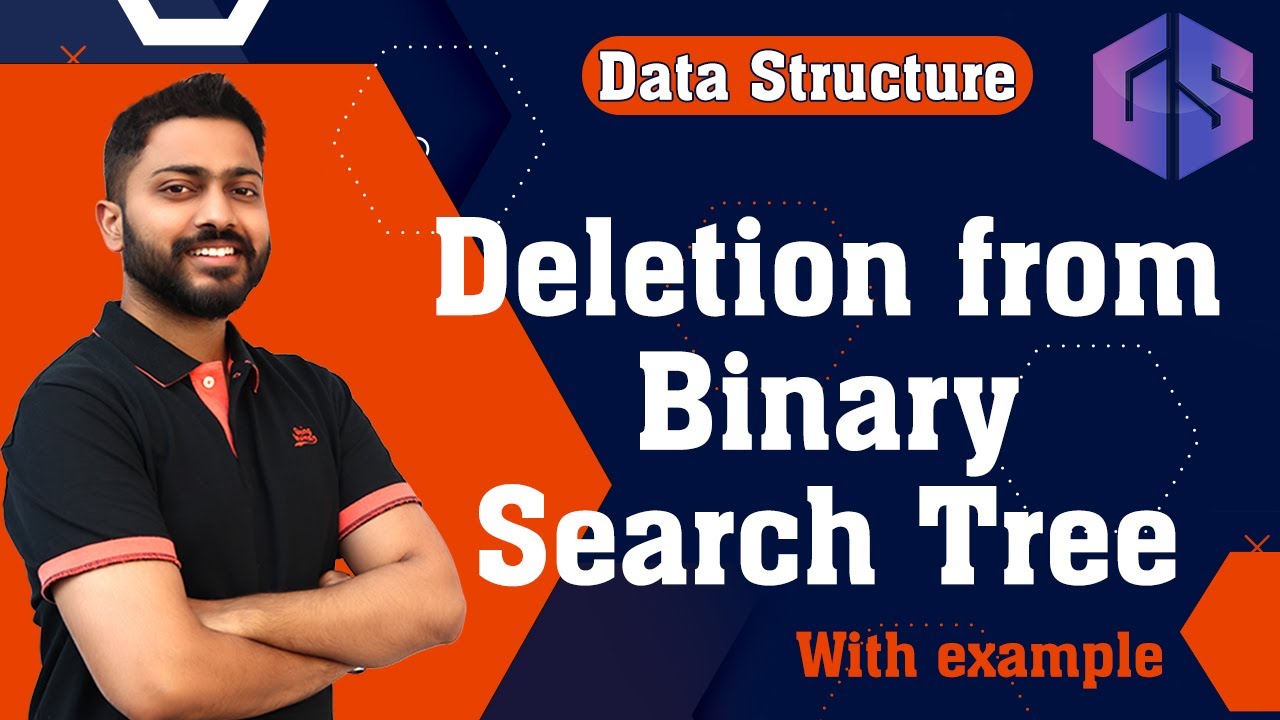
Lec-54: Deletion from Binary Search Tree(BST) with Example | Data Structure
5.0 / 5 (0 votes)