Pointers and arrays
Summary
TLDRThis lesson explores the relationship between pointers and arrays in C and C++. It explains how arrays are stored in memory as consecutive blocks and illustrates pointer arithmetic, showing how to access and manipulate array elements using pointers. The video highlights the equivalence of using array indexing and pointer dereferencing to retrieve values and addresses. It concludes with examples demonstrating how to print addresses and values of array elements, emphasizing the base address concept and the immutability of array names. Future lessons will cover character arrays and passing arrays as function arguments.
Takeaways
- 😀 Pointers and arrays in C/C++ have a strong relationship, allowing for efficient data manipulation.
- 📏 An array is a block of memory consisting of consecutive memory addresses for its elements.
- 💻 When an integer array `A` of size 5 is declared, it creates five integers stored sequentially in memory.
- 🔢 Each integer typically takes up 4 bytes, leading to a predictable memory layout (e.g., `A[0]` at address 200, `A[1]` at 204).
- 🔗 Pointers can hold the address of variables, allowing access to their values through dereferencing.
- ➕ Pointer arithmetic allows for incrementing pointers to navigate through arrays effectively (e.g., `P = P + 1` moves to the next integer).
- 🖥️ Using the array name `A` gives access to the base address, which can be utilized in pointer expressions.
- 🎯 The expressions `A[i]` and `*(A + i)` are equivalent for retrieving values at index `i` in an array.
- 📌 The address of an element can be accessed using either `&A[i]` or `A + i`, showcasing the interchangeable nature of array and pointer notation.
- 🚫 While pointer variables can be incremented, the array name itself cannot be changed (e.g., `A++` will cause a compilation error).
Q & A
What is the relationship between pointers and arrays in C/C++?
-Pointers and arrays are closely related; the name of an array acts as a pointer to its first element, enabling direct memory access.
How is an array declared in C/C++?
-An array is declared by specifying its type and size, for example, 'int A[5];' creates an integer array of size 5.
What happens to memory when an array is declared?
-When an array is declared, a block of memory is allocated to hold its elements consecutively, based on the size and type of the array.
If an integer array A starts at address 200, what are the addresses of its elements?
-The addresses of the elements would be: A[0] at 200, A[1] at 204, A[2] at 208, A[3] at 212, and A[4] at 216, assuming each integer takes 4 bytes.
What is pointer arithmetic, and how is it used with arrays?
-Pointer arithmetic allows you to perform operations like incrementing or decrementing pointers. For instance, if P points to A[0], then P + 1 points to A[1].
How can you access the value of an array element using a pointer?
-You can access the value of an array element using pointer dereferencing. For example, if P points to A, then *P retrieves the value of A[0].
What does the expression A[i] represent in terms of pointers?
-The expression A[i] is equivalent to *(A + i), meaning it retrieves the value at index i of the array by dereferencing the pointer to that location.
Can the base address of an array be modified, and why?
-No, the base address of an array (represented by its name) cannot be modified, as it is a constant pointer to the first element of the array.
What is the difference between using A and &A[0]?
-Using A gives the address of the first element of the array, while &A[0] explicitly retrieves the address of the first element; both expressions yield the same address.
What are the implications of incrementing a pointer versus the array name?
-Incrementing a pointer variable is valid and changes where the pointer points, while incrementing the array name itself results in a compilation error, as it cannot change its base address.
Outlines
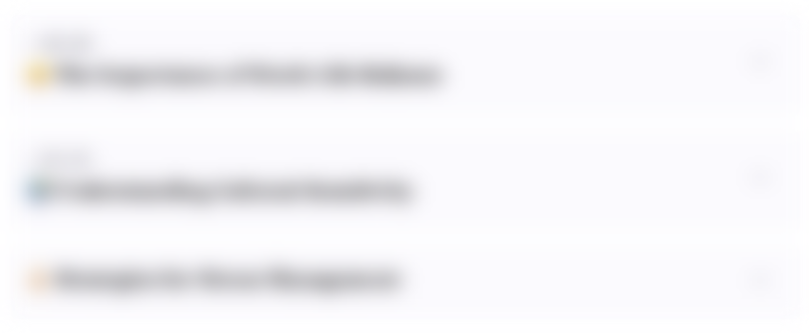
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
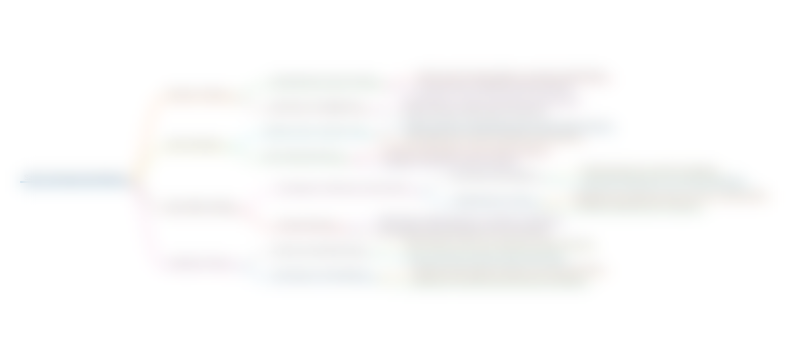
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
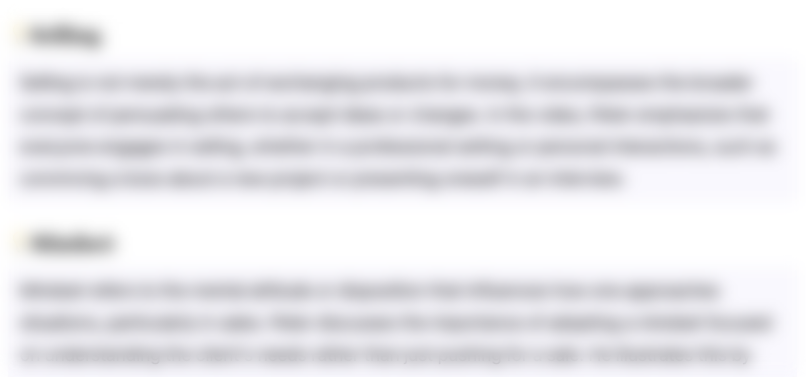
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
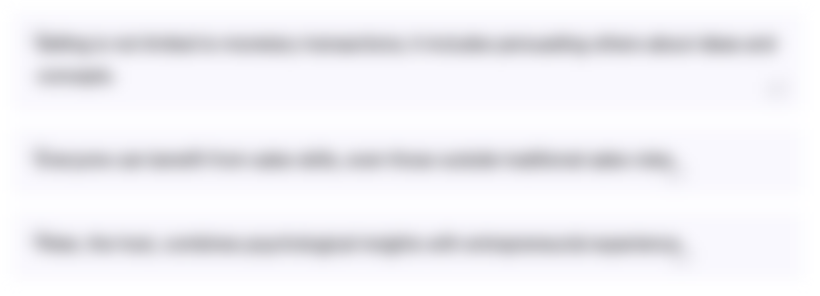
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
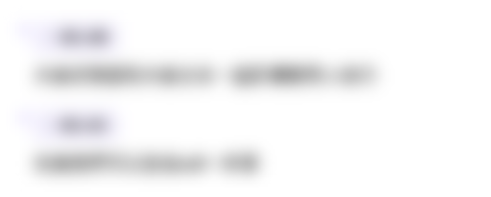
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
5.0 / 5 (0 votes)