31 nooby C++ habits you need to ditch
Summary
TLDRThis video highlights 31 common mistakes made by beginners in C++. It covers bad practices such as using 'namespace std' globally, misusing 'std::endl' in loops, and relying on C-style arrays instead of standard arrays. The video emphasizes the importance of using standard algorithms, proper casting, const correctness, and structured bindings. It also warns against unnecessary heap allocations, ignoring compiler warnings, and misunderstanding smart pointers. The aim is to help new programmers write more efficient, readable, and maintainable C++ code.
Takeaways
- ๐ Avoid using 'using namespace std;' globally, especially in header files, as it can lead to namespace pollution and force this choice on users of your code.
- ๐ Prefer using a simple newline character over 'std::endl' in loops to avoid unnecessary buffer flushing, which can degrade performance.
- ๐ Opt for range-based for loops when the index is not needed, reducing the chance of off-by-one errors and improving readability.
- ๐ Utilize standard algorithms like 'std::find_if' instead of writing custom loops for simple tasks, leveraging the power of the C++ Standard Library.
- ๐ Use standard arrays instead of C-style arrays to benefit from automatic management of array length and to avoid pointer decay.
- โ ๏ธ Be cautious with 'reinterpret_cast' as it can lead to undefined behavior; use 'bit_cast' in C++20 for safer type reinterpretation.
- ๐ซ Refrain from casting away 'const' unnecessarily, as it can lead to errors and misunderstandings about object mutability.
- ๐ Understand that 'operator[]' in maps can insert elements if they do not exist, which might be an unintended side effect when just trying to access an element.
- ๐ Prioritize 'const correctness' to ensure functions do not modify objects they should not, and communicate this intent clearly to the users of your code.
- ๐ฏ Know about string literal lifetimes; they are valid for the duration of the program, allowing for safe references to them even when returning from functions.
- ๐ Embrace structured bindings for cleaner and more readable code when dealing with pairs or tuples, making it easier to work with complex data structures.
Q & A
Why is 'using namespace std' considered a bad habit, especially at the global level?
-'using namespace std' is considered a bad habit because it can lead to name collisions and make it unclear where certain functions or objects are coming from. Using it at the global level can force this choice on everyone who uses your code, especially if done in a header file.
What is the main issue with using 'std::endl' in a loop?
-Using 'std::endl' in a loop is inefficient because it not only adds a new line but also flushes the output buffer, which can significantly slow down the program. It's better to use a newline character '\n' instead.
Why is it preferable to use range-based for loops over index-based for loops in C++?
-Range-based for loops are preferable because they reduce the risk of off-by-one errors and accidental typos related to index manipulation. They also make the code more expressive by focusing on the elements rather than the indices.
What advantage does using standard algorithms like 'std::find_if' have over writing custom loops?
-Standard algorithms like 'std::find_if' are generally more optimized, tested, and expressive. They make the code more readable and reduce the chance of bugs since you're reusing well-known, tested code.
Why should one avoid using C-style arrays in C++?
-C-style arrays should be avoided because they decay into pointers and require explicit management of the array length, increasing the risk of errors. Using standard arrays or vectors provides safer and more convenient alternatives.
What is the problem with using 'reinterpret_cast' in C++?
-'reinterpret_cast' can lead to undefined behavior unless cast back to the original type. Most uses of 'reinterpret_cast' are dangerous, and safer alternatives often exist. For instance, 'bit_cast' in C++20 offers a safer way to reinterpret the bytes of an object.
Why is it important to understand the difference between default and value initialization?
-Understanding the difference is crucial because default initialization leaves variables with indeterminate values, while value initialization guarantees they are set to zero or their default values. This knowledge helps prevent bugs related to uninitialized variables.
How can ignoring const correctness lead to issues in C++ programs?
-Ignoring const correctness can lead to unintended modifications of data, making the code less predictable and harder to debug. Marking parameters and methods as 'const' where appropriate ensures that they do not modify the data, leading to safer and more reliable code.
What is the danger of adding or removing elements from a container while iterating over it?
-Adding or removing elements from a container while iterating over it can invalidate iterators and cause undefined behavior. This often happens because operations like 'push_back' can reallocate the container, making previously valid iterators invalid.
Why is it recommended to use smart pointers like 'unique_ptr' and 'shared_ptr' over raw pointers?
-Smart pointers like 'unique_ptr' and 'shared_ptr' manage the memory automatically, ensuring that resources are properly cleaned up when they are no longer needed. This helps prevent memory leaks and other resource management issues.
Outlines
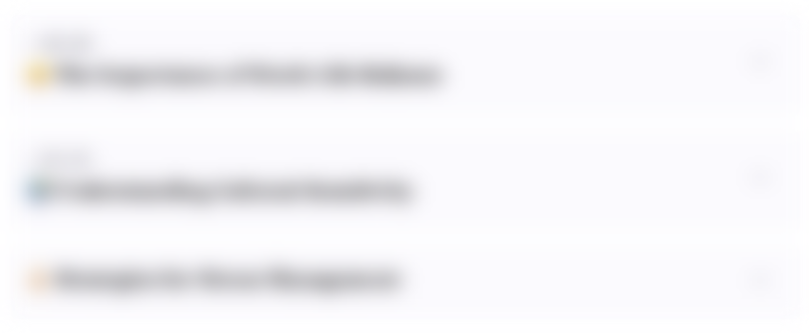
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
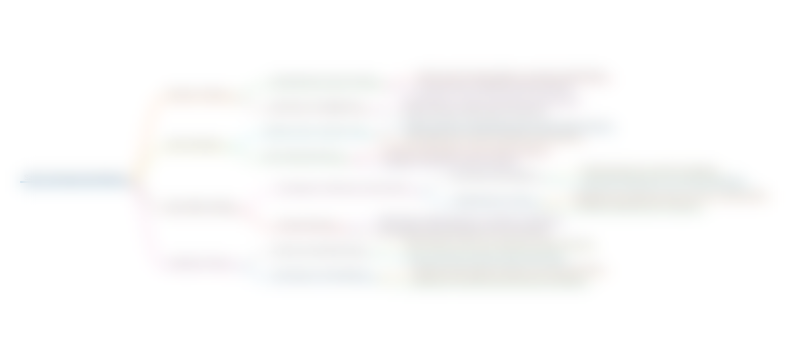
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
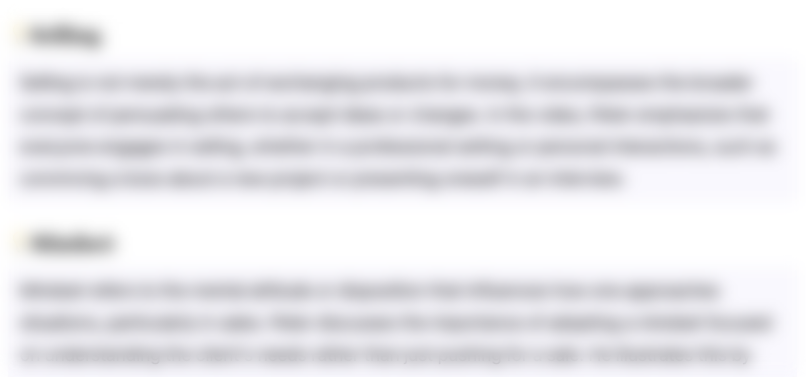
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
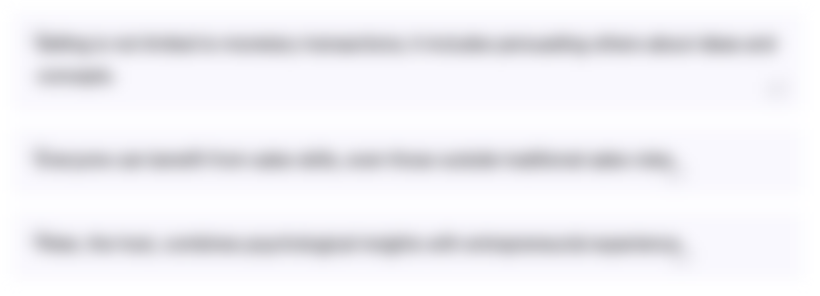
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
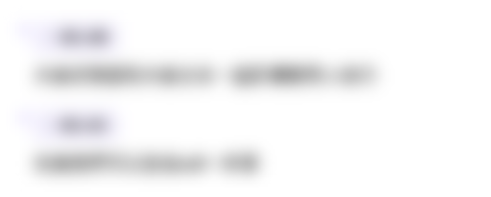
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
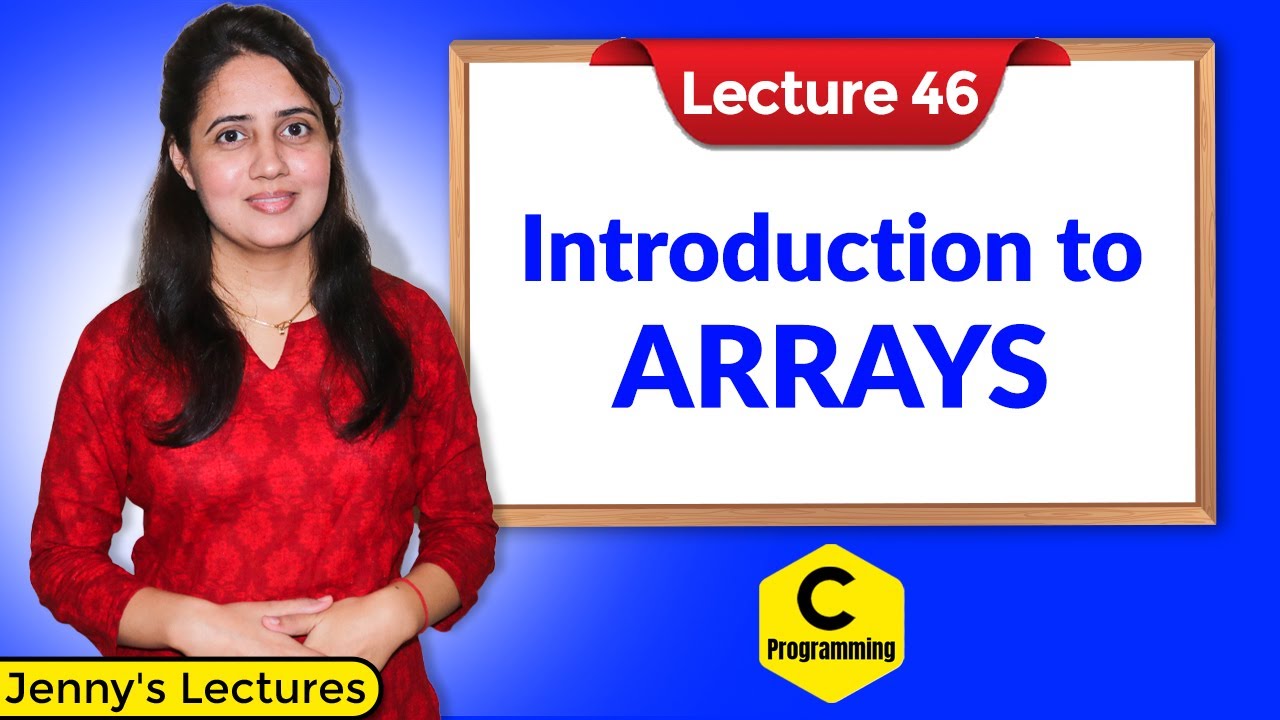
C_46 Arrays in C - part 1 | Introduction to Arrays
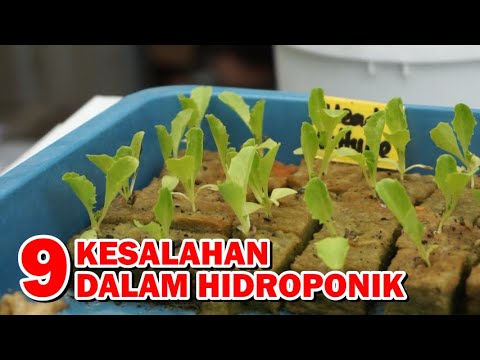
9 KESALAHAN YANG SERING DILAKUKAN OLEH PETANI HIDROPONIK PEMULA
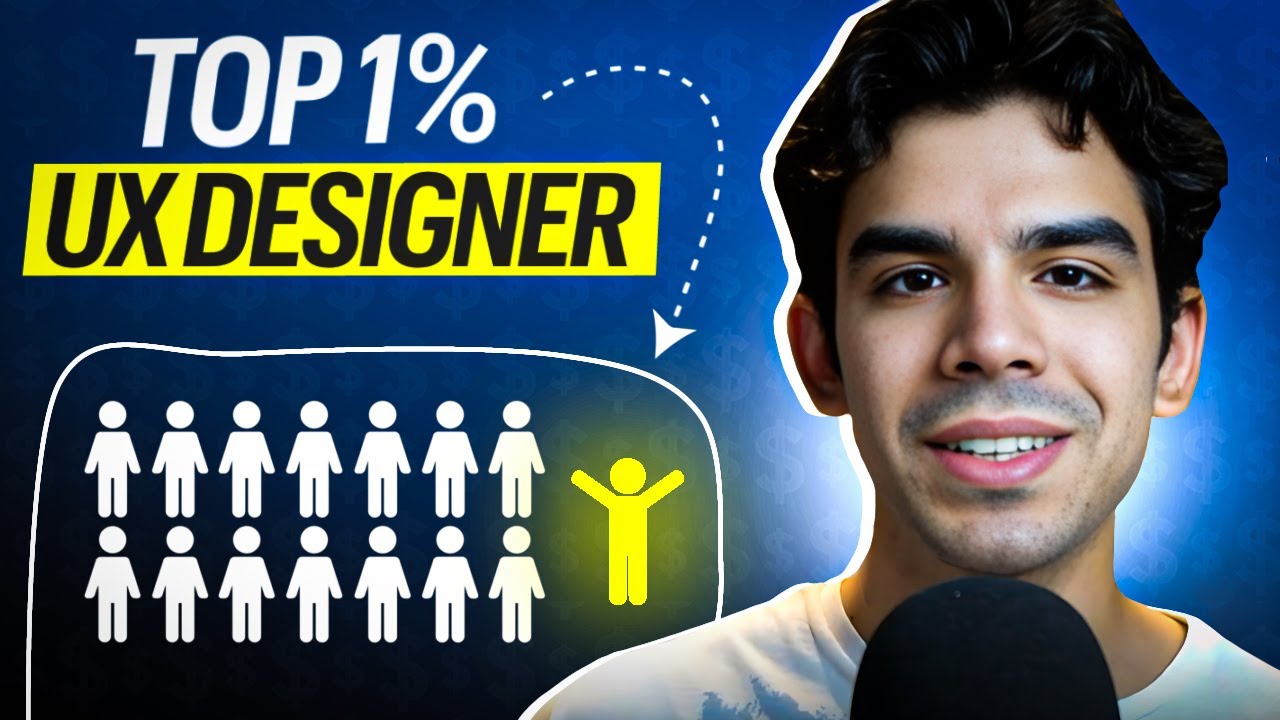
How to get ahead of 99% of Product Designers (Starting today)

10 KESALAHAN PEMULA DALAM LATIHAN BEBAN
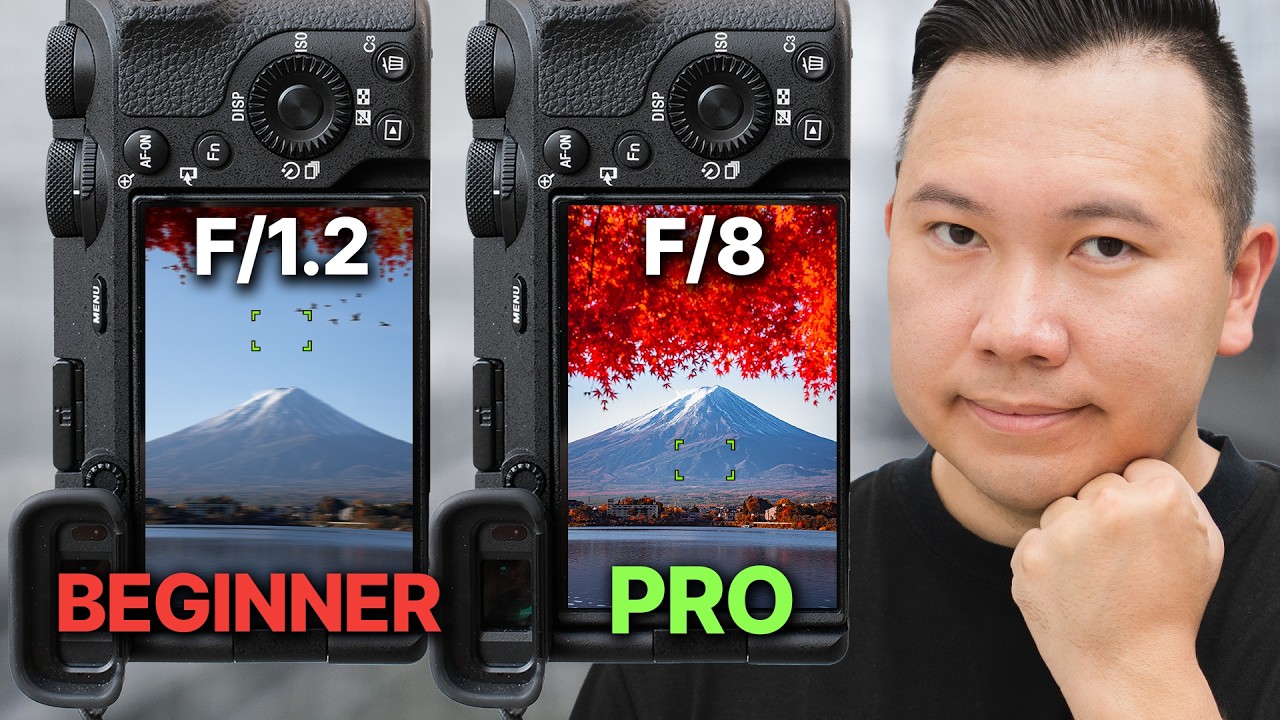
7 Photography Mistakes I See All Beginners Make
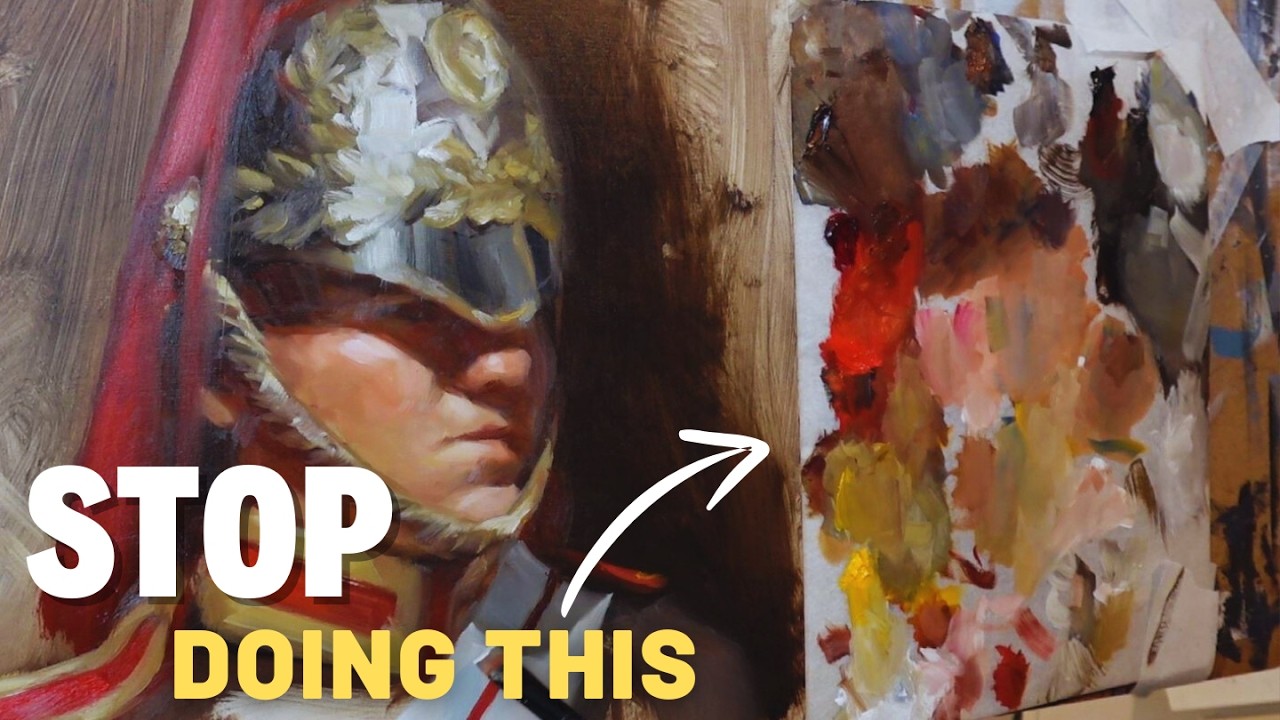
8 Things I Wish I Knew as a Beginner Oil Painter
5.0 / 5 (0 votes)