Precedence and Associativity of Operators
Summary
TLDRThis lecture delves into the critical concepts of operator precedence and associativity in programming. It explains how precedence determines the order of evaluation when multiple operators are present, using examples like multiplication vs. addition. The lecture then clarifies associativity, which comes into play when operators share the same precedence, and it decides the direction of evaluation (left-to-right or right-to-left). The instructor uses a table to illustrate various operators' precedence and associativity, emphasizing the importance of understanding these concepts for correct program behavior. The lecture concludes with a homework problem to test comprehension.
Takeaways
- 🔢 **Operator Precedence**: Determines the order in which operators in an expression are evaluated, with higher precedence operators being evaluated first.
- 🔄 **Multiplication vs. Addition**: Multiplication has higher precedence than addition, so it is evaluated first in expressions.
- 🔁 **Associativity**: Comes into play when operators have the same precedence, deciding whether operations are performed left-to-right or right-to-left.
- 🔄 **Division and Multiplication**: Both have the same precedence, so their order is determined by associativity, which is left-to-right for these operators.
- 📚 **Precedence Table**: A reference that lists operators by their level of precedence, from highest (at the top) to lowest (at the bottom).
- 👥 **Parentheses**: Have the highest precedence and are used to explicitly specify the order of operations in an expression.
- 🔄 **Postfix vs. Prefix**: Postfix increment/decrement operators have higher precedence than prefix ones, and their associativity is left-to-right, unlike prefix which is right-to-left.
- 🔄 **Associativity of Unary Operators**: Unary operators, like prefix and postfix, have right-to-left associativity, which is different from most other operators.
- ❗ **Undefined Behavior**: The order in which functions are called when there's only one operator (like assignment) is not defined and can vary by compiler.
- 📝 **Homework Problem**: A reminder that understanding precedence and associativity is crucial for predicting the behavior of complex expressions in programming.
Q & A
What is the precedence of operators and how does it affect expression evaluation?
-Operator precedence determines the order in which operators in an expression are evaluated when multiple operators are present. An operator with higher precedence is evaluated before an operator with lower precedence.
Can you provide an example to illustrate the concept of operator precedence?
-Yes, in the expression 5 + 3 * 2, the multiplication operator (*) has higher precedence than the addition operator (+), so 3 * 2 is evaluated first resulting in 6, and then 5 + 6 equals 11.
What is the associativity of operators?
-Associativity of operators comes into play when the precedence of the operators is the same. It determines whether an expression involving operators of the same precedence is evaluated from left to right or right to left.
How does the associativity of division and multiplication affect the evaluation of an expression?
-Division and multiplication have the same precedence, so the associativity determines the order of evaluation. If associativity is left to right, division is performed first followed by multiplication.
What is the significance of parentheses in an expression?
-Parentheses have the highest precedence among all operators and are used to explicitly specify the order of operations in an expression, overriding the default precedence and associativity rules.
Why are member access operators mentioned in the script, and what is their role?
-Member access operators are used to access members of structures and are mentioned to highlight their precedence, which is higher than that of the assignment operator.
What is the difference between postfix and prefix increment/decrement operators in terms of precedence and associativity?
-Postfix increment/decrement operators have higher precedence than prefix increment/decrement operators. Also, the associativity of postfix operators is from left to right, whereas for prefix operators it is from right to left.
How does the precedence and associativity of operators affect the evaluation of a program?
-The precedence and associativity of operators dictate the order in which expressions are evaluated, which can significantly affect the outcome of a program. Understanding these concepts is crucial for predicting and controlling program behavior.
What is the associativity of the assignment operator, and why is it important?
-The associativity of the assignment operator is from right to left, which is important for understanding how compound assignments are evaluated in expressions.
What does it mean when the script says that the behavior of calling functions in an expression is undefined?
-It means that if two functions are called in an expression separated by an operator with which they both associate, the order in which the functions are called is not specified by the language standard, and can vary between different compilers or even different runs with the same compiler.
How can understanding precedence and associativity help in writing clearer code?
-Understanding precedence and associativity helps in writing clearer code by allowing developers to use parentheses effectively to avoid ambiguity and to ensure that expressions are evaluated in the intended order.
Outlines
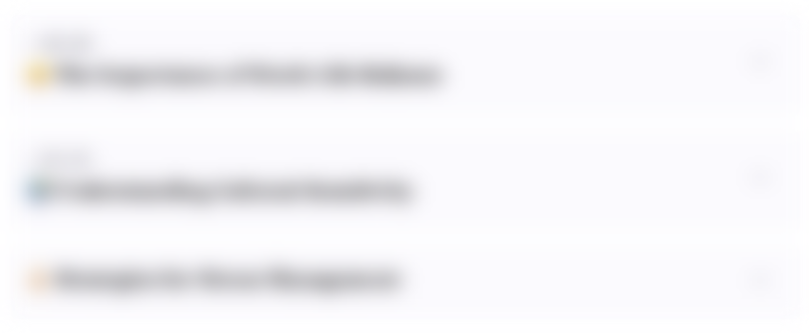
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
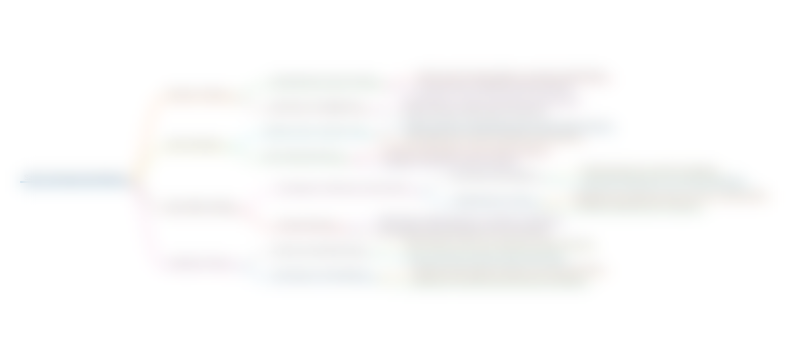
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
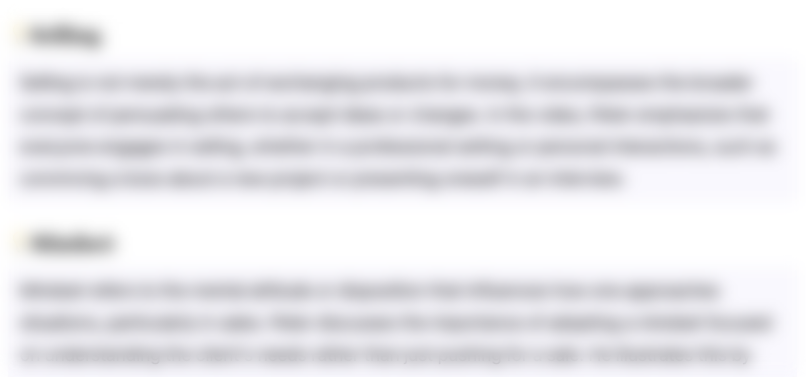
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
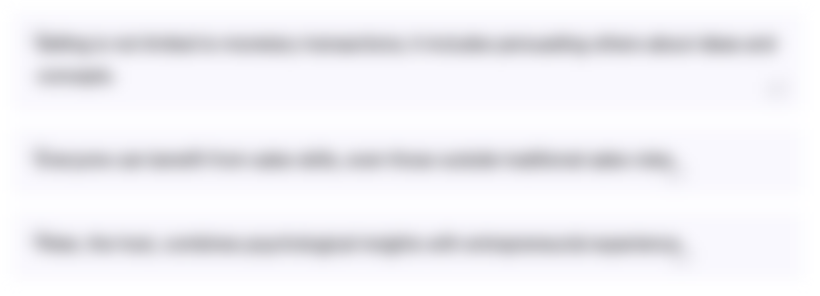
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
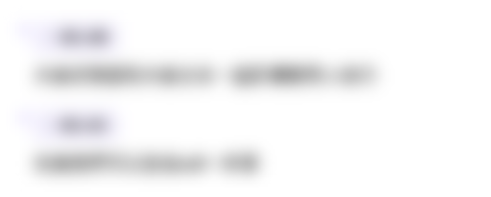
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)