The purest coding style, where bugs are near impossible
Summary
TLDRThis script introduces functional programming, a paradigm that emphasizes the use of pure functions, immutability, and higher-order functions to create a more declarative, deterministic, and maintainable code. It explains concepts like closures, currying, and the benefits of a functional approach, such as reduced side effects and improved code modularity, while acknowledging its complexity and the learning curve involved.
Takeaways
- 🌳 **Programming Paradigms**: Functional programming is a paradigm often used without awareness, branching from the declarative side of the programming language tree.
- 💡 **Core Concepts**: Functional programming emphasizes the use of functions, immutability, pure code, and the avoidance of side effects to create a more predictable and maintainable code structure.
- 🔄 **Closures**: Closures are functions that remember their scope, allowing access to parent function's data even after the parent function has finished executing, which is crucial for functional programming.
- 📈 **Higher Order Functions**: Functions that operate on other functions, such as filter(), sort(), and map(), are fundamental in functional programming, promoting reusable and composable code modules.
- 🛡️ **Immutability**: By avoiding changes to data, functional programming aims to eliminate side effects, ensuring the same output for the same input, which simplifies reasoning about code behavior.
- 🗝️ **Currying**: This technique involves breaking down a function with multiple arguments into a sequence of functions, each with a single argument, enhancing code flexibility and reusability.
- 📦 **Encapsulation**: Using closures to encapsulate data and state can mimic object-oriented programming principles, providing private data scopes and controlled access through returned closures.
- 🚀 **Optimization and Performance**: Functional programming can lead to optimizations like lazy evaluation and automatic parallelization due to its declarative nature and emphasis on immutability.
- 🤔 **Learning Curve**: While functional programming offers many benefits, it also presents challenges such as a steeper learning curve and potential difficulties in optimization compared to imperative styles.
- 🌟 **Benefits**: Adopting functional programming principles leads to more modular, readable, and maintainable code, although it may require a shift in mindset from imperative programming practices.
- 🌐 **Community and Tools**: The video script mentions the RUNME extension for VS Code, showcasing the community's efforts to create tools that blend functional programming concepts with practical development environments.
Q & A
What is functional programming?
-Functional programming is a paradigm that emphasizes structuring code and ensuring immutability. It revolves around the use of functions in a way where data is immutable and functions aim to have no side effects, making the code more predictable and easier to reason about.
How does functional programming differ from other programming paradigms?
-Functional programming differs by focusing on pure functions, immutability, and avoiding side effects, compared to object-oriented programming which organizes code around objects, or procedural programming that structures code as a sequence of steps to be carried out.
What are closures in functional programming?
-Closures are functions that can access and remember the scope around them even after the parent function has finished executing. This allows them to capture and maintain any local variables that were in scope at the time of their creation, enabling data encapsulation and higher order functions.
What role do higher-order functions play in functional programming?
-Higher-order functions are functions that take other functions as arguments or return them as results. They are crucial in functional programming for creating reusable and modular code, enabling operations like filter, sort, and map, which help write code in a declarative manner.
How does functional programming achieve immutability?
-Functional programming achieves immutability by avoiding changes to state or data after its creation. Instead of modifying existing data, operations in a functional paradigm produce new data structures based on existing ones, ensuring that functions have no side effects.
What is currying in functional programming?
-Currying is a technique in functional programming where a function with multiple arguments is transformed into a sequence of functions, each with a single argument. This utilizes closures to remember arguments provided in previous calls until all have been supplied, facilitating function composition.
Why would a developer choose the functional programming paradigm?
-A developer might choose functional programming to benefit from its emphasis on immutability, pure functions, and avoiding side effects, which can lead to more predictable, readable, and maintainable code. It also helps in writing code that is easier to debug and test.
What are the core values of purely functional programming?
-The core values of purely functional programming include declarative and deterministic code that is unchanging, with a strong emphasis on immutability, type safety, and the avoidance of side effects, drawing from mathematical principles to ensure predictability and reliability in code execution.
How does functional programming handle data manipulation?
-In functional programming, data manipulation is handled by creating new data structures from existing ones rather than altering the original structures. This is done to maintain immutability and prevent unintended side effects, ensuring that functions always produce the same output for the same input.
What is the significance of the quote 'A closure is a poor man's object, and an object is a poor man's closure'?
-This quote highlights the conceptual overlap between closures in functional programming and objects in object-oriented programming. It suggests that closures can encapsulate data and functionalities similar to objects, and vice versa, each serving as a fundamental tool in their respective paradigms for managing state and behavior.
Outlines
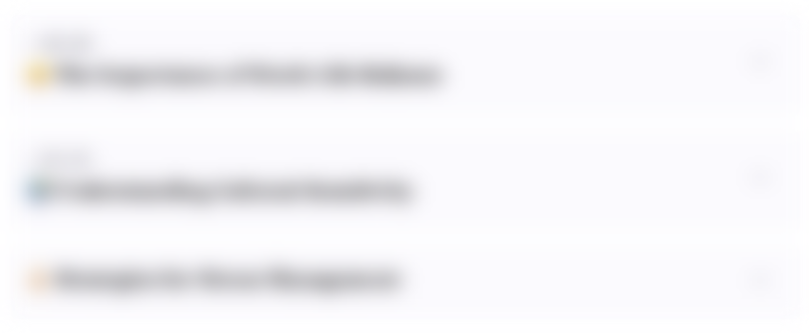
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
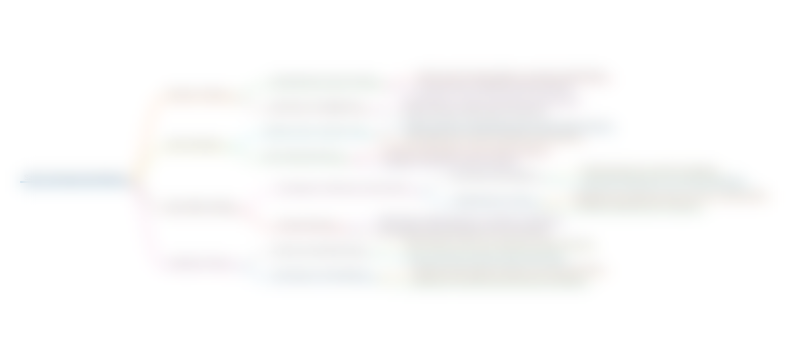
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
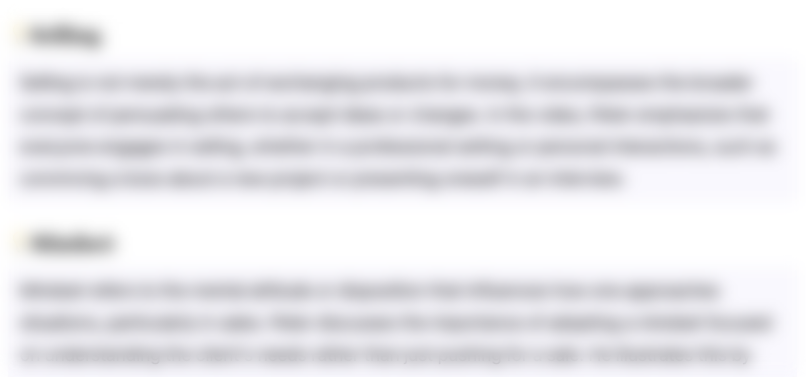
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
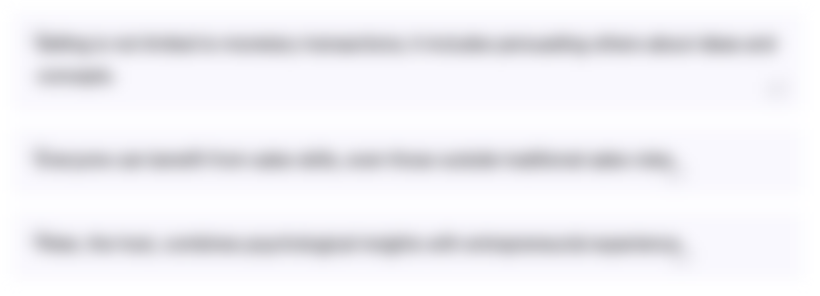
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
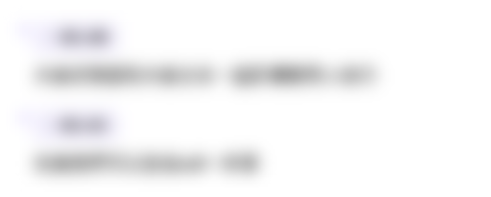
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

O que você não sabia sobre Funções: o coração da Programação Funcional
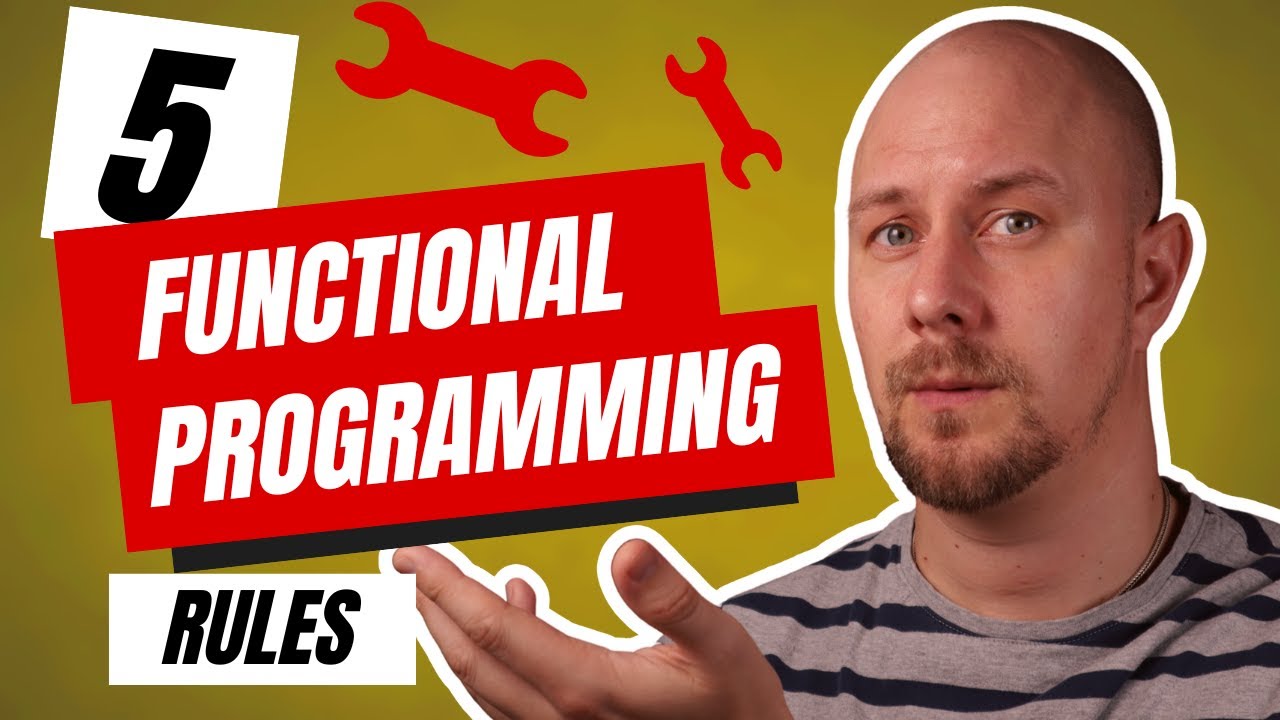
Follow These 5 Functional Programming Rules For Better Code
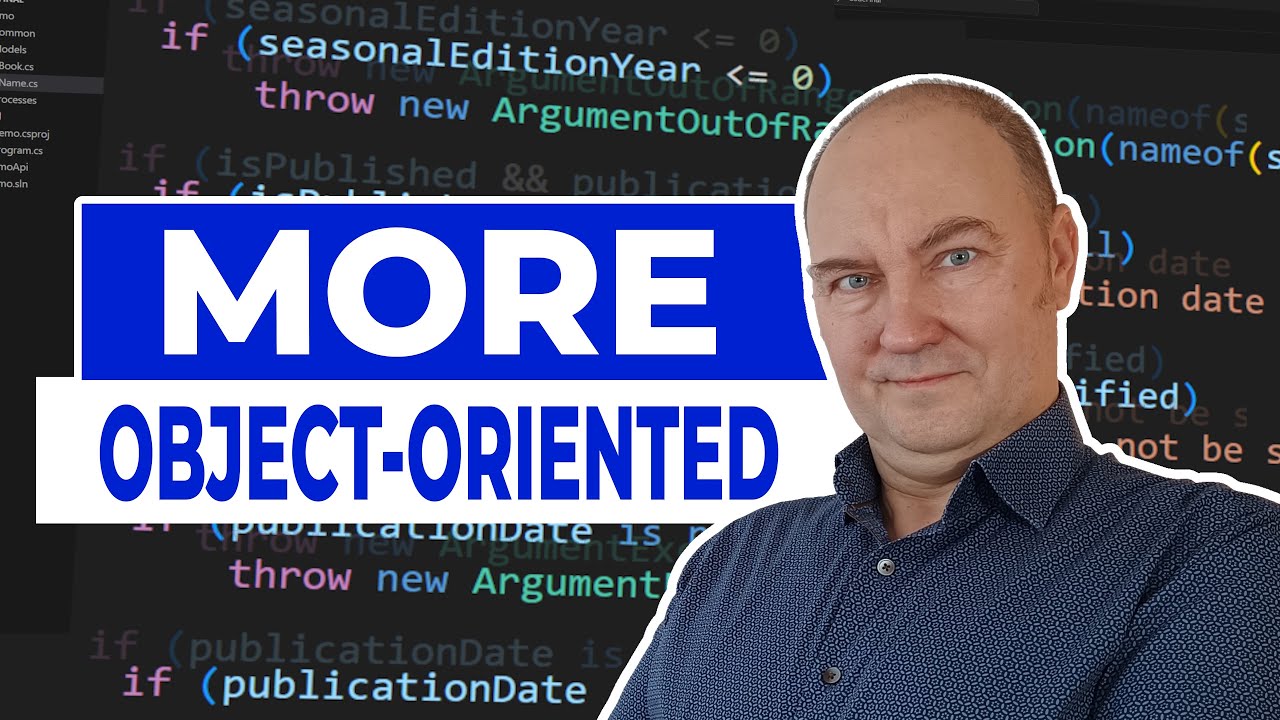
Make Your Object Model More Object-Oriented
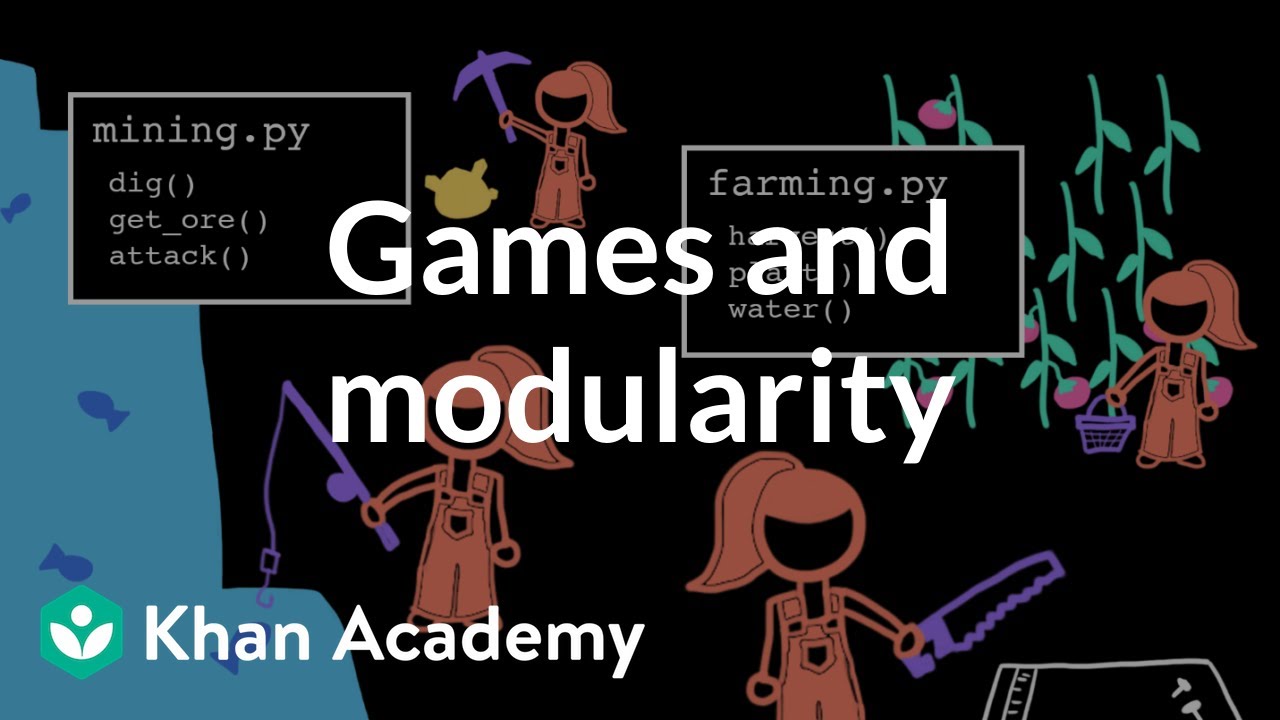
Games and modularity | Intro to CS - Python | Khan Academy
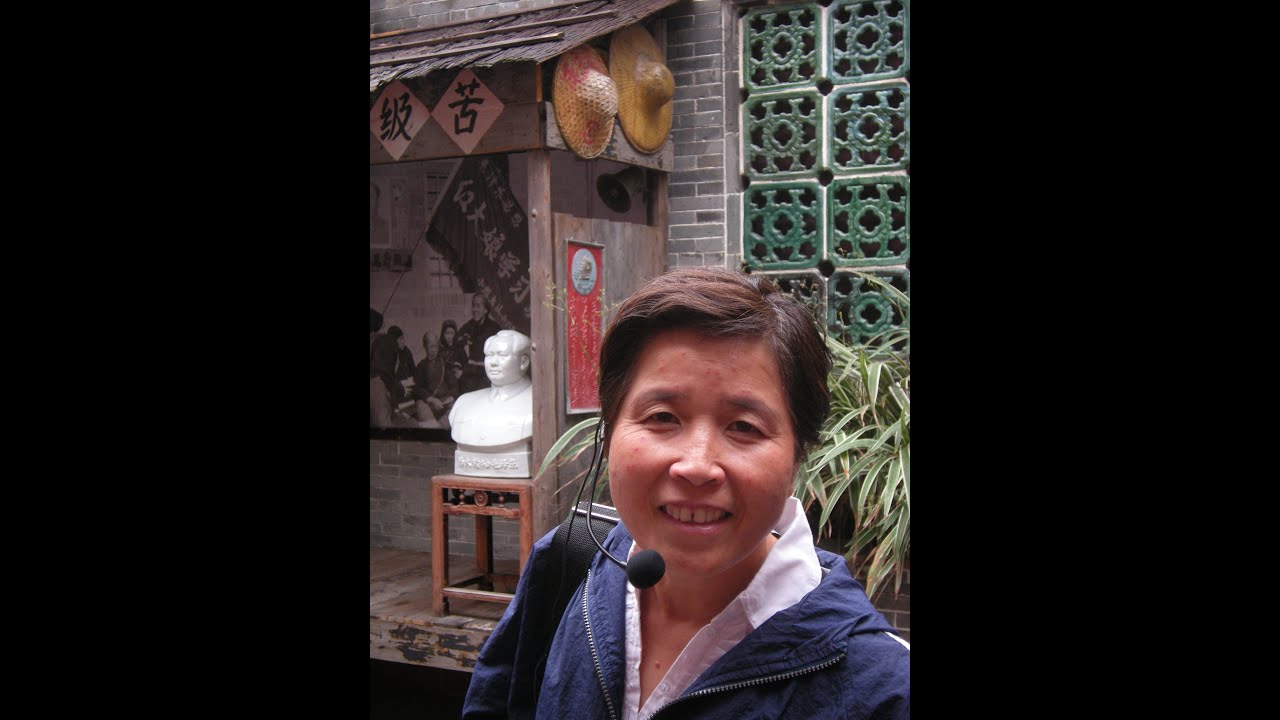
C++ programming call functions to input 2 numbers, calculate and display the sum and differences.
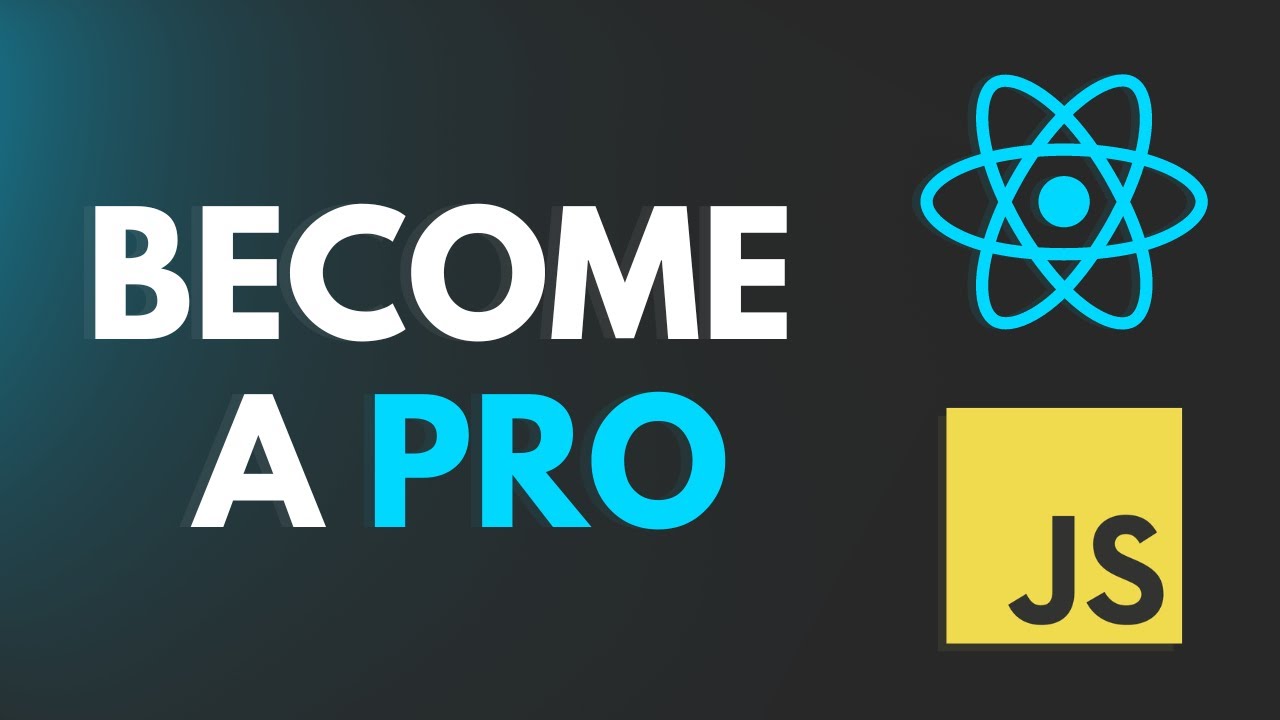
3 JavaScript Concepts that Will Make You A Better React Developer
5.0 / 5 (0 votes)