Every React Concept Explained in 12 Minutes
Summary
TLDRThe video script introduces key concepts of React, a popular JavaScript library for building user interfaces. It explains components as the fundamental building blocks and delves into JSX, a syntax extension for JavaScript. The script covers the use of props for passing data and the importance of the virtual DOM for efficient rendering. It also touches on state management with hooks like useState and the concept of controlled components. Additionally, it highlights the role of event handling, the use of React fragments, and the significance of purity in components. The script concludes with an overview of advanced features like context, portals, suspense, and error boundaries, emphasizing their importance in creating robust and dynamic applications.
Takeaways
- 🧱 Components are the fundamental building blocks of a React app, analogous to Lego bricks, and can be reused to construct various UI elements like buttons, inputs, and pages.
- 📝 React components are JavaScript functions that return JSX, which is a syntax extension for JavaScript, allowing developers to write HTML-like code within JavaScript.
- 🔧 Attributes in JSX are written in camelCase, as opposed to the kebab-case used in HTML, meaning 'class' becomes 'className' in JSX.
- 🔗 Dynamic values can be incorporated into JSX using curly braces, which can accept strings, numbers, and variables to create dynamic attributes and styles.
- 📊 React utilizes a Virtual DOM (VDOM) to optimize rendering by minimizing direct manipulation of the actual DOM, leading to better performance and responsiveness.
- 🔄 The process of updating the real DOM based on changes detected in the VDOM is called 'reconciliation', which is efficient and prevents unnecessary re-renders.
- 🔑 Props are used to pass data into components, allowing for dynamic and customizable behavior. Components can receive any type of data, including other components.
- 🎨 Composition is a technique for structuring components to create complex UIs by nesting and combining smaller, reusable components.
- 🔐 Controlled components are those whose values are managed by React's state, ensuring predictable and consistent behavior across user interactions.
- 📋 State management in React is achieved using hooks like `useState` and `useReducer`, which allow components to have a mutable state that triggers re-renders when updated.
- 🚨 Error boundaries are special components that catch and handle errors within the React app, preventing the entire application from crashing and providing feedback to the user.
Q & A
What are the building blocks of every React app?
-The building blocks of every React app are components, which allow us to create the visible parts of our applications such as buttons, inputs, or even entire pages.
What is JSX and why is it used in React?
-JSX, which stands for JavaScript XML, is a syntax extension for JavaScript that allows us to write HTML-like structures in our JavaScript code. It is used in React because it makes it easier to create and manipulate UI components, although it is not mandatory and could be replaced with the `createElement` function.
How do attributes differ in JSX compared to HTML?
-In JSX, attributes are written in camelCase rather than using hyphens as in HTML. For example, the HTML attribute 'class' becomes 'className' in JSX. This is because JSX attributes are JavaScript objects, and JavaScript object properties use camelCase.
What is the purpose of the 'key' prop in React?
-The 'key' prop is a built-in prop in React used to identify a list of elements. It helps React to track the identity of each element and is particularly useful when using the 'map' function to create lists. A unique key for each item helps React to efficiently update and re-render components.
What is the role of 'props' in React components?
-Props, short for properties, are used to pass data from a parent component to a child component. They act as custom attributes that can be added to any component, and they can be used to control the behavior and output of the child component based on the passed data.
How does React handle rendering and updates?
-React uses the virtual DOM (VDOM) to handle rendering and updates efficiently. When the state of a React app changes, React updates the VDOM, which is a lightweight representation of the real DOM. It then uses a process called 'diffing' to compare the updated VDOM with the previous version, and applies only the necessary changes to the real DOM through a process called 'reconciliation'.
What are 'hooks' in React and what are some examples?
-Hooks are a feature in React that allows us to 'hook into' React state and other features from function components. Examples of hooks include 'useState' for managing state, 'useEffect' for connecting to external systems like browser APIs, 'useContext' for passing data through the component tree, and 'useRef' for creating references to DOM elements or other values.
What is the significance of 'purity' in React components?
-In React, 'purity' refers to the idea that components should produce the same output for the same input. Pure components only return their JSX and do not change any objects or variables that existed before rendering. This ensures that the component behaves predictably and consistently.
What is 'strict mode' in React and how does it help?
-Strict mode is a special component in React that helps us identify potential issues in our app as we develop it. By wrapping our app component in 'React.StrictMode', we can detect problems that we might not see otherwise, and it helps us avoid mistakes that could lead to errors or performance issues.
How do you use 'refs' in React to interact with the DOM?
-Refs provide a way to access DOM nodes or other React elements created in a component. We can create a ref using 'React.createRef()' and then pass it to the element we want to reference using the 'ref' attribute. Once the component mounts, we can access the referenced DOM node or React element through the 'current' property of the ref object.
What is the purpose of 'context' in React applications?
-Context in React is a way to pass data through the component tree without having to pass props down through every single level. It allows us to share data across many components without having to manually pass them through every intermediary component, making it easier to manage global or shared state.
What are 'error boundaries' in React and how do they prevent app crashes?
-Error boundaries are React components that catch JavaScript errors in their child components, log those errors, and display a fallback UI instead of letting the error crash the entire app. By adding an error boundary, we can ensure that the app remains usable even if an unexpected error occurs.
Outlines
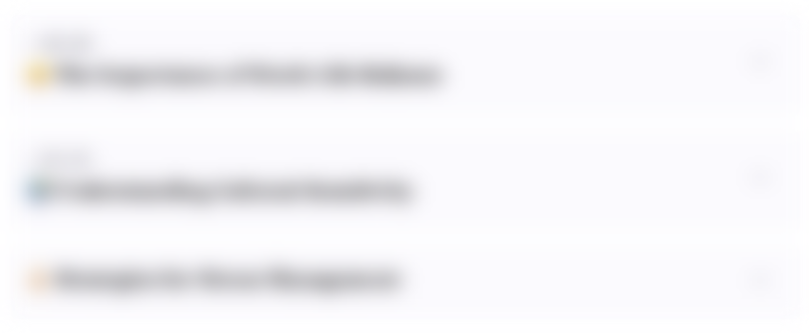
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
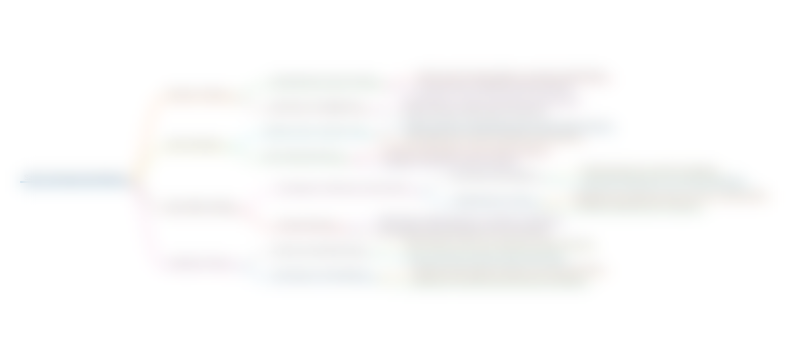
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
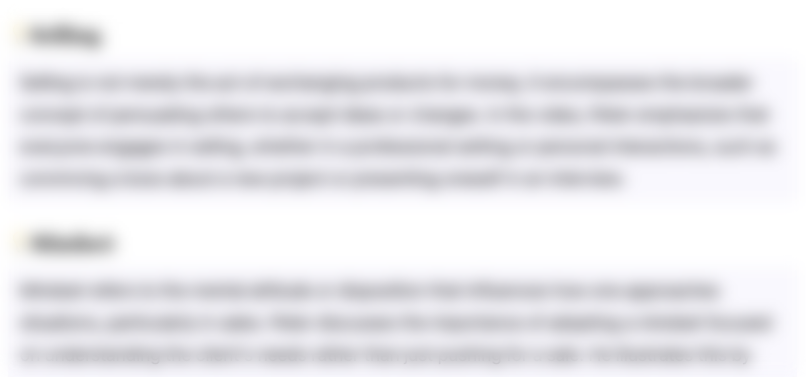
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
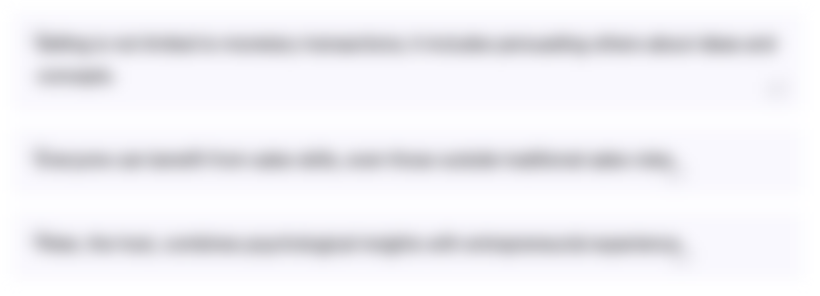
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
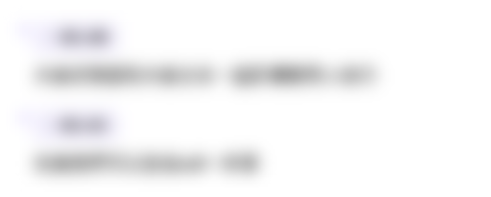
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)