What are Custom Hooks When to Create One | Lecture 166 | React.JS 🔥
Summary
TLDRCustom hooks in React are about reusability, allowing developers to share stateful or non-visual logic across multiple components. They are JavaScript functions that use one or more React hooks, often returning an object or array. Custom hooks must start with 'use' to be recognized by React, following the same rules as built-in hooks. Developers can create reusable and portable custom hooks, and many are available in libraries on NPM for use in projects.
Takeaways
- 🔄 Custom hooks in React are about reusability, allowing for the sharing of UI or logic components.
- 📦 When reusing UI, components are the standard solution, while custom hooks are for reusable logic involving React hooks.
- 🎣 If the logic to be reused doesn't involve hooks, a regular function is sufficient.
- 🔗 Custom hooks enable the sharing of stateful or non-visual logic across multiple components.
- 🚫 Custom hooks should be designed with a single purpose, focusing on reusability and portability.
- 🏠 Custom hooks are built from regular React hooks, and thus, adhere to the same rules.
- 📚 The use of custom hooks has led to a culture of sharing among developers, with many libraries available on NPM.
- 🧩 A custom hook is a JavaScript function that can accept and return data relevant to its purpose, often an object or array.
- ⚙️ Custom hooks must include one or more React hooks to function properly.
- 📝 The naming convention for custom hooks is mandatory, starting with the word 'use', e.g., 'useFetch'.
- 🛠️ Custom hooks abstract complex logic, like fetching data, into reusable, manageable pieces.
Q & A
What are custom hooks in React?
-Custom hooks in React are functions that allow for the reuse of stateful logic or any logic that involves one or more React hooks across multiple components. They are a way to encapsulate logic that can be shared among different parts of an application.
What can be reused in React besides UI components?
-Besides UI components, a piece of logic can also be reused in React through the use of custom hooks.
When is it necessary to create a custom hook?
-You should create a custom hook when the logic you want to reuse involves React hooks or when you need to share stateful logic across multiple components.
How do custom hooks differ from regular functions in React?
-Custom hooks differ from regular functions because they must use one or more React hooks and they are designed to be reusable across different components, whereas regular functions can live inside or outside components and do not necessarily involve React hooks.
What is the naming convention for custom hooks?
-Custom hooks must start with the word 'use' followed by a descriptive name, similar to the naming convention of built-in React hooks.
What is the purpose of the 'useFetch' custom hook mentioned in the script?
-The 'useFetch' custom hook is designed to abstract a simple fetch functionality, encapsulating the logic for making API requests within a reusable hook.
How can custom hooks be shared with the world?
-Developers can share their custom hooks by creating libraries and publishing them to the NPM registry, making them available for others to use in their projects.
What is the recommended approach to structuring custom hooks?
-Each custom hook should have a single purpose and be well-defined, focusing on a specific piece of logic or state management to ensure reusability and maintainability.
How do you know if the logic you want to reuse requires a custom hook?
-If the logic you want to reuse involves any React hooks, then you should create a custom hook. If it does not involve React hooks, a regular function will suffice.
What is the difference between components and custom hooks in terms of return values?
-Components in React can only receive props and must return JSX, whereas custom hooks can receive and return any data relevant to the hook, often returning objects or arrays.
Why is it important to adhere to the naming convention for custom hooks?
-Adhering to the naming convention with 'use' as a prefix is important because it signals to both developers and the React framework that the function is a custom hook, allowing for proper recognition and integration within the React ecosystem.
Outlines
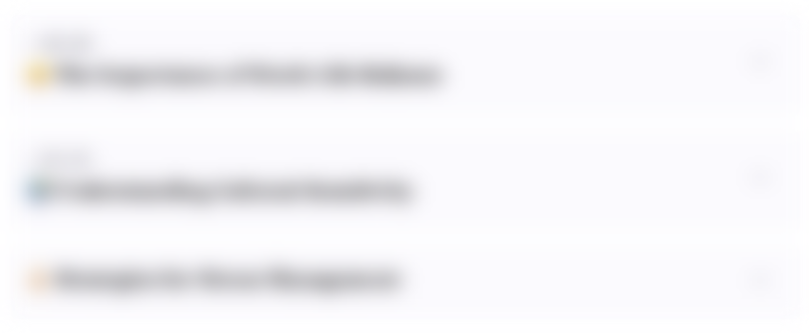
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
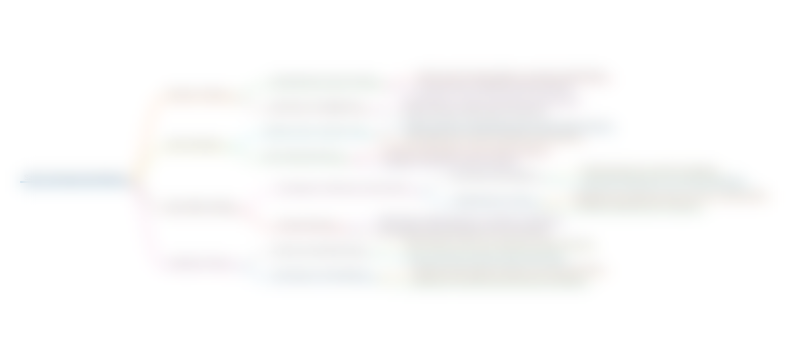
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
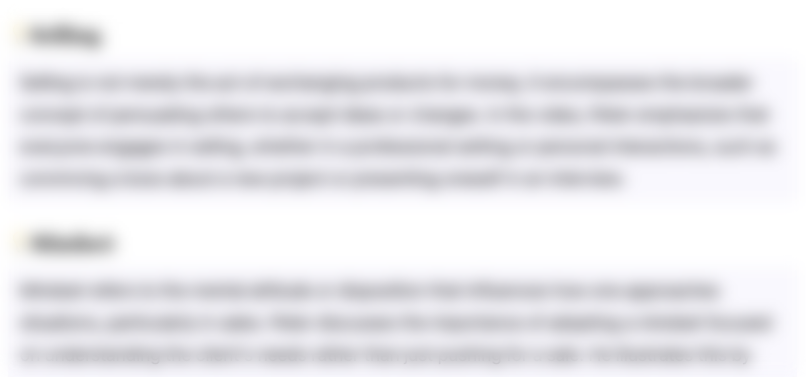
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
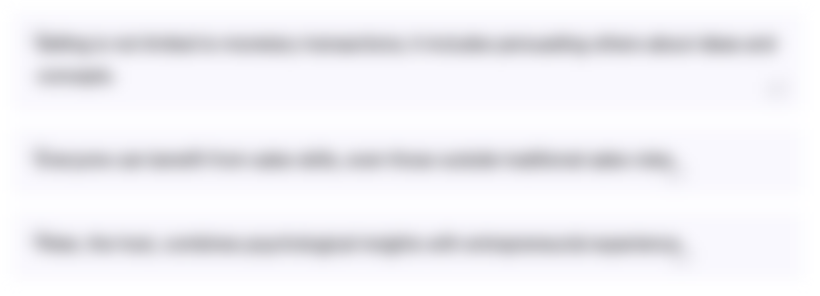
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
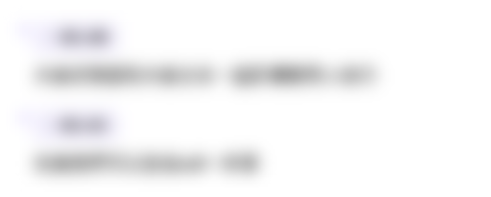
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

React Hooks and Their Rules | Lecture 157 | React.JS 🔥
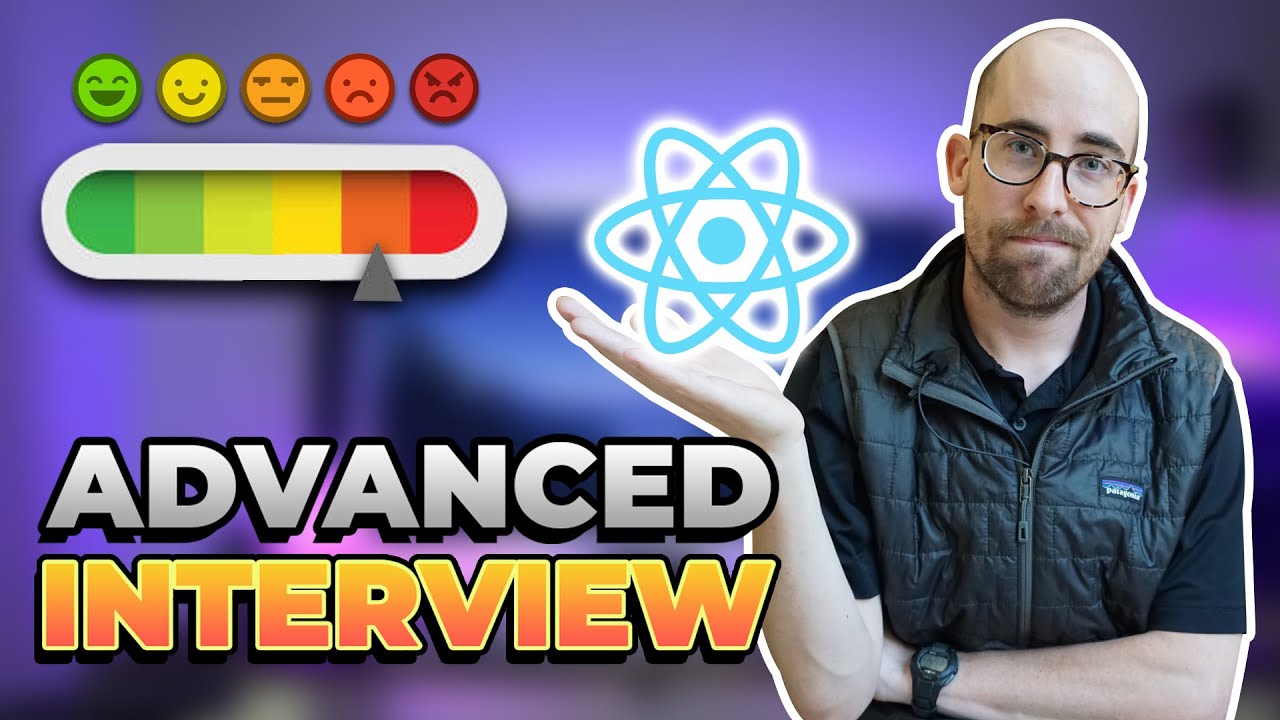
HARD React Interview Questions (3 patterns)
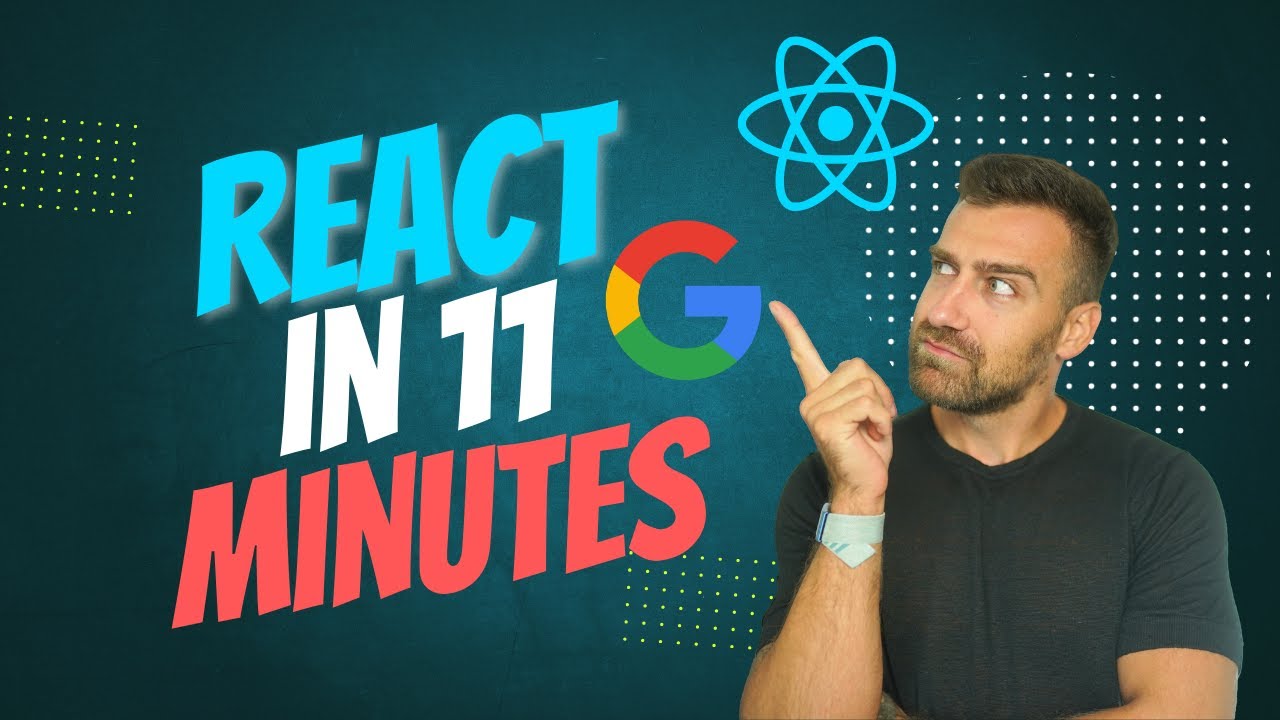
How to Learn React Fast in 2024: A Beginner's Guide
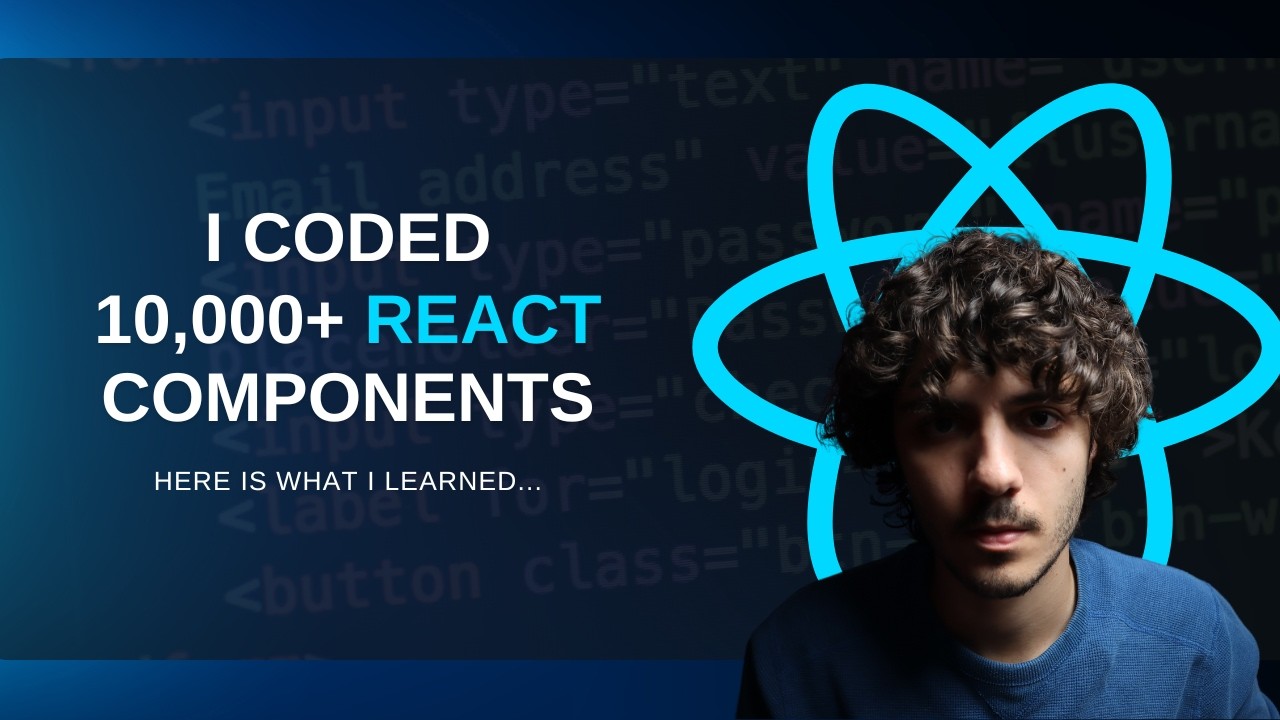
I Coded 10,000+ React Components, Here is What I Learned...
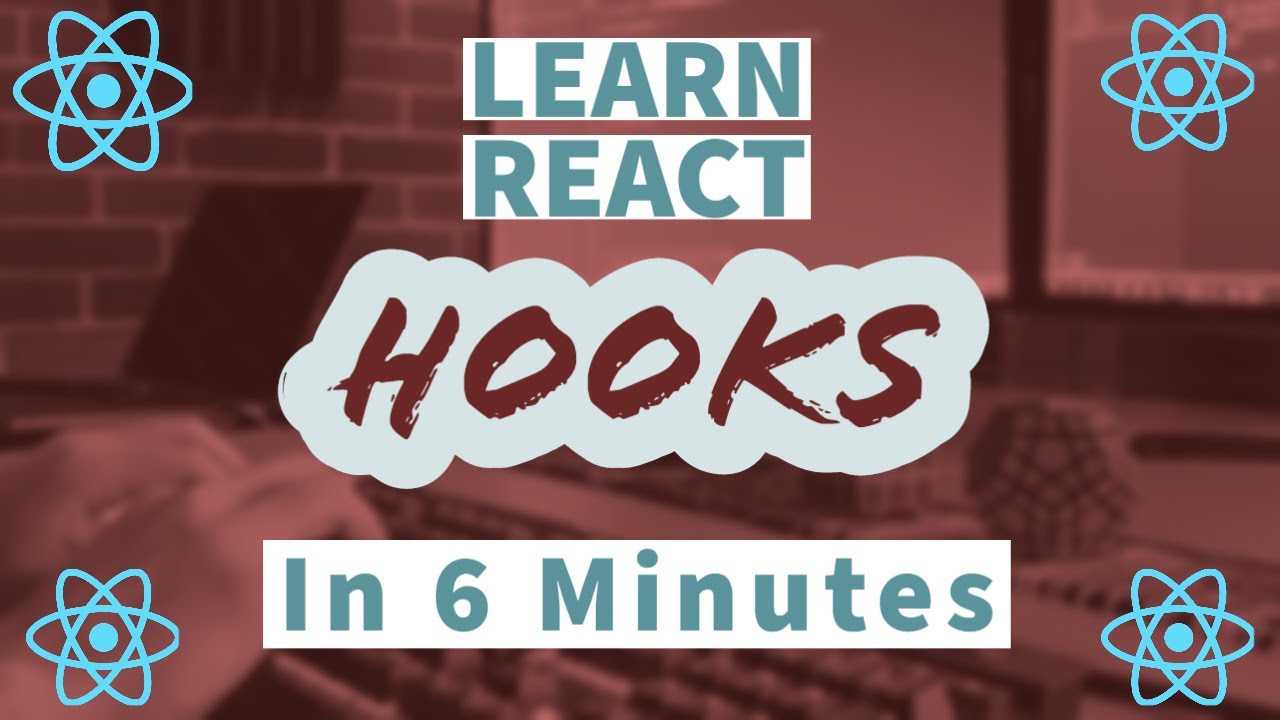
Learn React Hooks In 6 Minutes
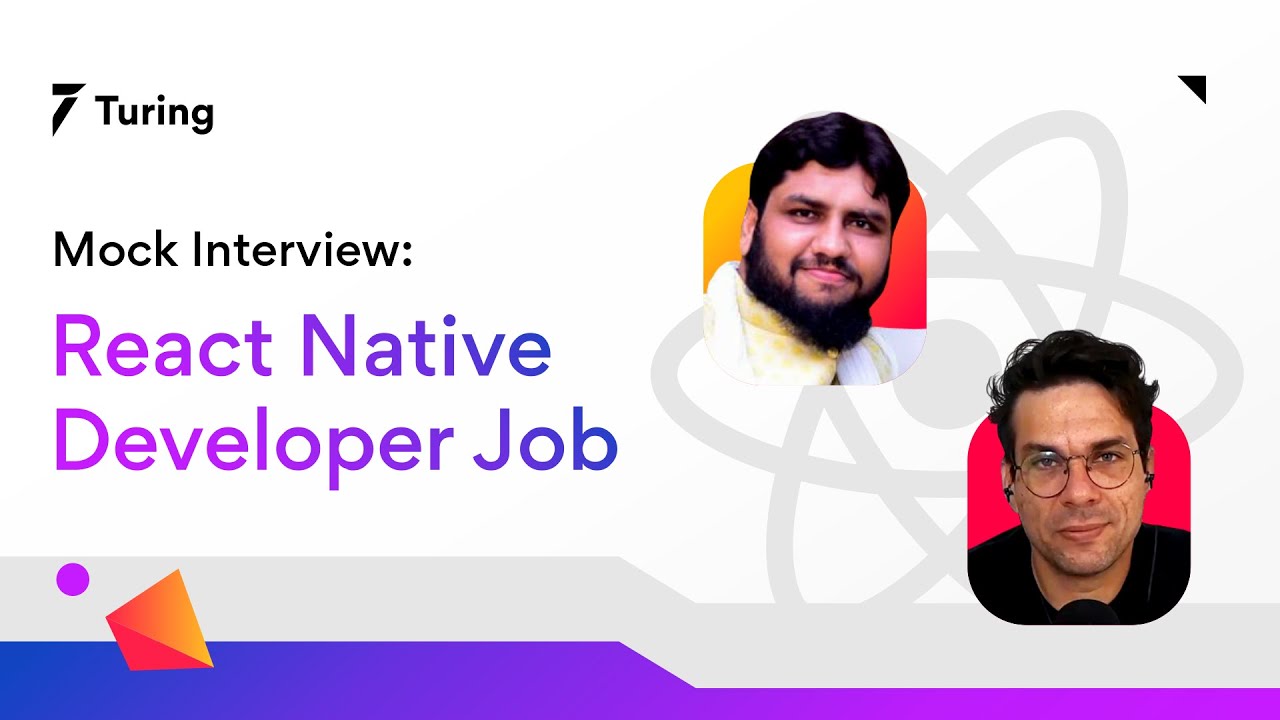
React Native Mock Interview | Interview Questions for Senior React Native Developers
5.0 / 5 (0 votes)