Constants in Java
Summary
TLDRThis lecture introduces the concept of constants in Java, emphasizing their immutability once defined with the 'final' keyword. It demonstrates how to declare and use constants, highlighting the benefits such as preventing accidental value changes and simplifying code maintenance by reducing repetitive string literals. The presenter illustrates these points with examples, including a practical demonstration in an IDE, where an attempt to reassign a constant's value results in a syntax error, reinforcing the concept of constants as fixed values throughout the program's execution.
Takeaways
- 📌 Constants in Java are variables whose values cannot be changed once set.
- 🔒 The 'final' keyword is used in Java to define constants, ensuring their value remains constant throughout the program.
- 🔑 Constants are named in uppercase with underscores separating words, following the snake case convention.
- 🚫 Attempting to change the value of a constant in Java will result in a syntax error, enforcing immutability.
- 🛡 Using constants helps prevent accidental changes to important values within a program.
- 🔄 Constants can simplify code by eliminating the need to repeatedly type the same value, reducing errors and improving maintainability.
- 🔄 If a value needs to be changed that is used multiple times, updating the constant is more efficient than changing each instance individually.
- 📚 Using descriptive names for constants can enhance code readability and understanding.
- 💡 The choice between using a variable or a constant depends on whether the value needs to be mutable or not.
- 📝 Demonstrating the use of constants in Java involves creating a new project, defining a constant with 'final', and attempting to reassign its value to showcase the error.
- 🎥 The video script includes a practical example and an error demonstration to illustrate the concept of constants in Java.
Q & A
What is a constant in Java?
-A constant in Java is a variable whose value cannot be changed once it has been assigned.
How do you define a constant in Java?
-To define a constant in Java, you use the 'final' keyword followed by the variable initialization.
What naming convention is typically used for constants in Java?
-Constants in Java are usually written in uppercase with snake case convention, where words are separated by underscores.
Why would you get a syntax error if you try to change the value of a constant in Java?
-You would get a syntax error because the 'final' keyword indicates that the variable's value is constant and cannot be reassigned.
What is an example of how to create a constant in Java?
-An example of creating a constant in Java would be: `final String companyName = "Nazar Academy";`
What are some benefits of using constants in Java?
-Benefits of using constants include preventing accidental value changes, reducing repetition of the same value, and improving code readability with descriptive names.
Why might a constant be preferred over a variable in certain situations?
-A constant is preferred when you want to ensure that a value does not change throughout the program, making the code more predictable and easier to maintain.
How does using a constant help in reducing errors in code?
-Using a constant helps in reducing errors by centralizing the value in one place, so if a change is needed, it only has to be made once, and the updated value is used everywhere in the code.
Can you provide an example of a scenario where using a constant would be beneficial?
-Using a constant is beneficial when you have a value that is used multiple times in your program, such as a company name or a configuration setting, to ensure consistency and prevent accidental changes.
What happens if you try to assign a new value to a constant after its initial assignment in Java?
-Attempting to assign a new value to a constant after its initial assignment in Java will result in a compile-time error, as constants cannot be reassigned.
How does the video demonstrate the creation and usage of a constant in Java?
-The video demonstrates the creation of a constant named 'companyName', initializes it with a string value, prints the constant, and then shows the error that occurs when trying to reassign a value to the constant.
Outlines
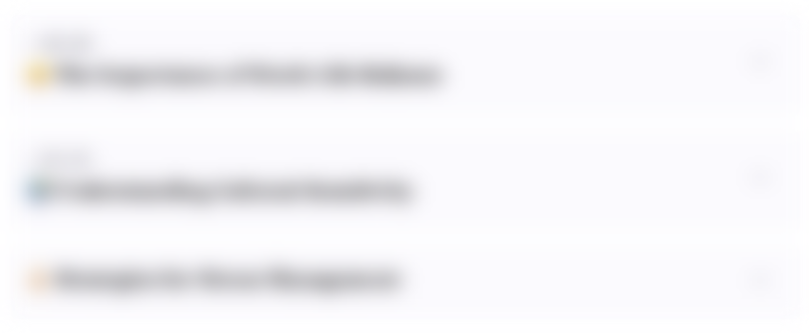
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
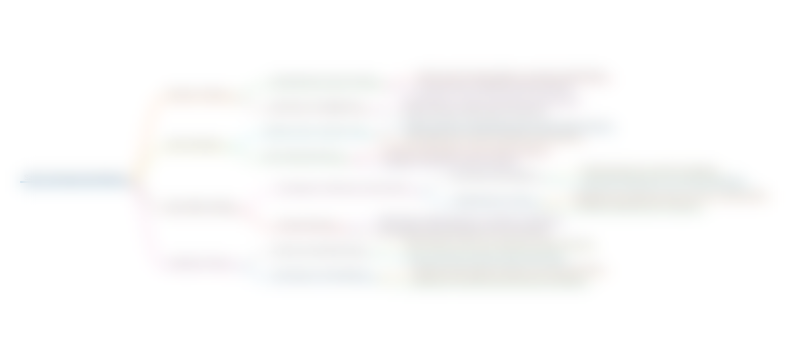
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
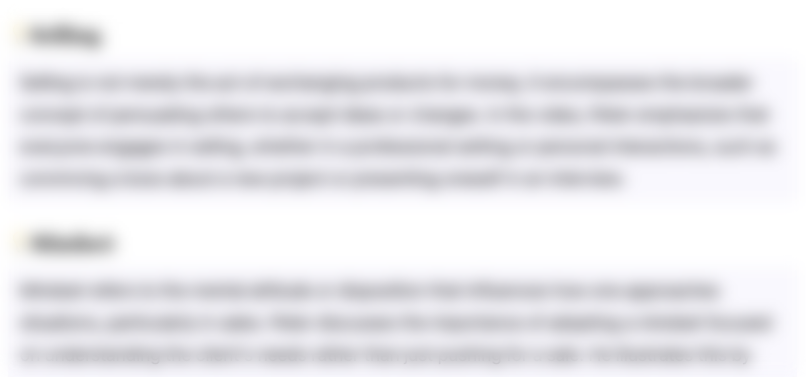
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
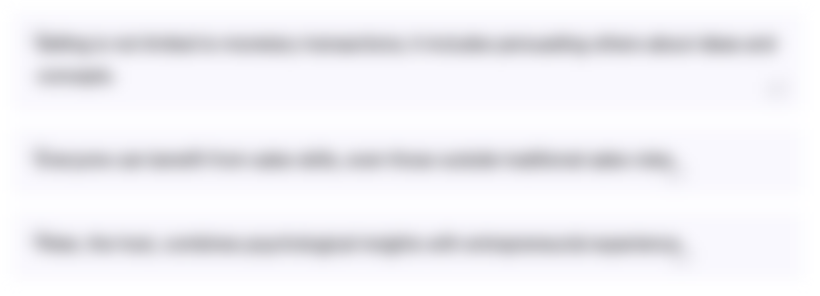
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
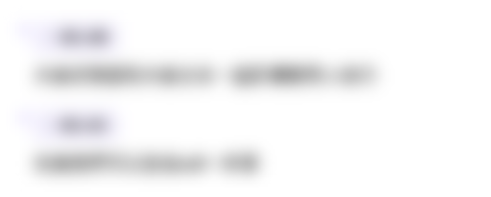
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)