Enumerations in C
Summary
TLDRThis presentation introduces enumeration in C, a user-defined type that assigns names to integral constants for easier program handling. It explains how enums are declared with the 'enum' keyword, and how they can be initialized with specific values or automatically by the compiler, starting from zero. The script also highlights the benefits of enums over '#define', such as local scope declaration and automatic initialization. Key facts about enums include the possibility of multiple names sharing the same value, assigning values in any order with automatic incrementation for unassigned names, the requirement of integral values only, and the necessity for unique enum constants within their scope. The presentation aims to clarify the utility and functionality of enums in C programming.
Takeaways
- 📚 Enumeration in C is a user-defined type used to assign names to integral constants for easier handling in programs.
- 🔍 Enums are similar to structures and unions, but their primary purpose is to simplify the use of integral constants through named constants.
- 👀 An example given in the script is the declaration of an 'enum bool' with named constants 'false' and 'true'.
- 🔑 Enums can be declared with or without explicit values; if not provided, the compiler assigns values starting from 0.
- 🎯 The automatic value assignment by the compiler means 'false' gets 0 and 'true' gets 1 by default.
- 🌟 Enums can be declared within a local scope, making them invisible outside the function they are declared in, unlike '#define' which is global.
- 👍 Enum names are automatically initialized by the compiler, providing a convenient feature for program development.
- 🔄 Multiple names in an enum can have the same value, allowing flexibility in how constants are named and used.
- 🔄 The order of value assignment in enums does not matter; unassigned names will receive the value of the previous name incremented by one.
- ❌ Only integral values are allowed for enum constants, attempting to assign a floating-point value will result in an error.
- 🚫 Enum constants must be unique within their scope; redeclaring the same name within the same scope is not permitted and will cause an error.
Q & A
What is an enumerator type in C?
-An enumerator type is a user-defined type used to assign names to integral constants, making them easier to handle in a program.
How is an enum declared in C?
-An enum is declared using the 'enum' keyword followed by the name of the enum and a list of names for the integral constants within curly braces, ending with a semicolon.
What happens if values are not assigned to enum names?
-If values are not assigned to enum names, the compiler automatically assigns values starting from 0.
What is the main purpose of using enums?
-The main purpose of using enums is to assign names to integral constants, making the code easier to read and maintain.
How can enums be declared within a local scope?
-Enums can be declared within the local scope by defining them inside a function, making them visible only within that function.
What are the two important reasons for using enums instead of hash define?
-The two important reasons are: enums can be declared in the local scope, and enum names are automatically initialized by the compiler.
Can two or more enum names have the same value?
-Yes, two or more enum names can have the same value. For example, in an enum, multiple names can be assigned the value 0.
Can enum values be assigned in any order?
-Yes, enum values can be assigned in any order. Any unassigned names will get a value as the previous name's value plus one.
Are only integral values allowed for enums?
-Yes, only integral values are allowed for enums. Assigning a non-integral value, such as a float, will result in an error.
Must all enum constants be unique within their scope?
-Yes, all enum constants must be unique within their scope. Redefining an enum name within the same scope is not allowed and will produce an error.
Outlines
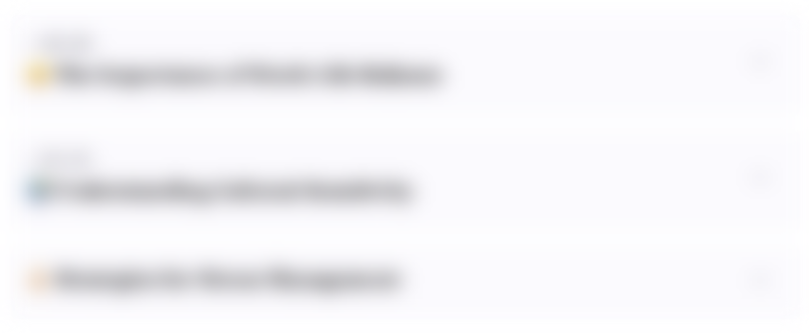
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
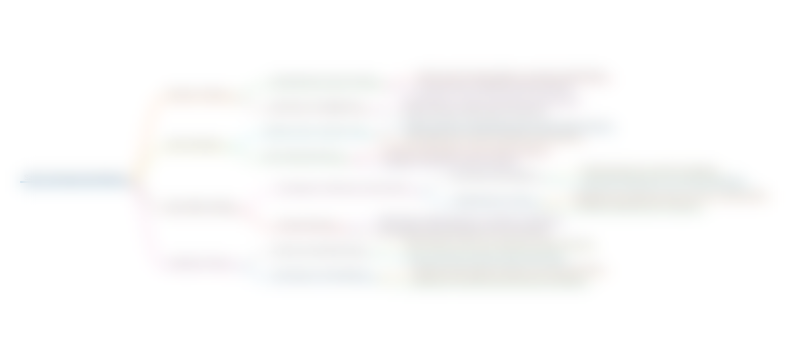
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
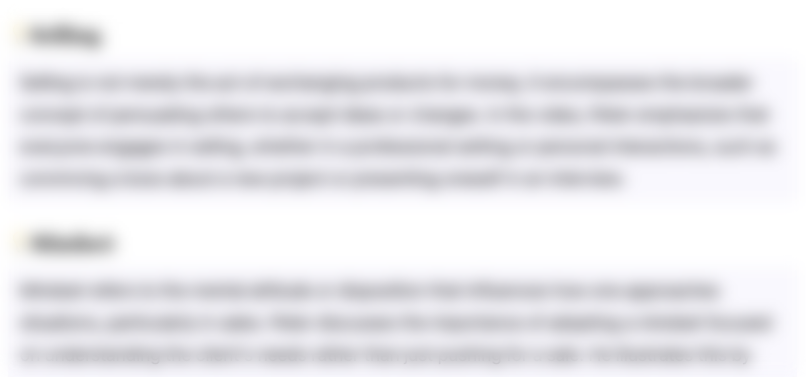
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
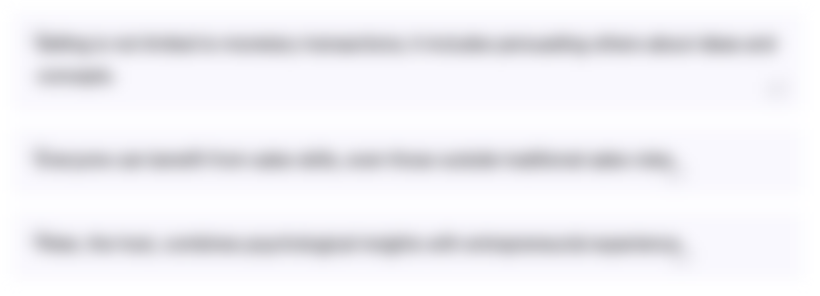
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
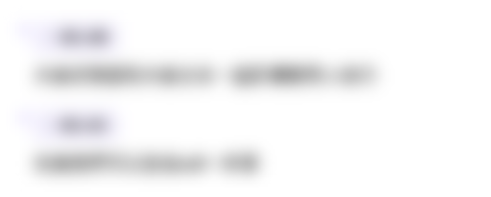
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
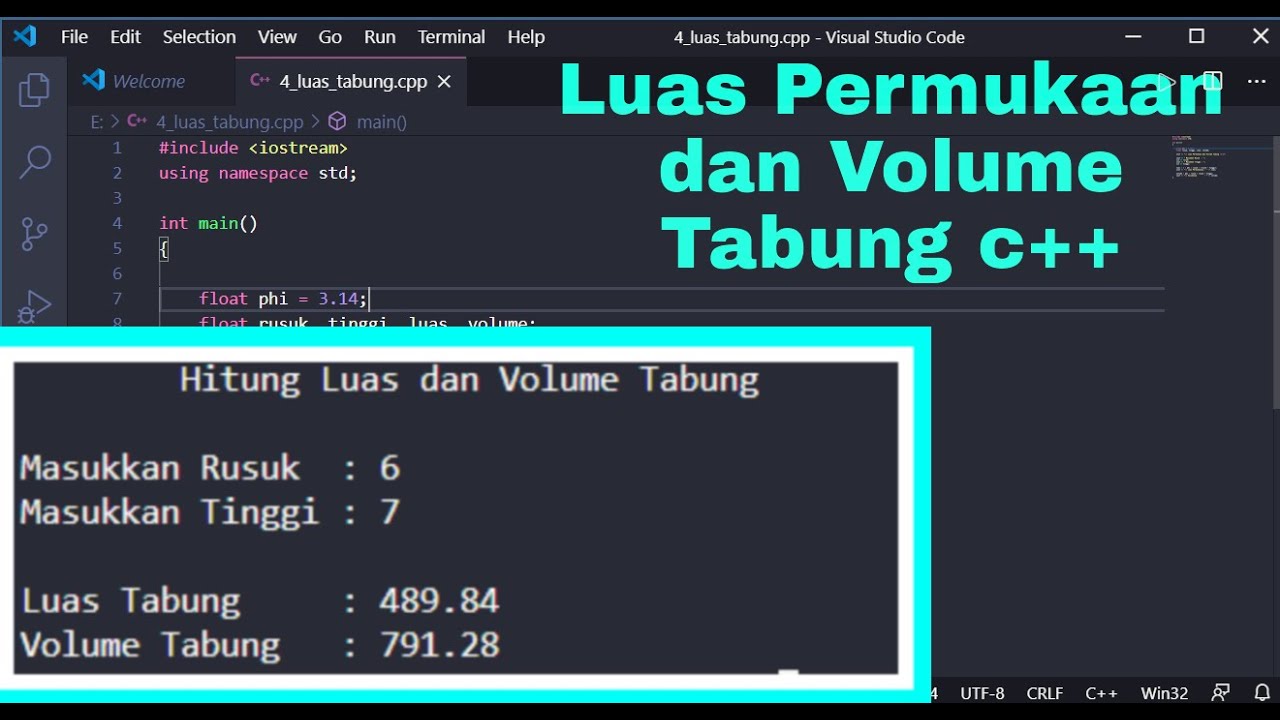
Menghitung Luas Permukaan dan Volume Tabung c++
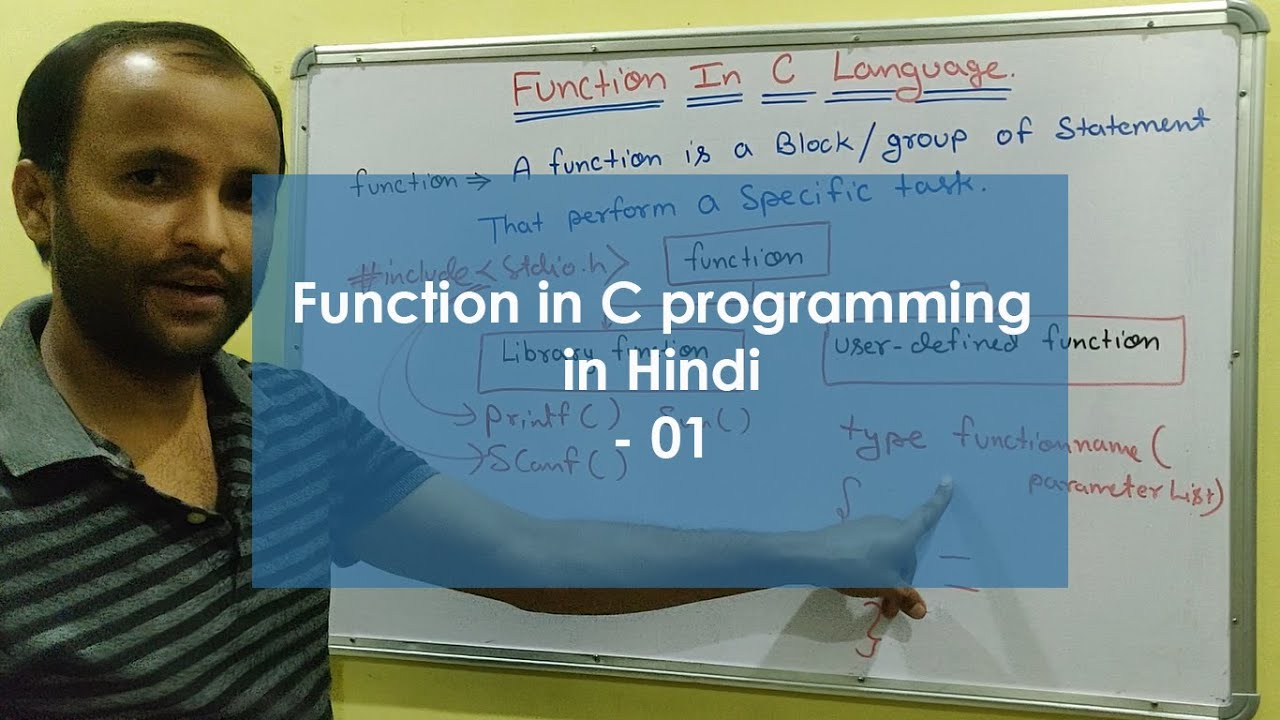
Functions in C programming in Hindi 01 | Type of function | Library & User defined function
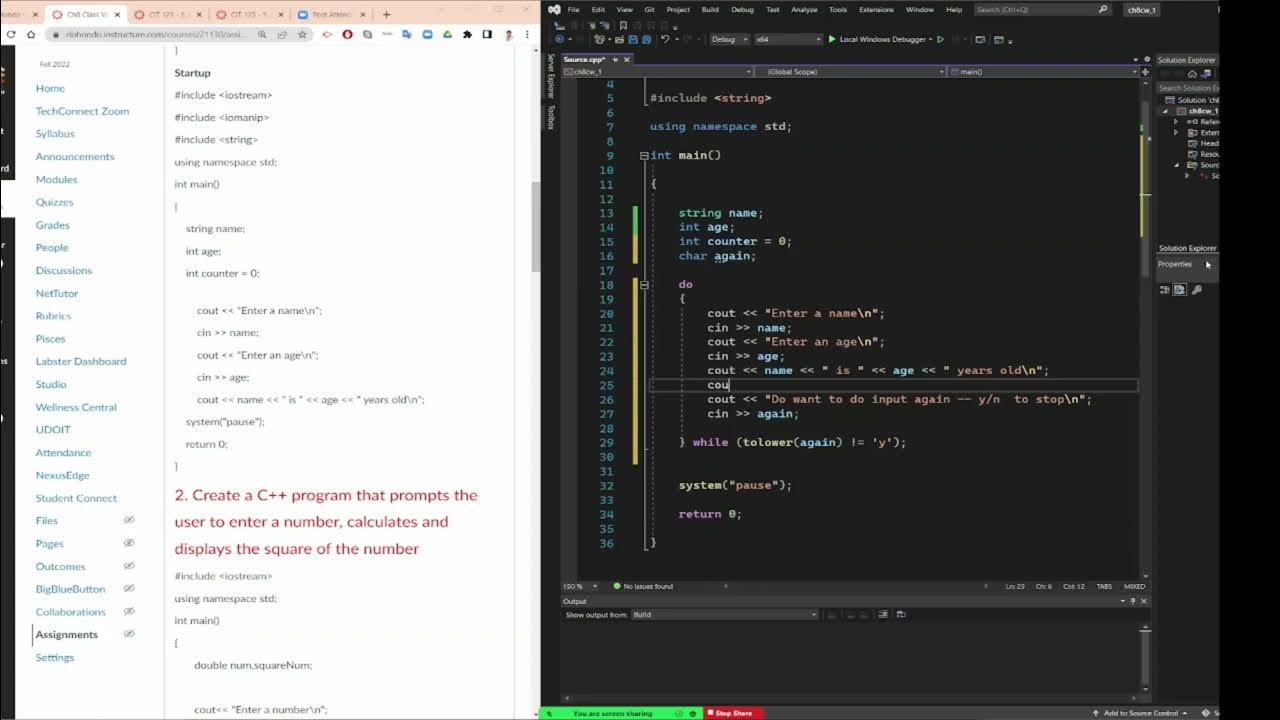
C++ programming , read a name and an age repeatedly with do while loop
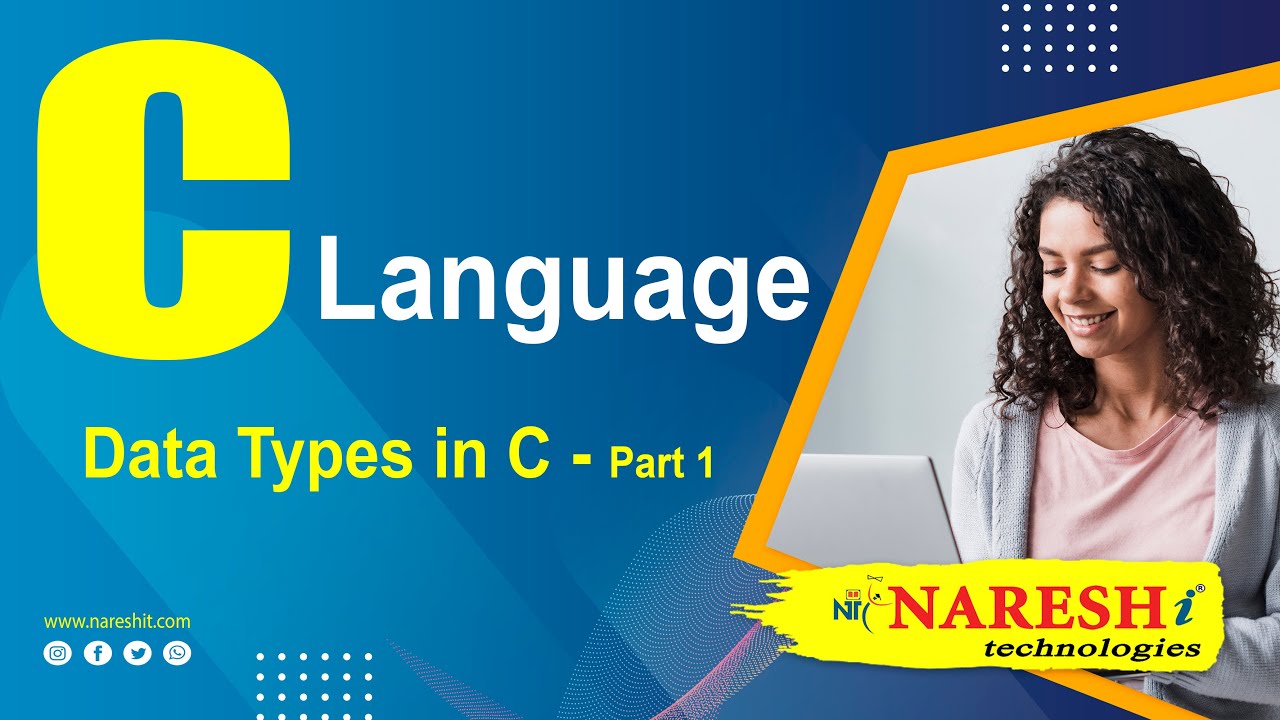
Data Types in C - Part 1 | C Language Tutorial
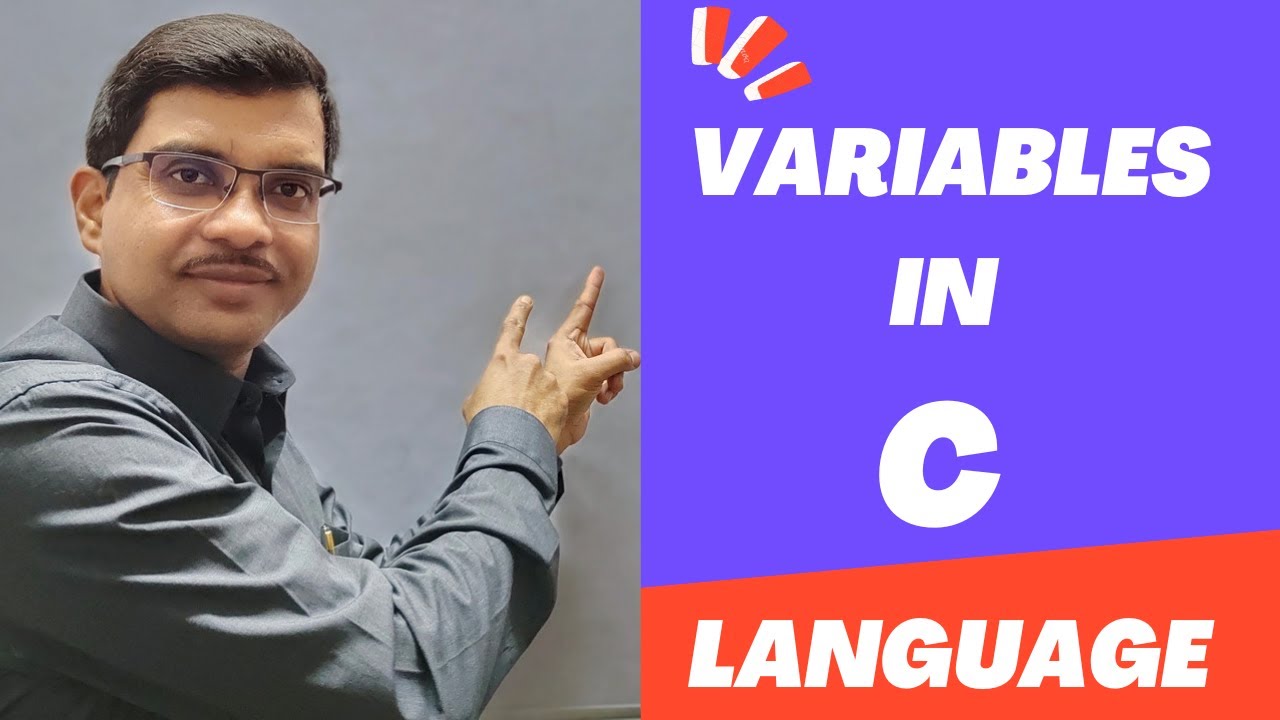
C_07 Variables in C Language | C Programming Tutorials

COS 333: Chapter 11, Part 1
5.0 / 5 (0 votes)