React Like a Pro: React Best Practices
Summary
TLDRThis video offers an in-depth exploration of best practices for React architecture, essential for both large-scale applications and frontend enthusiasts. It emphasizes the importance of single responsibility in components, discusses state management with Redux, Context API, and modern alternatives like Zustand or React Query. The video also covers side effect management with useEffect, highlighting the need for cleanup to prevent memory leaks. Lastly, it touches on project structuring, suggesting that while there are best practices, the key is a clear and workable structure for the team.
Takeaways
- đ The video is a deep dive into best practices for React architectures, aimed at both beginners and experienced developers looking to build sustainable, efficient, and scalable applications.
- đ The presenter emphasizes the importance of subscribing and liking the content for support and mentions that it helps more than viewers might know.
- đ The video features a sponsorship by Brilliant, a platform that offers interactive lessons on various topics including math, data analysis, and programming, promoting a hands-on learning approach.
- đ The presenter shares a personal experience with Brilliant, highlighting the value of engaging with content through puzzles and problems to better understand the material.
- đ§ The video discusses the concept of learning AI, including large language models, and how the presenter used Brilliant to sharpen their skills in this area.
- đ Viewers are offered a 30-day free trial and a 20% discount on the annual premium subscription to Brilliant through a link provided in the video description.
- đïž The foundation of React is built on components, which should follow the single responsibility principle to ensure maintainable and clean code.
- đ Components are categorized into 'dumb' (presentational) and 'smart' (container) components, with the former focusing on appearance and the latter on functionality.
- đ§© The video touches on state management in complex React applications, suggesting the use of Redux, the Context API, or modern solutions like Zustand or React Query for better structure and predictability.
- đ« Side effect management is crucial in React; the presenter advises on proper use of the useEffect hook to avoid memory leaks and performance issues.
- đ ïž The video concludes with a discussion on project structure, suggesting that while there are best practices, the most important aspect is that the structure is understandable to the development team.
- đ Three different folder structures are presented as examples for structuring a React project, catering to different levels of complexity and use cases.
Q & A
What is the main focus of the video?
-The video focuses on best practices for building scalable and efficient React applications, discussing topics like component architecture, state management, side effect handling, and project structure.
Why is the single responsibility principle important in React component design?
-The single responsibility principle is crucial because it helps in writing maintainable and clean code. Components with one responsibility are easier to test and manage, improving the overall architecture of the React application.
What are the two major types of React components mentioned in the video?
-The two major types of React components mentioned are 'dumb' (presentational) components and 'smart' (container) components. Dumb components focus on the presentation and rely on props, while smart components handle data fetching, state management, and complex operations.
How does the video suggest learning and mastering new skills like AI?
-The video suggests using interactive platforms like Brilliant, which offer hands-on learning through puzzles and problems, to understand and apply new knowledge effectively.
What is the context of the sponsorship mentioned in the video?
-The sponsorship is for the educational platform Brilliant, which the video creator has used and recommends for learning various skills, including AI, through interactive lessons.
What are some challenges in state management for complex React applications?
-Challenges in state management include potential chaos and disorganization if states are not structured properly, which can lead to difficulties in maintaining and scaling the application.
Why is it recommended to use a state management library or the context API in React applications?
-Using state management libraries like Redux or the context API helps enforce a pattern for state management, preventing chaos and making the application more maintainable and scalable.
What is the role of React Query in the context of state management mentioned in the video?
-React Query is a data fetching library that can be used alongside the context API for managing state. It allows for efficient data handling and can reduce the need for additional state management libraries in some cases.
What are some common issues with side effect management in React?
-Common issues include memory leaks and performance problems due to improper cleanup of side effects, such as not clearing timeouts when a component unmounts.
How can you avoid memory leaks when using the useEffect hook in React?
-To avoid memory leaks, ensure that you return a cleanup function from the useEffect hook to clear any asynchronous operations or event listeners when the component unmounts.
What are some factors to consider when structuring a React project?
-Factors to consider include maintainability, scalability, team understanding, and the specific needs of the application. While there are best practices, the most important aspect is that the structure works well for the development team.
What are the three different folder structures presented in the video for a React application?
-The video presents three folder structures: one using Redux, one for a simple application, and an advanced structure with multiple components. These structures are meant to serve as examples and can be adapted based on the project's requirements.
Outlines
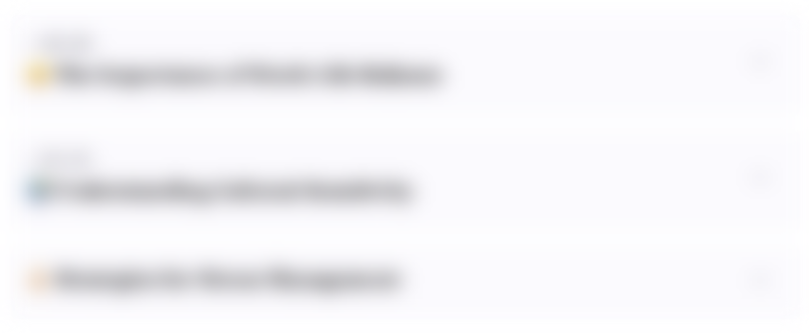
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
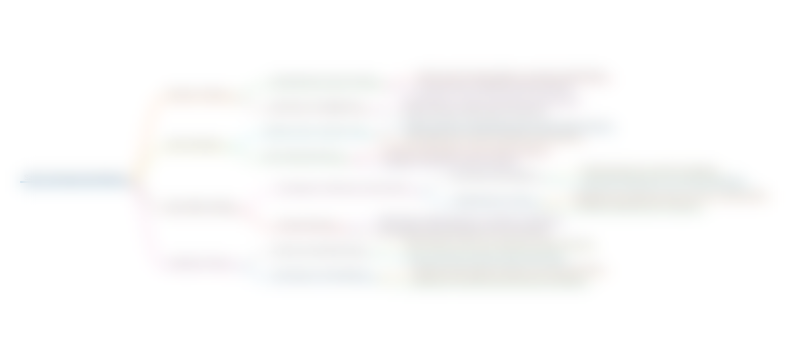
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
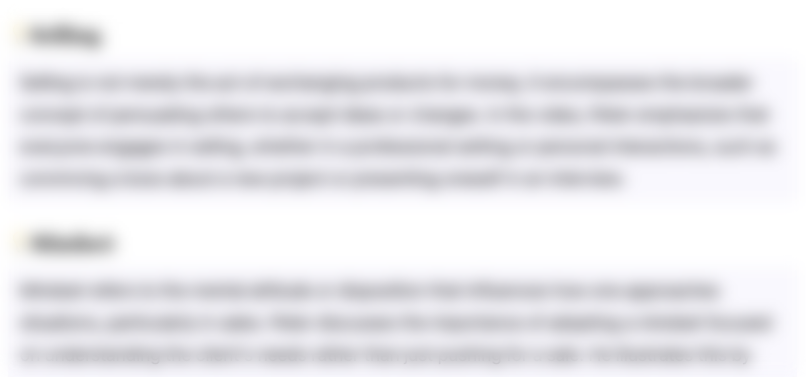
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
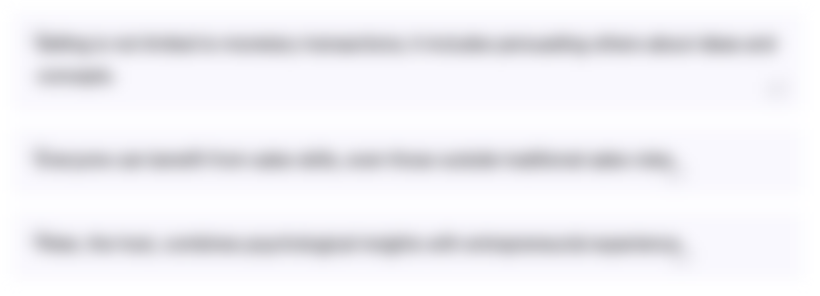
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
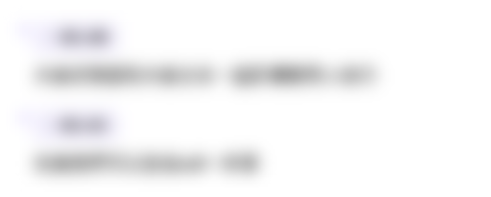
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
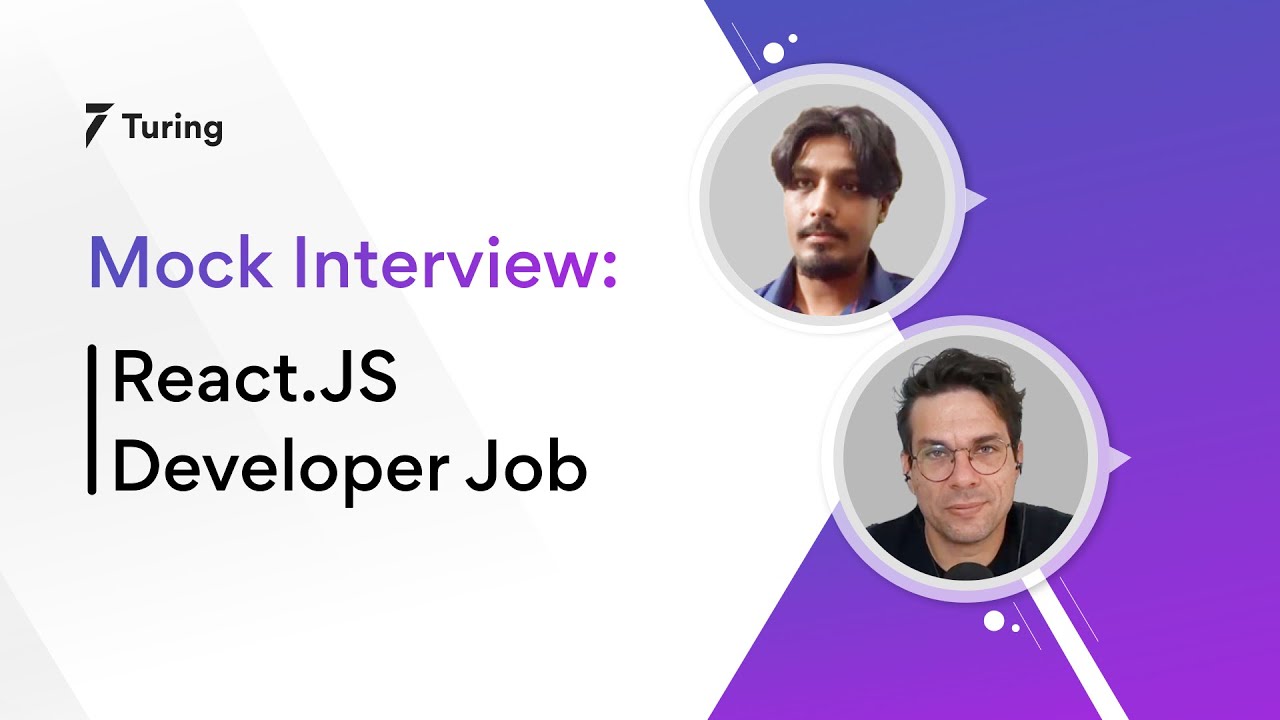
React.JS Mock Interview | Interview Questions for Senior React.JS Developers
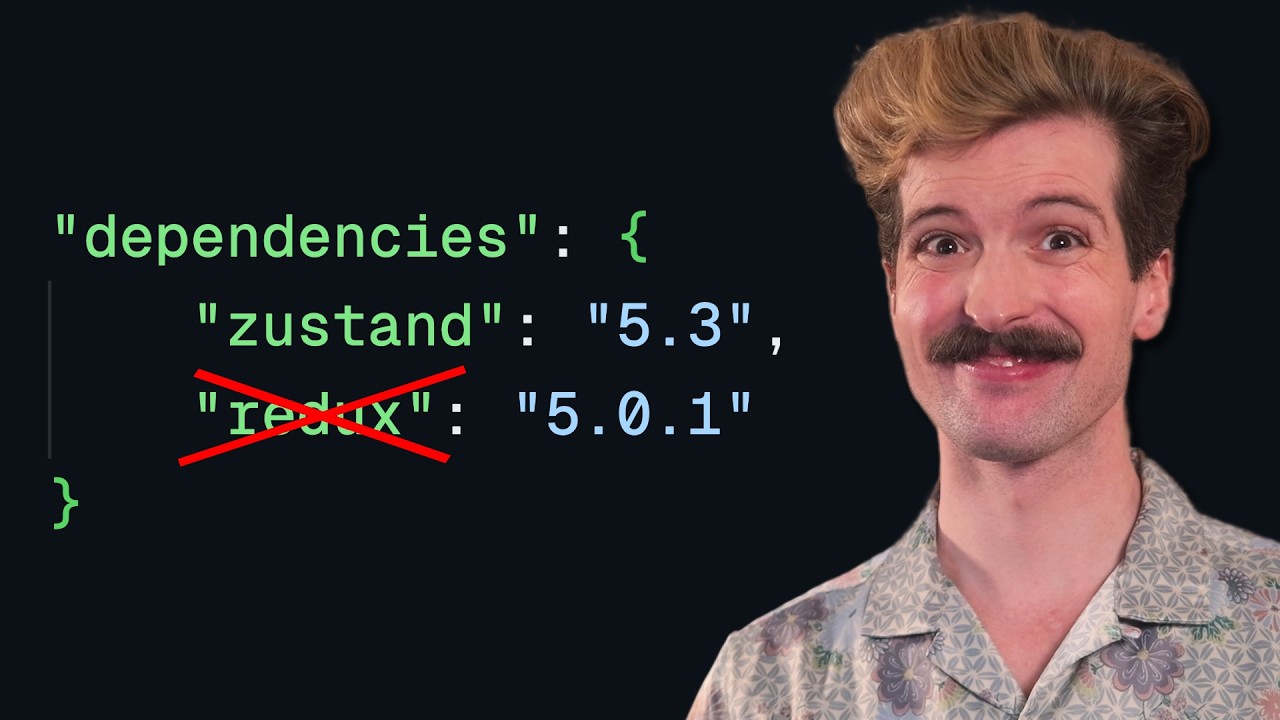
Why Everyone Loves Zustand
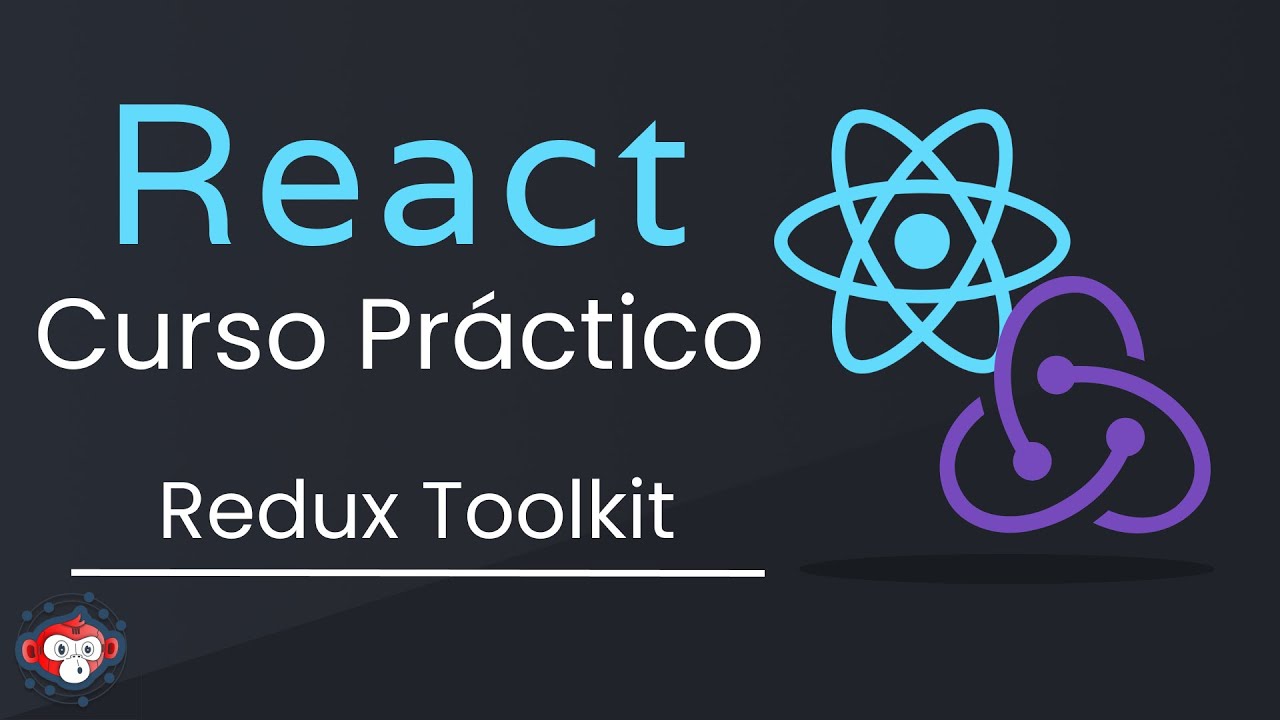
React & Redux Toolkit - Bases y proyecto prĂĄctico
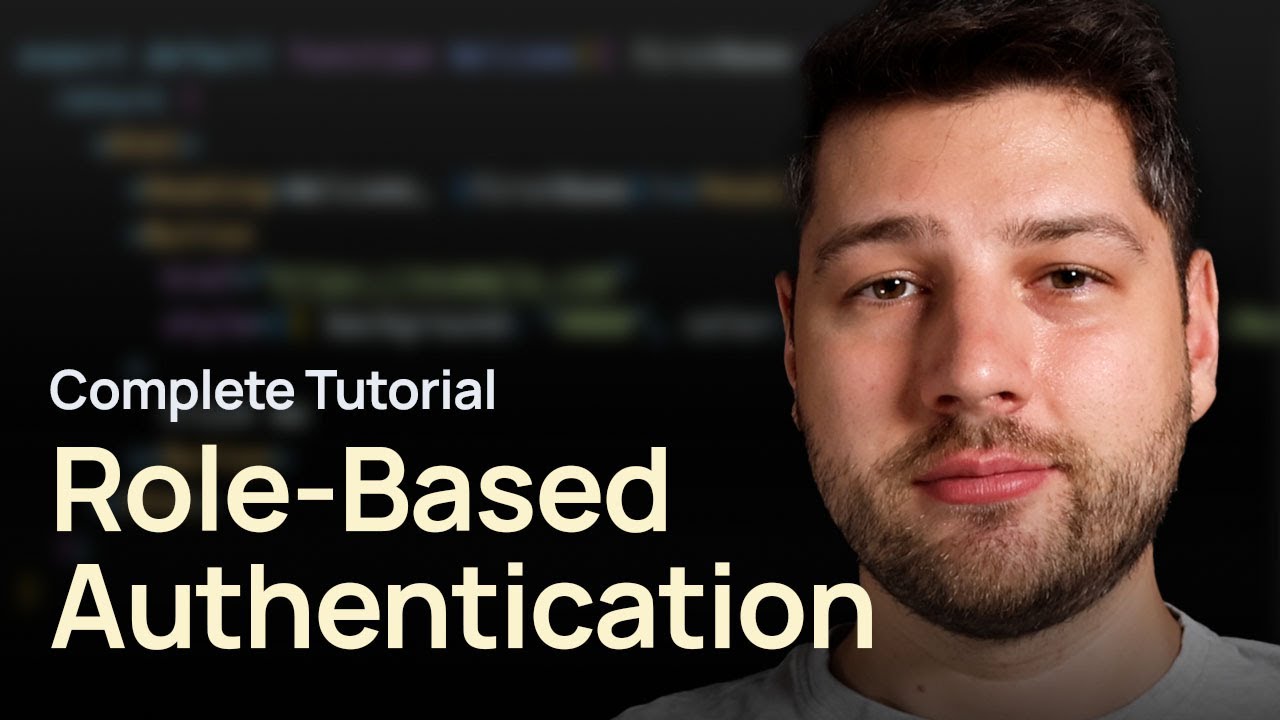
Role-Based Authentication in React (Complete Tutorial)
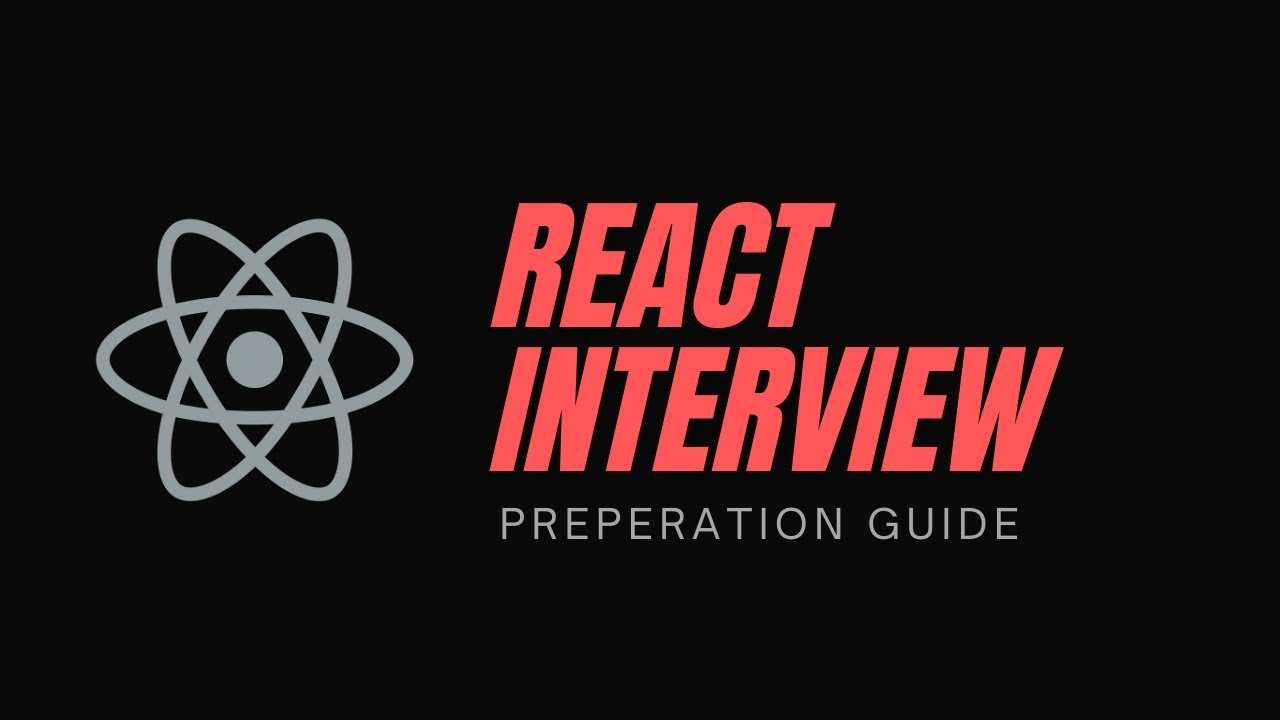
React Interview Questions | A Preparation Guide
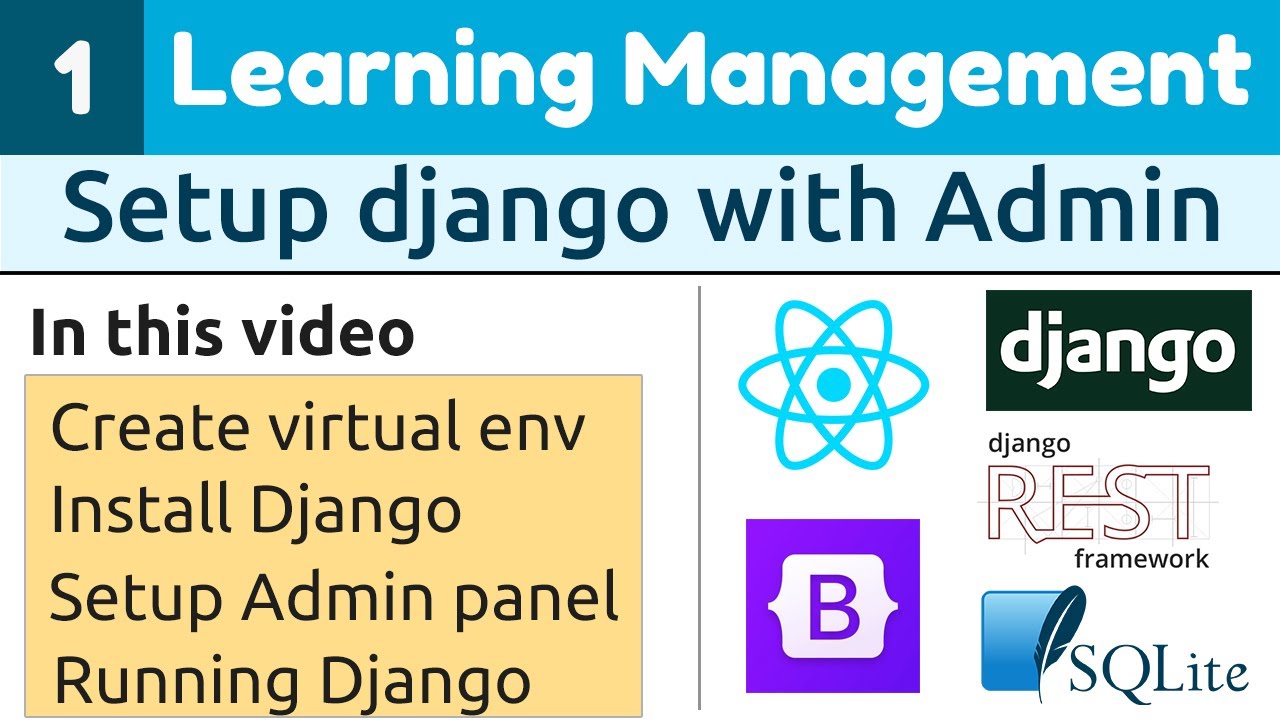
Learning Management System in Django ReactJs #1|Setup django with admin panel
5.0 / 5 (0 votes)