C_77 Pointers in C- part 7 | Pointer Arithmetic (Increment/Decrement) program
Summary
TLDRIn this video on C programming, we explore pointers, focusing on how to perform increment and decrement operations. We cover the differences between post-increment (p++) and pre-increment (++p), as well as post-decrement (p--) and pre-decrement (--p) with pointers. Using practical examples and visual representations, the video clarifies these operations' functioning, including how they interact with array pointers. Viewers are encouraged to practice these concepts to understand the subtle nuances and avoid common pitfalls. The video concludes with a practical demonstration and invites questions for further clarification.
Takeaways
- đą Pointers in C can be incremented and decremented using ++ and -- operators.
- đ There are two types of increment/decrement: pre (e.g., ++p) and post (e.g., p++).
- đ Pre-increment (++p) increases the pointer's value before returning it.
- đ Post-increment (p++) returns the pointer's value before incrementing it.
- đ The name of an array acts as a constant pointer to its first element.
- đ« The array name (e.g., a) cannot be modified or incremented.
- đ The size of the data type determines the increment step (e.g., int size is 4 bytes).
- đ Using p++ or p = p + 1 both effectively move the pointer to the next element.
- đĄ Post-decrement (p--) and pre-decrement (--p) function similarly but decrease the pointer's value.
- đ§ Understanding operator precedence and associativity is crucial for evaluating expressions involving pointers.
Q & A
What are post-increment and post-decrement operators in C?
-Post-increment (p++) means the current value of the pointer is used in the expression first, and then the pointer is incremented. Post-decrement (p--) means the current value of the pointer is used in the expression first, and then the pointer is decremented.
What are pre-increment and pre-decrement operators in C?
-Pre-increment (++p) means the pointer is incremented first, and then the new value is used in the expression. Pre-decrement (--p) means the pointer is decremented first, and then the new value is used in the expression.
How do you increment a pointer in C?
-To increment a pointer in C, you can use either p++ or p = p + 1. Both expressions will move the pointer to the next memory location based on the data type it points to.
Why is 'a = p' not a valid assignment in C?
-'a' is the name of an array and acts as a constant pointer to the base address of the array. Since it is a constant pointer, you cannot assign a new address to it.
What happens when you perform p++ on a pointer?
-When you perform p++ on a pointer, the pointer is moved to the next memory location based on the size of the data type it points to. For example, if p is a pointer to an int and the current address is 1000, p++ will move the pointer to 1004, assuming int is 4 bytes.
How does the expression *p++ differ from *++p?
-*p++ returns the value at the current pointer address and then increments the pointer, while *++p increments the pointer first and then returns the value at the new address.
Can you assign a new address to an array name in C?
-No, you cannot assign a new address to an array name in C because it is a constant pointer that always points to the base address of the array.
What does the expression *p-- do?
-*p-- returns the value at the current pointer address and then decrements the pointer to the previous memory location.
Why is it important to understand the difference between pre-increment and post-increment?
-Understanding the difference between pre-increment and post-increment is important because it affects the value used in expressions and can lead to different results in your program. Pre-increment modifies the value before using it, while post-increment uses the current value before modifying it.
How does pointer arithmetic differ for different data types?
-Pointer arithmetic takes into account the size of the data type the pointer is pointing to. For example, if an int is 4 bytes, incrementing an int pointer moves it by 4 bytes. For a char pointer, which is 1 byte, incrementing moves it by 1 byte.
Outlines
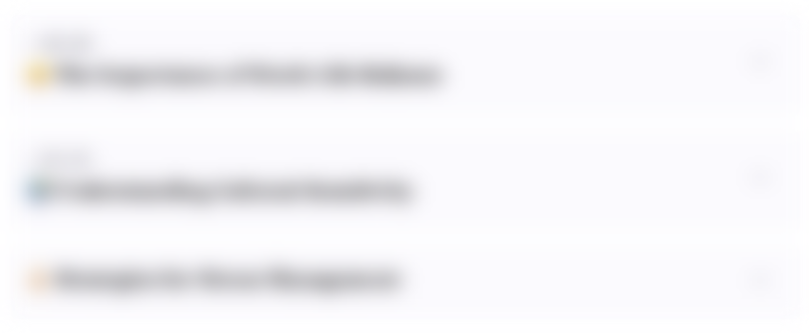
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
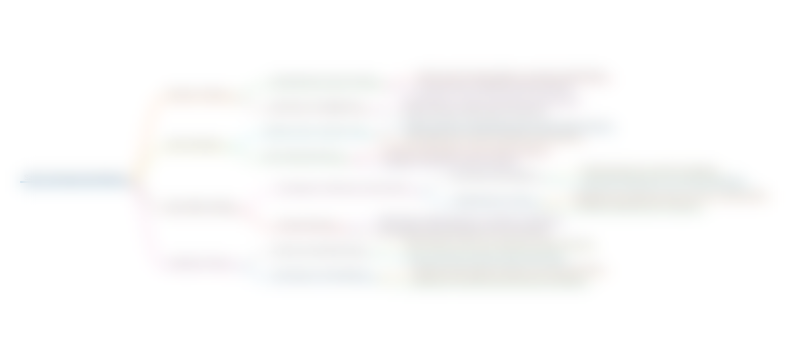
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
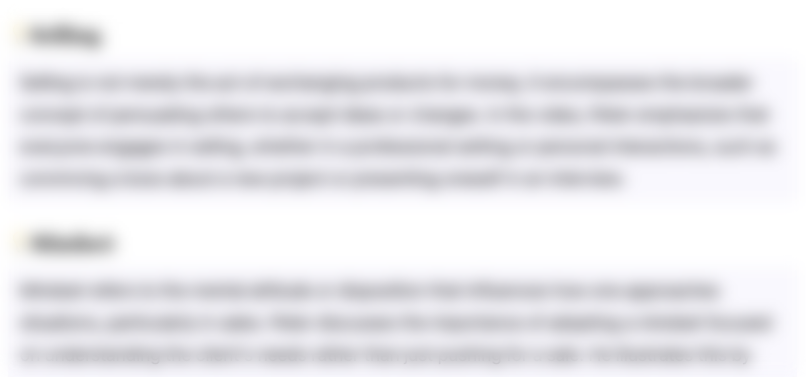
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
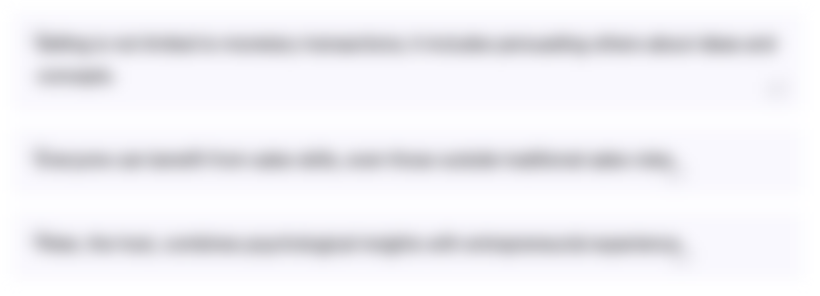
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
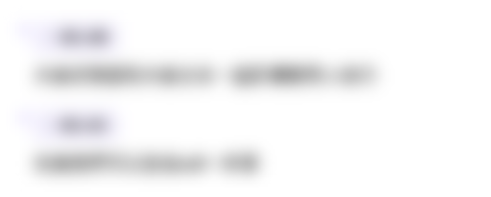
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

C_75 Pointers in C-part 5 | Pointer Arithmetic (Addition) with program
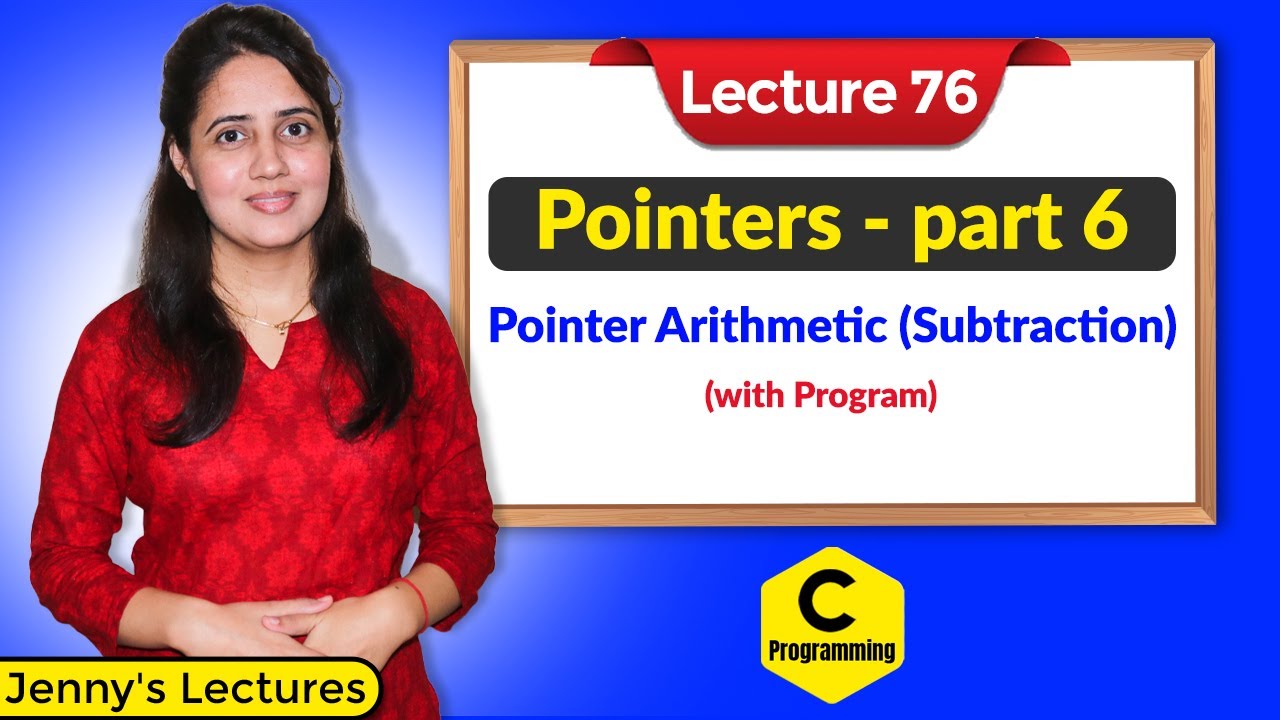
C_76 Pointers in C- part 6 | Pointer Arithmetic (Subtraction) with program
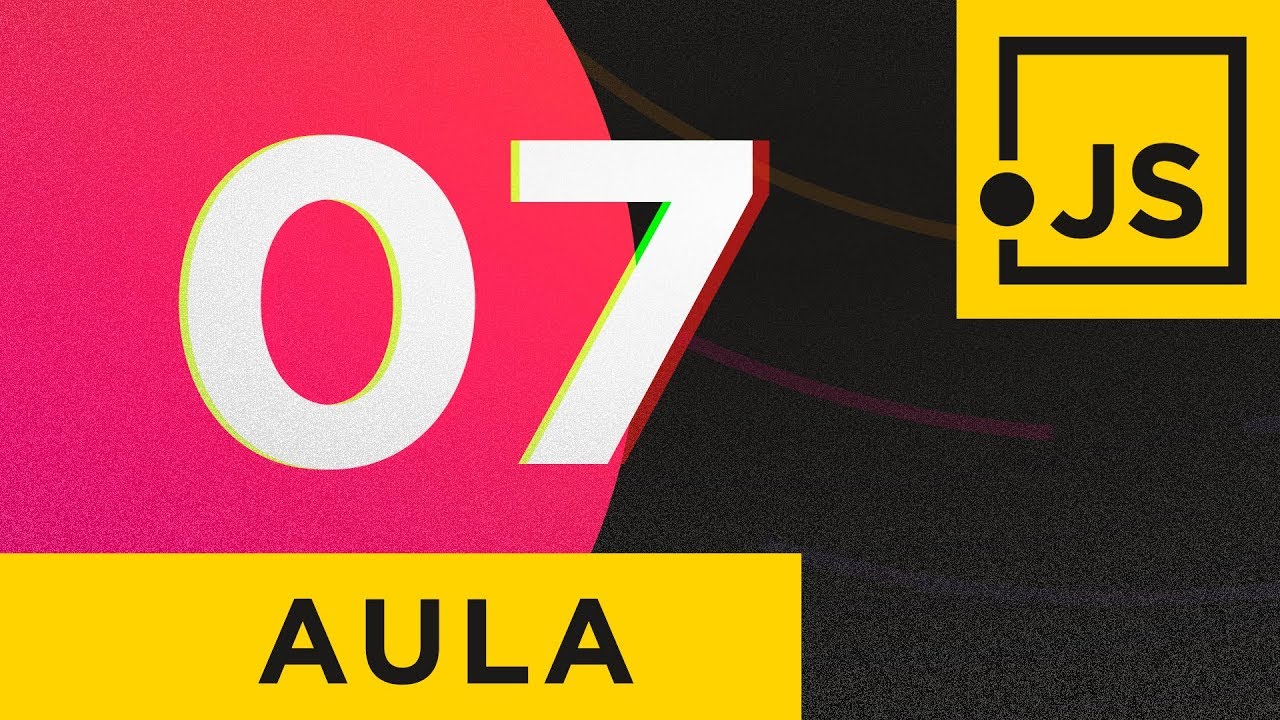
Operators (Part1) - JavaScript Course #07
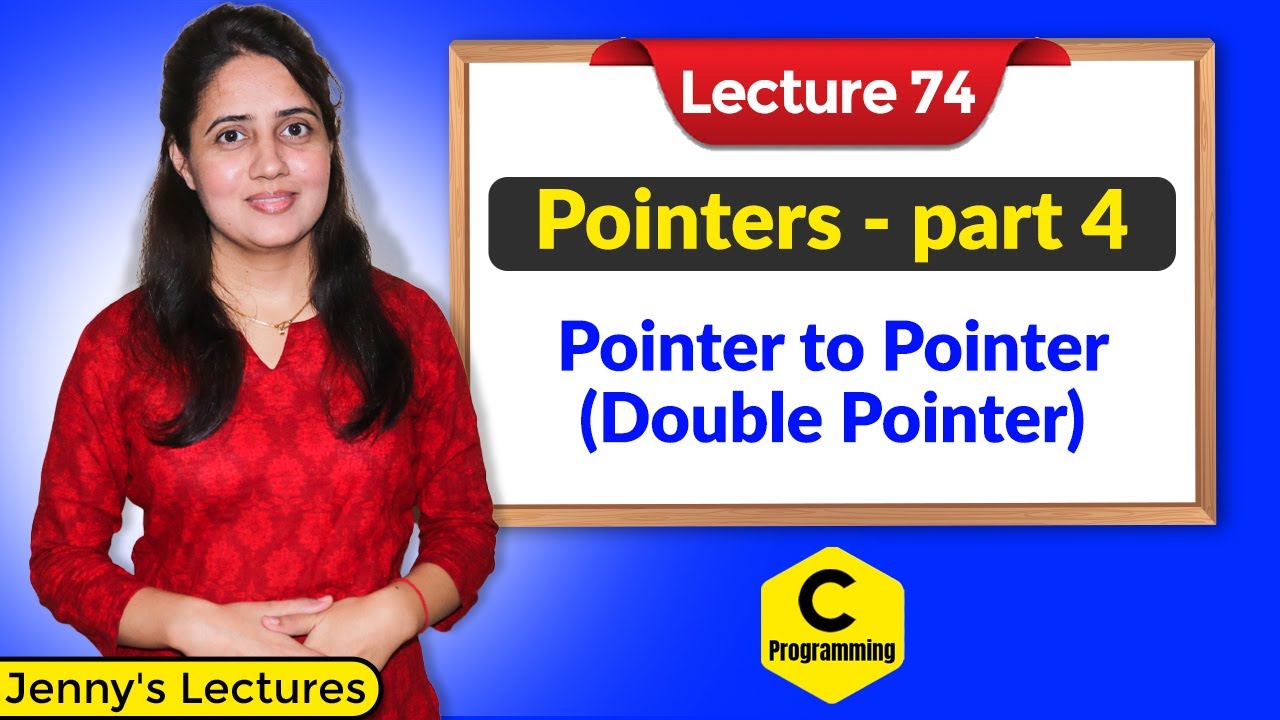
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
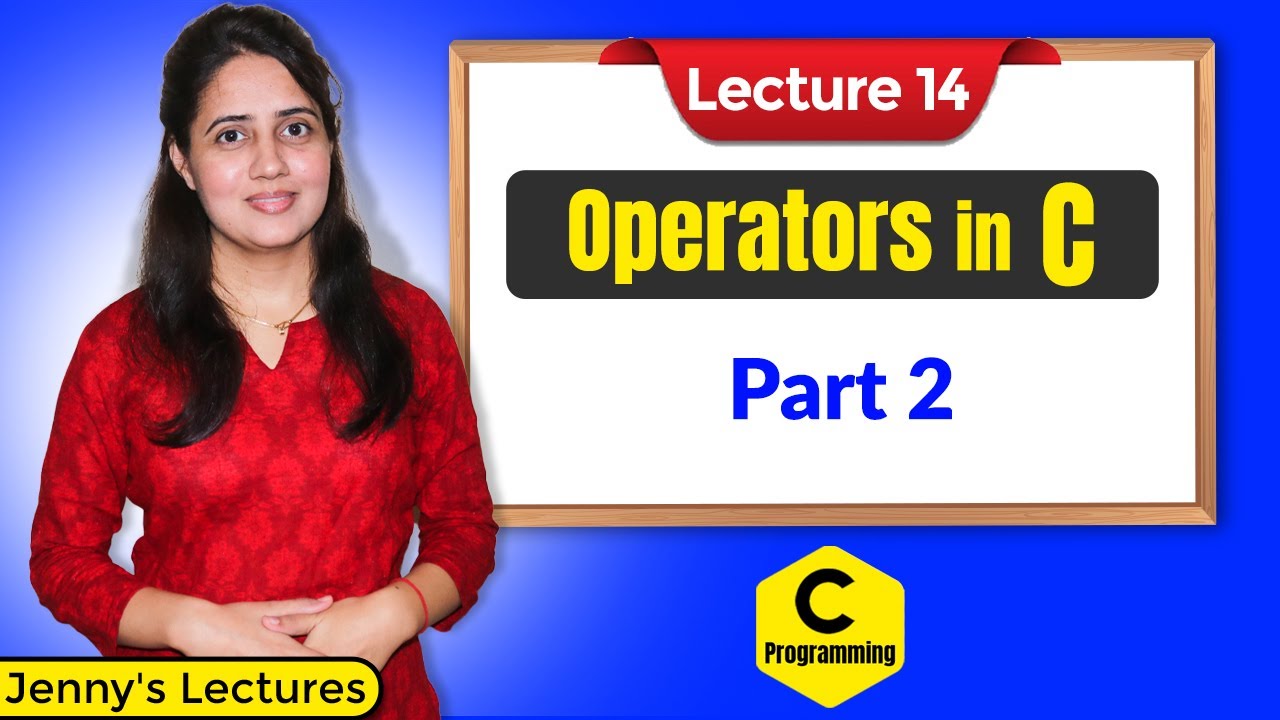
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials

C_15 Operators in C - Part 3 | C Programming Tutorials
5.0 / 5 (0 votes)