Designing scalable Compose APIs
Summary
TLDRSimona Milanovic's session on designing scalable Compose APIs emphasizes the importance of creating high-quality, use-case-based APIs in the Jetpack Compose framework. She discusses best practices for planning, structuring, and naming components like FilterChip, leveraging Kotlin conventions, and maintaining API consistency. The talk also covers accessibility, testing, and documentation to ensure long-term API stability and usability, guiding developers towards better design practices.
Takeaways
- đ The core of Jetpack Compose is the composable function, which is essential for building UI and establishing a scalable API.
- đ When creating Compose APIs, it's important to have guidelines that ensure code scalability, consistency, and intuitiveness, as well as to guide best practices.
- đĄ Start by identifying a single problem to solve with a new API, adhering to the principle of 'one component, one problem' for clarity and ease of use.
- đ For variations of a component with similar UI but different purposes, consider building layered, higher-level APIs that are more constrained and offer fewer customization options.
- đ Naming conventions in Compose are crucial; use PascalCase for composable functions returning Unit, and standard Kotlin conventions for functions returning values.
- đ« Avoid implicit or obscure inputs in APIs; instead, use explicit parameters to enhance readability, adjustability, and ease of use.
- đš Modifiers are key in Compose for defining the appearance and behavior of composables; ensure every UI-emitting component has a modifier parameter for customization.
- đ Prefer stateless composables that accept state as input and are controlled by the caller, which improves reusability and testability.
- đ§ Parameters should be ordered logically, starting with required parameters indicating the main purpose, followed by modifiers and optional parameters with defaults.
- đ Use default parameter values and nullability to convey meaningful API descriptions, distinguishing between default, empty, and absent values.
- đ§© Slot parameters or slot content allow for flexibility within components, enabling users to insert custom content while the component handles its main responsibilities.
- đ Ensure API documentation is thorough, including capabilities, parameters, expectations, and usage examples, and maintain backward compatibility for long-term stability.
Q & A
What is the basic building block in Jetpack Compose?
-The basic building block in Jetpack Compose is the composable function, which is used to write UI components that call other functions.
Why is it important to establish guidelines for writing Compose code?
-Establishing guidelines for writing Compose code is important for creating high-quality, scalable code that is easier to evolve with minimum friction, consistent across the Compose ecosystem, and follows known patterns to guide others towards better practices.
What are the three parts of the best practices and guidelines for developing idiomatic Compose APIs?
-The three parts are: planning for your components, leveraging Kotlin and naming conventions to define a solid structure of your API, and verifying and maintaining these APIs.
What should be the approach when considering creating a new API, like a FilterChip?
-When considering creating a new API, start by determining if the API is meant to solve a single problem and ensure it is use-case based, making it easy and clear to use.
What is the concept of layered higher-level APIs in Compose?
-Layered higher-level APIs in Compose are more constrained and provide specific behavior and defaults with fewer customization options. They are usually built on top of extracted lower-level components that define a common surface and expectations for all layers.
How should naming be approached for composable functions in Compose?
-Composable functions should be named using PascalCase and a noun, with or without prefixes, to establish a persistent identity across recompositions and reinforce the declarative model of describing UI with Compose.
Why is it recommended to use explicit parameters for defining an API's purpose and requirements?
-Explicit parameters make it easy to present, adjust, preview, test, and use the API. They also ensure the API is readable from the start and easier for users to understand at a glance.
What is the significance of modifiers in Compose and how should they be used in API parameters?
-Modifiers define the composable behavior and appearance. They should be at the top of the mind when defining API parameters, as Compose users already have expectations around their use. Every component that emits UI should have a modifier parameter that is the first optional parameter, has a default no-op value, and is the only parameter of its type.
How should the order of parameters be determined when defining an API?
-The order should prioritize readability and ease of use, starting with required parameters indicating the main purpose, followed by the modifier for customization, optional parameters with default values, and a trailing composable lambda for nested content if needed.
Why is it preferable to use stateless composables in API design?
-Stateless composables that hold no state of their own but instead accept it as input are more reusable and testable, as the caller dictates the changes and the component follows, a practice known as state hoisting.
How can an API be made more testable and what are some considerations for testability?
-An API can be made more testable by avoiding the use of platform-specific resources, providing explicit parameters instead of implicit ones, exposing all possible states as parameters, and using interactionSource for complex states. It's also important to ensure that the API can be easily verified in isolation.
What are some key considerations for API documentation and backward compatibility?
-Documentation should clearly communicate the API's capabilities, purpose, parameters, expectations, and provide usage examples following KTDoc guidelines. For external usage, ensuring backward compatibility is crucial for long-term stability and maintenance.
How does the concept of 'one component, one problem' benefit API design in Compose?
-'One component, one problem' ensures that the API is focused and use-case based, making it easier to understand and use, and reducing the complexity of the component.
Outlines
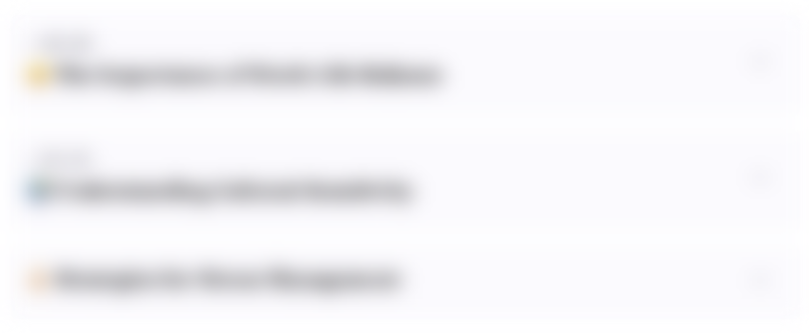
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
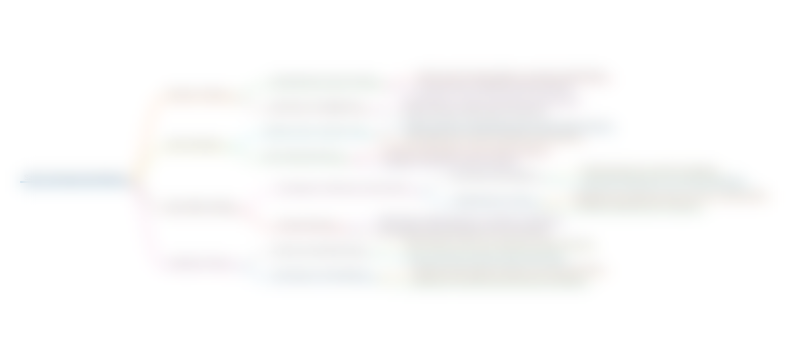
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
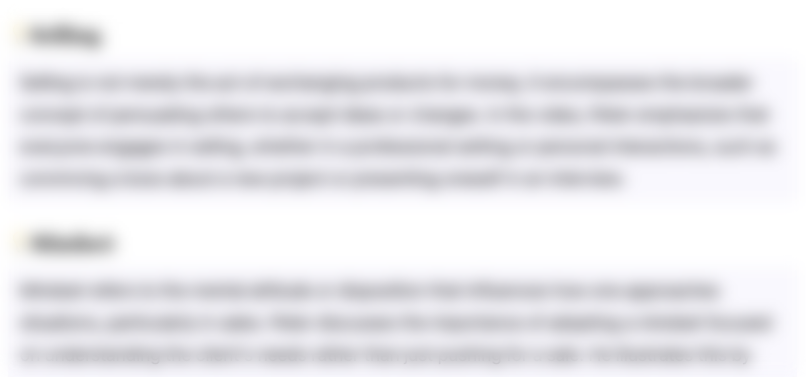
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
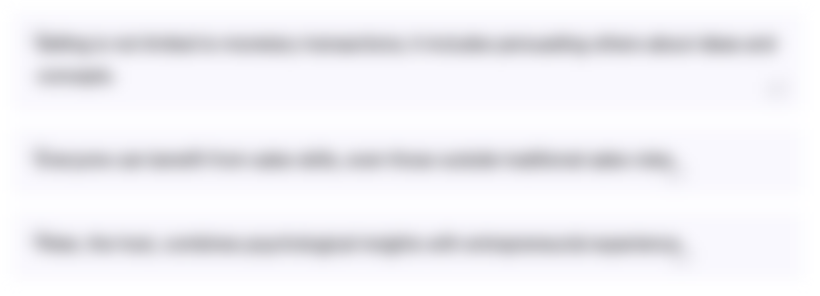
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
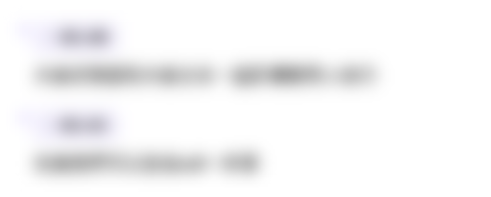
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
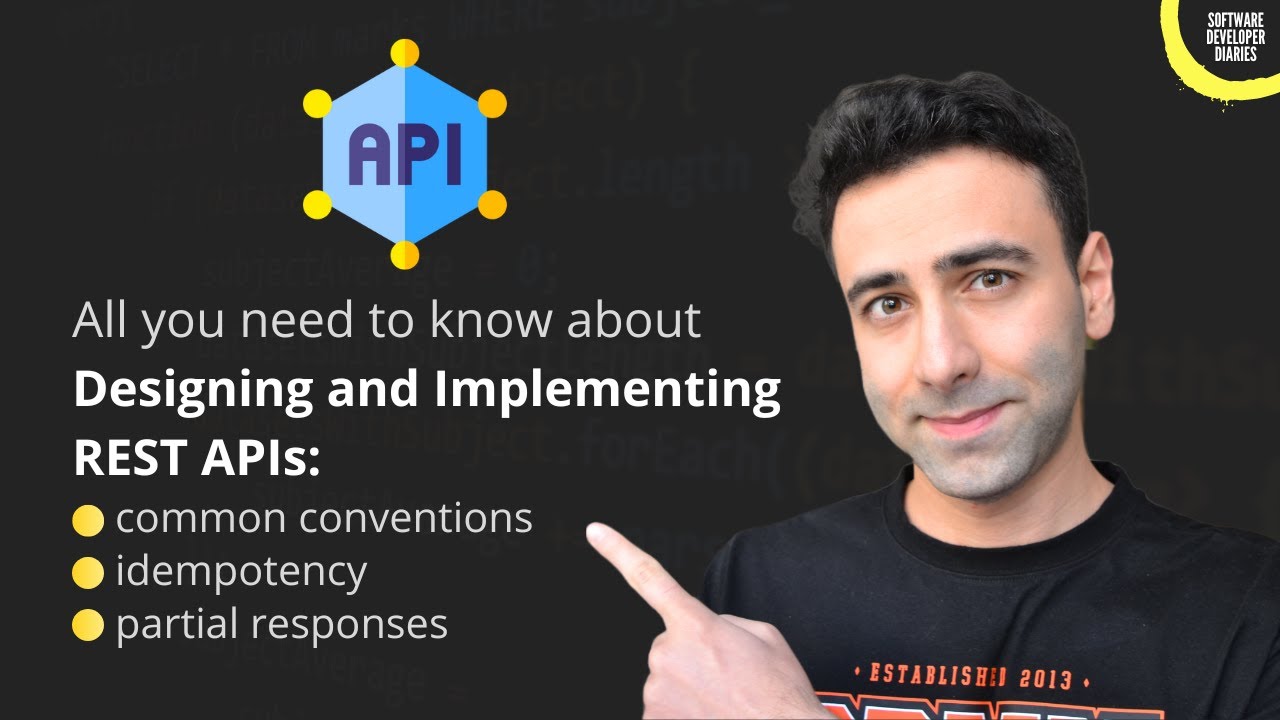
Deep Dive into REST API Design and Implementation Best Practices

The Right Way To Build REST APIs
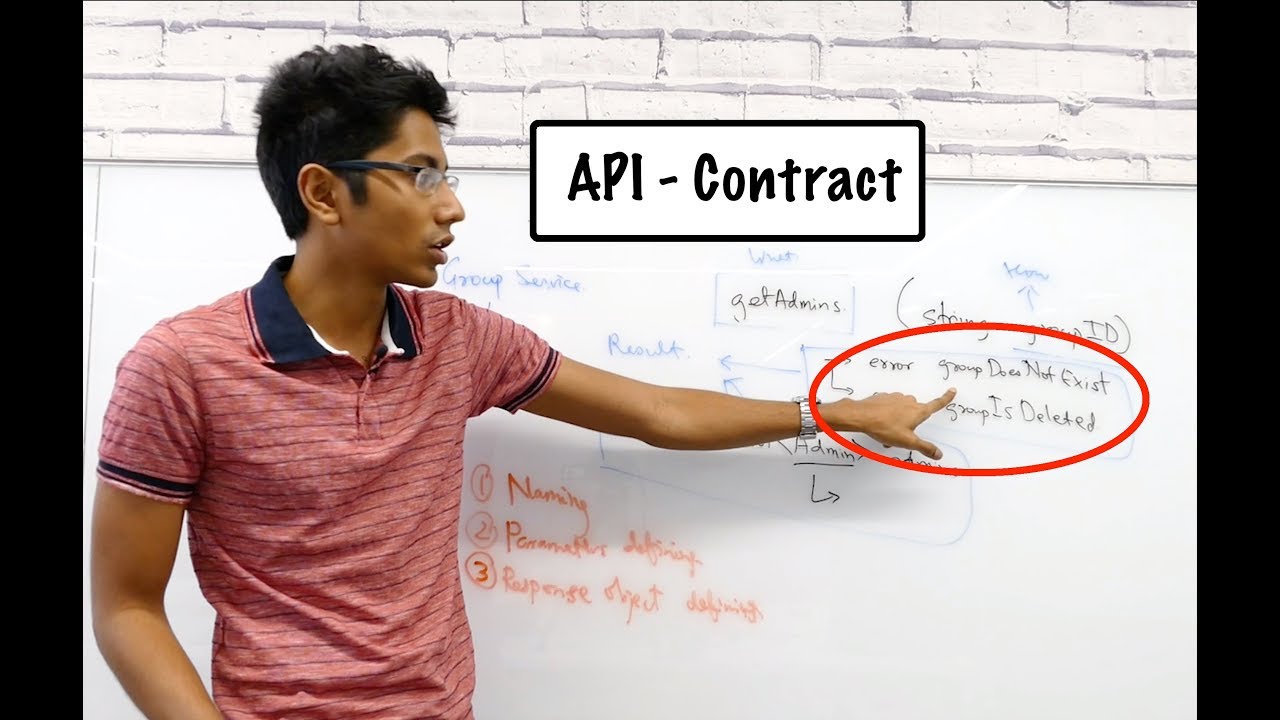
What is an API and how do you design it? đïžâ
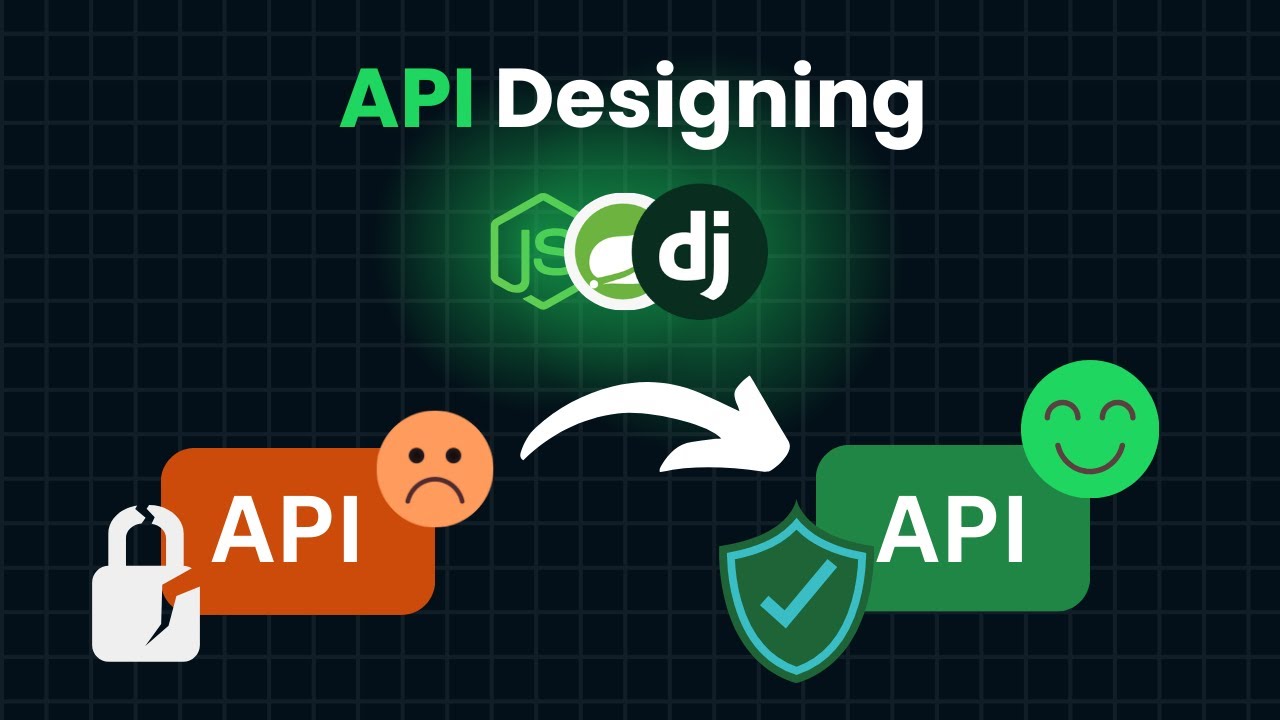
API designing: How to Design Best APIs | Best Practices | #api #backenddevelopment

Intuitive: Thinking in Compose - MAD Skills
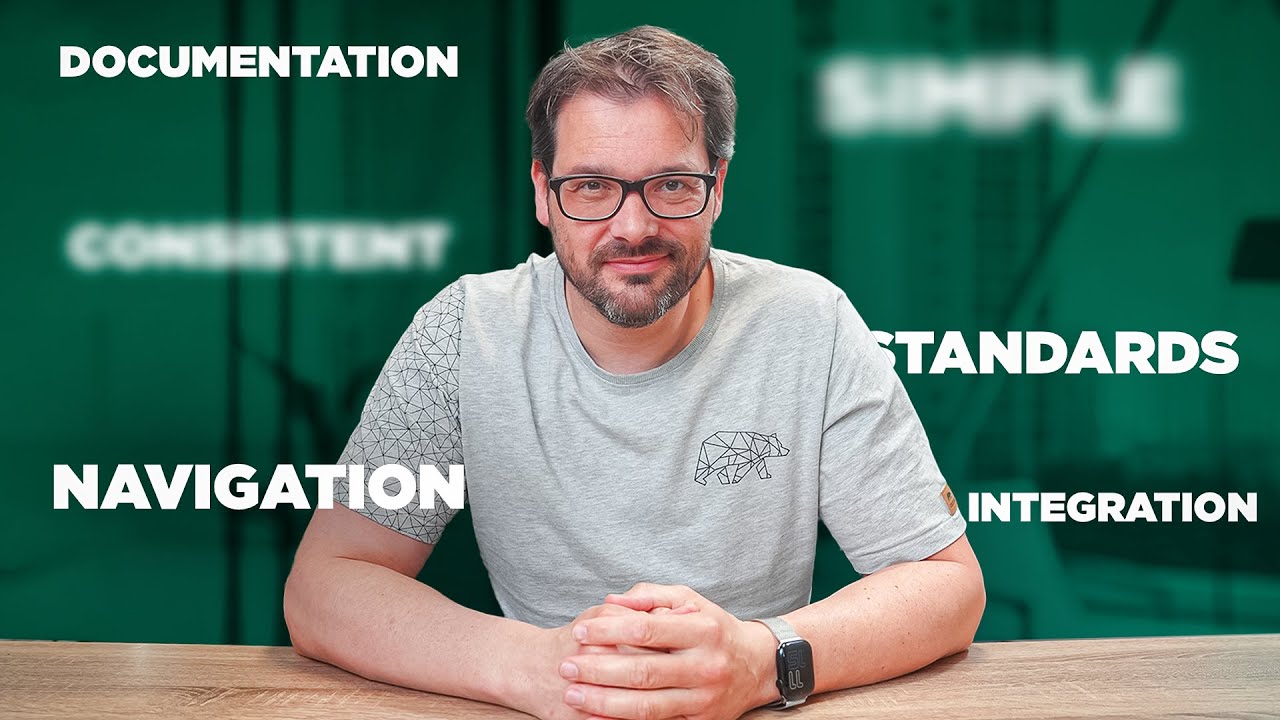
How to Design a REST API That Doesnât SUCK
5.0 / 5 (0 votes)