Django Testing Tutorial with Pytest #3 - Coverage (2018)
Summary
TLDRThis video tutorial teaches viewers how to refactor common setup code into functions and measure test coverage in Django. It demonstrates using the 'setUpClass' method to streamline test setup, reducing redundancy and improving performance. The video also guides on setting up a test coverage report with 'coverage', customizing it to exclude unnecessary files, and viewing results in an HTML format for a clear understanding of code execution coverage.
Takeaways
- 🔧 Extract common setup code into its own function to improve code organization and performance.
- 📈 Use Django's TestCase for unit testing to gain access to the 'setUpClass' method for shared setup tasks.
- 📝 Call the 'super().setUpClass()' to ensure the base class's setup is executed before custom setup code.
- 🔄 Instantiate shared objects like 'requestfactory' once in 'setUpClass' to avoid redundancy and improve efficiency.
- 📑 Utilize 'setUpClass' as a decorator to ensure the method is run once for the class, not every time a test is executed.
- 📊 Set up test coverage reports to measure the effectiveness of your tests and identify untested code.
- 🛠️ Install necessary packages to enable test coverage reporting if not already present in your environment.
- 📋 Use the 'coverage' flag with 'pytest' to generate a coverage report, indicating the percentage of code executed during tests.
- 📁 Exclude irrelevant files such as test files, migrations, and certain configuration files from the coverage report for accuracy.
- 📝 Create a '.coveragerc' file to specify directories and files to omit from the coverage report for cleaner results.
- 🌐 Use 'pytest' options like '--cov-report=html' to export the coverage report in an HTML format for easy visualization.
- 🔄 Regularly review and adjust test coverage to ensure all critical parts of the application are adequately tested.
Q & A
What is the main purpose of the video?
-The video teaches viewers how to refactor common setup code into a separate function and how to measure test coverage in a Django project.
Why is it necessary to extract the mixer of blend line code into a separate function?
-Extracting the mixer of blend line code into a separate function is necessary because it's not part of the main function's behavior but is required for the code to run successfully, avoiding failures due to missing objects with a private key of one.
What is the benefit of instantiating the requestfactory object only once?
-Instantiating the requestfactory object only once improves performance, especially when there are many test functions, as it reduces the overhead of creating the object multiple times.
How does the 'setup_class' method in Django's TestCase work?
-The 'setup_class' method in Django's TestCase is a class method that runs before all other test methods in the class. It is used for setting up code that needs to be executed once for the entire test class.
What is the purpose of the 'super' function in the 'setup_class' method?
-The 'super' function is used to call the 'setup_class' method of the parent class (TestCase) to ensure that any setup actions defined in the parent class are also executed.
Why is it important to measure test coverage in a project?
-Measuring test coverage is important to ensure that the codebase is adequately tested. It helps identify untested parts of the code which could lead to potential bugs or issues in production.
What does the '--coverage' flag do when running tests with 'manage.py test'?
-The '--coverage' flag generates a test coverage report, showing which parts of the code are covered by tests and which are not.
How can you specify which files to exclude from the test coverage report?
-You can specify files to exclude from the test coverage report by setting the 'omit' option in the '.coveragerc' file to the paths of the directories or files you want to omit.
What is the benefit of exporting the test coverage report to an HTML format?
-Exporting the test coverage report to an HTML format makes it easier to navigate and visually inspect which parts of the code are covered by tests. It also provides a more user-friendly way to review the report.
How can you open and view the HTML coverage report in an editor like Atom?
-You can open and view the HTML coverage report in Atom by installing the 'atom-html-preview' package, then opening the 'index.html' file and using the shortcut 'Ctrl + Shift + H' to preview it.
What should you consider when deciding which files to include or exclude from test coverage measurement?
-You should consider excluding files that do not require testing, such as test files themselves, migration files, and certain configuration files like 'wsgi.py'. These files are typically not the focus of test coverage as they are either tested by definition or do not contain executable business logic.
Outlines
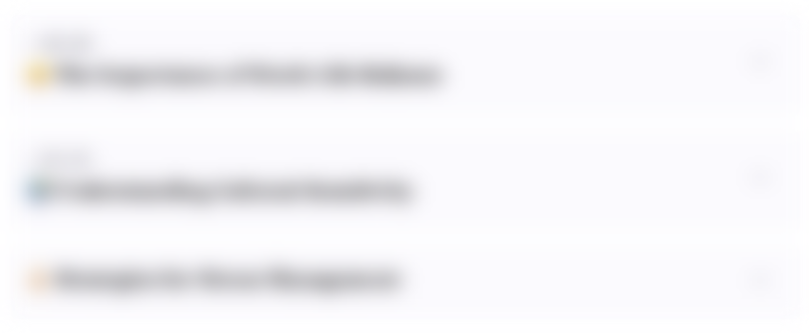
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
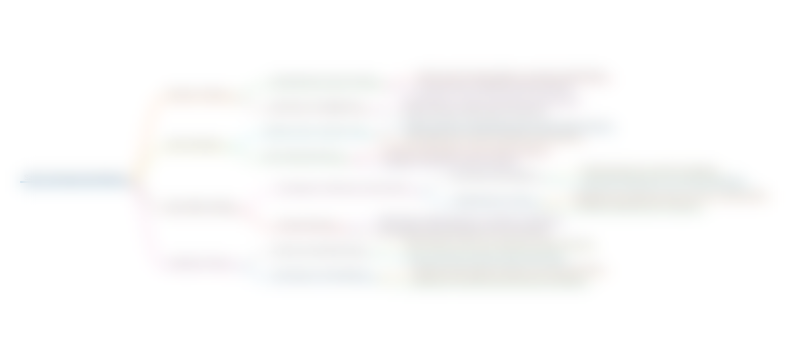
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
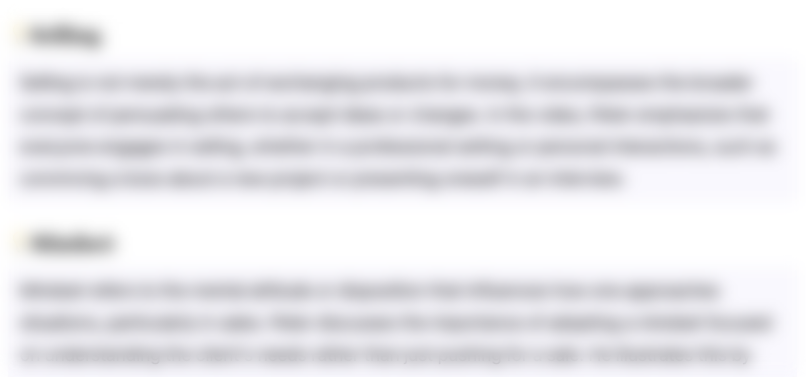
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
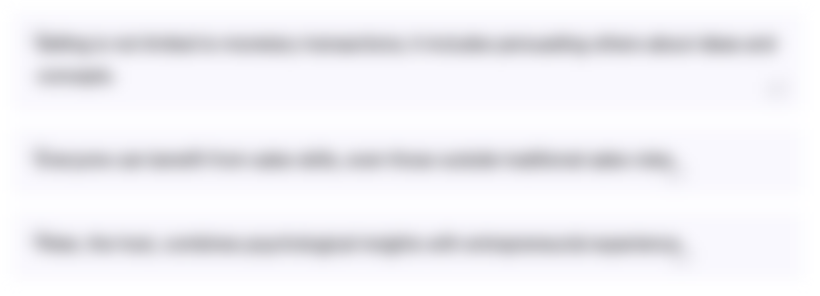
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
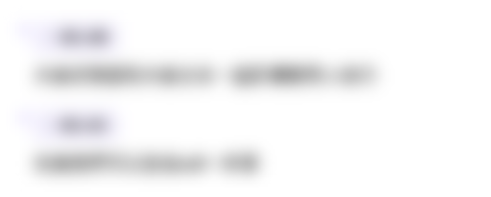
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
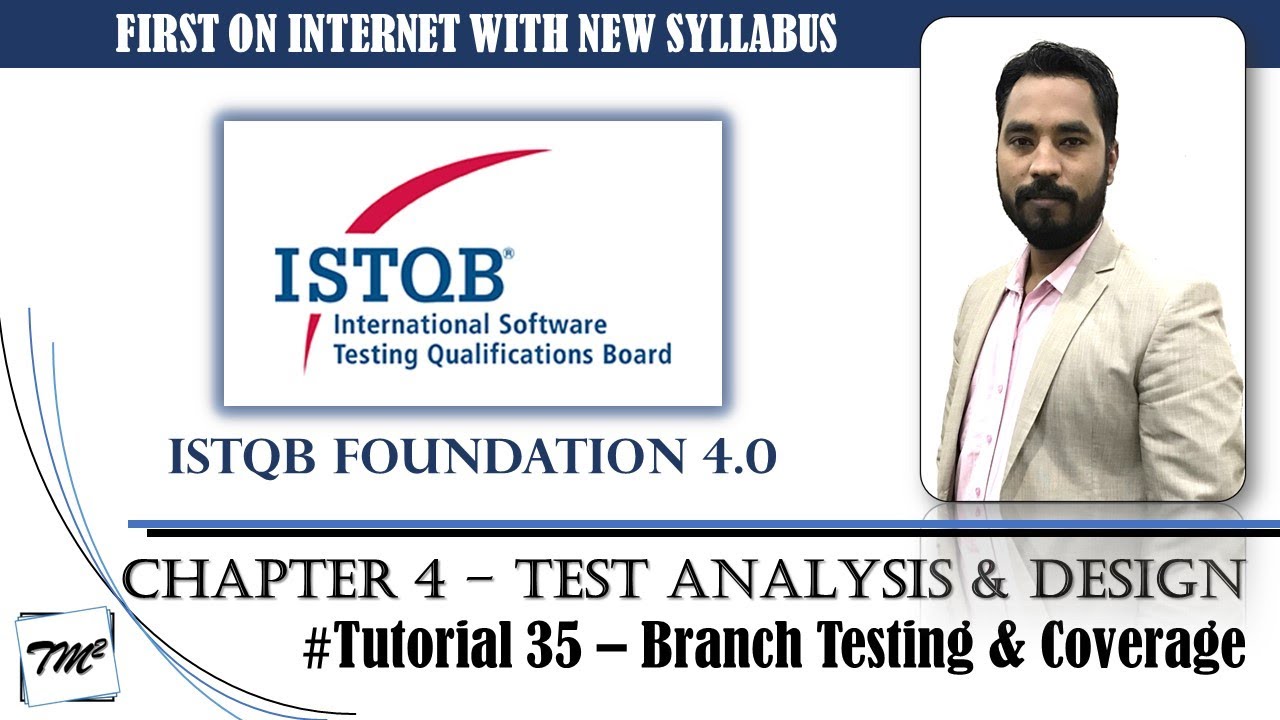
ISTQB FOUNDATION 4.0 | Tutorial 35 | Branch Testing & Branch Coverage | Test Case Techniques | CTFL

Criando Métodos de Objetos - Curso Python Orientado a Objetos [Aula 03]
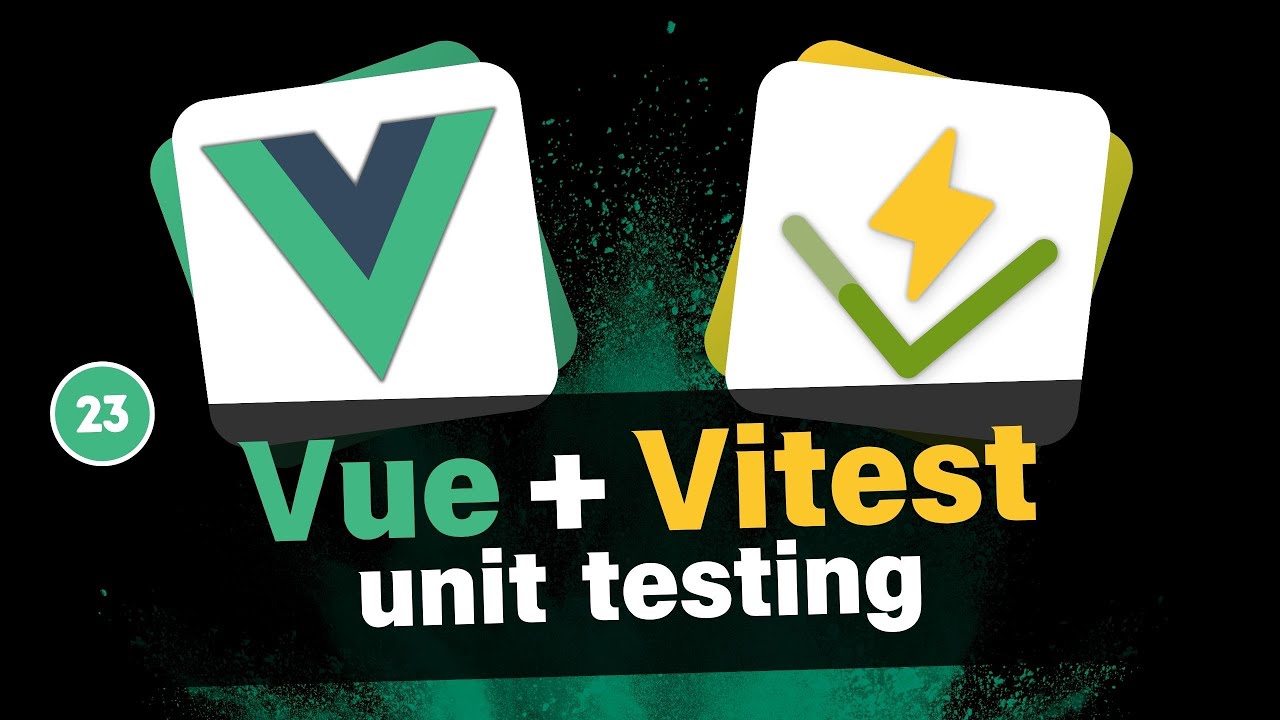
Vue Unit Testing #23 - Activity item component test
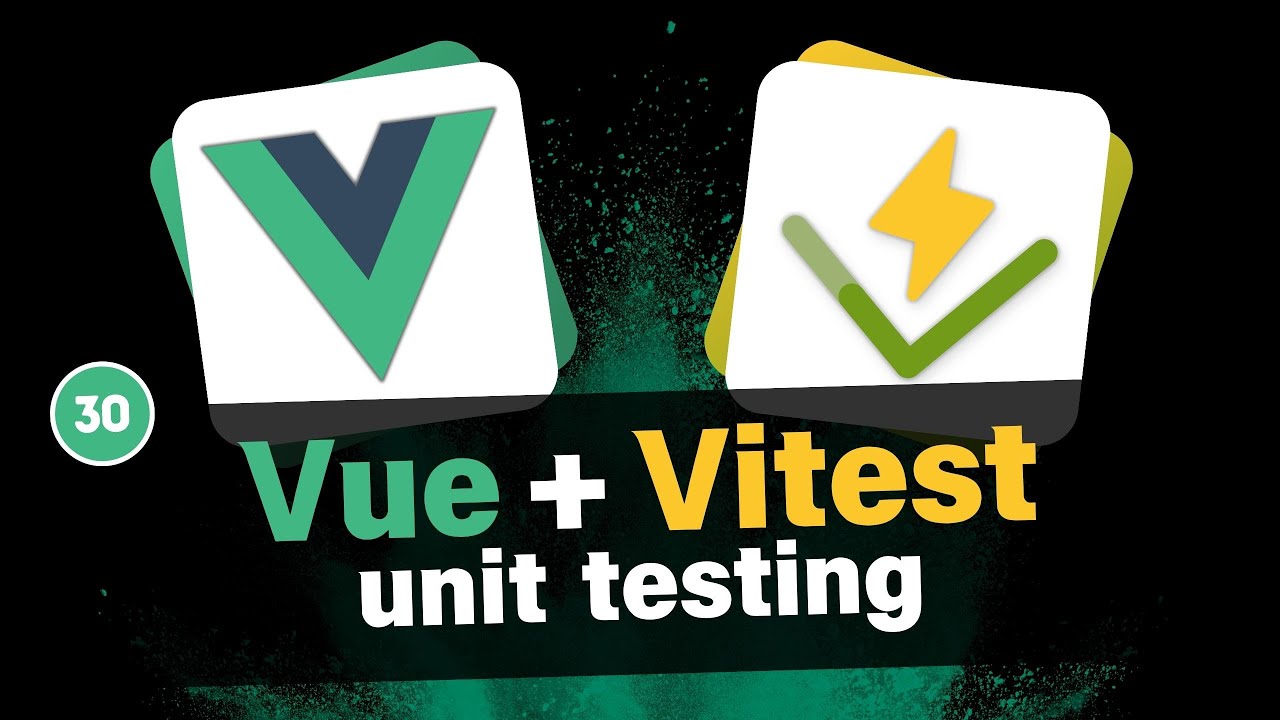
Vue Unit Testing #30 - Nav item component test
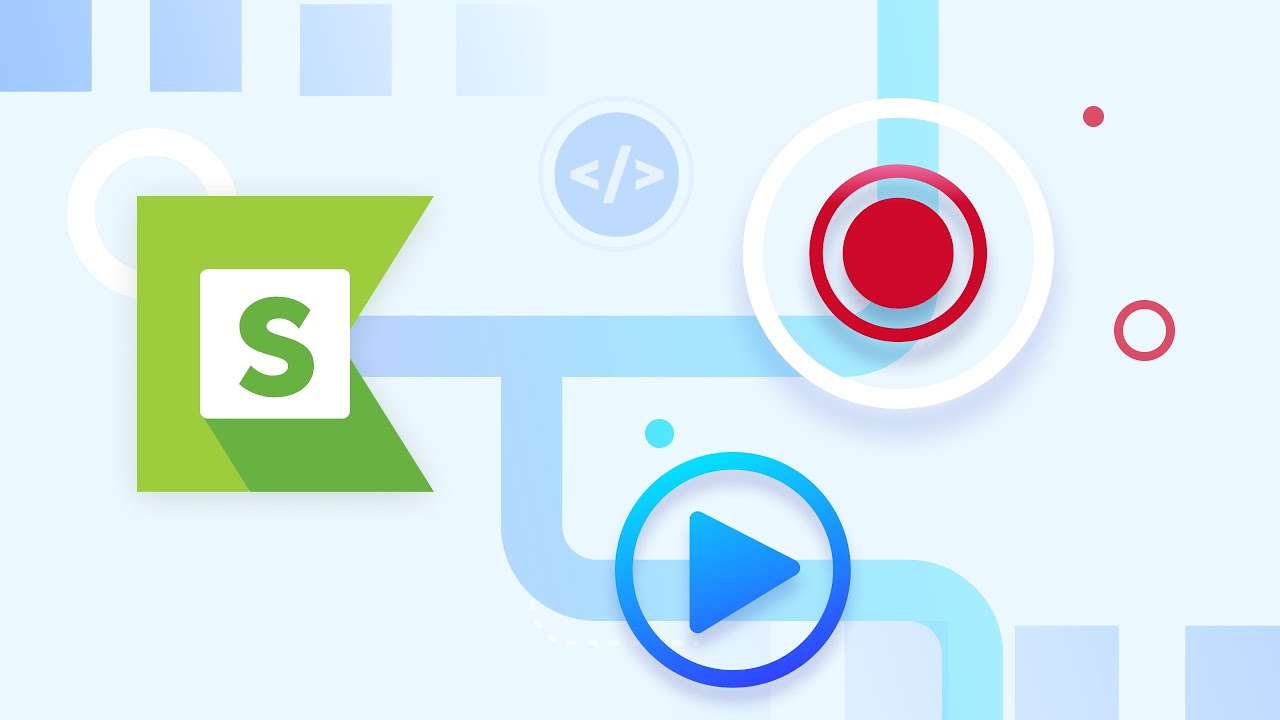
Katalon Academy: Create Automated Tests with Record & Playback (Intro)

Branch Coverage - Georgia Tech - Software Development Process
5.0 / 5 (0 votes)