Django Testing Tutorial with Pytest #2 - Unit Testing (2018)
Summary
TLDRThis tutorial offers a step-by-step guide on setting up and writing tests for a Django project. It begins with configuring a 'settings.py' file for testing, then moves on to creating test files for URLs, models, and views. The script demonstrates testing URL paths, model functions, and view access with both authenticated and unauthenticated users. It also touches on using the 'mixer' library for creating mock data and emphasizes the importance of testing to ensure project robustness.
Takeaways
- 📝 Start by setting up a file named 'pi_tests.py' in the home directory to specify the Django settings module for tests.
- 🔧 Consider creating separate settings for production and testing to avoid issues like sending emails during tests.
- 📁 Create a 'test' folder to house all test files and a 'tests_urls.py' file to begin testing URLs.
- 🔗 Use Django's 'reverse' function to get the URL path for a given view and 'resolve' to check if the URL resolves correctly to a view.
- 📝 Write test functions within a class dedicated to URL tests, ensuring each function has a single assert statement for clarity.
- 🛠️ Utilize 'mixer' to create instances for testing model methods without manually specifying all model fields.
- 🔍 Test model methods like 'is_in_stock' by creating product instances with varying quantities and asserting the correct outcomes.
- 📑 In 'tests_views.py', write tests for views ensuring they behave correctly with authenticated and unauthenticated users.
- 👤 Test views that require user authentication by mocking requests with an authenticated user and checking for the correct response.
- 🚫 For unauthenticated user tests, assert that the response redirects to the login page and contains the expected URL.
- 🔄 Address database access issues in tests by using the '@pytest.mark.django_db' decorator to enable database access for test cases.
Q & A
What is the purpose of the 'PI tests.py' file in the Django testing setup described in the script?
-The 'PI tests.py' file is used to configure the test environment for a Django project. It specifies the settings module to be used for testing, which is typically different from the production settings to avoid side effects like sending out emails during tests.
Why is it recommended to create a separate settings file for testing in a production environment?
-Creating a separate settings file for testing in production helps to avoid unintended consequences such as sending emails or making changes to the database that could interfere with the live system.
What is the significance of the 'reverse' function in Django testing?
-The 'reverse' function in Django is used to obtain the URL path for a given view name and parameters. It's the equivalent of the URL in the templates and is used to ensure that the URLs are correctly resolving in tests.
What is the role of the 'resolve' function in the context of testing URLs in Django?
-The 'resolve' function is used to revert a URL path back to a view function. It's essentially the opposite of the 'reverse' function and helps in testing that the correct view is being called for a given URL.
Why is it suggested to have only one assert statement per test function, and when should you split it up?
-Having one assert statement per test function makes it easier to identify what each test is checking. If a test needs to perform more than one assertion, it's recommended to split it into multiple functions to keep the tests focused and easier to maintain.
What is the purpose of the 'mixer' library in the context of creating test instances for models in Django?
-The 'mixer' library is used to create test instances of models quickly and easily. It allows for the specification of which fields should be filled in, simplifying the process of setting up test data for unit tests.
How does the 'is_in_stock' function work in the product model, as described in the script?
-The 'is_in_stock' function in the product model checks whether the quantity of a product is greater than zero. If the quantity is greater than zero, it returns True, indicating that the product is in stock.
What does the 'Django DB mark' decorator do, and why is it used in the test functions?
-The 'Django DB mark' decorator is used to mark a test as requiring access to the database. It ensures that the test will run with a real database connection, which is necessary for tests that interact with the database.
Why is it important to test both authenticated and unauthenticated access to views in Django?
-Testing both authenticated and unauthenticated access ensures that the view behaves correctly under different user conditions. It helps to verify that authentication requirements are enforced and that unauthenticated users are redirected appropriately.
What is the expected outcome when an unauthenticated user tries to access a view that requires authentication in Django?
-When an unauthenticated user tries to access a view that requires authentication, the expected outcome is a redirect to the login page. The test should assert that the response includes a redirect to the login URL.
How can you measure test coverage in a Django project, as hinted at the end of the script?
-Test coverage in a Django project can be measured using tools like 'coverage.py', which analyzes the parts of the codebase that are covered by tests. The script suggests that future parts of the tutorial will delve into this topic.
Outlines
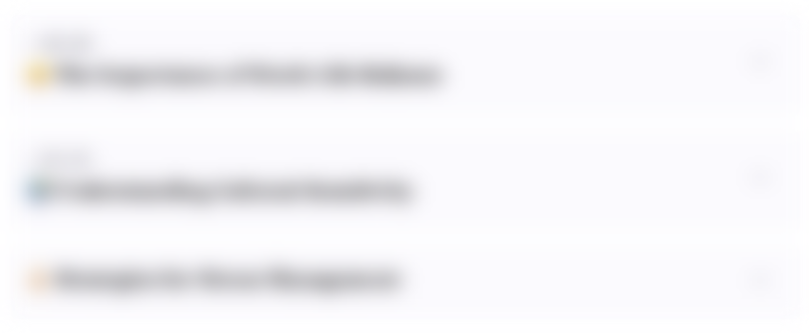
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
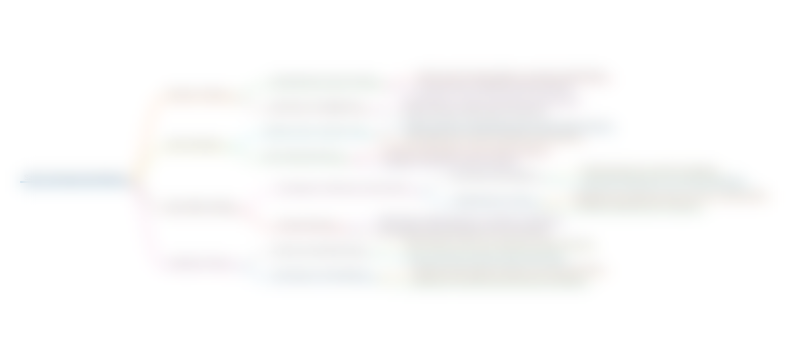
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
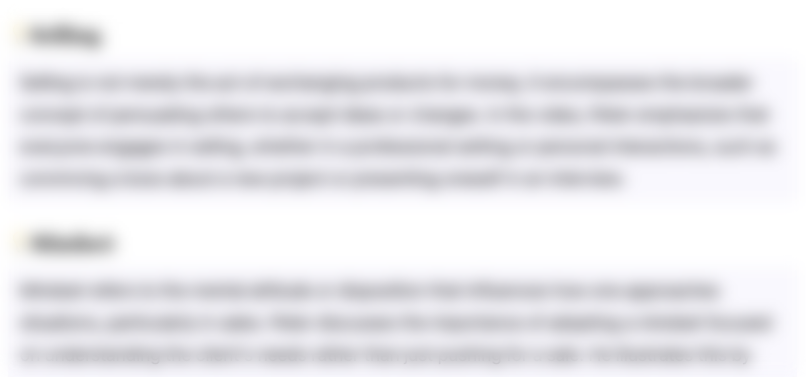
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
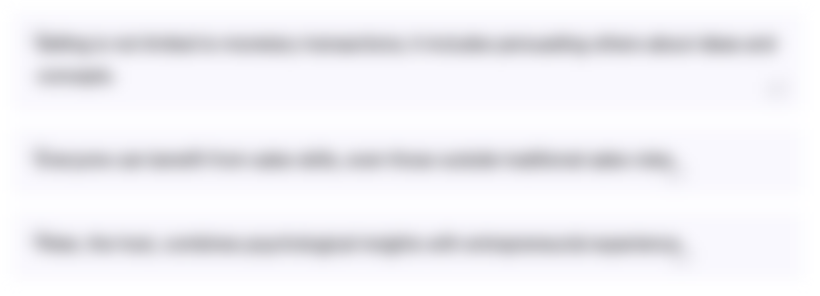
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
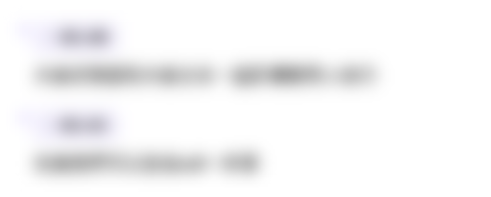
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
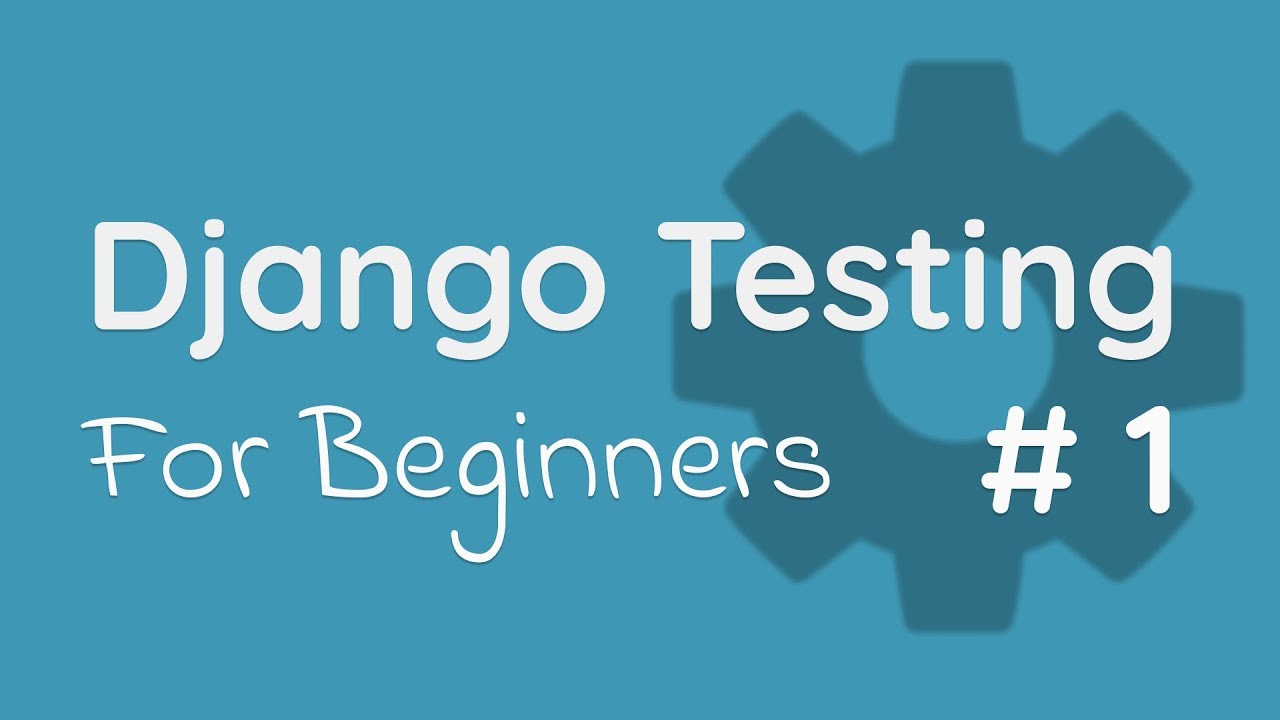
Django Testing Tutorial with Pytest #1 - Setup (2018)
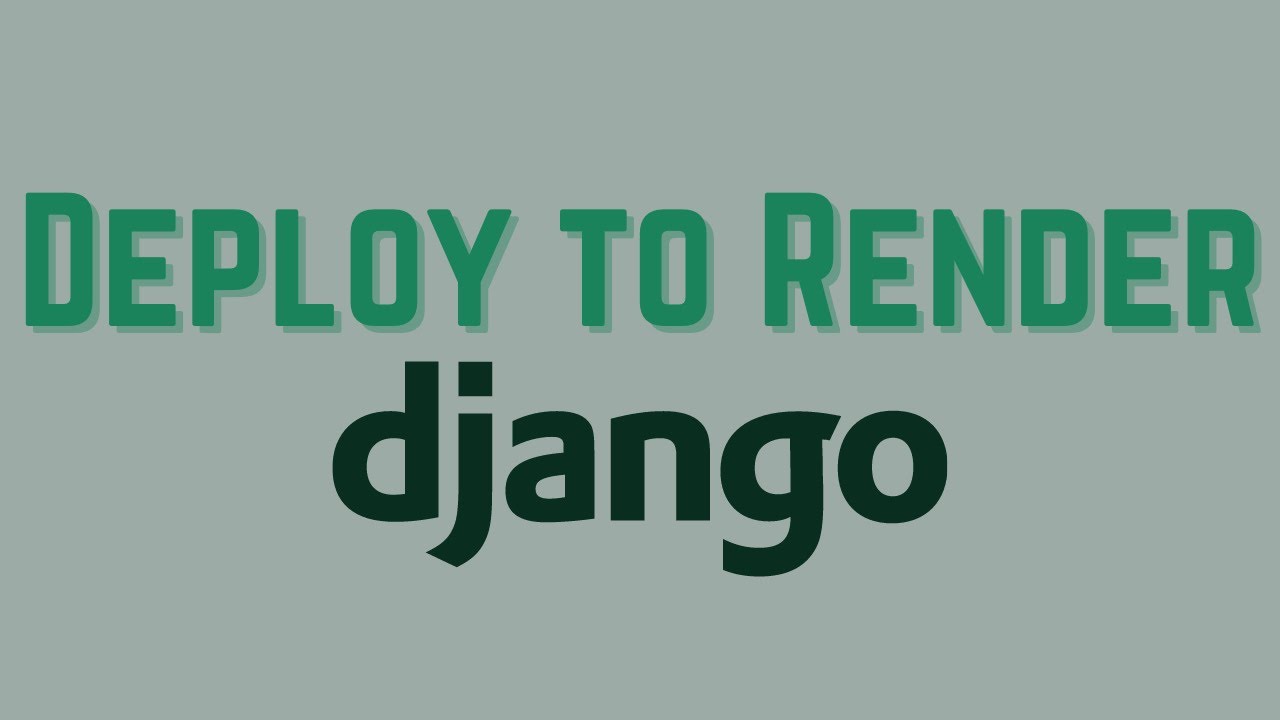
How to Deploy a Django App and Postgres Database to Render
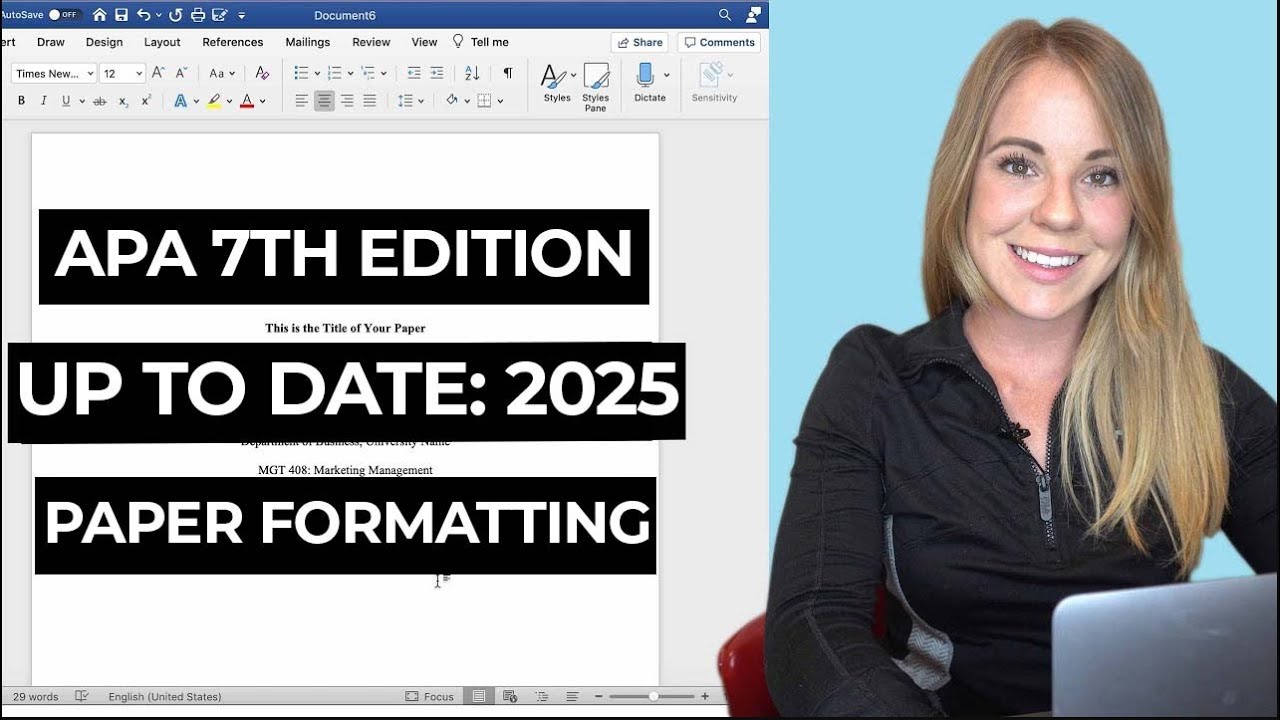
How to format your paper in APA style in 2024

Prinsip Kerja Sensor Ultrasonic | Ultrasonic Sensor HC-SR04 Dengan Arduino

Earn $3 Every 5 Minutes On Your Phone 2024 #money #moneytips #moneyonline
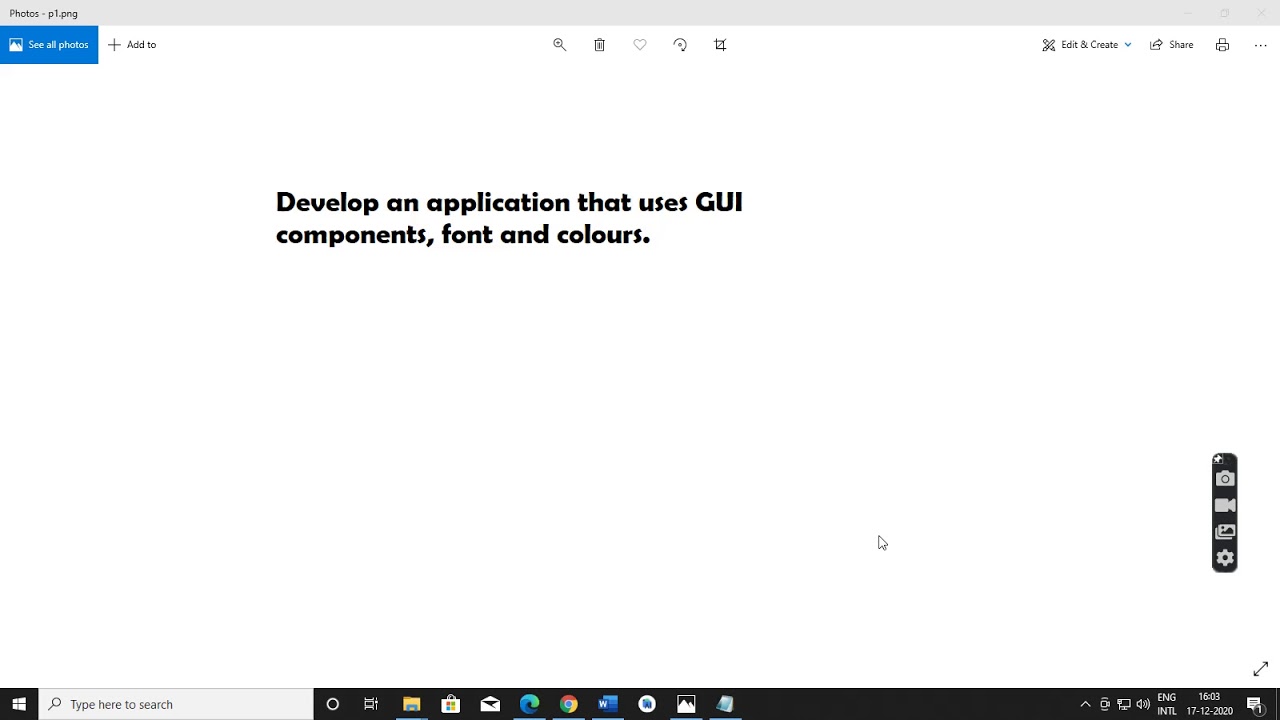
Android application that uses GUI components, font and colors - 01
5.0 / 5 (0 votes)