Django Testing Tutorial with Pytest #1 - Setup (2018)
Summary
TLDRThis tutorial series introduces viewers to the fundamental concepts of testing Jenga applications. By the end, participants will grasp the testing lifecycle's role in project integrity and learn to write their own tests. The script guides through setting up a Django project, creating a 'Product' model with stock checks, and setting up views and templates. It also covers initial testing steps, including making a view login-required, and installing necessary packages for further testing, promising a comprehensive guide for ensuring application reliability.
Takeaways
- 📝 This tutorial series aims to teach the major concepts of testing a Jenga application, focusing on the testing lifecycle and how it should be integrated into projects.
- 🔍 Automated testing involves writing code to test other code snippets, providing confidence that the production code is functioning correctly and allowing for safe changes without breaking the application.
- 💻 The setup begins with creating a new Django project named 'testing' and then an app called 'friendship' with a 'products' model.
- 🛠️ A 'Product' model is defined with fields for name, description, price, quantity, and published date, including a method to check if the product is in stock.
- 📝 A property decorator is used to define a method that returns a boolean indicating whether the product quantity is greater than zero, signifying stock availability.
- 🔗 In the Django URL configuration, a path is added to redirect to a product detail view based on a primary key (PK).
- 📑 A view function 'product_detail' is created to fetch and render a product based on its ID, using Django's 'get_object_or_404' function for error handling.
- 📁 A 'templates' folder is added within the 'products' app to create an HTML template for displaying product details.
- 🔄 Database migrations are performed using 'manage.py makemigrations' and 'manage.py migrate' to apply changes to the database schema.
- 🚀 The server is run using 'manage.py runserver' to test the application, with manual creation of a product in the Django shell for demonstration.
- 🔒 The 'product_detail' view is updated to require user login, demonstrating how to restrict access to certain views for authenticated users only.
- 📦 Additional packages are installed for testing, including 'pytest', 'pytest-django', 'pytest-cov' for coverage, and 'mixer' for mocking database entries.
Q & A
What is the main goal of this tutorial series?
-The main goal of this tutorial series is to teach the major concepts of testing a Jenga application, enabling viewers to understand the testing lifecycle in projects and to write their own tests to ensure the integrity of their applications.
What does automated testing provide to a developer?
-Automated testing provides developers with the confidence that their code is working correctly. It allows them to make changes and run tests to ensure that nothing is broken, thus preventing end-users from having a negative experience with the application.
How does the tutorial start the process of setting up testing?
-The tutorial starts by creating a new project using Django admin startproject, naming it 'testing', and then setting up an actual app called 'friendship' with a 'products' model.
What are the fields defined in the 'Product' model?
-The 'Product' model defines fields for 'name' (a CharField with a max length of 100), 'description' (a TextField), 'price' (a DecimalField with five digits and two decimal places), 'quantity' (an IntegerField), and 'published_on' (a DateField).
What is the purpose of the 'is_in_stock' function in the 'Product' model?
-The 'is_in_stock' function is used to check if there are any units of the product left in stock. It returns a boolean value indicating whether the quantity of the product is greater than zero.
How is the 'product detail' view set up in the Django URL configuration?
-The 'product detail' view is set up by adding a new path in the URL configuration that leads to an integer named 'pk'. When this route is hit, it calls the 'product_detail' function, which is assigned the name 'product_detail'.
What is the purpose of the 'get_object_or_404' function imported in the 'product detail' view?
-The 'get_object_or_404' function is used to retrieve a product object by its primary key. If the product does not exist in the database, it returns a 404 error.
What is the significance of creating a 'templates' folder and a 'detailed.html' file in the 'products' app?
-The 'templates' folder and 'detailed.html' file are created to define the HTML template that will be rendered when a product detail page is accessed. It displays the product's name in an h1 tag.
How does the tutorial ensure that the 'product detail' view is accessible only to logged-in users?
-The tutorial modifies the 'product detail' view by adding the '@login_required' decorator to ensure that only logged-in users can access the view.
What packages are installed for testing purposes in the tutorial?
-The tutorial installs 'pytest', 'pytest-django', 'pytest-cov' for coverage testing, and 'mixer' for mocking database entries, to facilitate various aspects of testing.
Outlines
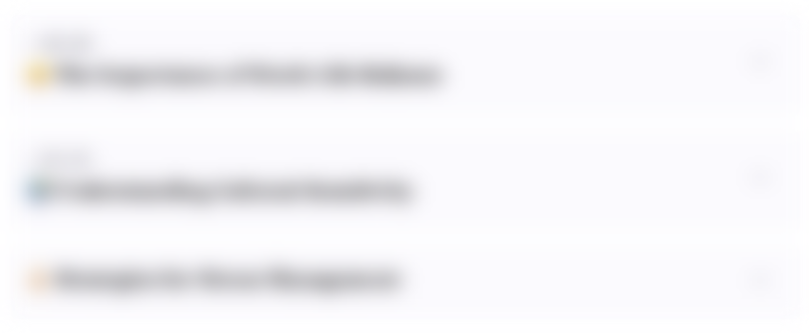
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
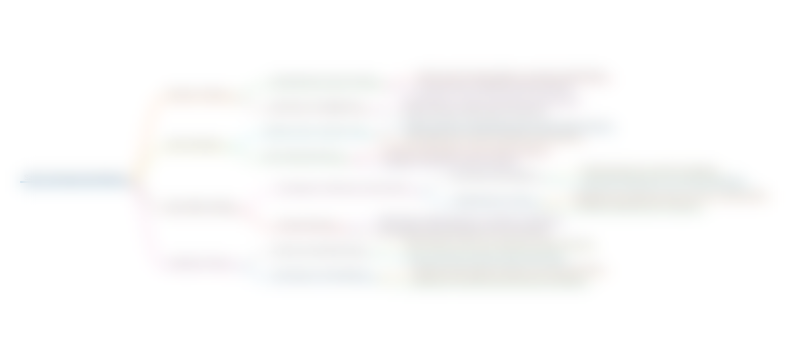
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
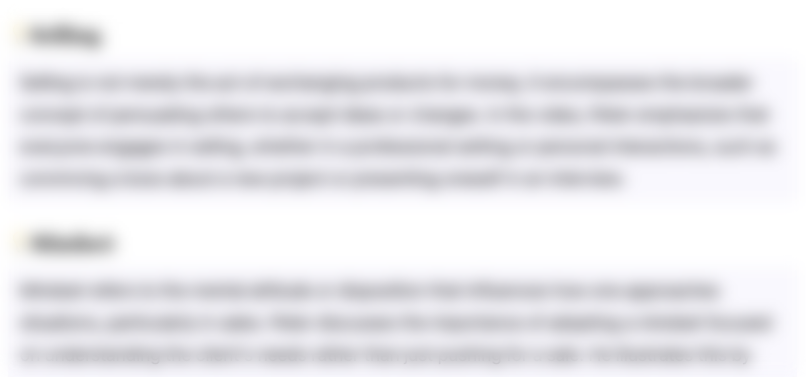
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
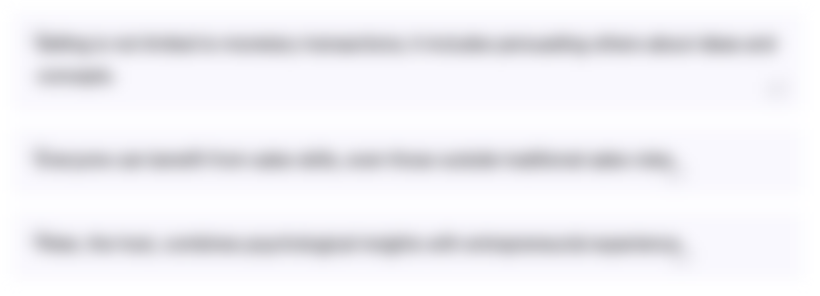
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
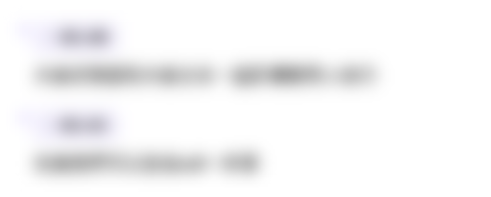
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

Appium Tutorial 01 :Introduction To Mobile App Testing | Appium
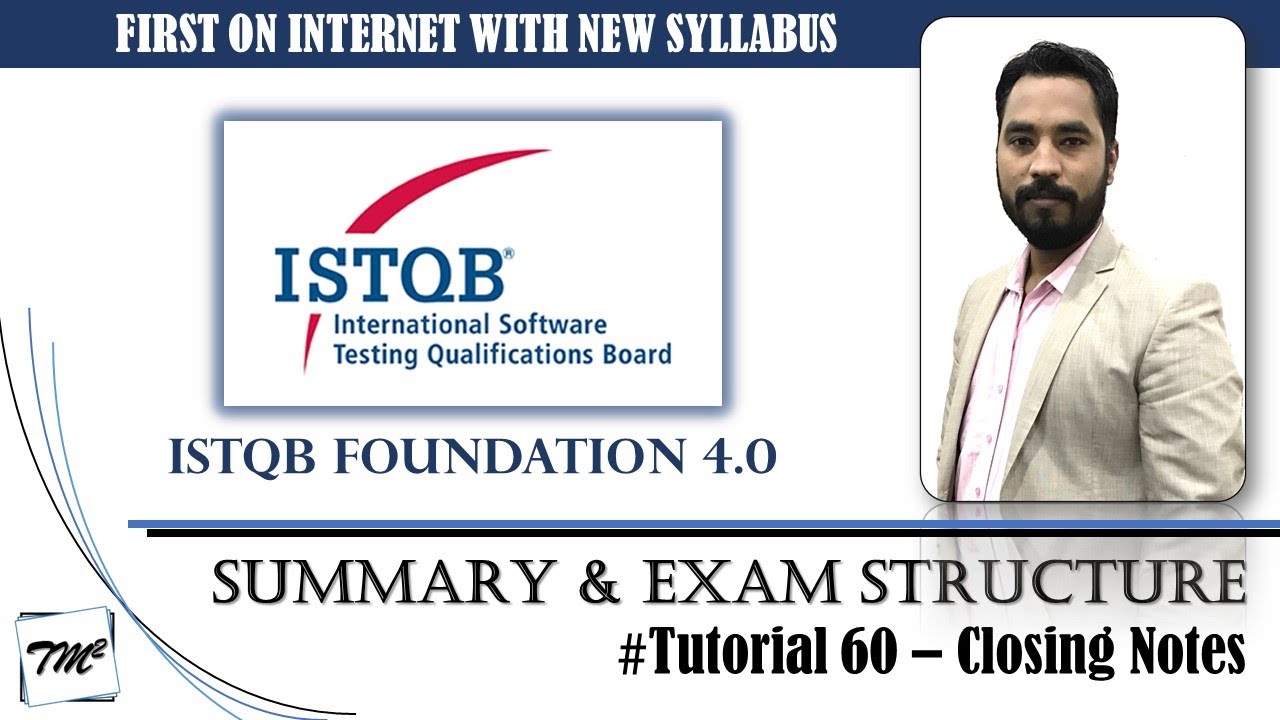
ISTQB FOUNDATION 4.0 | Tutorial 60 | Exam Details & Tips | ISTQB Foundation Level Summary in 30 Mins

Fisika Kelas XI : Massa Jenis, Tekanan Hidrostatis dan Hukum Utama Hidrostatis
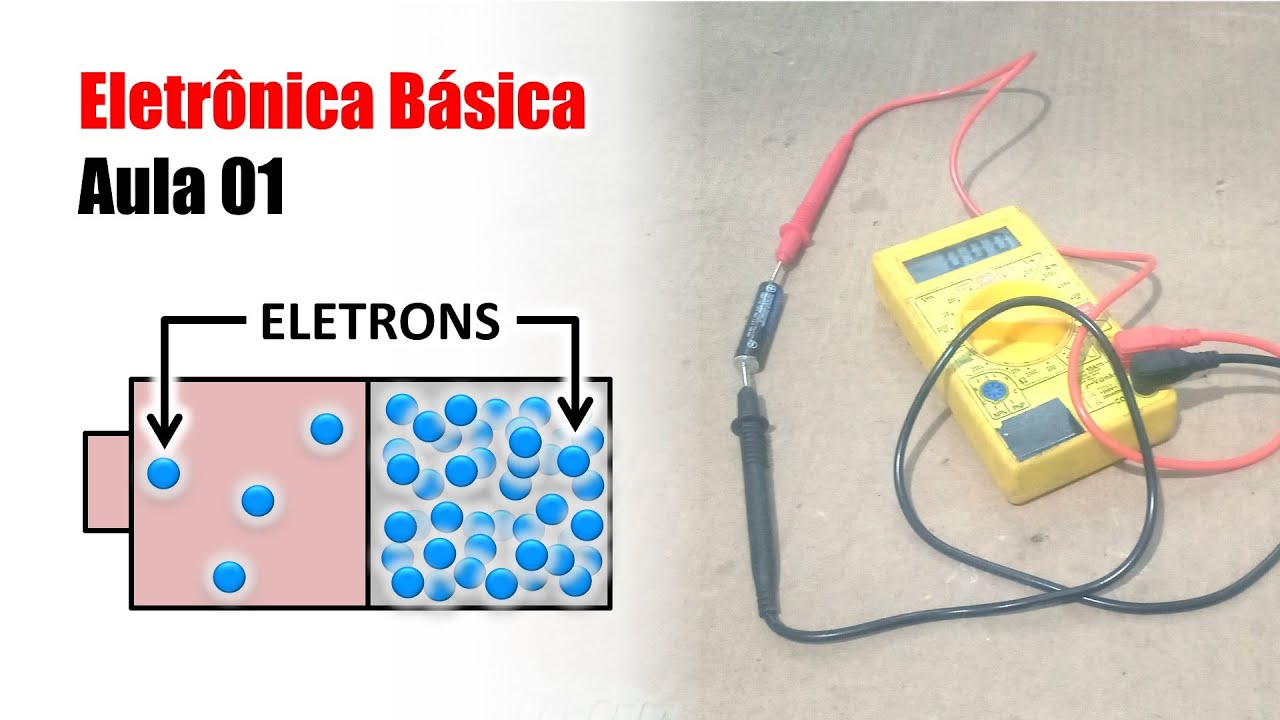
Eletrônica Básica para Iniciantes - Aula 01 - Como a Eletricidade Funciona?
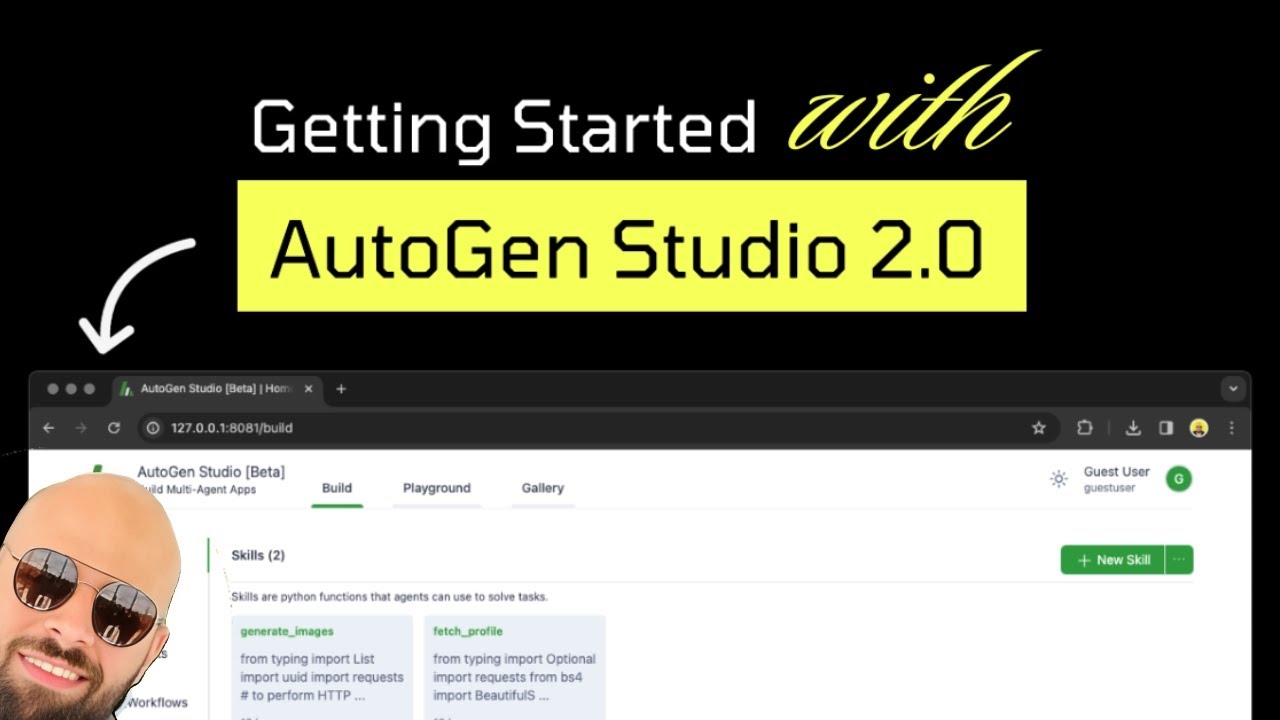
An overview of AutoGen Studio 2.0 in under 10 minutes!
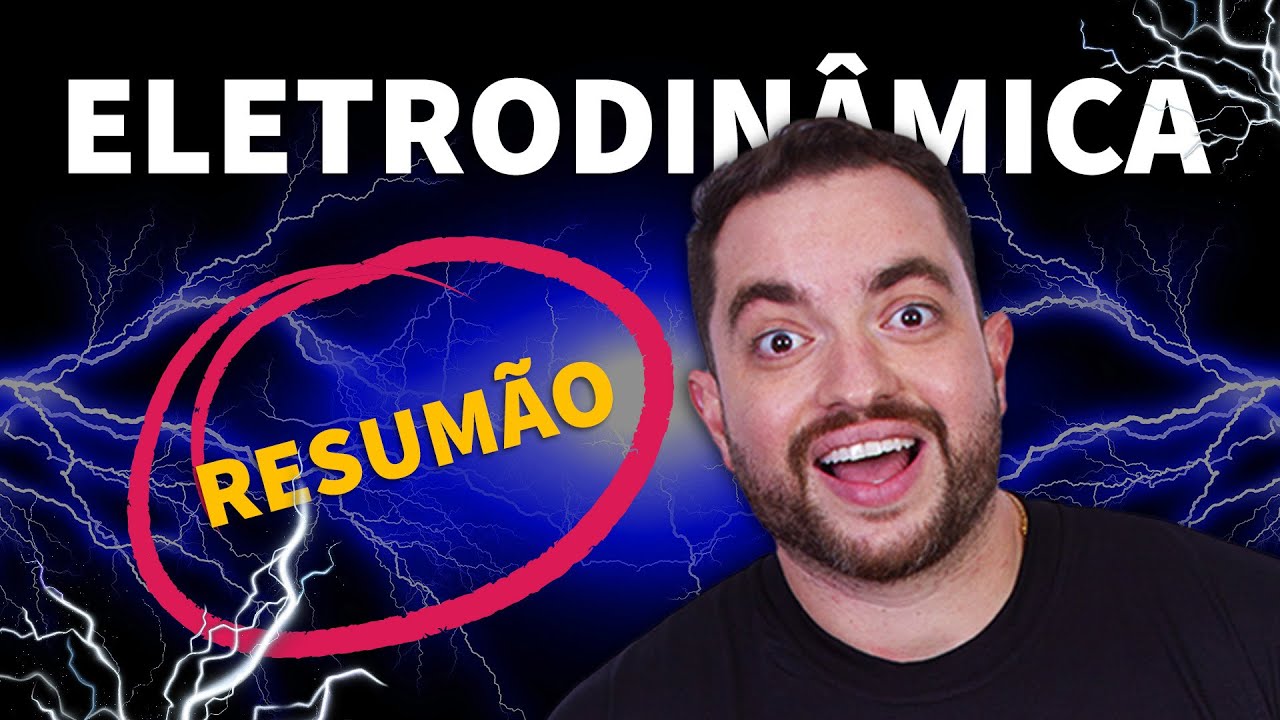
RESUMÃO: eletrodinâmica
5.0 / 5 (0 votes)