#3 Variables in JavaScript
Summary
TLDRIn this video, Davin Vetti introduces the basics of JavaScript, focusing on the importance of variables. He explains how variables store data temporarily for processing and how they can be reused. Davin demonstrates creating variables with the `let` keyword and emphasizes using meaningful names for better readability. The video covers common errors such as variable redeclaration and provides tips on handling strings and naming conventions. It also discusses how variables can be reassigned and modified, making them essential for dynamic programming. The session concludes with best practices for writing clean, readable code.
Takeaways
- đ Variables are essential for storing data temporarily in programming languages like JavaScript.
- đ In JavaScript, variables can store different types of data, such as numbers or strings.
- đ To declare a variable in JavaScript, you can use the keyword 'let' followed by the variable name and its value.
- đ You can print the value of variables using 'console.log' to check the output.
- đ JavaScript allows flexibility in naming variables, but itâs important to use meaningful names for better readability.
- đ When assigning strings to variables, use either single or double quotes to avoid syntax errors.
- đ JavaScript variables can store values that change during program execution, making them dynamic.
- đ Errors often occur when trying to redeclare a variable, and JavaScript will throw an error if you declare the same variable twice using 'let'.
- đ JavaScript variables can have names with numbers (but not start with a number), underscores, and dollar signs.
- đ Best practices for naming variables include using camelCase or snake_case for readability when the variable name contains multiple words.
- đ Always read error messages carefully when debugging your code to identify the issue and learn from it.
Q & A
What is the purpose of variables in JavaScript?
-Variables in JavaScript are used to store data temporarily. This data can then be manipulated or used in various operations within the code.
Why do we need variables in programming?
-Variables are essential because they allow us to store and manage data. In the context of programming, data needs to be stored so it can be processed, manipulated, or retrieved for further operations.
What is the difference between a variable and a permanent storage in programming?
-A variable is used to store data temporarily within a program. For permanent storage, you would use databases or files, which retain data even after the program ends.
How can you store a value in a variable in JavaScript?
-In JavaScript, you can store a value in a variable by using the 'let' keyword, followed by the variable name and an assignment operator (e.g., `let num = 4`).
What happens when you assign a value to a variable in JavaScript?
-When you assign a value to a variable, JavaScript creates a memory location for the variable and stores the assigned value there. This allows you to refer to the value by the variable name throughout the program.
Can you reuse the value stored in a variable multiple times?
-Yes, once a value is stored in a variable, you can reuse it multiple times within the code. This helps avoid repetitive calculations and improves code readability.
What happens if you try to declare a variable that already exists in JavaScript?
-If you try to redeclare a variable that already exists using the 'let' keyword, JavaScript will throw an error. You can only declare a variable once within a given scope.
What is the significance of using 'let' when declaring a variable in JavaScript?
-'let' is a keyword used to declare variables in JavaScript. It is part of modern JavaScript and is preferred over the older 'var' keyword because it provides better scope management and avoids some issues related to variable hoisting.
How do you handle text (strings) in variables in JavaScript?
-To store text or strings in JavaScript, you need to enclose the text in either single quotes or double quotes. For example, `let name = 'Naveen'` or `let name = "Naveen"`.
What should you do if you have special characters like apostrophes inside a string?
-If you have special characters like apostrophes inside a string, you can either escape them with a backslash (e.g., 'Naveen's phone') or use a different type of quote for the string (e.g., `let name = "Naveen's phone"`).
Outlines
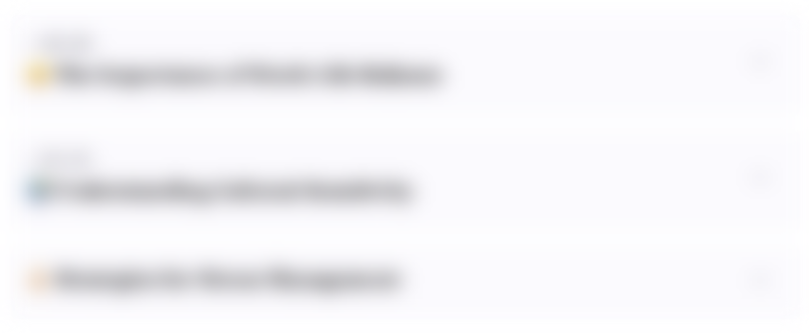
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
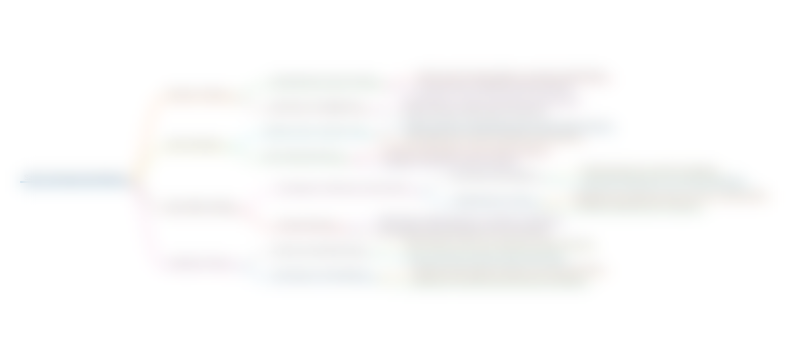
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
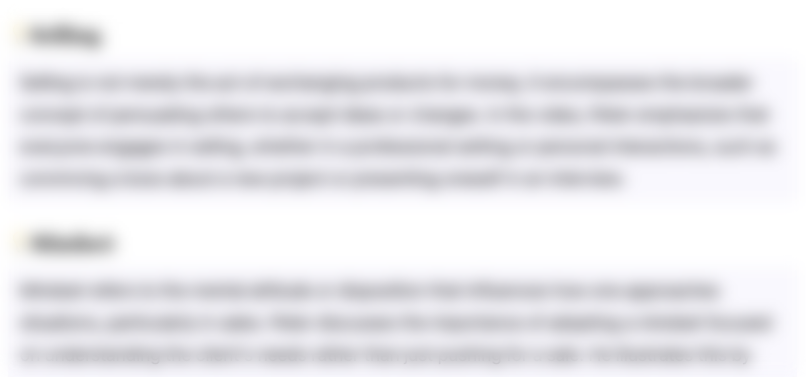
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
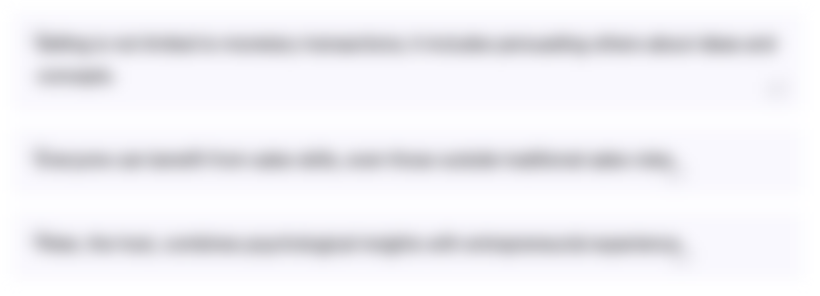
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
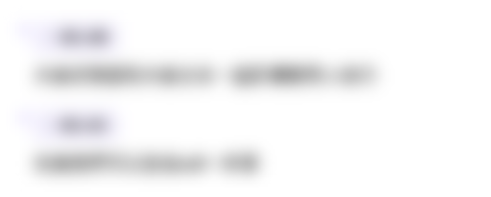
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
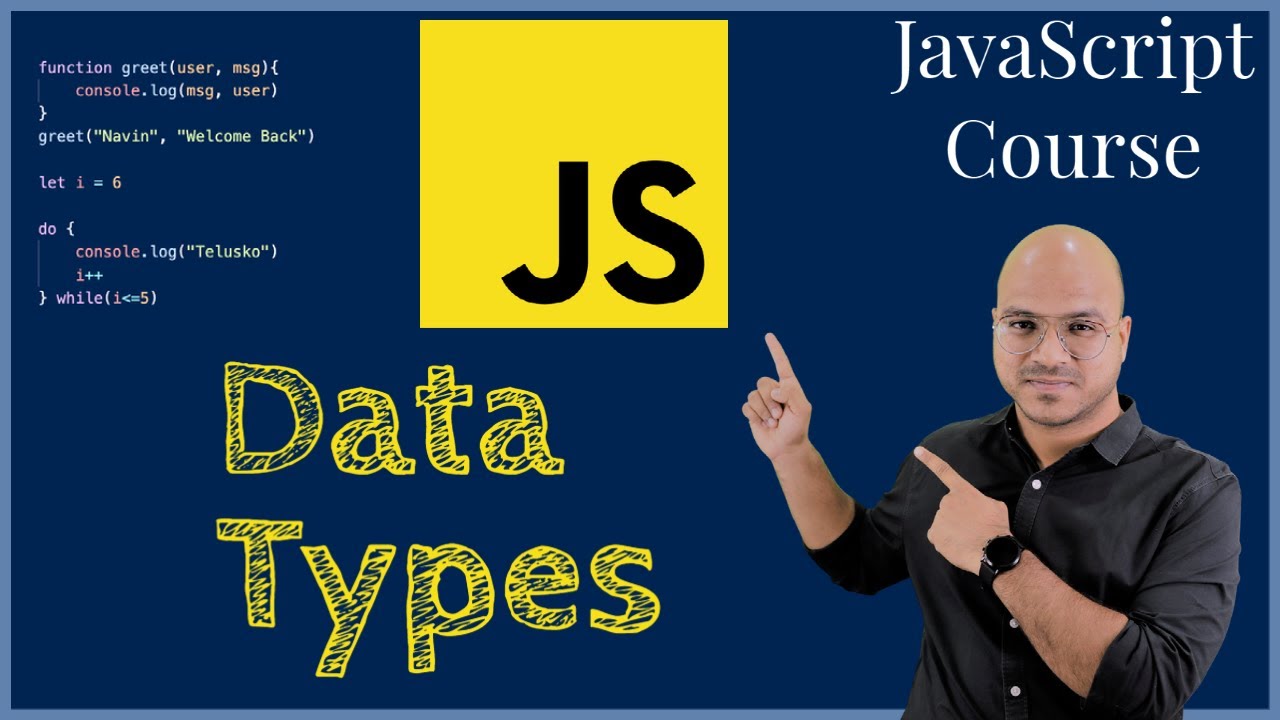
#5 Data Types in JavaScript - 1 | JavaScript Tutorial
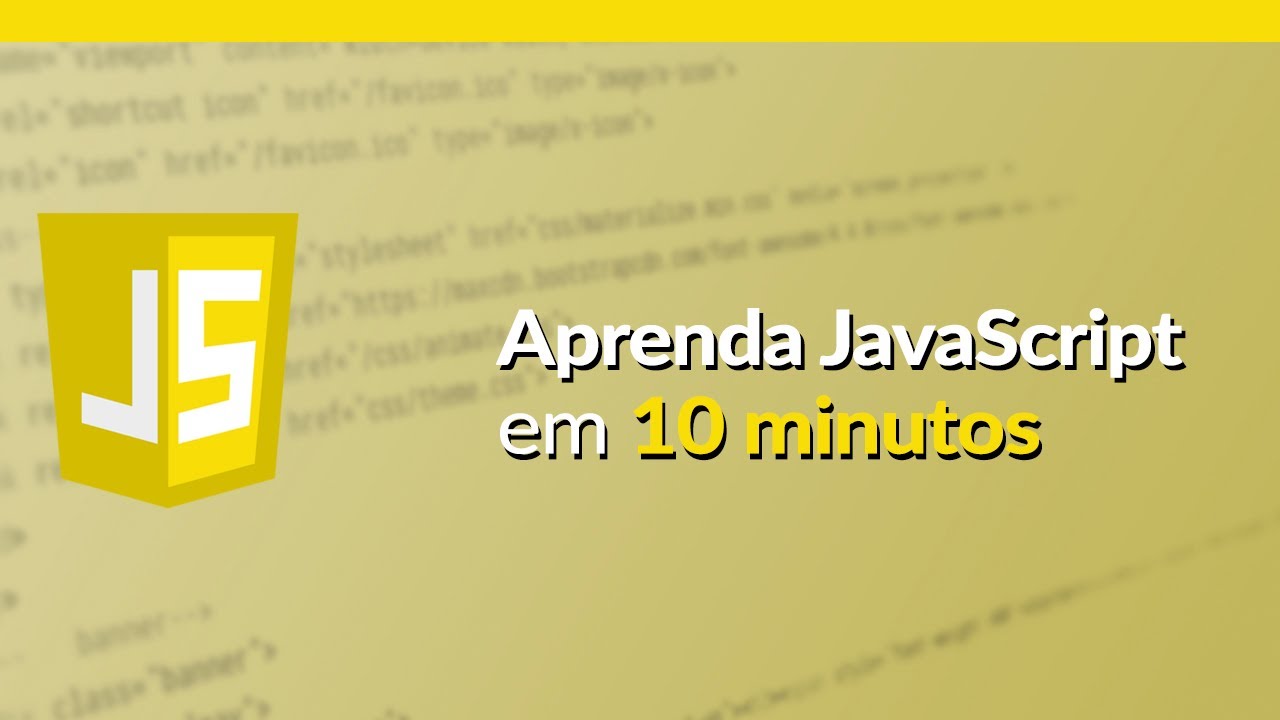
APRENDA JAVASCRIPT EM 10 MINUTOS
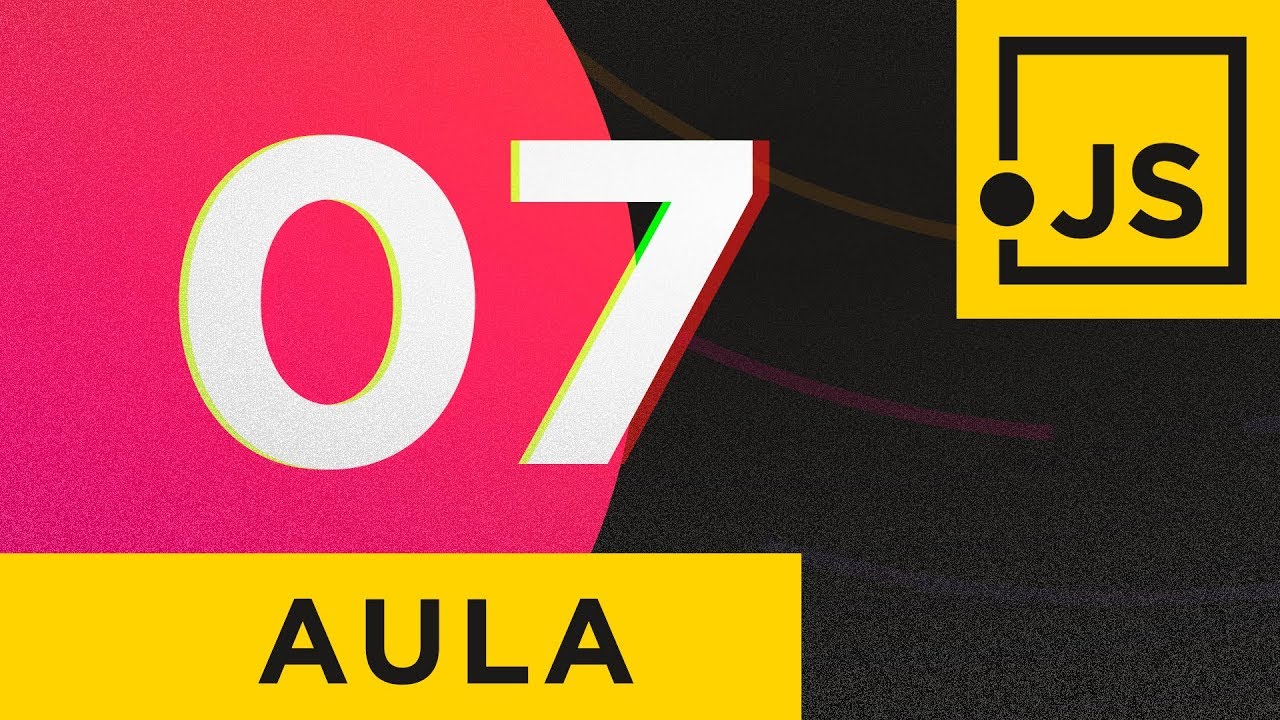
Operators (Part1) - JavaScript Course #07
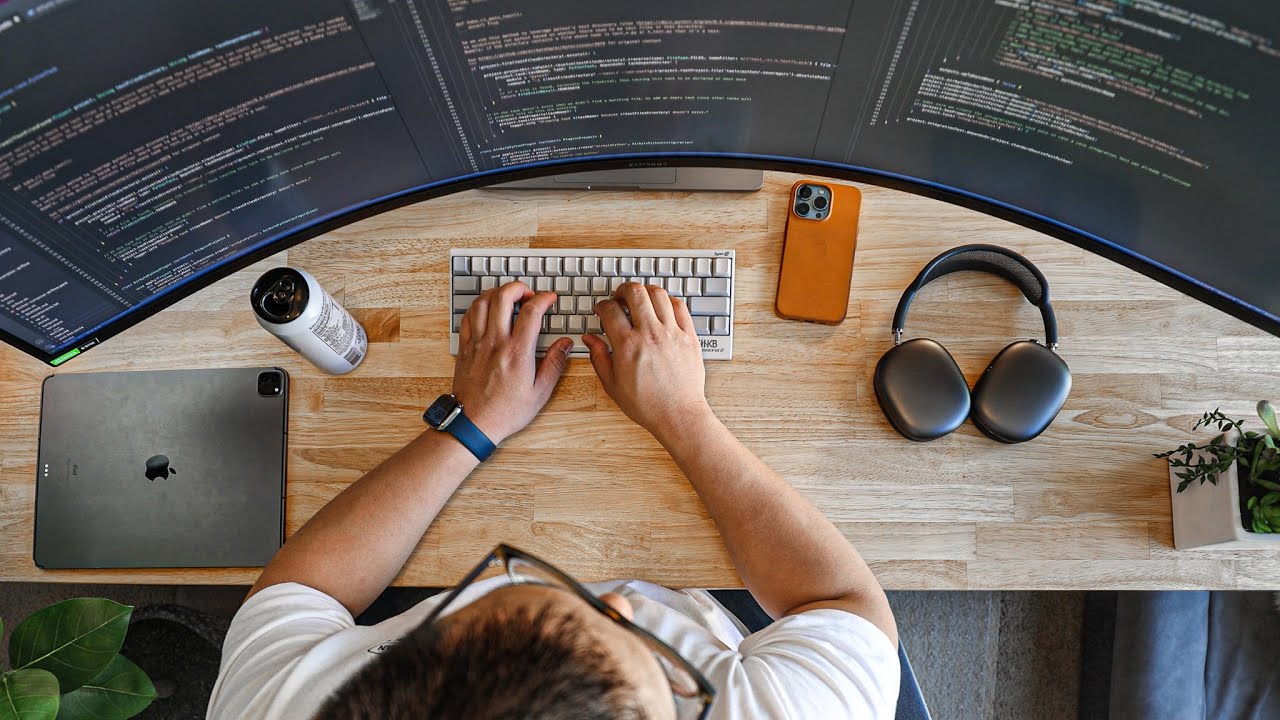
How Much HTML & CSS Do You Need To Know as a Web Developer

How JavaScript Code is executed? â€ïž& Call Stack | Namaste JavaScript Ep. 2

Javascript for beginners | chai aur #javascript
5.0 / 5 (0 votes)