x86 Assembly Crash Course
Summary
TLDRThis tutorial introduces binary exploitation by explaining how compiled executables work at a low level, focusing on x86 assembly. It covers the process of compilation, memory management (heap, stack, registers), and key assembly instructions (e.g., add, sub, push, pop). The video walks through how function calls manipulate the stack, how data is stored in memory, and the control flow of an executable. Designed for beginners, the content prepares users to understand the underlying mechanics of programs, which is essential for practical binary exploitation and security research.
Takeaways
- 😀 Binary exploitation involves manipulating compiled programs to make them behave in unintended ways, often by understanding how the executable works at a low level.
- 😀 A C program is compiled by a compiler into machine code, which is represented as a sequence of opcodes (operation codes) executed by the computer.
- 😀 Assembly language acts as a human-readable translation of machine code, allowing us to understand the operations a computer is executing.
- 😀 The four main components of an executable are the Heap, Stack, Registers, and Instructions. Each plays a critical role in the program’s execution.
- 😀 The Heap is used for dynamic memory allocation, and memory is allocated using functions like `malloc` and `calloc`.
- 😀 Registers are small storage areas in the CPU that hold values, memory addresses, and data that the processor is currently working with.
- 😀 The Stack is a data structure where function calls and local variables are stored. It grows toward lower memory addresses and operates using push and pop operations.
- 😀 Every function call sets up a stack frame to store local variables, function arguments, and the return address. This stack frame is managed by the EBP (Base Pointer) and ESP (Stack Pointer) registers.
- 😀 Common assembly instructions include `add` (addition), `sub` (subtraction), `push` (place a value on the stack), and `pop` (retrieve a value from the stack). These control the flow and data manipulation in the program.
- 😀 The Instruction Pointer (EIP) controls which instruction is being executed next. It moves automatically to the next instruction unless altered by jump or call instructions.
- 😀 Control flow instructions, such as `cmp` (comparison) and conditional jump instructions (e.g., `jmp`, `je`, `jne`), are essential for branching and loop control in programs.
Q & A
What is binary exploitation?
-Binary exploitation is the process of manipulating compiled executables to make them perform actions intended by the attacker, often by exploiting vulnerabilities in the program's execution.
What is a C program compiled into and how does it relate to binary exploitation?
-A C program is compiled into machine code, which consists of opcodes that the computer can execute. To exploit a binary, one must understand how the machine code is structured and how to manipulate it at a low level.
What role do compilers play in the creation of executables?
-Compilers take the source code written in C (or another language) and convert it into machine code, a sequence of operations that the computer can execute directly.
How does assembly language help in understanding compiled executables?
-Assembly language provides a human-readable representation of machine instructions, helping you understand how the computer will execute a program. It acts as a bridge between high-level programming languages and machine code.
What are the four main components of an executable in C?
-The four main components of a C executable are the heap, the stack, registers, and instructions. These elements play key roles in how the program stores data and executes instructions.
What is the heap, and how is it used in a C program?
-The heap is a memory area used for dynamic memory allocation. Functions like `malloc()` and `calloc()` allocate memory on the heap, and global or static variables are also stored there.
What is the function of general-purpose registers in x86 architecture?
-General-purpose registers (eax, ebx, ecx, edx, esi, edi) in x86 architecture store temporary data such as values and memory addresses that the CPU uses during computation.
How does the stack work, and how does it grow in memory?
-The stack is a data structure that stores function call information, local variables, and return addresses. It grows downward in memory, with higher memory addresses at the bottom and lower addresses at the top.
What is a stack frame, and how is it set up when a function is called?
-A stack frame is a section of the stack that contains information for a function call. When a function is called, the return address, the base pointer, and the function's local variables are pushed onto the stack, creating the stack frame.
How does the EBP and ESP register interaction work in managing stack frames?
-The EBP (Base Pointer) register points to the base of the current stack frame, while the ESP (Stack Pointer) points to the top of the stack. The ESP is adjusted to make space for local variables, while the EBP helps maintain the integrity of the stack frame during function calls.
What is the role of the 'compare' instruction in assembly language?
-The 'compare' instruction is used to subtract two values without storing the result, but it sets the processor's flags based on the result. These flags can then be used to control the flow of the program, such as determining whether a jump or conditional operation should occur.
What is the function of the 'call' and 'return' instructions in a program?
-The 'call' instruction pushes the return address onto the stack and transfers control to a function. The 'return' instruction pops the return address off the stack and transfers control back to the caller.
What happens during the 'leave' instruction in a function?
-The 'leave' instruction restores the stack pointer to the base pointer, effectively dismantling the current stack frame. This prepares the program to return from the function call.
Why is understanding assembly necessary for binary exploitation?
-Understanding assembly is crucial for binary exploitation because it allows attackers to read and manipulate the machine-level instructions that the CPU executes, helping them find vulnerabilities or unintended behavior in the program.
What are the differences between AT&T and Intel assembly syntax?
-The main difference between AT&T and Intel assembly syntax lies in the order of operands and the way registers are represented. In Intel syntax, the destination operand comes first (e.g., `add eax, 5`), whereas in AT&T syntax, the source comes first (e.g., `add $5, %eax`).
How do jump instructions work in assembly, and how do they relate to flags?
-Jump instructions in assembly move the instruction pointer (EIP) to a new address, depending on the condition set by the flags. For example, a 'jump if less than' instruction will only jump if the comparison result was less than zero.
Outlines
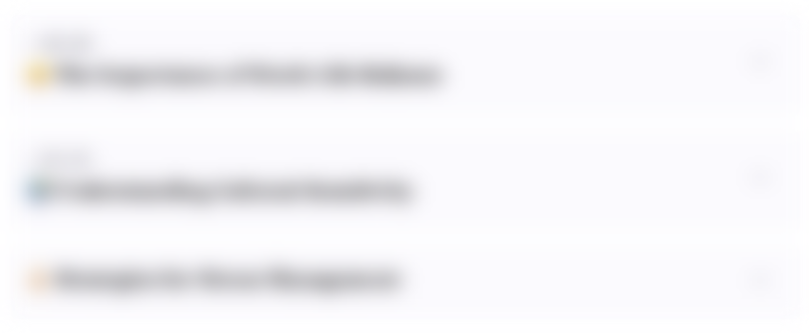
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
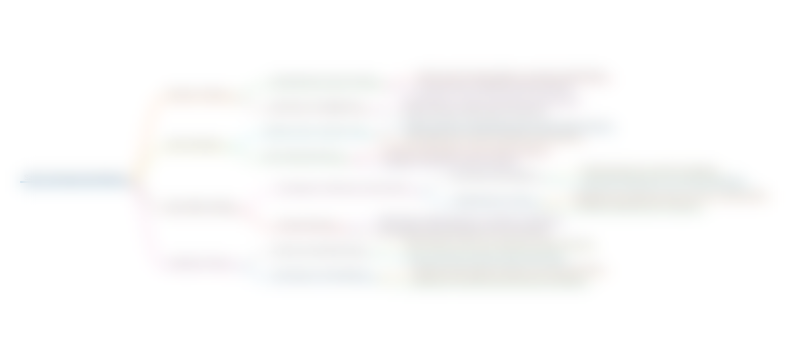
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
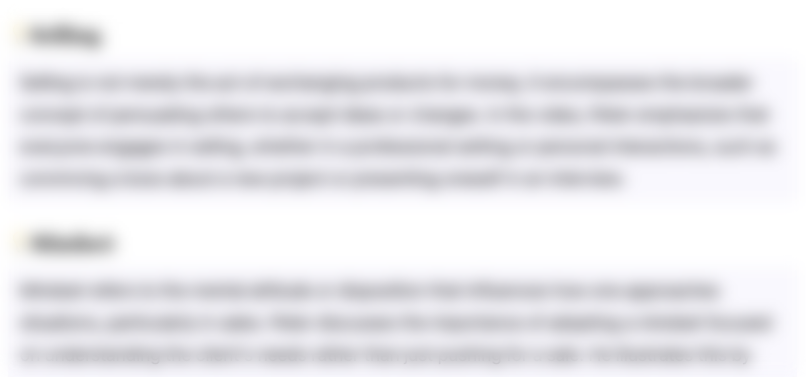
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
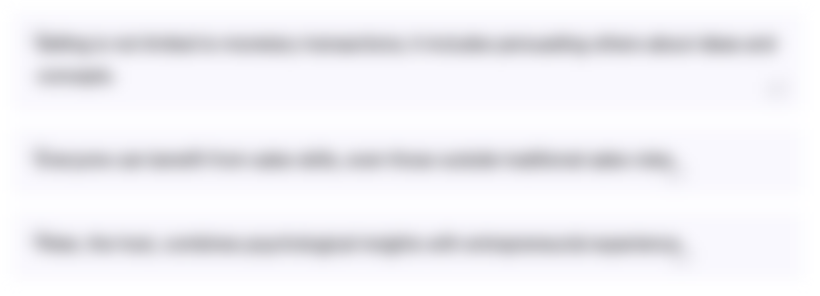
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
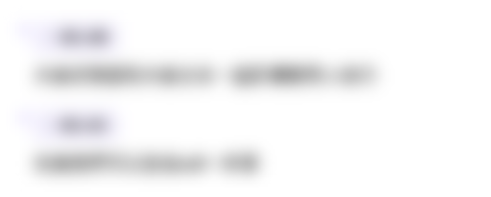
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
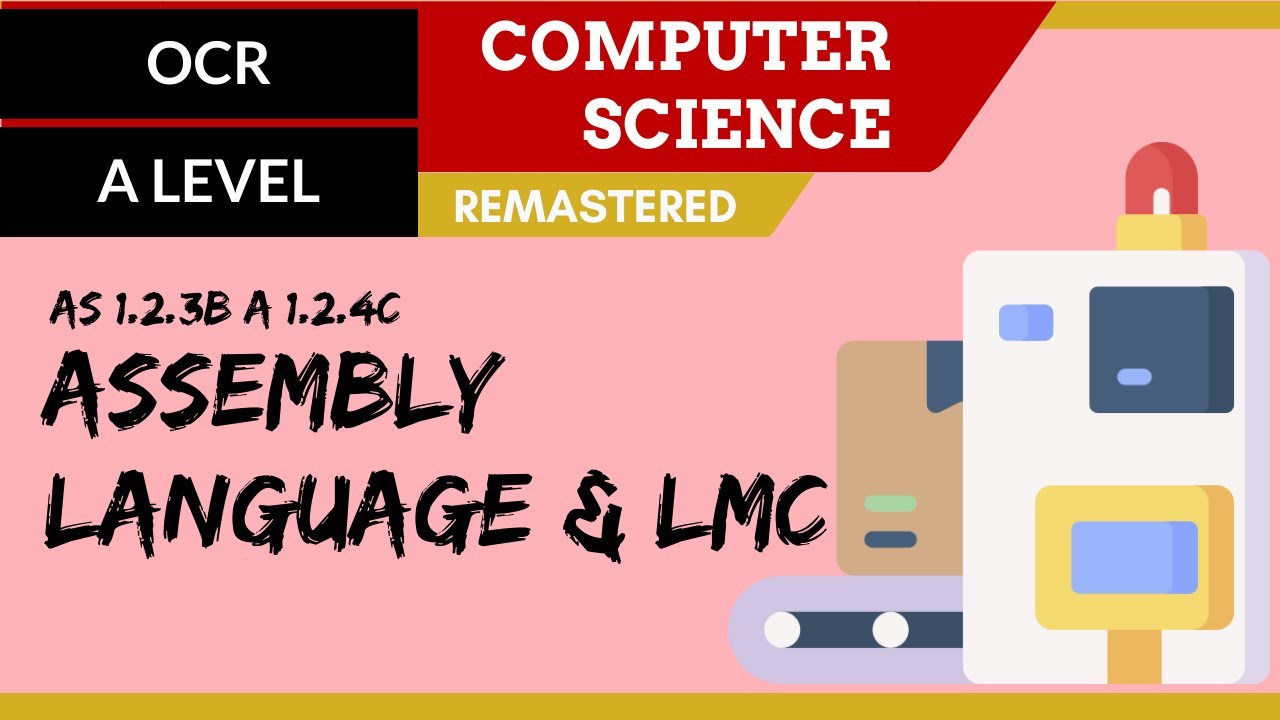
34. OCR A Level (H046-H446) SLR7 - 1.2 Assembly language and LMC language
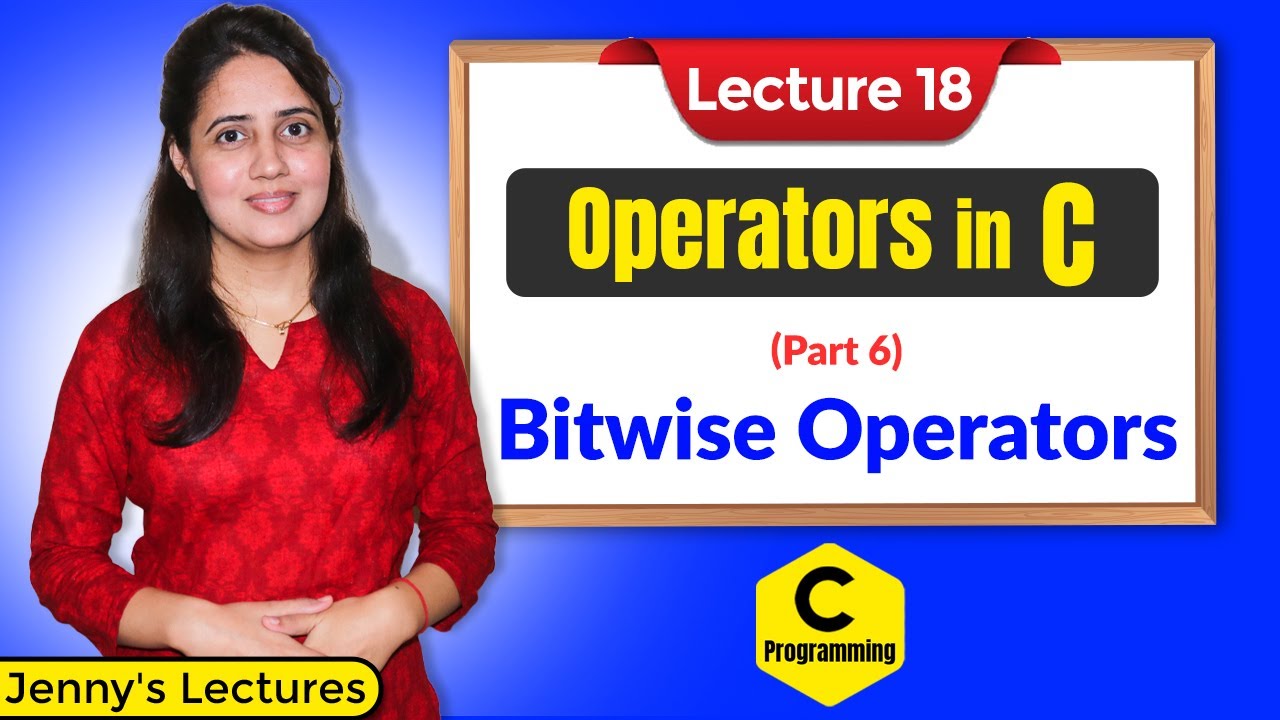
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials

x64 Assembly Tutorial 19: FLAGS Register, Conditional Jumps and Moves
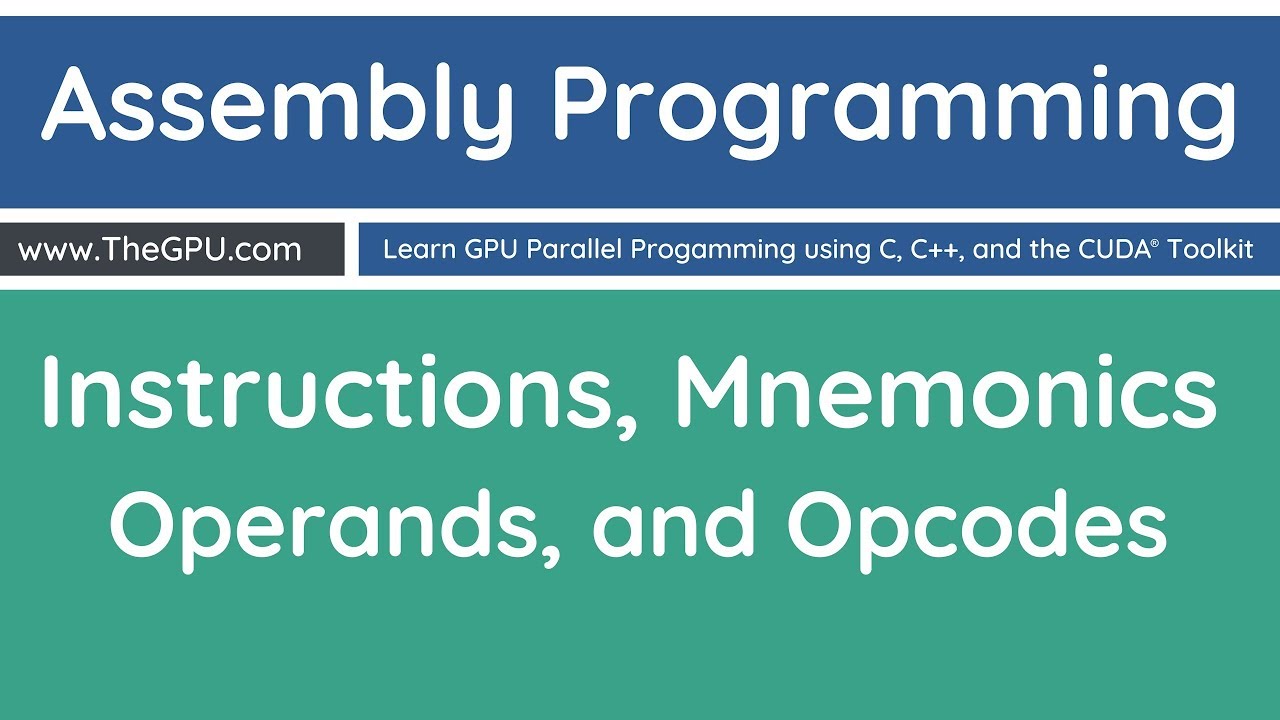
Learn Assembly Programming - Instructions, Mnemonics, Operands, and Opcodes
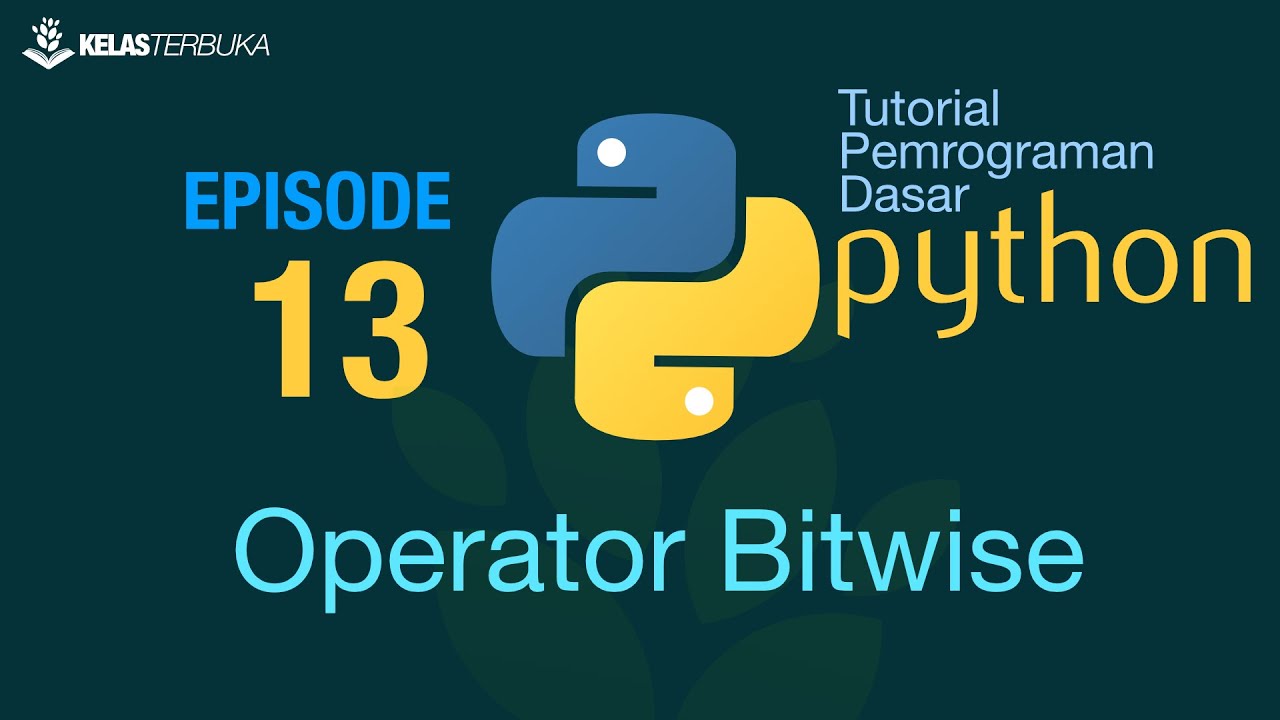
Belajar Python [Dasar] - 13 - Operator Bitwise
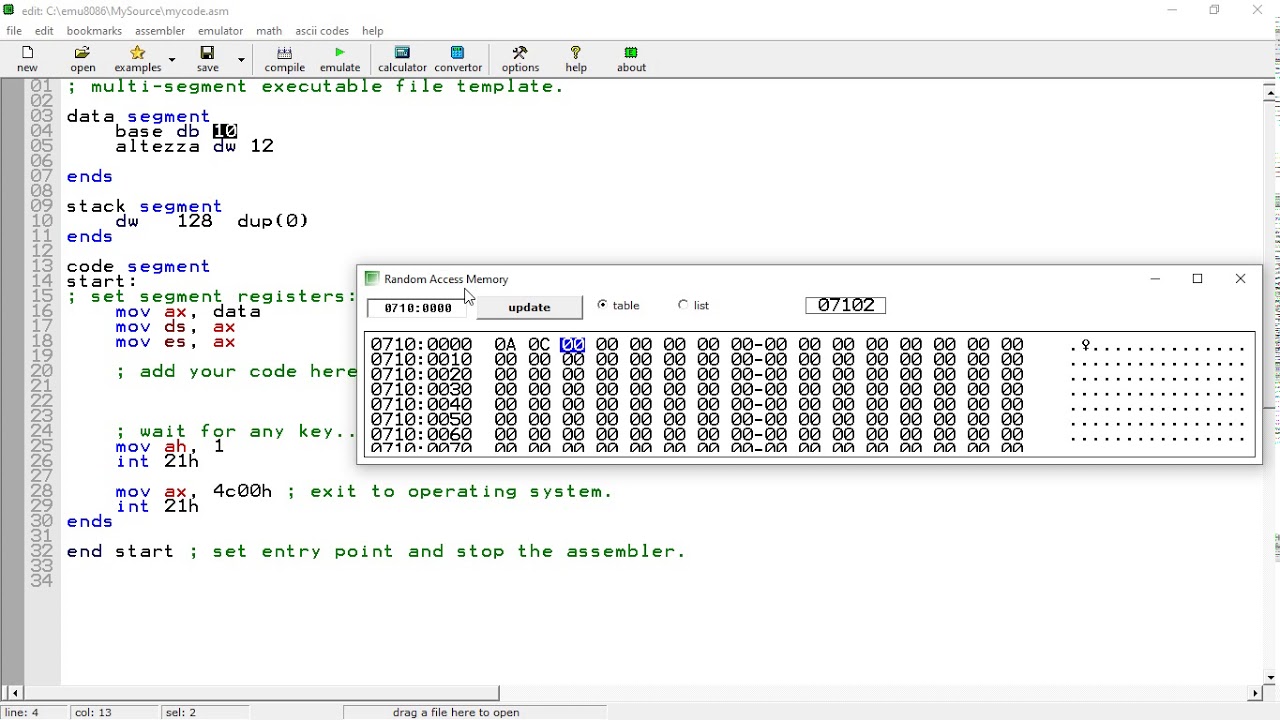
Il DataSegment 8086 Emulatore
5.0 / 5 (0 votes)