Belajar Python [Dasar] - 13 - Operator Bitwise
Summary
TLDRThis tutorial provides an in-depth introduction to bitwise operators in Python. The instructor explains the concept of bitwise operations using binary representations, demonstrating how operators like OR, AND, XOR, and NOT work. Practical examples are given to show how these operations manipulate data at the bit level, including the use of bit shift operators (left and right shifts). Additionally, the tutorial covers the significance of signed integers and two's complement in Python. Ideal for learners interested in understanding low-level programming concepts and optimizing performance with bitwise operations.
Takeaways
- 😀 Bitwise operators are binary operations that work on individual bits of data, such as integers, rather than entire values.
- 😀 The bitwise operators include OR, AND, XOR, and NOT, and each performs a specific operation between corresponding bits of the operands.
- 😀 The OR operator ('|') compares two binary numbers and results in a 1 if either of the corresponding bits is 1, otherwise 0.
- 😀 The AND operator ('&') compares two binary numbers and results in a 1 only if both corresponding bits are 1.
- 😀 The XOR operator ('^') compares two binary numbers and results in a 1 if the corresponding bits are different, otherwise 0.
- 😀 The NOT operator ('~') inverts the bits of a number, changing 0s to 1s and vice versa.
- 😀 Bitwise operations can be performed in Python with operators such as |, &, ^, and ~ for OR, AND, XOR, and NOT respectively.
- 😀 A practical example of using the OR operator is shown with two integers: 9 (1001 in binary) and 5 (0101 in binary), producing 13 (1101 in binary).
- 😀 The AND operation between 9 (1001 in binary) and 5 (0101 in binary) results in 1 (0001 in binary).
- 😀 Shifting operators such as left shift ('<<') and right shift ('>>') can be used to shift bits in numbers, which effectively multiplies or divides the number by powers of 2.
- 😀 Shifting left by 1 bit multiplies the number by 2, while shifting right by 1 bit divides the number by 2, using the shift operators in Python.
Q & A
What is the purpose of bitwise operators, as explained in the script?
-The purpose of bitwise operators is to perform operations on individual bits of binary numbers. These operations include AND, OR, XOR, and NOT, which manipulate data at the bit level.
What does the term 'bitwise' refer to in the context of this tutorial?
-In the context of the tutorial, 'bitwise' refers to operations performed on individual bits of data. Each bit is treated separately in operations, allowing for low-level data manipulation.
How is an integer represented in binary as described in the script?
-An integer is represented in binary as a sequence of bits (0s and 1s). For example, the number 2 is represented as '00000010' in an 8-bit binary form, where each position corresponds to a power of 2.
Can you explain the bitwise OR operation and how it works?
-The bitwise OR operation compares corresponding bits of two numbers and returns 1 if either bit is 1. For example, 2 (00000010) OR 1 (00000001) results in 3 (00000011).
What does the bitwise AND operation do?
-The bitwise AND operation compares corresponding bits of two numbers and returns 1 only if both bits are 1. For example, 9 (00001001) AND 5 (00000101) results in 1 (00000001).
How does the bitwise XOR (exclusive OR) work?
-The bitwise XOR operation compares corresponding bits and returns 1 only if the bits are different. For example, 9 (00001001) XOR 5 (00000101) results in 12 (00001100).
What is the purpose of the bitwise NOT operation, and how does it behave with negative numbers?
-The bitwise NOT operation inverts all bits of a number. When applied to a number like 9 (00001001), it flips the bits and results in a negative number. This is because the representation of negative numbers uses a sign bit in binary.
What happens when a number is shifted using the shift right operator?
-Shifting a number to the right moves its bits towards the right, effectively dividing the number by 2 for each shift. For example, shifting '1010' (binary for 10) right by one position results in '0101' (binary for 5).
How is the shift left operator different from the shift right operator?
-The shift left operator moves bits to the left, effectively multiplying the number by 2 for each shift. For example, shifting '1010' (binary for 10) left by one position results in '10100' (binary for 20).
Why is understanding bitwise operators important, as mentioned in the script?
-Understanding bitwise operators is important because they allow for efficient low-level data manipulation, which can be critical in certain programming tasks, such as performance optimization, cryptography, and hardware control.
Outlines
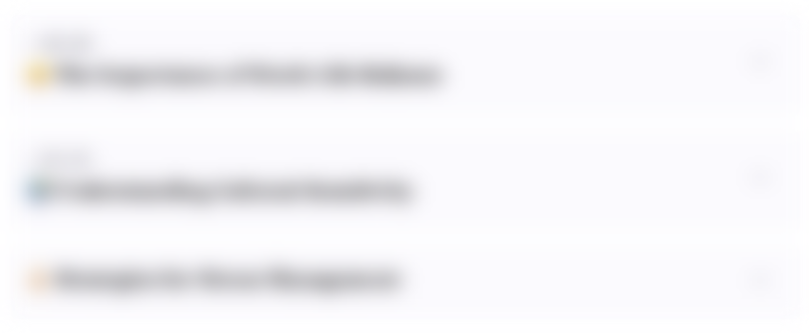
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
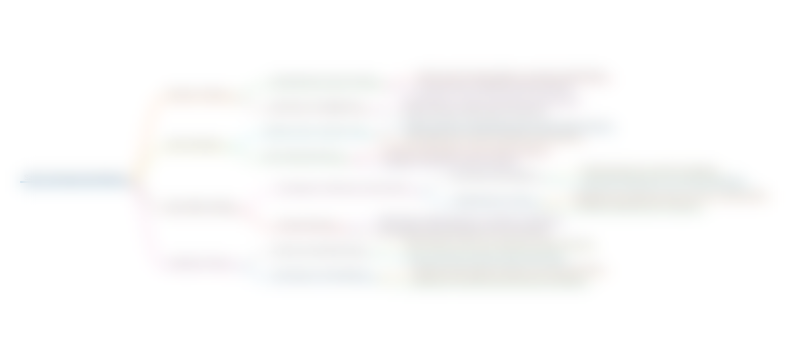
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
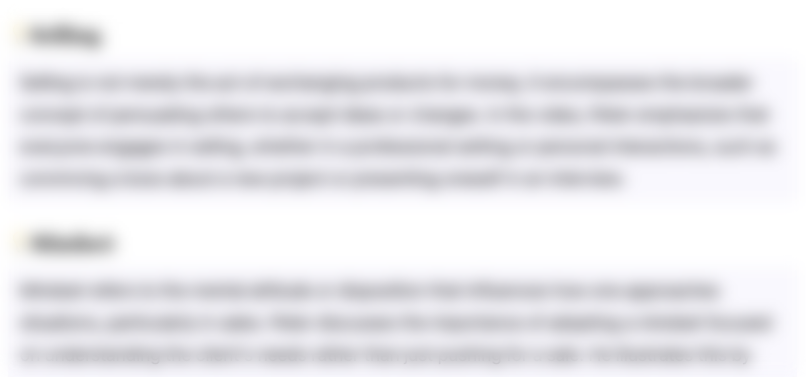
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
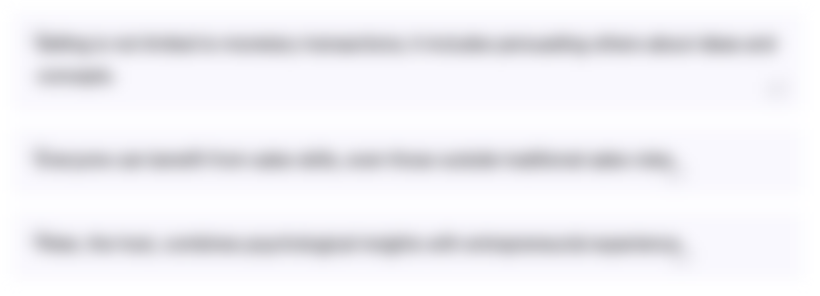
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
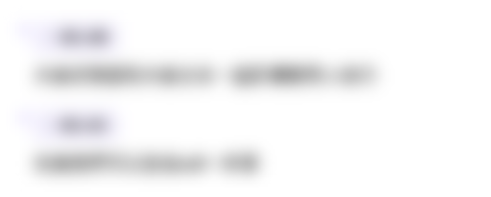
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Belajar Python [Dasar] - 14 - Operator Assignment
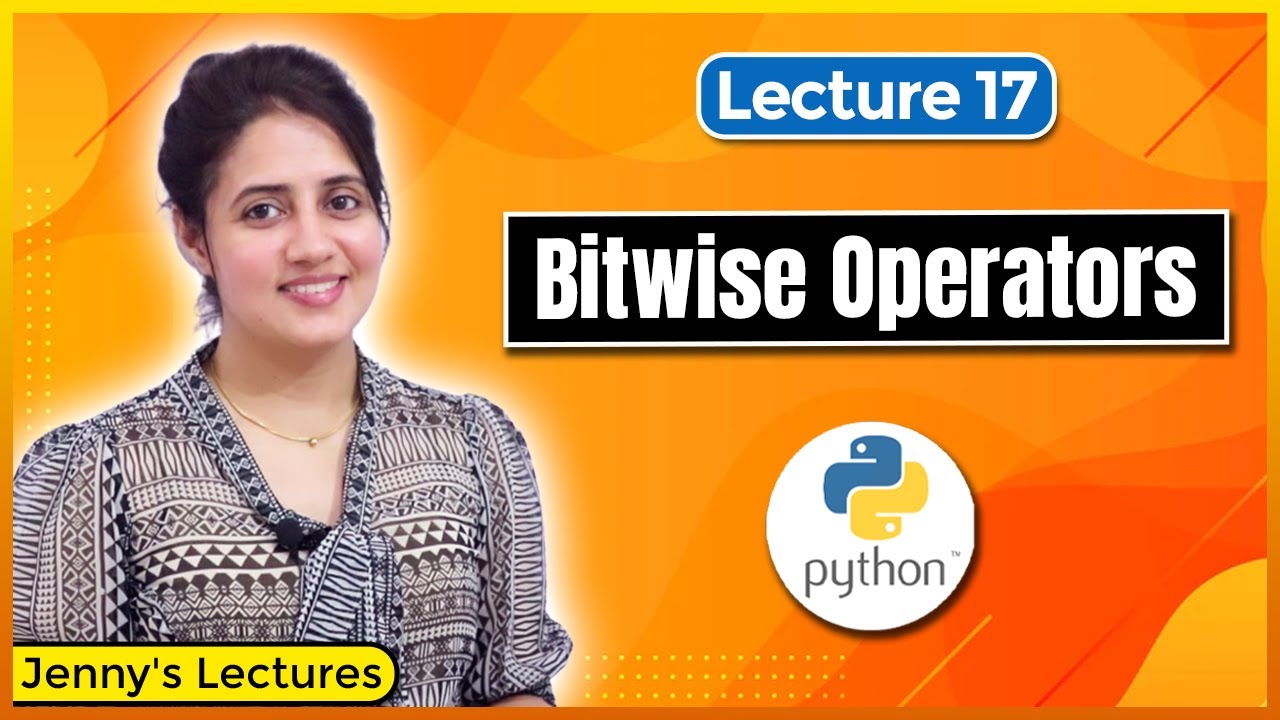
Operators in Python | Bitwise Operators | Python Tutorials for Beginners #lec17
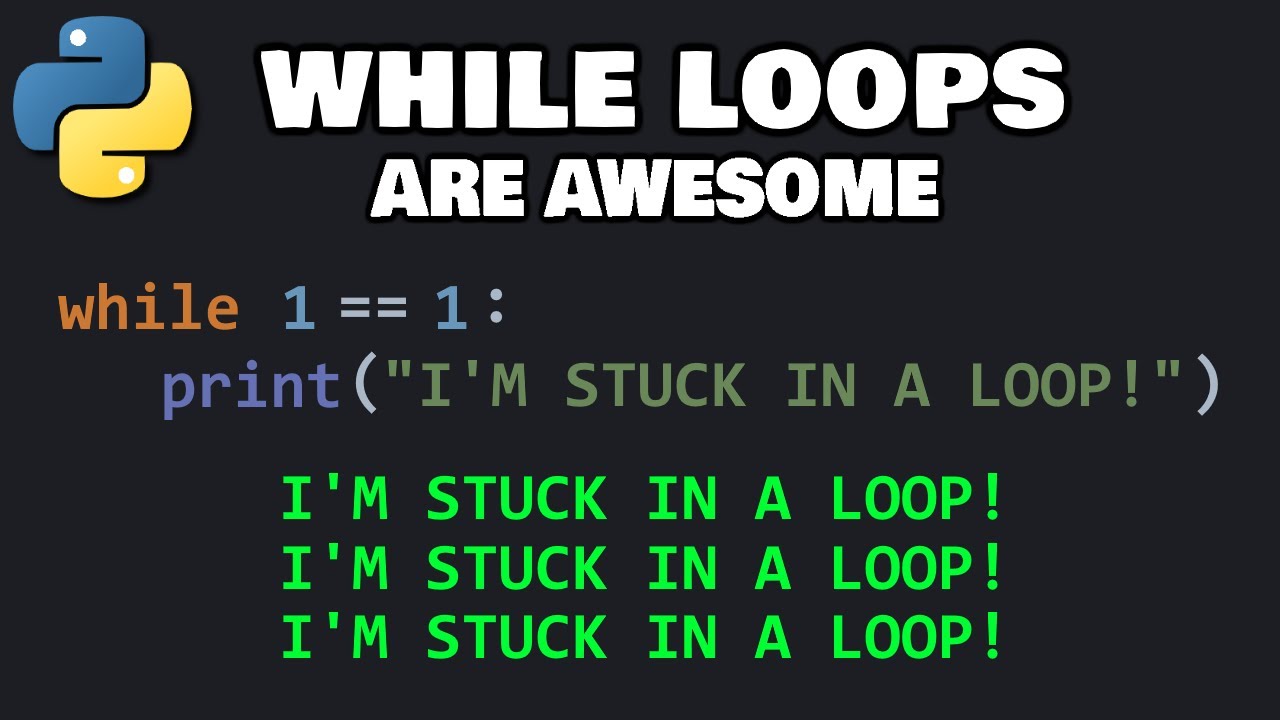
While loops in Python are easy ♾️
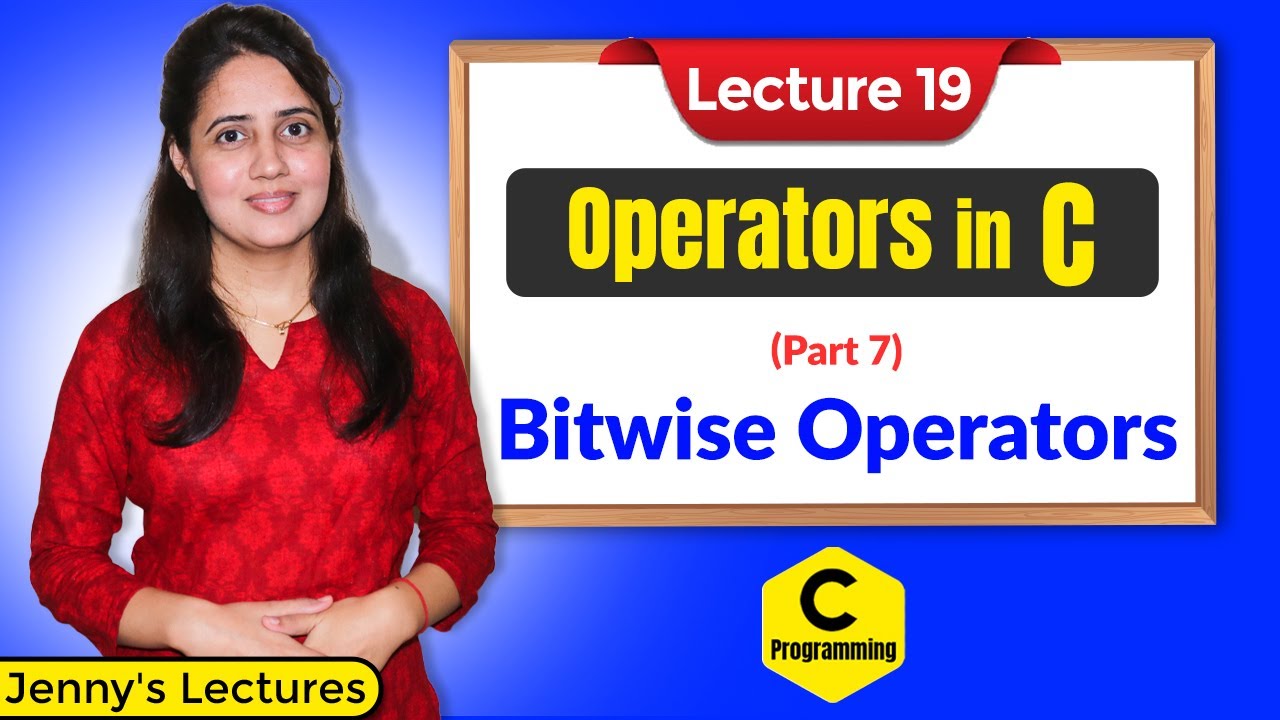
C_19 Operators in C - Part 7 (Bitwise Operators-II) | C Programming Tutorials
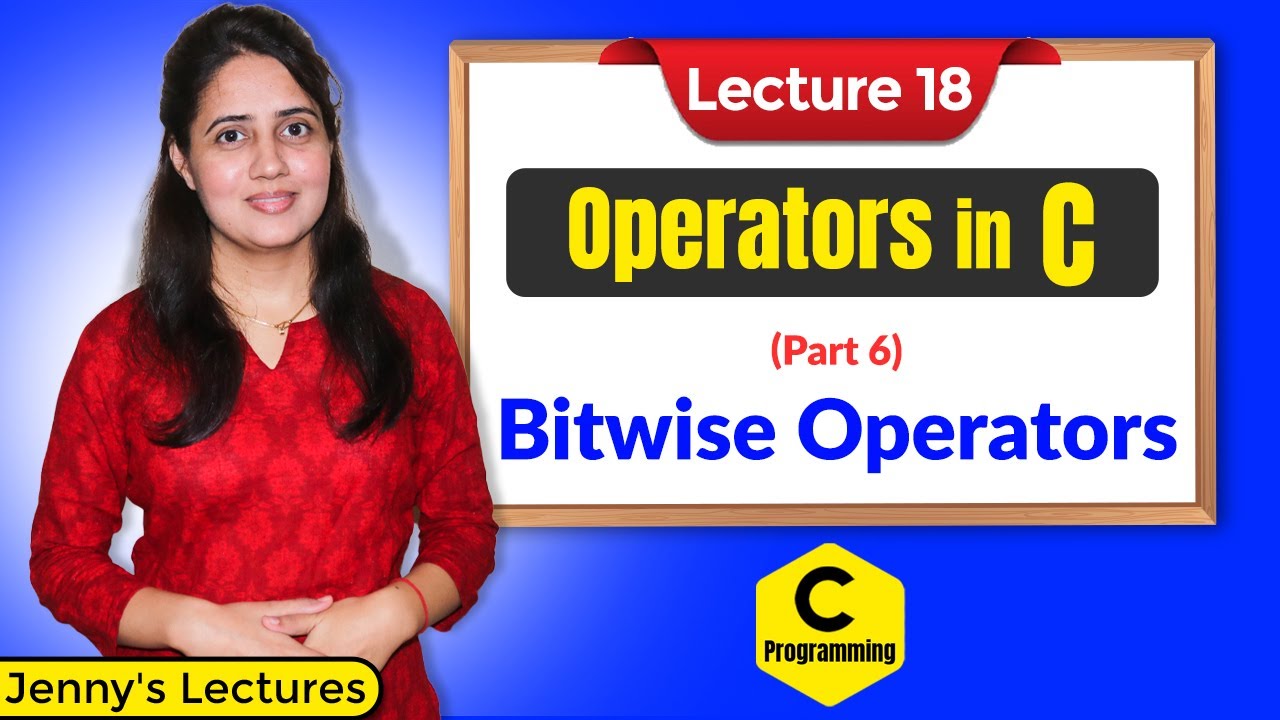
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials
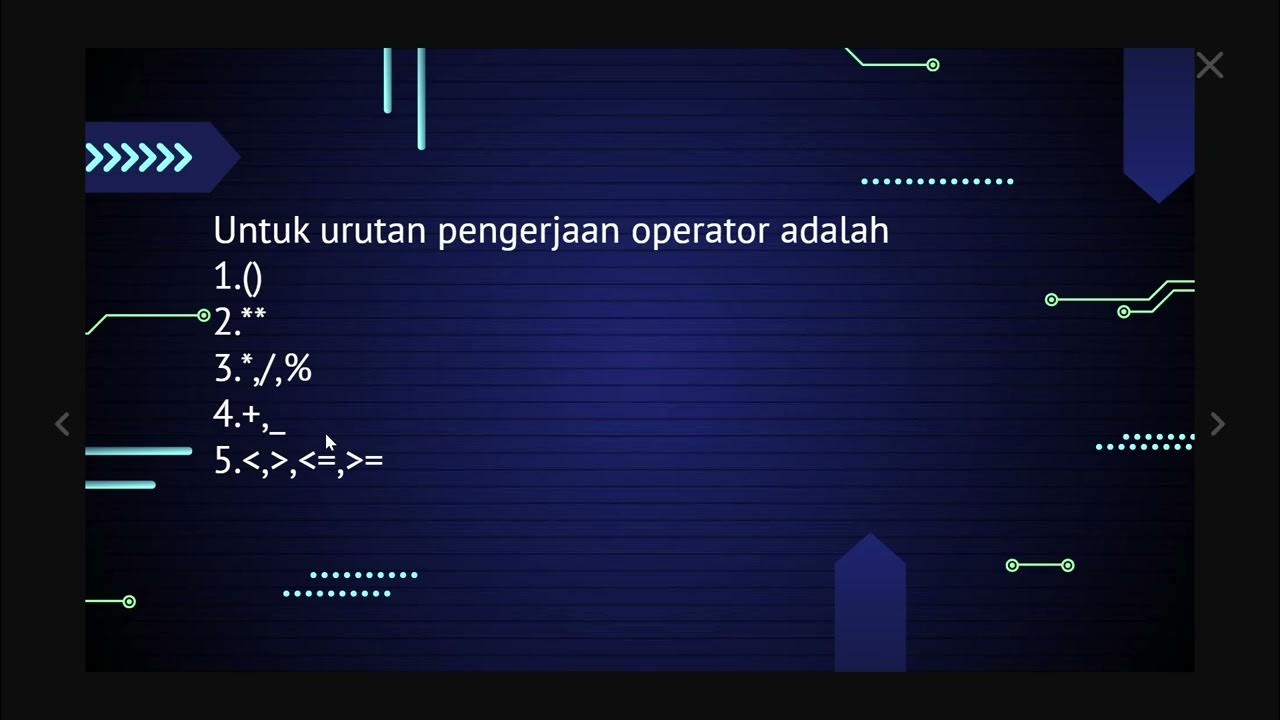
ALGORITMA dan PEMROGRAMAN || OPERATOR
5.0 / 5 (0 votes)