JavaScript Classes #3: Static Methods - JavaScript OOP Tutorial
Summary
TLDRIn this video, DOM explains static methods in JavaScript classes. He demonstrates how static methods are defined on a class but don't require an instance to be used. Static methods are often used for helper functions that operate independently of class instances. Through examples with a `Square` class, DOM shows how static methods can be used for comparisons and validations, such as checking if two squares have equal areas or if the dimensions form a valid square. The video provides a clear understanding of the purpose and usage of static methods in JavaScript classes.
Takeaways
- 😀 Static methods are defined on a class but are not part of the instantiated object.
- 😀 Static methods do not require an instance of the class to be used, making them useful for utility functions.
- 😀 They are often referred to as 'helper' methods because they provide functionality related to the class but don’t interact with instance-specific data.
- 😀 Static methods are called directly on the class, not on an instance of the class.
- 😀 In the example, the `Square` class has a constructor that assigns both width and height to make a square.
- 😀 The `equals` static method checks whether two `Square` instances have the same area by comparing their width and height.
- 😀 Static methods can be used for comparing objects, such as checking if two squares have the same area, without needing to instantiate them.
- 😀 The `isValidDimensions` static method validates whether a given width and height form a valid square by ensuring they are equal.
- 😀 Static methods don’t require access to `this` since they do not interact with instance-specific properties.
- 😀 You can call static methods even if no instances of the class are created, as they are bound to the class itself.
- 😀 Static methods are useful for validation, comparison, and other operations that don't require individual object state.
Q & A
What is a static method in JavaScript?
-A static method is a function defined in a class but not bound to any specific instance of the class. It can be called directly on the class itself, without needing an instance of the class.
How do you define a static method in a JavaScript class?
-A static method is defined using the `static` keyword followed by the method name. For example: `static equals(a, b) { ... }`.
What is the main difference between a static method and an instance method in JavaScript?
-A static method is called on the class itself, not on an instance of the class. An instance method, on the other hand, is called on an instance and typically uses the `this` keyword to access instance properties.
What is an example use case for a static method?
-Static methods are often used for utility or helper functions that are related to the class but do not require an instance of the class. For example, a static method might compare two square instances to see if their areas are equal.
How do you call a static method in JavaScript?
-A static method is called directly on the class itself, using the class name followed by the method name. For example: `Square.equals(square1, square2)`.
Can static methods access instance properties like `this`?
-No, static methods cannot access instance properties or methods because they are not bound to any specific instance of the class. They can only access other static properties or methods of the class.
What happens if you try to call a static method on an instance?
-Static methods cannot be called on an instance directly. If you try to call a static method on an instance, it will result in an error.
Why would you use a static method instead of an instance method?
-You would use a static method when you need to perform operations that are related to the class as a whole and do not require access to instance-specific data. Static methods are useful for utility functions or validation checks.
In the provided example, what does the `equals` static method do?
-The `equals` static method compares two instances of the `Square` class by checking if their areas (width * height) are equal, and returns `true` or `false` based on the comparison.
What is the purpose of the `isValidDimensions` static method in the example?
-The `isValidDimensions` static method checks whether the dimensions of a square (width and height) are equal, returning `true` if they are and `false` if they are not. It helps validate whether a square has equal sides.
Outlines
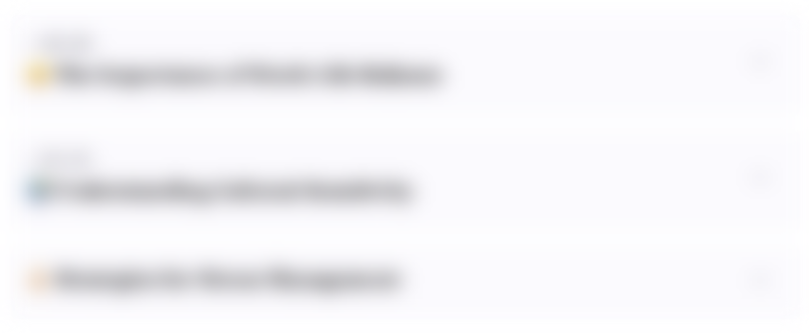
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
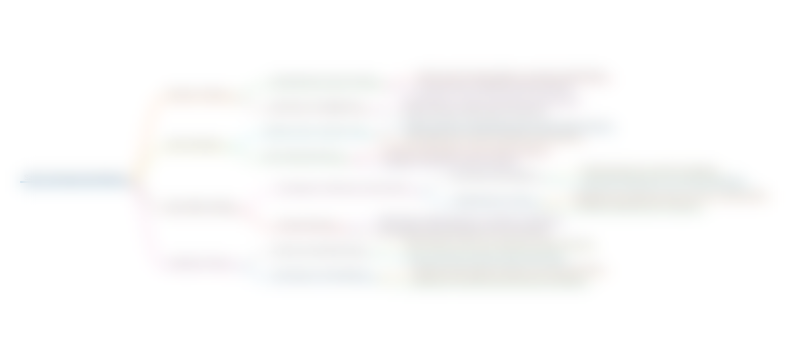
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
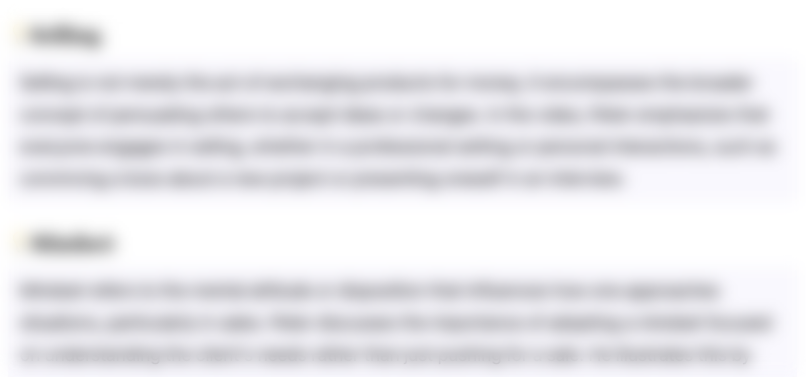
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
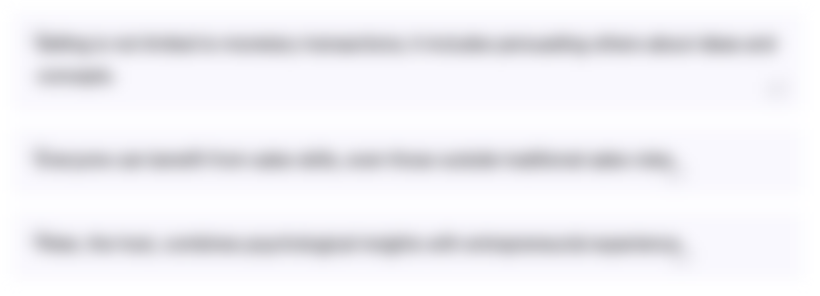
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
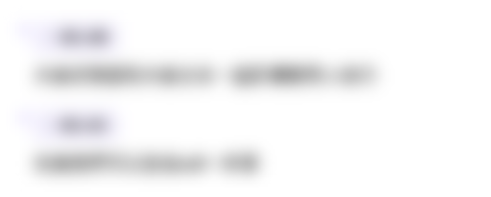
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
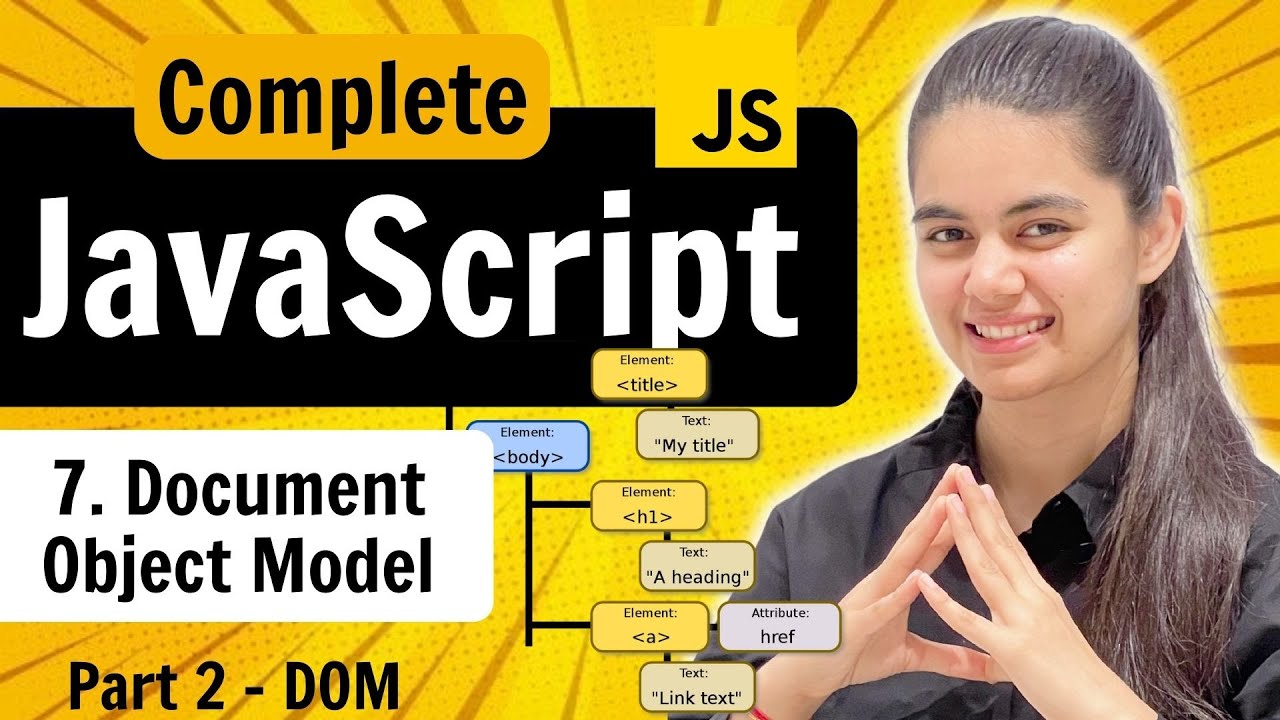
Lecture 7 : DOM (Part 2) | Document Object Model | JavaScript Full Course
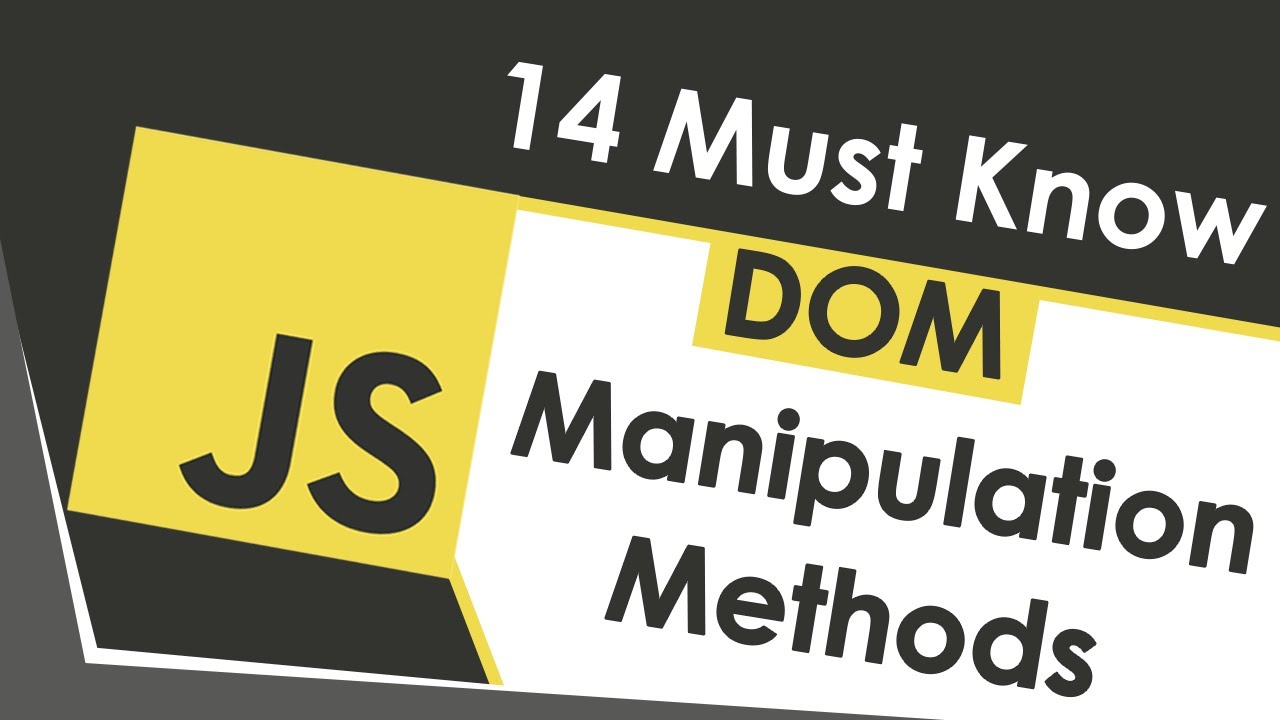
Learn DOM Manipulation In 18 Minutes
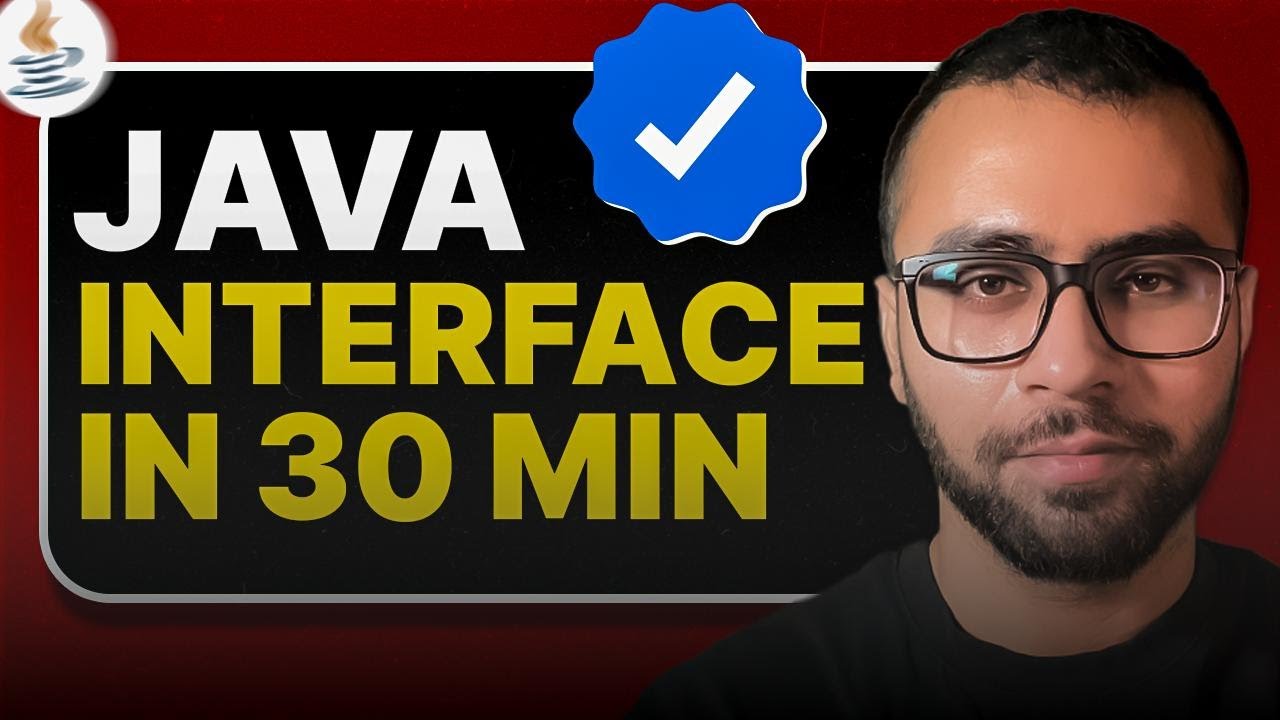
Mastering Java Interfaces: Static & Default Methods, Multiple Inheritance Explained
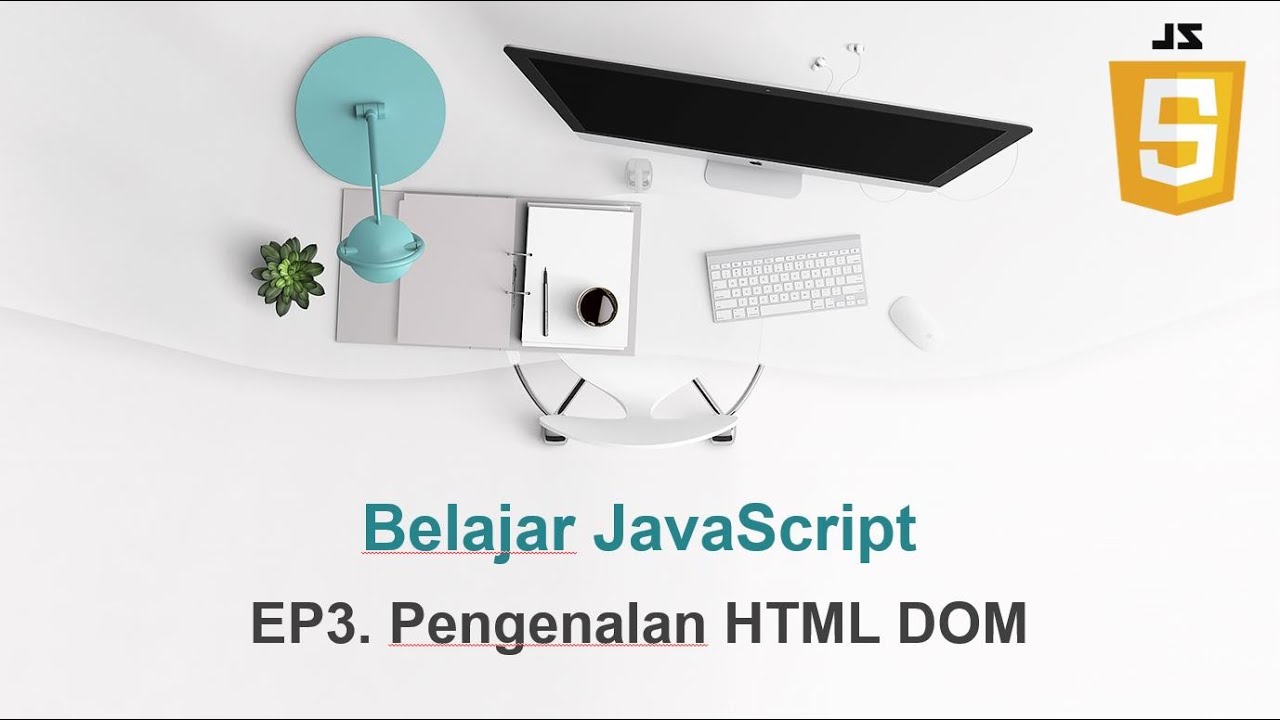
#3 Mengubah HTML elemen menggunakan DOM
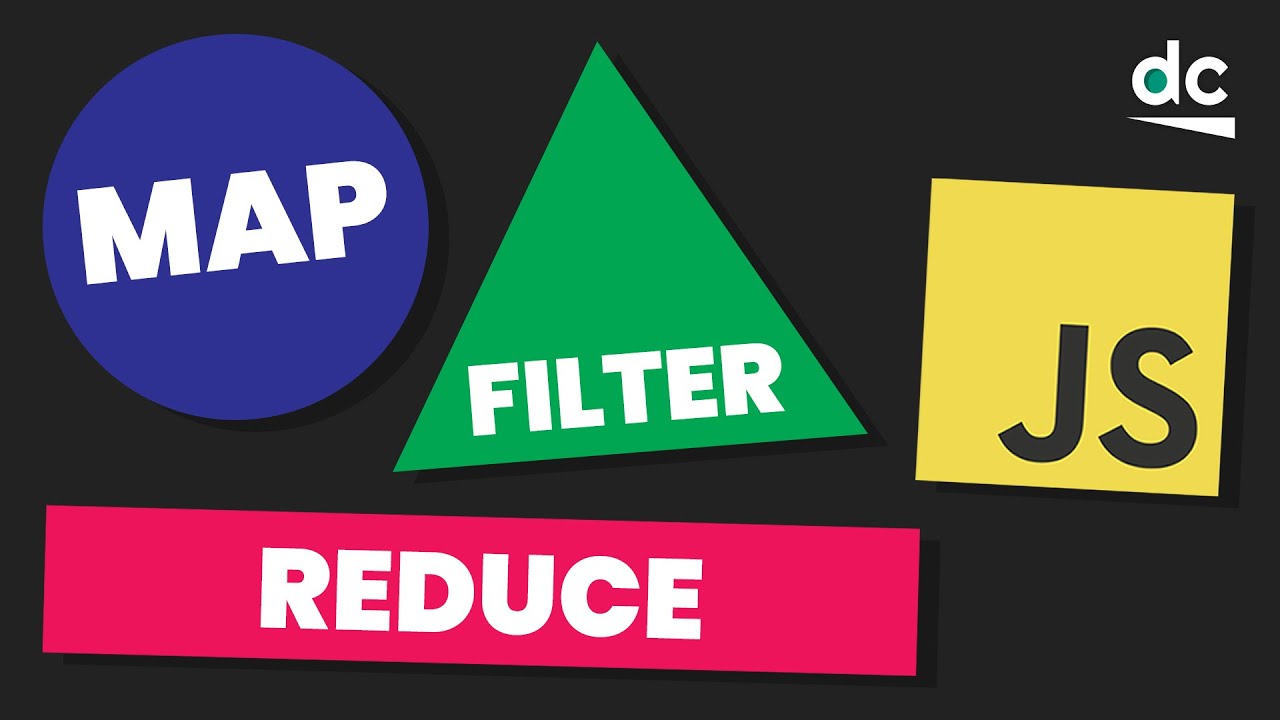
Map, Filter & Reduce EXPLAINED in JavaScript - It's EASY!
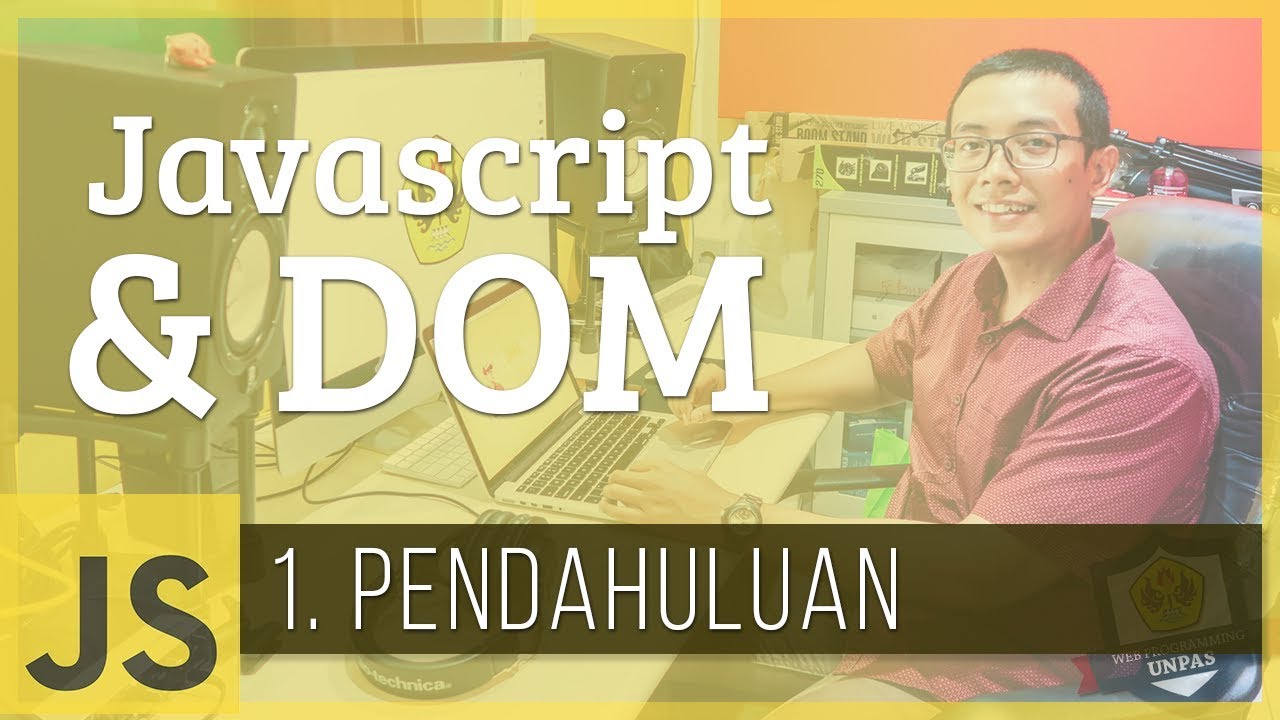
Javascript & DOM #1 - Pendahuluan
5.0 / 5 (0 votes)