How to Implement Biometric Auth in Your Android App
Summary
TLDRIn this video, the presenter demonstrates how to implement biometric authentication in an Android app using the Android biometric library. The tutorial covers integrating fingerprint, face ID, and device patterns for user authentication. The presenter walks through creating a custom class to manage the biometric prompt, handling authentication results, and setting up the UI to display authentication success, failure, or other error states. The tutorial also includes tips for handling different Android API levels and offering users a way to set up their biometric authentication if it's not yet configured.
Takeaways
- 😀 The tutorial demonstrates how to implement biometric authentication in Android using fingerprint, face ID, pattern, or PIN.
- 😀 To get started, you need to add the Android Biometric Library to your project dependencies in the `build.gradle` file.
- 😀 A custom `BiometricPromptManager` class is created to handle showing biometric prompts, checking authentication availability, and observing results.
- 😀 The `BiometricPromptManager` class requires an `AppCompatActivity` context since biometric prompts use fragments that are only supported in AppCompat activities.
- 😀 Biometric authentication can include multiple methods (fingerprint, face ID, device pattern, PIN) depending on the device and Android API level.
- 😀 The prompt UI can be customized using the `BiometricPrompt.PromptInfo.Builder` to set title, description, and other parameters like the confirmation requirement.
- 😀 To handle errors or missing biometric hardware, a sealed class (`BiometricResult`) is used to categorize outcomes like success, failure, or unavailable features.
- 😀 Authentication results are communicated to the UI using a `Channel` to handle multiple result emissions over time.
- 😀 The app checks whether biometric authentication is available on the device using `BiometricManager`, and provides feedback for different scenarios (e.g., hardware unavailable, feature not set up).
- 😀 If biometric authentication is not set up on the device, the user is directed to the settings to configure it via an intent with the action `Settings.ACTION_BIOMETRIC_ENROLL`.
- 😀 The implementation supports Android API 30+ features, like combining biometric authentication with device credentials (PIN, pattern) for enhanced security.
Q & A
What is the main focus of the tutorial in the video?
-The main focus of the tutorial is to teach how to implement biometric authentication in an Android app, using methods like fingerprint, face ID, security pattern, or PIN code.
What is the role of the BiometricPromptManager class in the tutorial?
-The BiometricPromptManager class is responsible for managing the biometric authentication prompts. It configures the biometric prompt, handles authentication results, and ensures proper communication with the UI through a result channel.
How does the tutorial handle different types of biometric authentication?
-The tutorial allows for multiple authentication methods by using the BiometricManager's authenticators, such as fingerprint (BIOMETRIC_STRONG), face ID, and device credentials like security patterns or PIN codes.
What happens when the biometric authentication is not set up on the device?
-If biometric authentication is not set up, the app prompts the user to set up a biometric method by directing them to the device's settings. This is handled using an 'Enroll Intent' to guide the user through setting up their preferred authentication method.
What are some of the potential results that the app handles during biometric authentication?
-The app handles multiple authentication results, including authentication success, failure, hardware unavailability, feature unavailability, and if no biometric authentication is set up on the device.
How does the app manage error states during biometric authentication?
-The app uses a custom 'BiometricResult' enum to handle different error states, such as authentication failure due to user mistakes (wrong fingerprint), hardware unavailability, or a lack of enrolled biometric data.
What is the significance of using a Channel for managing biometric results?
-Using a Channel allows the app to send multiple biometric results over time and observe them in the UI. This enables handling different authentication states asynchronously, ensuring that the UI can react to real-time changes.
Why does the tutorial include checks for Android version 30 and above?
-The tutorial checks for Android API level 30 or higher because certain biometric features, like supporting both biometric authentication and device credentials (PIN or pattern), are only available starting from Android 30.
What happens if the device's biometric hardware is unavailable?
-If the device's biometric hardware is unavailable or in use by another app, the app informs the user that the biometric authentication feature is temporarily unavailable.
How does the tutorial ensure compatibility with both Android's Jetpack Compose and traditional views?
-The tutorial uses Jetpack Compose for the UI, but ensures compatibility with traditional Android views by handling the activity lifecycle and managing the BiometricPrompt in an AppCompatActivity, which is compatible with older Android versions.
Outlines
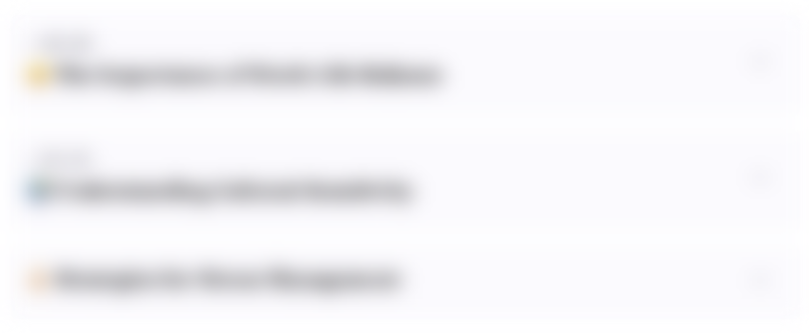
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
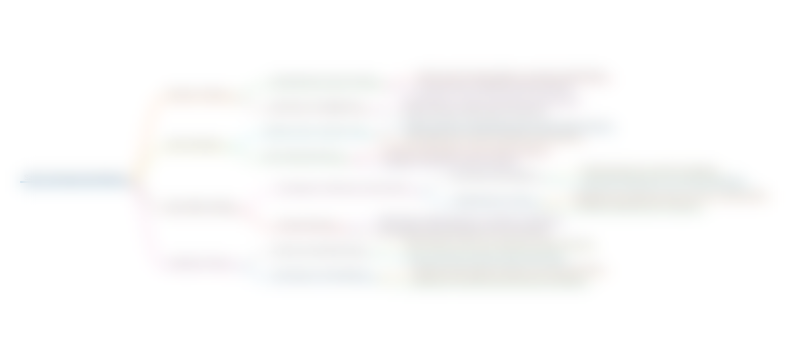
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
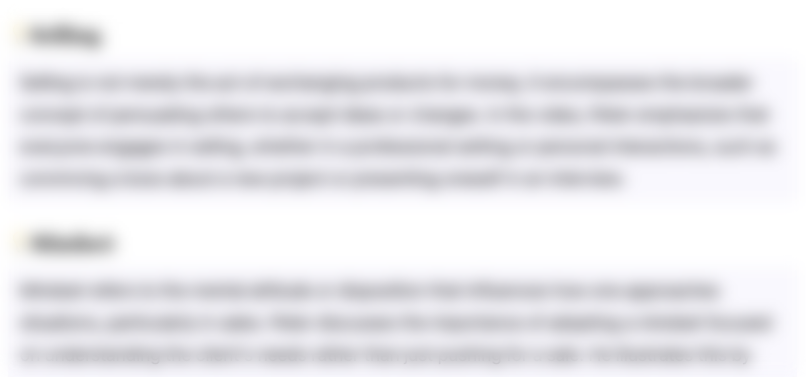
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
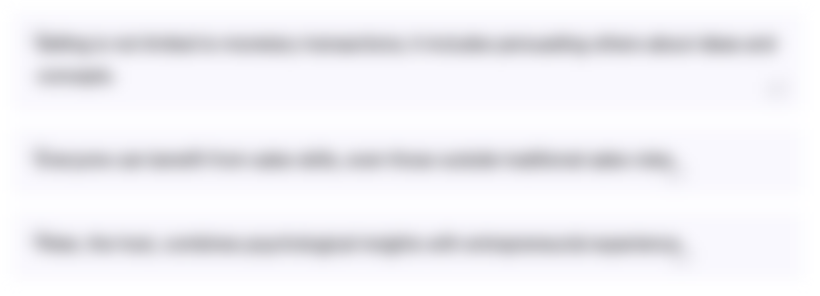
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
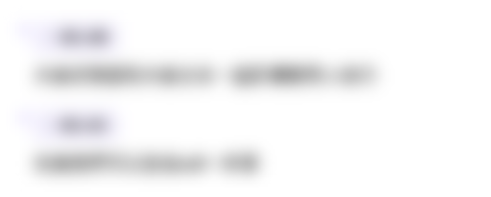
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
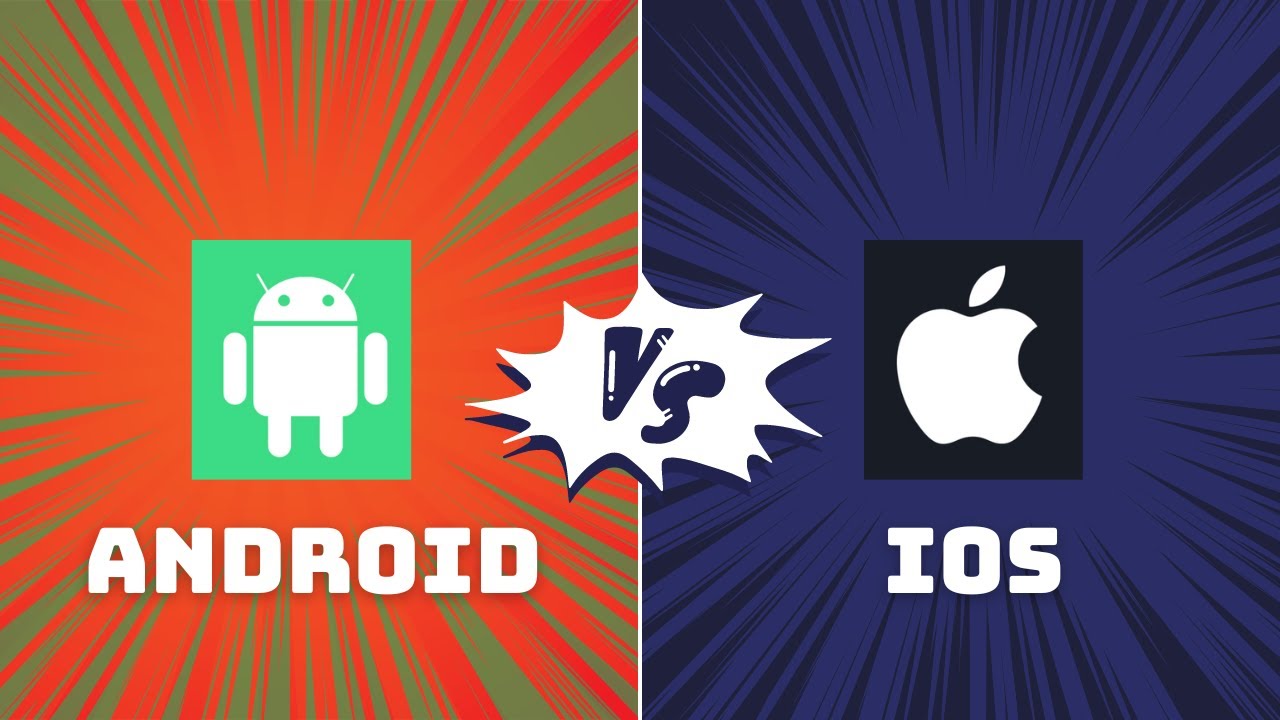
iOS vs Android... cual es mas seguro?
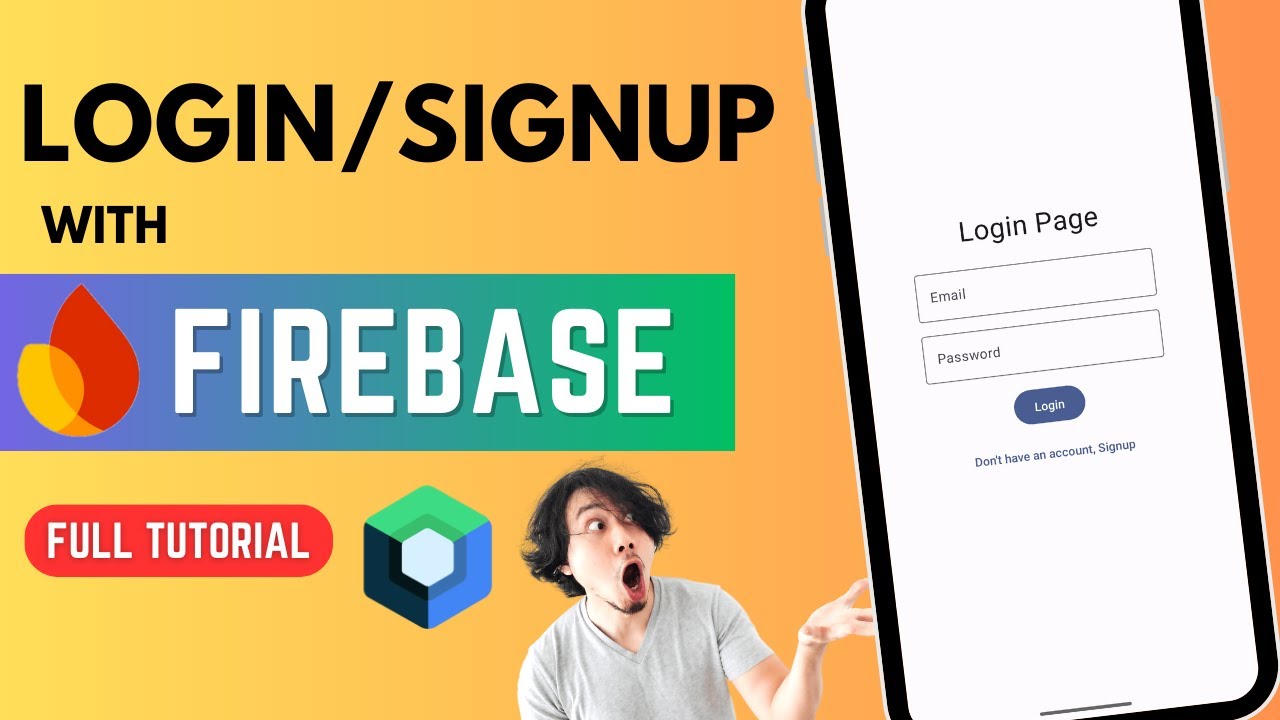
All about Firebase Authentication 🔥 | Login & Signup | Jetpack Compose
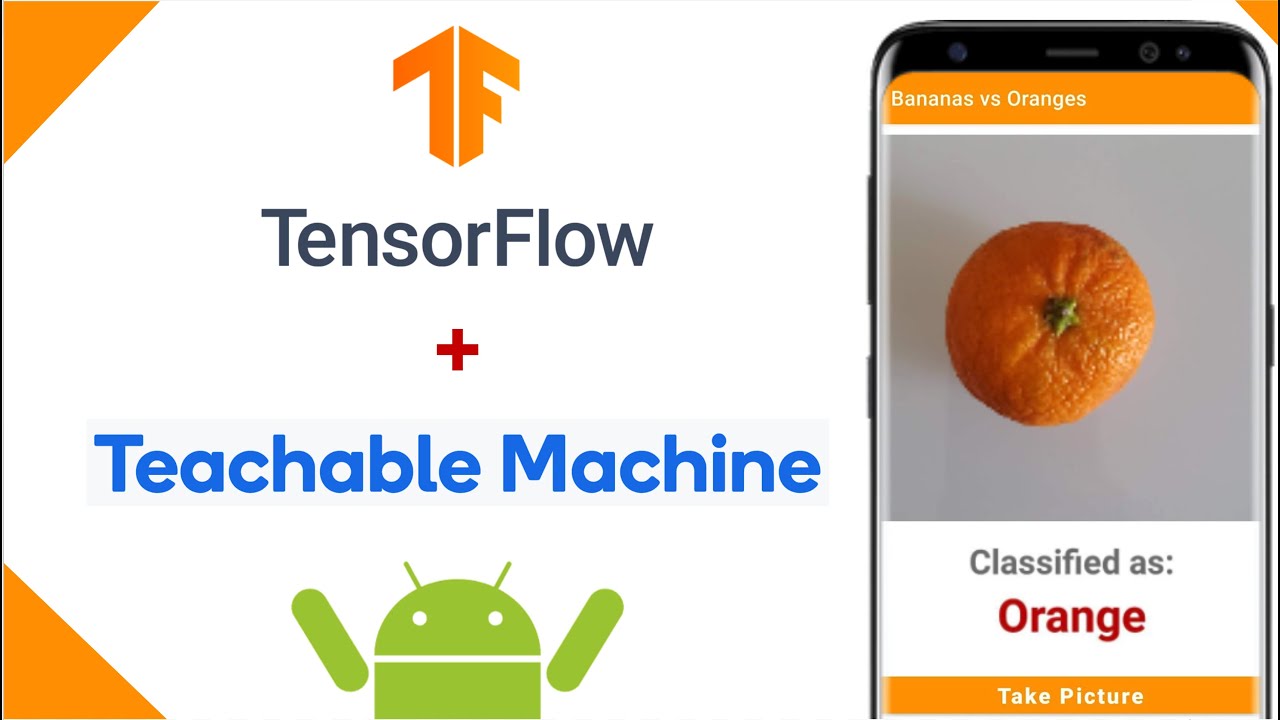
Image Classification App | Teachable Machine + TensorFlow Lite
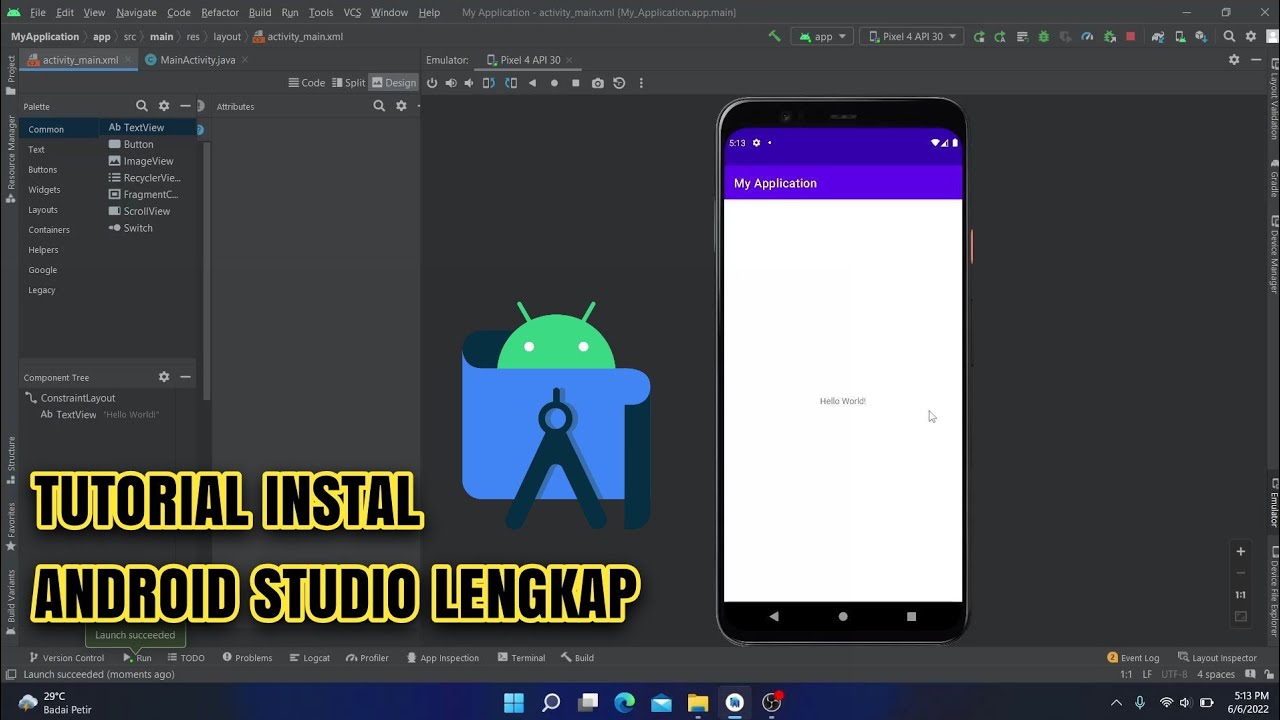
Cara Instal Android Studio di Windows Lengkap (JDK, Android Studio, SDK, AVD/Emulator)

Read & Write ESP32 From Android using Kodular Via Bluetooth Serial
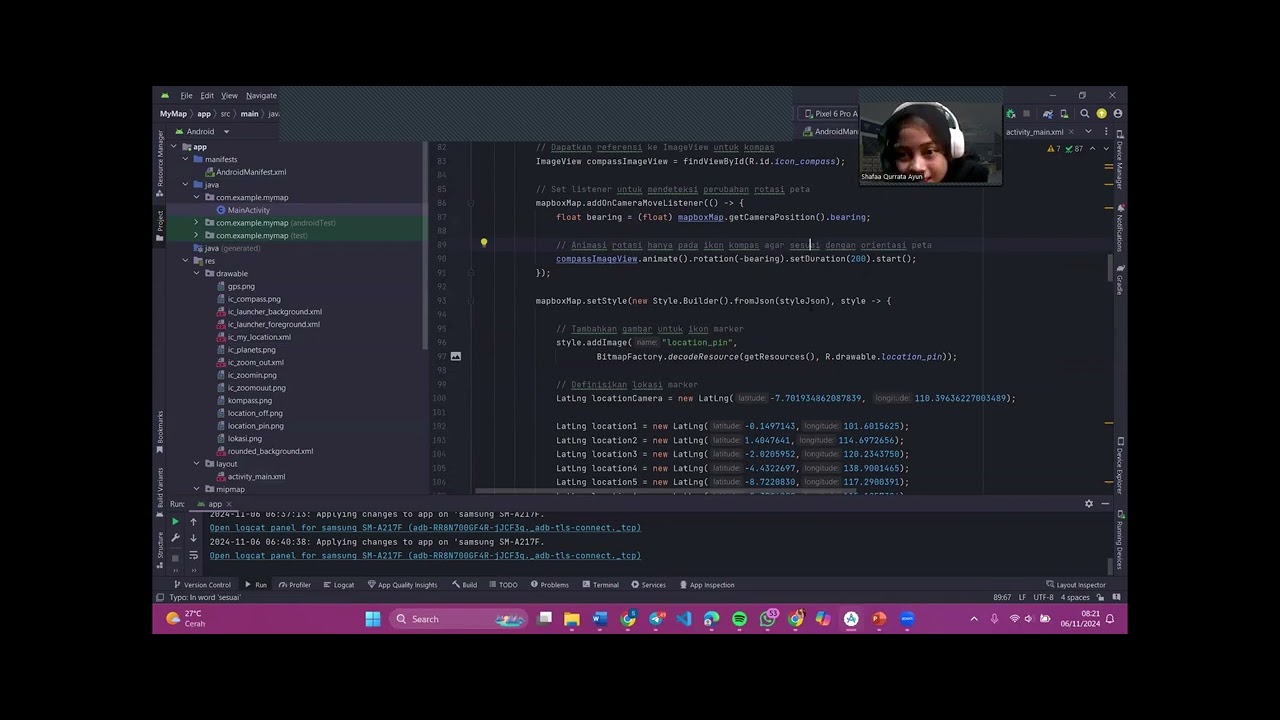
PGPB Acara 9 Maps Activity
5.0 / 5 (0 votes)