Python OOP Tutorial 2: Class Variables
Summary
TLDRIn this video tutorial, viewers learn the critical distinctions between instance variables and class variables in Python. Instance variables are unique to each class instance, while class variables are shared across all instances. The tutorial uses an Employee class to illustrate these concepts, demonstrating how to apply raises using a class variable for a consistent raise percentage. It also highlights the importance of properly managing class variables, such as maintaining a count of employees. By the end, viewers gain a solid understanding of when and how to use these variables effectively in their programming.
Takeaways
- 😀 Class variables are shared among all instances of a class, unlike instance variables that are unique to each instance.
- 😀 A good use case for class variables is to store data that is consistent across all instances, such as annual raise amounts.
- 😀 Class variables can be accessed through the class itself or any instance of the class.
- 😀 Modifying a class variable affects all instances that haven't overridden that variable with their own instance variable.
- 😀 If an instance has an attribute with the same name as a class variable, the instance's attribute will take precedence.
- 😀 It's beneficial to keep constants like raise amounts as class variables to avoid hard-coding values in multiple locations.
- 😀 Class variables can be modified using class names, ensuring that all instances reflect the updated value unless overridden.
- 😀 When creating new instances, it's useful to maintain a count of the total number of instances using a class variable.
- 😀 Class variables should be used for attributes that should remain constant across all instances, such as the total number of employees.
- 😀 Future lessons will cover static methods and class methods, which serve different purposes from class and instance variables.
Q & A
What are instance variables in a class?
-Instance variables are attributes that hold data unique to each instance of a class, defined using the 'self' argument.
How do class variables differ from instance variables?
-Class variables are shared among all instances of a class, while instance variables can vary for each individual instance.
Why would a company use a class variable for the annual raise amount?
-A class variable for the annual raise amount allows the company to easily manage a common value applied to all employees, ensuring consistency.
How can you access a class variable in Python?
-A class variable can be accessed either through the class itself (e.g., 'Employee.raise_amount') or through an instance of the class (e.g., 'self.raise_amount').
What happens if you set a class variable using an instance?
-If you set a class variable using an instance, it creates a new instance attribute for that specific instance, which overrides the class variable only for that instance.
What is the significance of the __init__ method in relation to class variables?
-The __init__ method is called every time an instance is created, making it a suitable place to increment class variables like the total number of employees.
Can class variables be overridden in subclasses?
-Yes, class variables can be overridden in subclasses, allowing different behavior or values for class variables based on the subclass.
Why is it beneficial to use class variables for tracking the number of employees?
-Using a class variable for tracking the number of employees ensures that all instances share a single count, simplifying management and consistency across instances.
What will happen if a class variable is modified using the class name?
-Modifying a class variable using the class name will update that variable for all instances of the class.
What are the next topics to be covered in future videos?
-The next videos will cover class methods and static methods, which further enhance the understanding of class design in Python.
Outlines
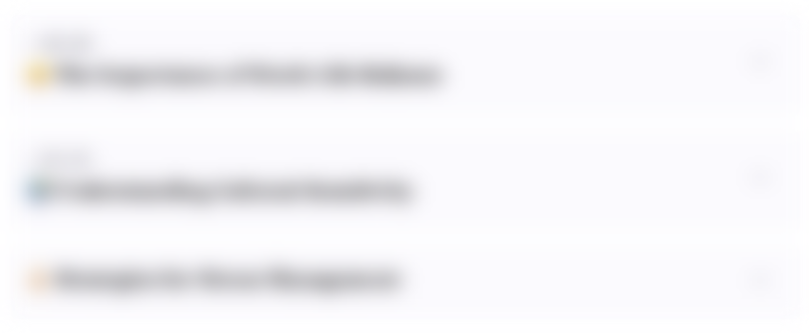
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
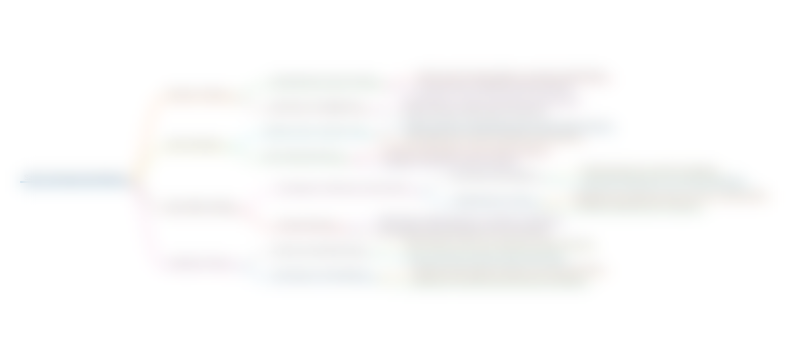
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
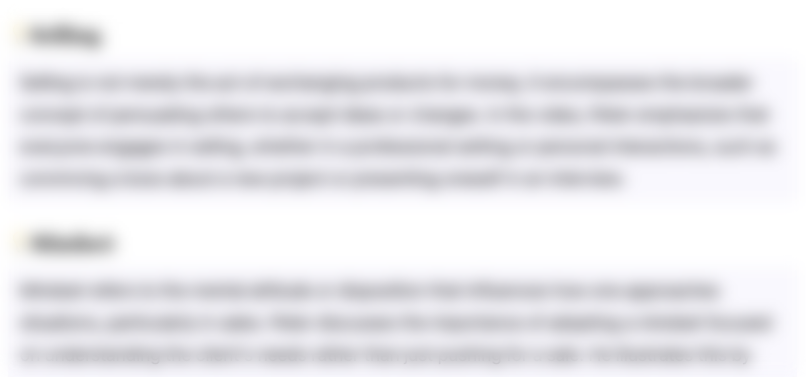
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
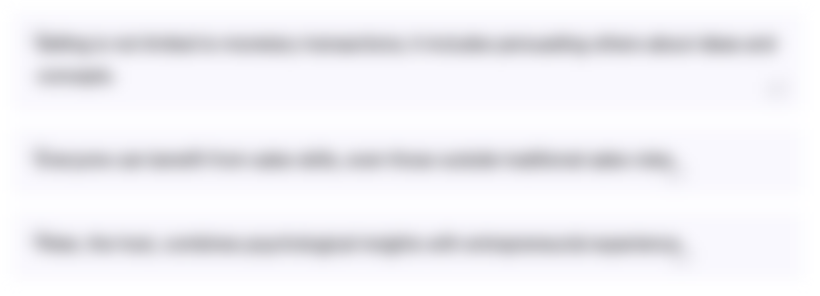
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
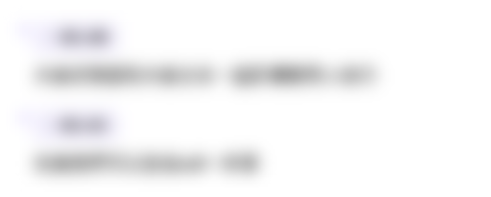
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenant5.0 / 5 (0 votes)