Internals of Maps in Golang
Summary
TLDRThis video delves into the internal workings of Go's map data type, explaining how it utilizes a map header and buckets to store key-value pairs efficiently. It highlights the role of hash functions in selecting appropriate buckets and the significance of seed values in generating hash values. The implementation of various operations, including insertion and retrieval, is explored, emphasizing the use of pointers and type descriptors to manage different data types. The video provides a comprehensive overview of how Go manages its map structure behind the scenes, showcasing its design for performance and flexibility.
Takeaways
- 🗺️ The Go programming language uses a built-in map data type that stores key-value pairs, managed by a map header containing metadata.
- 🔍 Each map header points to an array of buckets, with each bucket containing eight slots for key-value pairs.
- 📈 Go maps grow dynamically; when buckets are around 80% full, memory is allocated for twice the current number of buckets.
- ⚙️ A hash function determines the bucket and slot for each key-value pair, ensuring an efficient and even distribution of values.
- 🛡️ The hash function's output is affected by a random seed, making the same key generate different hash values across different instances.
- 📦 The `maphash` package provides hash functions, but currently only for byte sequences, with proposals for more data types in the future.
- 🔄 Go simulates generics for map data types, enabling the use of multiple types while maintaining performance.
- 🏗️ Key insertion involves generating a hash, identifying the corresponding bucket, and iterating over slots to find a free one or overwrite existing values.
- 🔑 Each slot uses a top hash value (the most significant byte of the hash) for efficient key comparison during insertion.
- 🚧 Additional logic is implemented to manage overflow buckets when current and overflow buckets reach capacity.
Q & A
What is the structure of a map in Go?
-A map in Go consists of a map header, which is a struct that contains metadata about the map, and an array of buckets where the key-value pairs are stored.
How does Go manage memory when a map grows?
-When a map's buckets become full, Go allocates new memory with twice the current number of buckets and copies the data incrementally from the old buckets to the new ones, starting the growth process when buckets are about 80% full.
What role does a hash function play in the Go map data structure?
-A hash function in a Go map converts keys into integer values to determine the bucket index where key-value pairs will be stored, ensuring efficient distribution of keys across buckets.
What issues can arise from a poorly distributed hash function?
-If a hash function does not provide a good distribution of values, multiple keys may end up in the same bucket, leading to longer retrieval times and slower overall map operations.
How does the maphash package function in Go?
-The maphash package provides hash functions primarily for byte sequences. It does not currently support all data types, but there is a proposal to extend this functionality to any comparable type.
What is the significance of the seed in hash functions in Go?
-The seed affects the hash values generated; each instance of the maphash's Hash object is initialized with a different seed, resulting in different hash values for the same key across different map instances.
How do generics relate to maps in Go?
-Generics, introduced in Go 1.18, allow maps and other container data types to support multiple types. The Go runtime manages this through unique hmap structs for each map and a single maptype for each unique map declaration.
What is the process for inserting a key-value pair in a Go map?
-The insertion process involves generating a hash value for the key, using a mask to determine the bucket index, and iterating over the bucket to find an available slot or overwrite an existing value.
What does the tophash value represent in a Go map bucket?
-The tophash value is the top byte of the hash value for each key in the bucket, allowing for efficient comparisons when checking for existing keys during insertion.
What happens if the current bucket and its overflow buckets are full?
-The code manages overflow buckets by allocating additional memory when the current bucket and connected overflow buckets are full, ensuring that the map can continue to store new key-value pairs efficiently.
Outlines
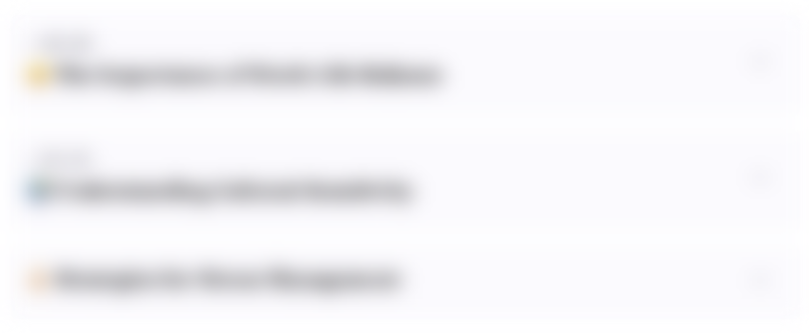
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
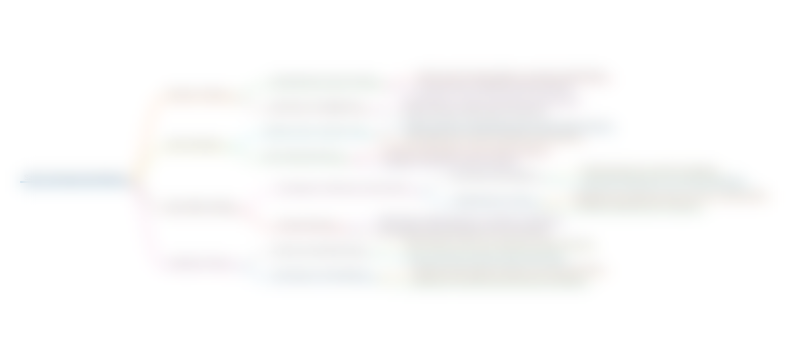
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
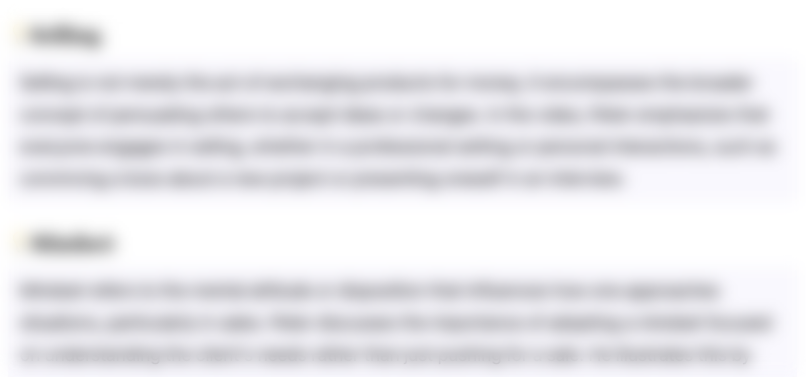
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
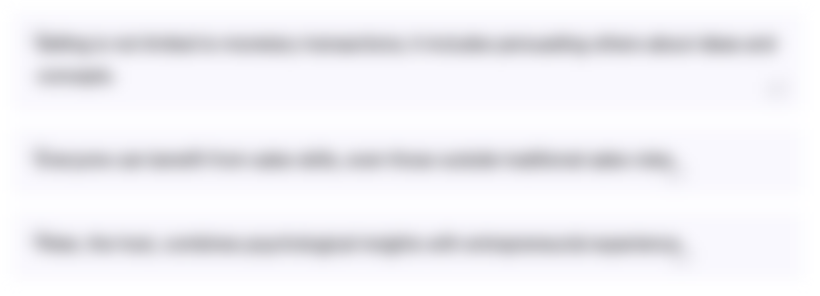
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
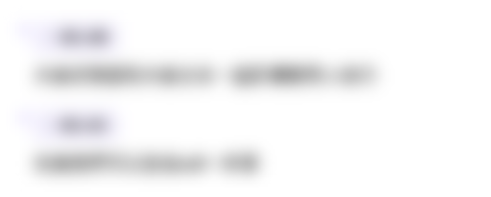
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
5.0 / 5 (0 votes)