Inheritance in Java - Java Inheritance Tutorial - Part 2
Summary
TLDRThis Java inheritance tutorial covers key concepts such as calling superclass methods using the 'super' keyword, using 'instanceof' to check object types, and the implications of shadowing superclass fields. The video also discusses how constructors aren't inherited, requiring explicit calls to superclass constructors. Additionally, it explores how nested classes behave in inheritance, with different access modifiers controlling accessibility. Finally, abstract classes are explained, highlighting their role in object-oriented programming where working with real-life objects like cats and dogs makes abstract classes useful. The tutorial is practical and informative for understanding inheritance in Java.
Takeaways
- đ The tutorial covers Java inheritance concepts, focusing on key topics such as method overriding, calling superclass methods, and using the `super` keyword.
- đ§© `super` keyword is used to call a superclass method, especially when the same method is overridden in the subclass.
- đŸ Demonstration of using `super` in the `Cat` class to call `setNumberOfLegs` method from its superclass `Animal`.
- đ§Ș The `instanceof` keyword helps identify if an object is an instance of a particular class or its superclass.
- đ Example provided shows that `Cat` is an instance of both `Cat` and `Animal`, but not of a different subclass `Dog`.
- đ Variables in a subclass with the same name as those in a superclass hide the superclass variables, a concept known as shadowing or variable hiding.
- â ïž Shadowing can lead to confusion, as shown in the example where the `numberOfLegs` variable behaves differently when accessed in different contexts.
- đïž Constructors in inheritance are not inherited automatically; they must be explicitly called using `super()`.
- đ Nested classes in inheritance have access restrictions based on their modifiers (`private`, `protected`, and `default`), impacting their accessibility in subclasses.
- đĄ Abstract classes cannot be instantiated and are useful when a superclass is only meant to provide a template for subclasses, as demonstrated with the `Animal` class.
Q & A
What is the use of the `super` keyword in Java inheritance?
-The `super` keyword in Java is used to refer to the superclass's methods or variables. It allows access to the superclass's version of a method or variable, even if it has been overridden in the subclass.
How can `super` be used in constructors?
-In constructors, the `super` keyword can be used to call the superclass's constructor explicitly. This must be done as the first statement in the subclass's constructor, and it helps initialize superclass properties when creating subclass objects.
What is the purpose of the `instanceof` keyword in Java?
-The `instanceof` keyword is used to check whether an object is an instance of a specific class or a subclass. It returns a boolean value indicating whether the object is of the specified type.
What is shadowing or hiding in Java inheritance?
-Shadowing or hiding occurs when a subclass defines a variable with the same name as a variable in its superclass. This hides the superclass variable, and when accessed within the subclass, it refers to the subclass variable unless `super` is used.
What happens when fields are shadowed in a subclass?
-When fields are shadowed in a subclass, the subclass variable takes precedence, and the superclass variable is hidden. However, by using the `super` keyword, the superclass's field can still be accessed.
Are constructors inherited in Java?
-No, constructors are not inherited in Java. If a subclass needs to use the constructor of a superclass, it must explicitly call it using `super`. If no constructor is defined, Java provides a default no-argument constructor.
What are abstract classes, and how are they used in Java inheritance?
-Abstract classes are classes that cannot be instantiated and may contain abstract methods, which are methods without a body. Inheritance is used to extend these abstract classes, and the subclass must implement the abstract methods.
Why would you mark a class as abstract in Java?
-A class is marked as abstract when it is not meant to represent concrete objects but serves as a blueprint for subclasses. It allows for the creation of subclasses with more specific implementations, while preventing direct instantiation of the abstract class.
How do nested classes behave in Java inheritance?
-Nested classes in Java inherit the same access rules as other members (methods and variables) of a class. A nested class marked as `private` won't be accessible to subclasses, whereas `protected` and `default` access modifiers allow subclasses to access nested classes depending on the package and inheritance context.
What is the significance of casting objects in the context of inheritance?
-Casting allows objects of a subclass to be treated as objects of a superclass or interface. This is useful in scenarios where a method accepts a superclass type but can be passed a subclass object. However, runtime casting checks ensure the object is of the expected type, and using `instanceof` helps confirm the objectâs type before casting.
Outlines
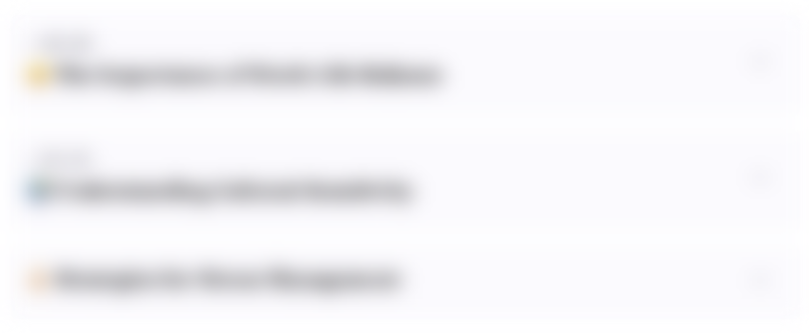
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
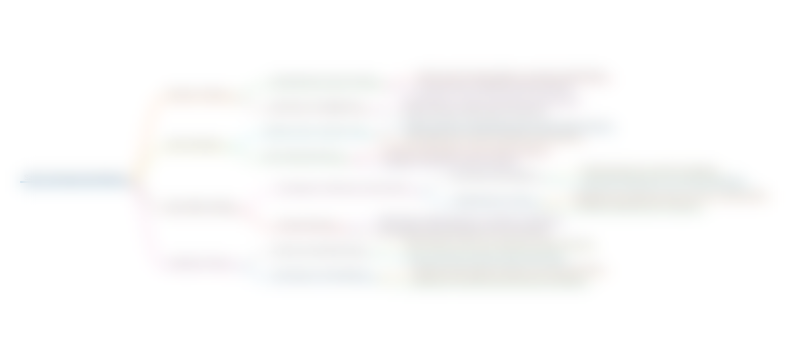
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
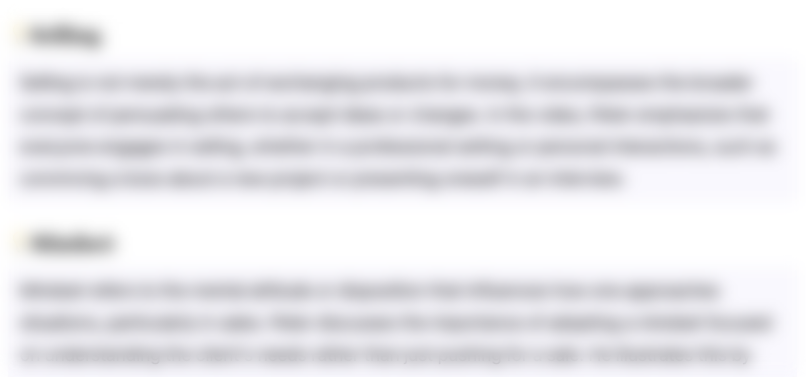
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
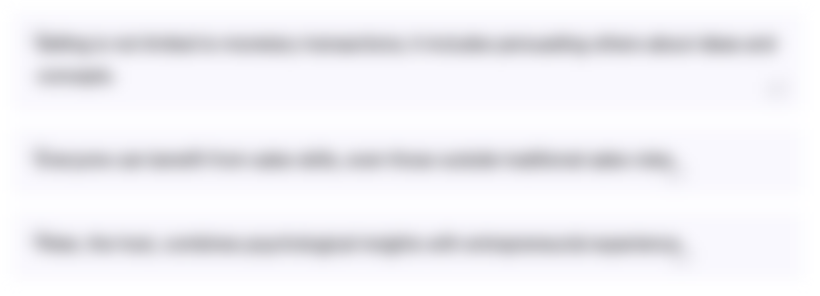
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
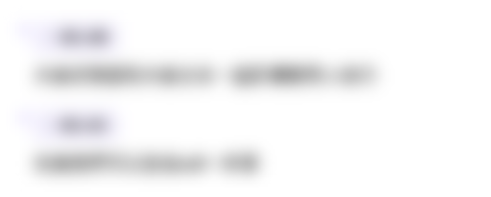
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
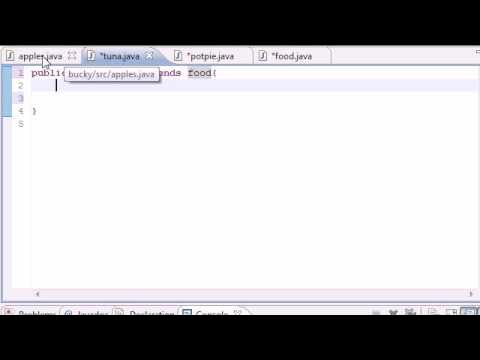
Java Programming Tutorial - 49 - Inheritance
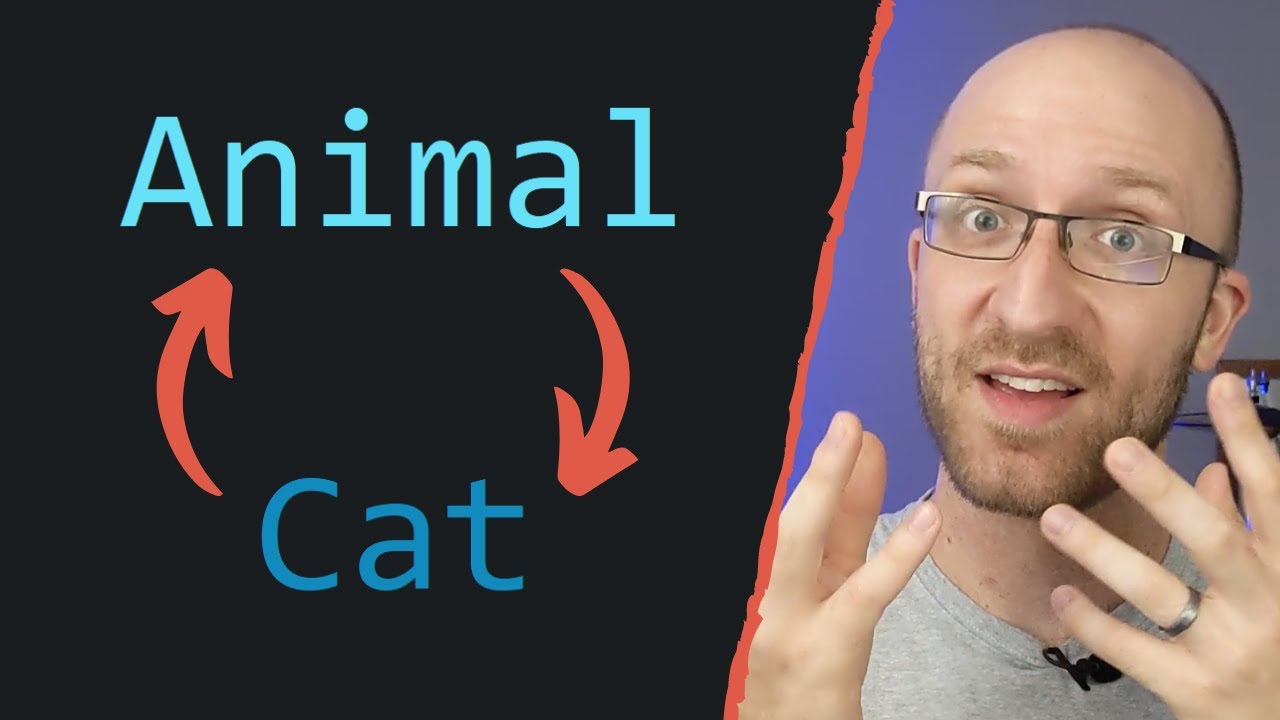
Upcasting and Downcasting in Java - Full Tutorial

Inheritance in Java - Java Inheritance Tutorial - Part 1
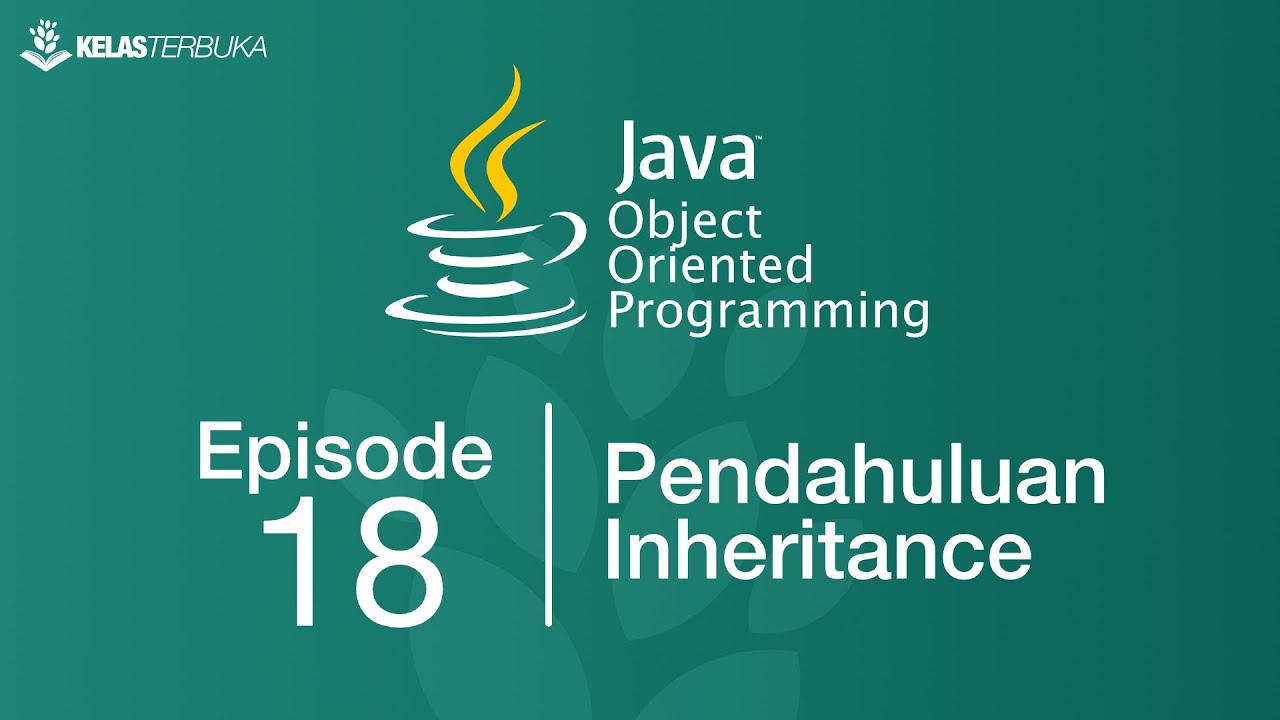
Belajar Java [OOP] - 18 - Pengenalan Inheritance
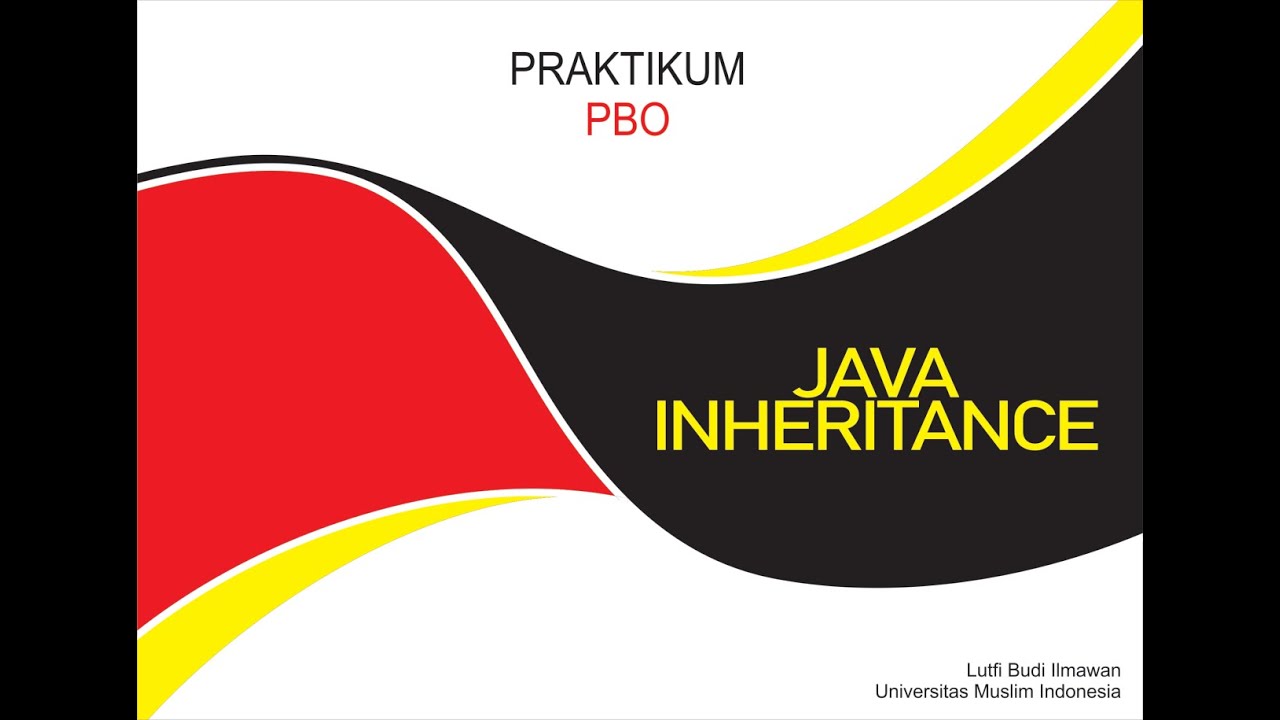
Praktikum 2 PBO - Java Inheritance

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
5.0 / 5 (0 votes)