C# Primitive Types and Variables | Datatype Literals and Variables | C# Tutorial | Simplilearn
Summary
TLDRPepper Khandelwal introduces C# primitive types and variables in a Simply Learn YouTube session. The tutorial covers variable concepts, types (primitive and reference), and details on signed, unsigned, and floating-point data types. It concludes with a practical implementation, demonstrating how to declare and use these variables in C#, including examples for integers, floating points, characters, booleans, and strings.
Takeaways
- 😀 The video introduces C# primitive types and variables.
- 🔔 The presenter encourages viewers to subscribe for daily tech updates.
- 💾 Variables in C# are storage locations with a specific type that determines memory size and operations.
- 📈 There are two types of variables in C#: primitive and reference/object.
- 🔢 Primitive types include numbers and boolean values, with numbers further divided into signed and unsigned.
- 👍 Signed data types like sbyte, short, int, and long have negative to positive value ranges.
- 🚫 Unsigned data types like byte, ushort, uint, and ulong can only store non-negative values.
- 🔄 Floating-point types (float, double, decimal) store approximate values with different precision levels.
- 📝 The char type stores Unicode characters, and the bool type stores true or false values.
- 📑 The string type, although not a primitive type in some languages, is considered a primitive type in C#.
- 💻 Practical examples are given for declaring and printing different types of variables in C#.
Q & A
What is the primary purpose of a variable in programming?
-A variable is a named storage location that programs can access, and it has a type that governs its memory size, layout, and the operations that can be applied to it.
What are the two types of variables in C#?
-There are two types of variables in C#: primitive type variables and reference or object variables.
What is a primitive data type?
-Primitive data types are the basic data types built into the language, which can be further divided into numbers and boolean values.
What is the value range of a signed byte in C#?
-The value range of a signed byte in C# is from -128 to 127.
What is the value range of an unsigned integer (uint) in C#?
-The value range of an unsigned integer (uint) in C# is from 0 to 4 billion.
How do you define a floating-point variable in C#?
-Floating-point variables in C# are defined with a specific suffix: 'f' for float, 'd' for double, and 'm' for decimal.
What is the difference between signed and unsigned data types?
-Signed data types can store both positive and negative values, while unsigned data types can only store positive values.
What is the purpose of the 'bool' data type in C#?
-The 'bool' data type in C# is used to store Boolean values which are 'true' or 'false', commonly used in conditional statements.
How does C# handle string data types differently from other primitive types?
-In C#, strings are considered a reference type, unlike other primitive types, and they can contain a word or a sentence.
What is the significance of the 'char' data type in C#?
-The 'char' data type in C# is used to store a single Unicode character.
How can you toggle the value of a Boolean variable in C#?
-You can toggle the value of a Boolean variable in C# by using the '!' operator to change 'true' to 'false' or vice versa.
Outlines
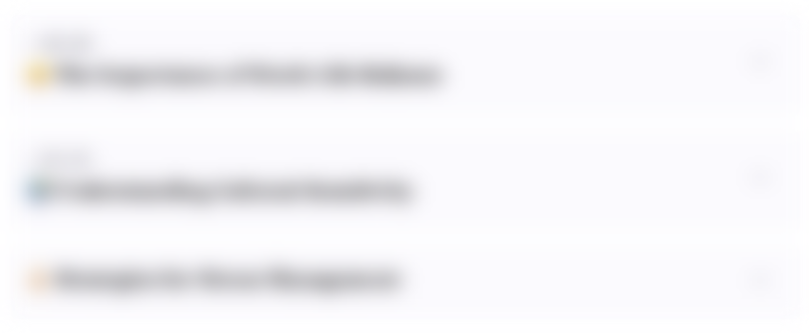
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
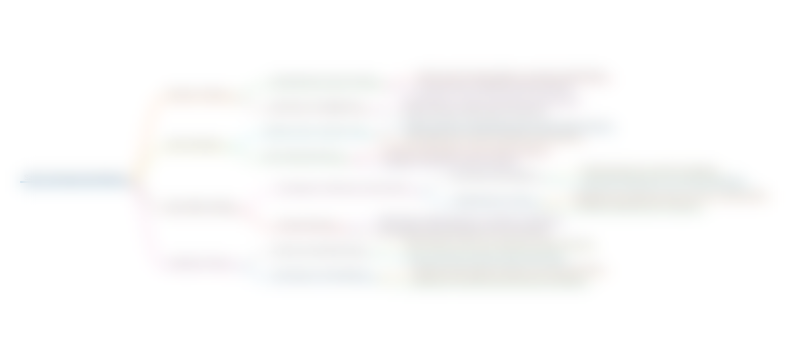
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
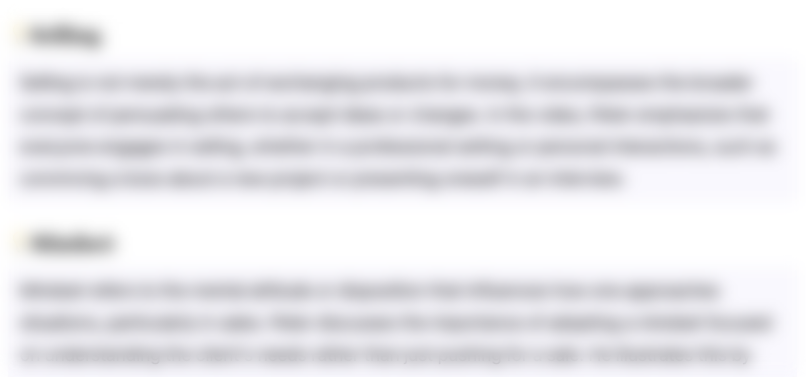
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
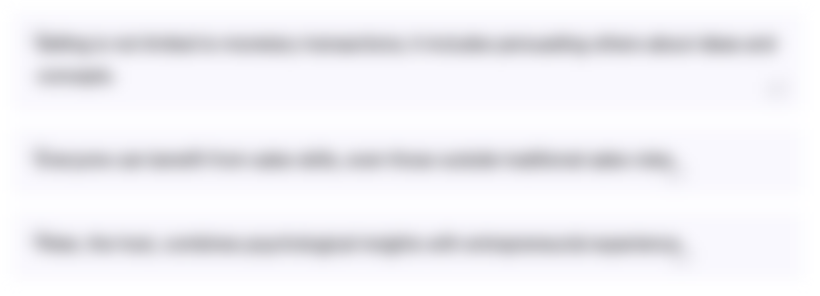
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
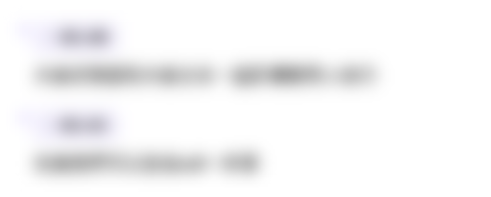
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
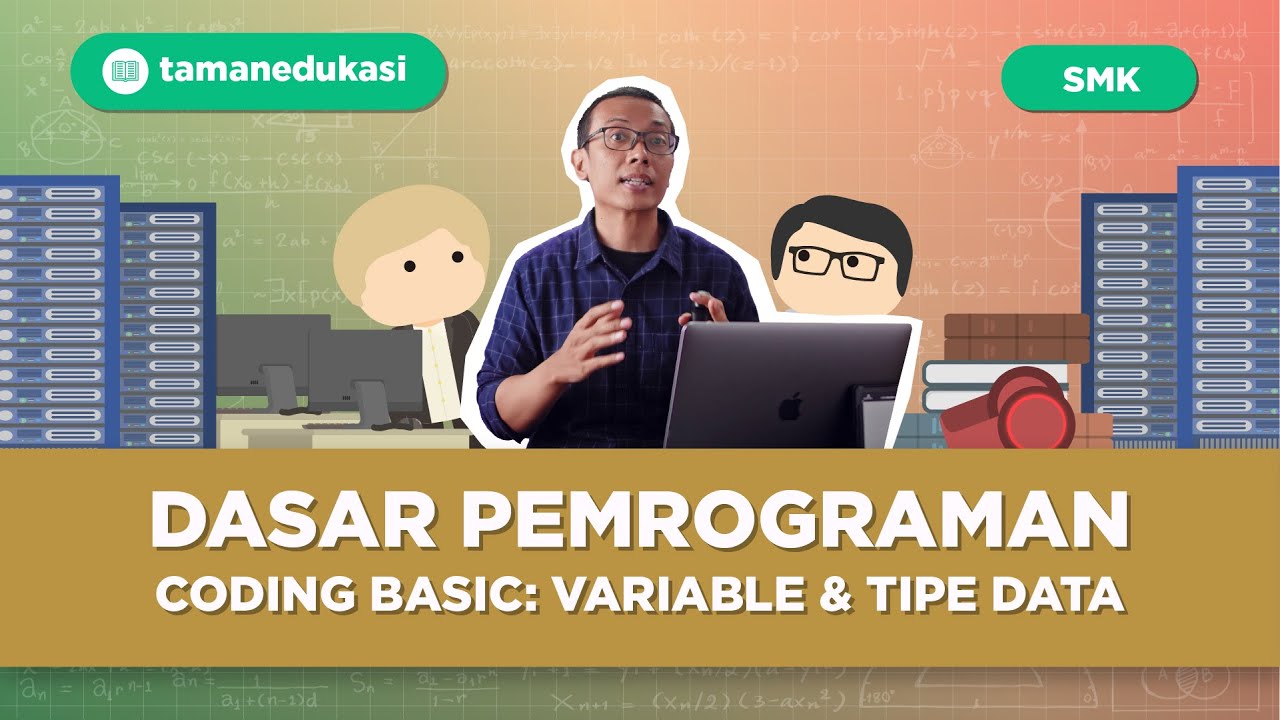
VARIABEL dan TIPE DATA dalam pemrograman yang penting untuk diketahui
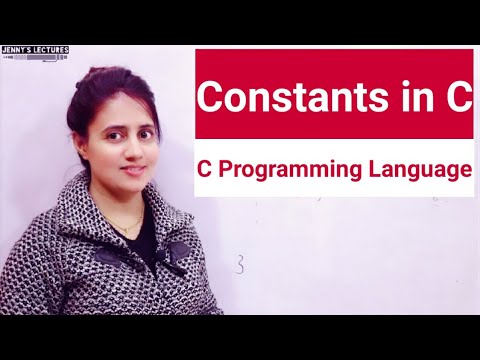
C_07 Constants in C | Types of Constants | Programming in C

Variables in Java ✘【12 minutes】
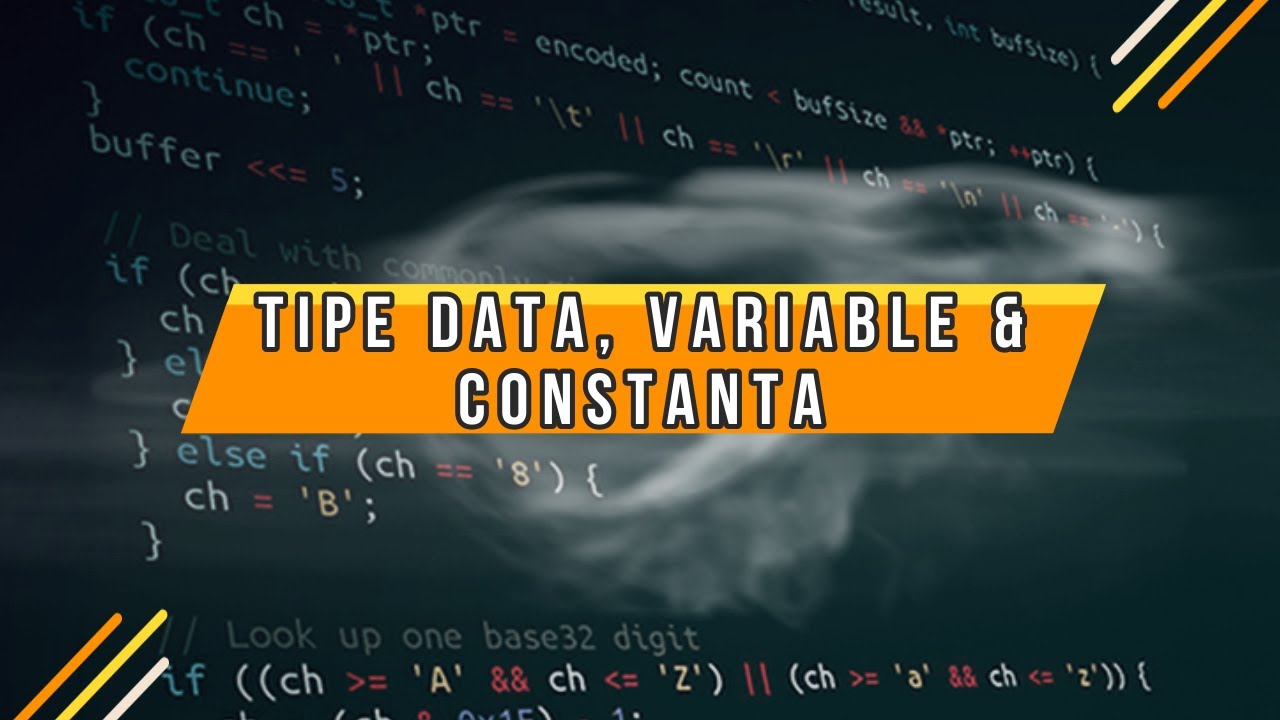
Pemrograman Dasar - Tipe Data, Variable & Constanta
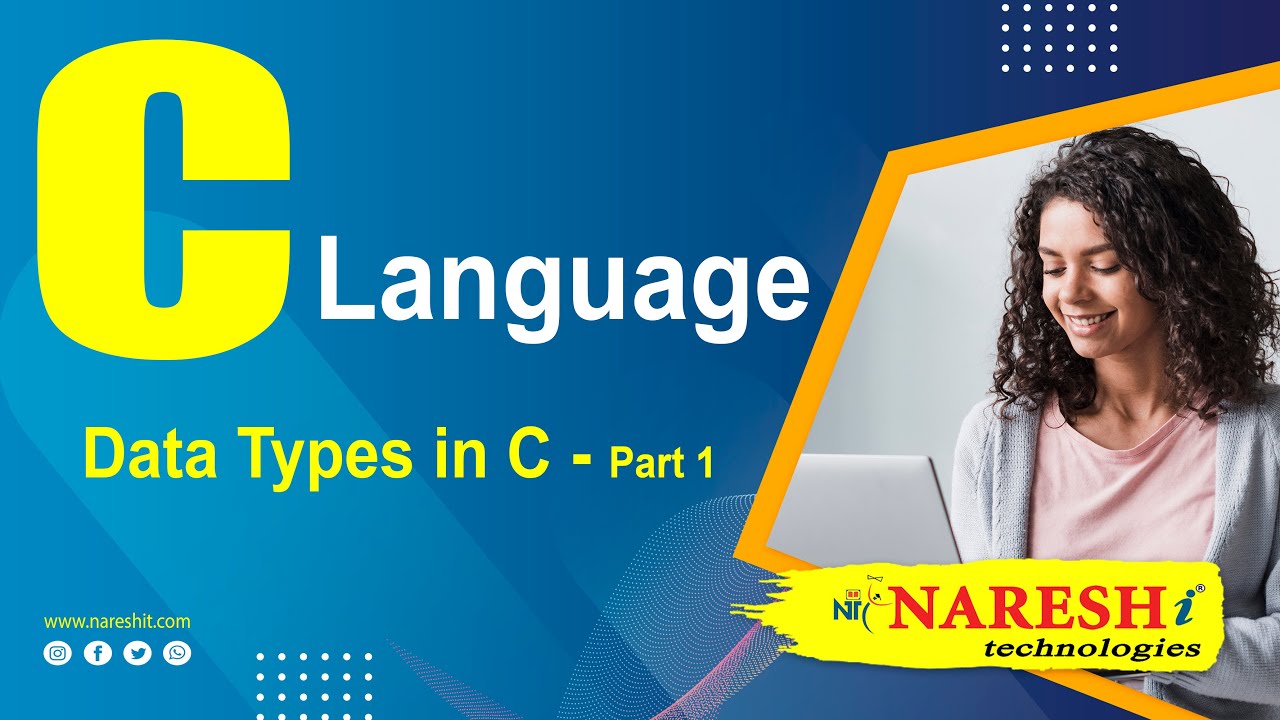
Data Types in C - Part 1 | C Language Tutorial

P_11 Data Types in Python | Python Tutorials for Beginners
5.0 / 5 (0 votes)