Introduction to Linked List
Summary
TLDRThis presentation introduces linked lists as a data structure for arranging elements like student names alphabetically. It contrasts linked lists with arrays, highlighting linked lists' ability to overcome arrays' limitations. The video explains different types of linked lists: single, doubly, and circular. It details how a single linked list is composed of nodes with data and a link to the next node, allowing forward navigation only. The script concludes by discussing how to access and traverse a linked list using a 'head' pointer.
Takeaways
- đ The presentation introduces linked lists as a new data structure for arranging data, specifically student names by the first letter.
- đ The goal is to maintain a list in memory, with linked lists being one of the two methods (the other being arrays).
- đ« Arrays have limitations and disadvantages, which linked lists aim to overcome.
- đ Linked lists come in different types: singly linked, doubly linked, and circular linked lists.
- âĄïž In a singly linked list, navigation is forward-only, making it the most basic type of linked list.
- đ A doubly linked list allows for both forward and backward navigation.
- đ Circular linked lists have their last element linked to the first, creating a loop.
- đĄ A node in a linked list consists of two parts: data and a link to the next node.
- đ Nodes in a linked list do not need to be stored in sequential order in memory, unlike arrays.
- đ The link part of each node contains the address of the next node, enabling traversal of the list.
- đ A pointer named 'head' is used to access the first node of the linked list, making the entire list accessible.
Q & A
What is the main topic of this presentation?
-The main topic of this presentation is linked lists, specifically introducing what a linked list is and how it looks like.
What is the purpose of arranging student names in the presentation?
-The purpose of arranging student names is to demonstrate how to maintain a list in memory, specifically in sequential order according to the first letter of their names.
What are the two ways mentioned to maintain a list in memory?
-The two ways to maintain a list in memory are using an array data structure and using a linked list data structure.
What is a single linked list?
-A single linked list is a list made up of nodes where each node contains data and a link to the next node in the list, allowing forward-only navigation.
How does a doubly linked list differ from a single linked list?
-A doubly linked list differs from a single linked list in that it allows forward and backward navigation due to each node containing a link to both the next and previous nodes.
What is a circular linked list?
-A circular linked list is a type of linked list where the last element is linked back to the first element, creating a circular structure.
What are the two main parts of a node in a single linked list?
-The two main parts of a node in a single linked list are the data part, which contains the actual data, and the link part, which contains the address of the next node in the list.
Why don't nodes in a linked list need to be stored in sequential order in memory?
-Nodes in a linked list do not need to be stored in sequential order in memory because the list's structure is defined by the links between nodes, not by their physical memory addresses.
What does the NULL value in the last node of a linked list signify?
-The NULL value in the last node of a linked list signifies that it is the end of the list, indicating that there are no more nodes to follow.
How is the first node of a linked list accessed?
-The first node of a linked list is accessed using a pointer, typically named 'head', which contains the address of the first node.
What is the next step after understanding how a single linked list looks like?
-The next step after understanding how a single linked list looks like is to learn how to create a single linked list programmatically and perform different operations on it.
Outlines
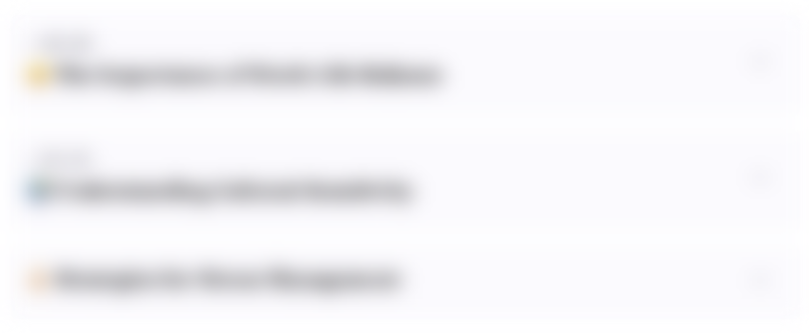
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
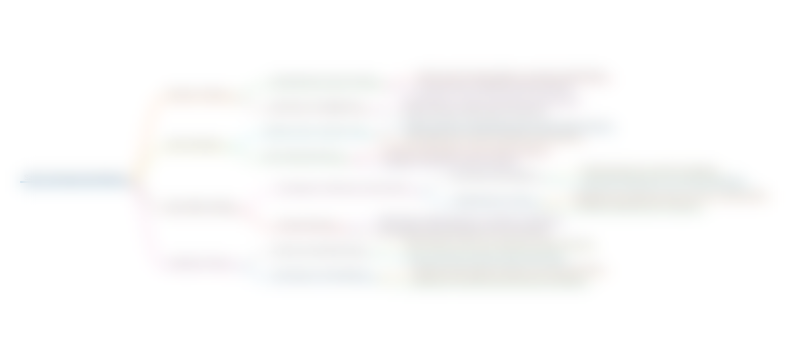
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
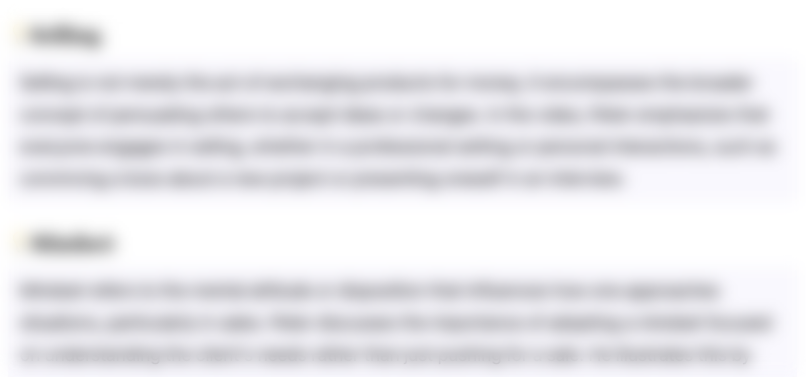
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
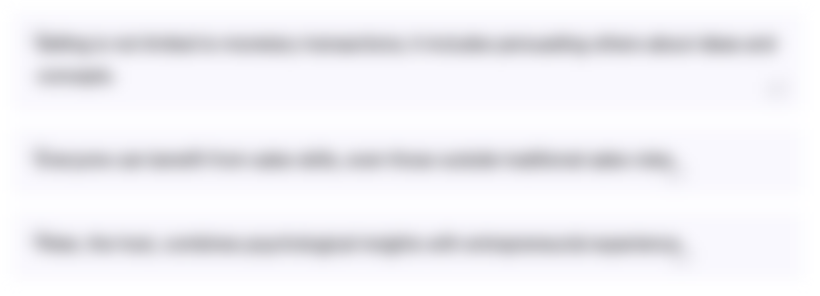
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
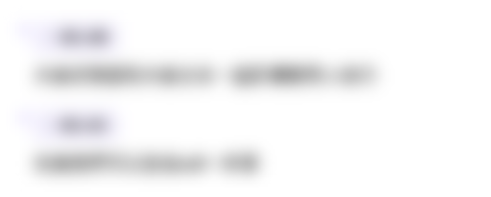
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
5.0 / 5 (0 votes)