Rules for Render Logic: Pure Components | Lecture 131 | React.JS 🔥
Summary
TLDRThis script delves into the fundamental concepts of render logic and event handler functions in React components. It emphasizes the importance of maintaining purity in render logic, adhering to functional programming principles, and avoiding side effects. The video explains how render logic describes the component's view and should always return the same output for the same input (props). In contrast, event handlers are responsible for handling user interactions, updating state, making HTTP requests, and manipulating the application. The script underscores the significance of separating these two types of logic to ensure the application's proper functioning and maintainability. It also touches upon the useEffect hook, which allows side effects to be registered and executed when the component mounts or updates.
Takeaways
- 😃 Render logic refers to the code that participates in describing how a component's view should look like, executed when the component is rendered.
- 😎 Event handler functions are executed as a consequence of an event they are listening to, containing code that makes things happen in the application like state updates and HTTP requests.
- 🤔 Pure functions do not have side effects, meaning they don't depend on or modify data outside their scope, and return the same output for the same input.
- 🚫 Side effects are interactions with the outside world, like modifying external data, making HTTP requests, or writing to the DOM.
- ✨ React requires components to be pure functions when it comes to render logic, meaning render logic must not produce any side effects.
- 🔒 Render logic is not allowed to mutate objects or variables outside its scope, including props and state, as it would create side effects.
- 🔄 Updating state in render logic would create an infinite loop, which is why it's not allowed.
- 👍 Side effects like console.log and random number generation are technically not allowed in render logic but are generally safe to use.
- 🎯 Side effects are allowed and encouraged in event handler functions, which are not part of render logic.
- 🔍 The useEffect hook can be used to register side effects that need to be executed when the component is first rendered.
Q & A
What is render logic in React?
-Render logic is all the code that participates in describing how the view of a certain component instance should look like. It includes the code at the top level of the component function and the return block where the component returns its JSX.
What are event handler functions in React?
-Event handler functions are pieces of code that are executed as a consequence of the event that the handler is listening to. They contain code that makes things happen in the application, such as state updates, HTTP requests, reading input fields, and page navigation.
Why is it important to differentiate between render logic and event handler functions in React?
-It's important to differentiate between these two types of logic because they do fundamentally different things. While render logic is code that renders the component, event handlers contain code that actually changes and manipulates the application in some way.
What does it mean for a component to be pure when it comes to render logic in React?
-For a component to be pure when it comes to render logic, it means that if we give a certain component instance the same props (input), then the component should always return the exact same output in the form of JSX. In other words, render logic is not allowed to produce any side effects.
What are side effects in functional programming?
-A side effect happens whenever a function depends on or modifies data that is outside its scope. It can be thought of as a function's interaction with the outside world, such as mutating an outside object, making HTTP requests, writing to the DOM, or setting timers.
What are pure functions in functional programming?
-Pure functions are functions without side effects. They do not change any variable outside their scope, and when given the same input, they'll always return the same output, making them predictable.
What are the rules for render logic in React components?
-The main rule is that components must be pure functions when it comes to render logic. This means that render logic is not allowed to perform network requests, create timers, directly work with the DOM API, mutate objects or variables outside the component scope, or update state or refs.
Why can't we mutate props in React?
-We cannot mutate props in React because doing so would be a side effect, and side effects are not allowed in render logic. This is one of the hard rules of React, as mutating props would violate the principle of components being pure functions when rendering.
Where are side effects allowed in React components?
-Side effects are allowed and encouraged to be used inside event handler functions, as these are not considered render logic. Additionally, if we need to create a side effect as soon as the component function is first executed, we can register that side effect using the useEffect hook.
What is the next topic mentioned in the script after discussing render logic and side effects?
-The next topic mentioned in the script after discussing render logic and side effects is state update batching.
Outlines
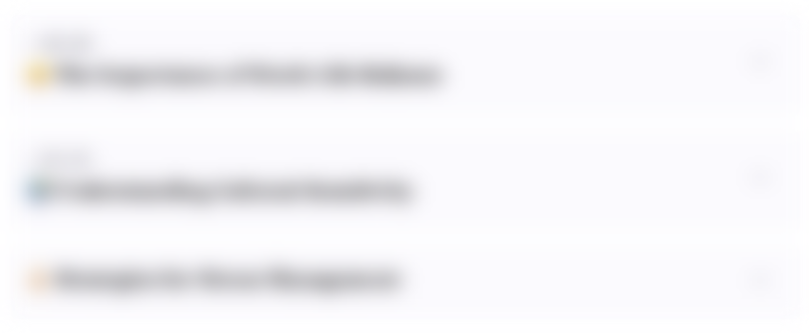
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
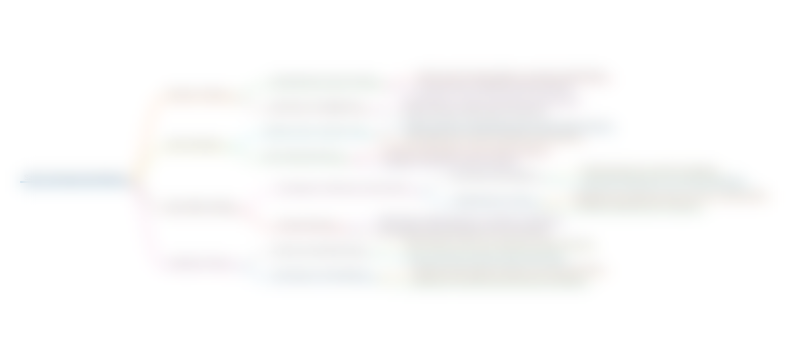
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
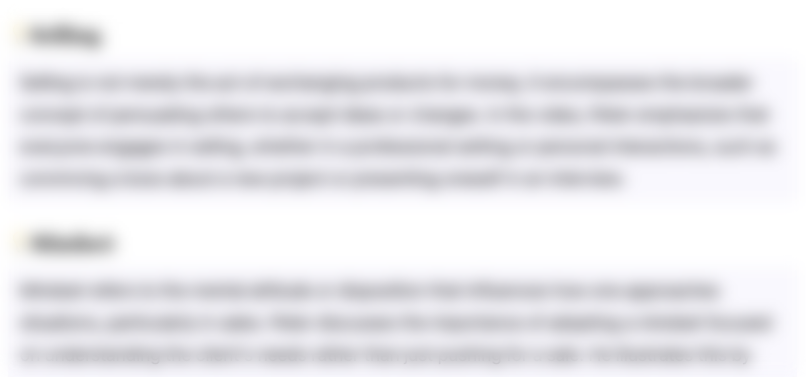
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
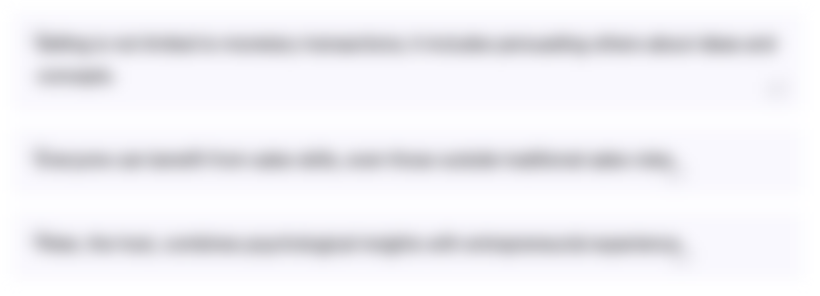
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
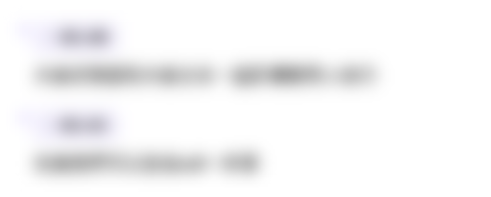
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

How NOT to Fetch Data in React | Lecture 139 | React.JS 🔥

A First Look at Effects | Lecture 141 | React.JS 🔥

useEffect to the Rescue | Lecture 140 | React.JS 🔥

Managing State With useReducer | Lecture 185 | React.JS 🔥
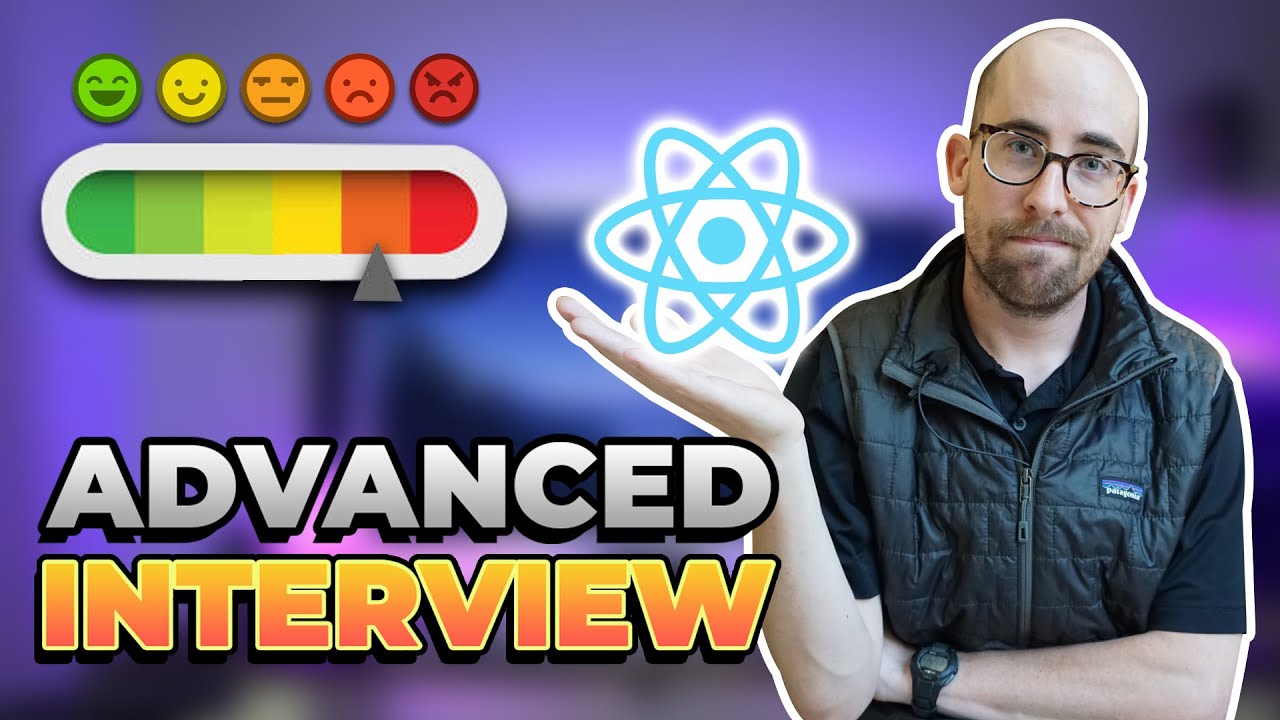
HARD React Interview Questions (3 patterns)

Understanding memo | Lecture 242 | React.JS 🔥
5.0 / 5 (0 votes)