Javascript Interview Questions ( map, filter and reduce ) - Polyfills and Output Based Questions
Summary
TLDRThis video script offers an in-depth exploration of JavaScript's array methods: map, filter, and reduce. It begins by explaining the purpose and functionality of each method, demonstrating their use through practical examples. The script then delves into creating polyfills for these methods from scratch, showcasing a deep understanding of JavaScript's prototypical inheritance. To solidify concepts, the script addresses common interview questions, providing solutions using map, filter, and reduce in various scenarios. The tutorial aims to prepare viewers for technical interviews, emphasizing the importance of these methods in JavaScript development.
Takeaways
- 📚 The video discusses the use of JavaScript array methods: map, filter, and reduce, which are fundamental for transforming and computing data within arrays.
- 🔍 Interviewers often ask about these methods in JavaScript interviews, including their functionality, differences, and how to implement them from scratch.
- 🛠 The 'map' method is used to create a new array by applying a function to each element of an existing array, potentially returning a transformed array.
- 🔎 The 'filter' method evaluates each element in the array against a condition; elements that meet the condition are included in the output array.
- 🔄 The 'reduce' method condenses an array into a single value by applying a function to each element that accumulates a result.
- 💡 The script provides examples of using these methods with JavaScript code, demonstrating how to multiply array elements, filter based on conditions, and calculate sums.
- 👩🏫 The presenter also covers how to create polyfills for these methods, showing custom implementations of 'myMap', 'myFilter', and 'myReduce'.
- 🔑 Differences between 'map' and 'forEach' are highlighted, emphasizing that 'map' returns a new array while 'forEach' does not return anything and can mutate the original array.
- 🔗 The ability to chain methods after 'map' and 'filter' due to their return value is contrasted with 'forEach', which cannot be chained because it does not produce an array.
- 📝 The script includes practical interview questions and demonstrates how to solve them using the discussed array methods, such as extracting student names in capital letters or filtering students based on marks.
- 📈 Advanced use cases are also covered, such as combining 'map' and 'filter' to solve more complex problems, like adding grace marks to students and then calculating the sum of their scores.
Q & A
What are the primary purposes of the 'map', 'filter', and 'reduce' array methods in JavaScript?
-The 'map', 'filter', and 'reduce' methods are used to iterate over an array and perform transformations or computations. The 'map' method creates a new array by applying a function to each element of the original array. 'Filter' applies a conditional statement to each element and returns a new array with elements that meet the condition. 'Reduce' reduces the array to a single value by applying a function that accumulates results.
Can you explain how the 'map' method works with an example?
-The 'map' method applies a function to each element of an array and returns a new array with the results. For example, if you have an array of numbers and you want to create a new array with each number multiplied by 3, you can use 'map' to iterate over the original array and apply the multiplication operation.
How does the 'filter' method differ from the 'map' method?
-While both 'map' and 'filter' iterate over array elements, 'map' transforms each element and returns a new array with all elements, 'filter' only returns elements that meet a specified condition. It does not transform the elements but rather selects a subset of the original array based on the condition.
What is the main difference between 'reduce' and the other two methods?
-Unlike 'map' and 'filter', which return arrays, 'reduce' collapses the array into a single value. It applies a function to each element in the array, accumulating the result in an accumulator variable, which is then returned as the final output.
Can you provide an example of creating a polyfill for the 'map' method from scratch?
-A polyfill for the 'map' method can be created by extending the Array prototype with a new function that iterates over the array elements, applies the callback function, and collects the results in a new array. The polyfill would look something like this: `Array.prototype.myMap = function(callback) { let temp = []; for (let i = 0; i < this.length; i++) { temp.push(callback(this[i], i, this)); } return temp; };`
What is the purpose of the callback function in the 'reduce' method?
-The callback function in 'reduce' is responsible for accumulating the result. It takes four parameters: the accumulator, the current value, the current index, and the array being reduced. The accumulator accumulates the callback's return values; it's the accumulated value previously returned in the last invocation of the callback, or the initial value if provided.
How can you chain methods like 'map' and 'filter' together?
-Chaining methods in JavaScript allows you to use the output of one method as the input for another. For example, you can first filter an array to get elements that meet a certain condition and then map the resulting array to transform those elements further.
What is the difference between using 'map' and a traditional 'for' loop for transforming array elements?
-While both 'map' and a 'for' loop can be used to transform array elements, 'map' is more concise and functional, automatically creating a new array with the transformed elements. A 'for' loop, on the other hand, requires manual array creation and element assignment, which can be more error-prone and less readable.
Can you give an example of how to use 'filter' to get students with marks greater than 60?
-You can use 'filter' to create a new array that only includes students who scored more than 60 marks. The syntax would be something like: `const highMarksStudents = students.filter(student => student.marks > 60);`
How can you combine 'map', 'filter', and 'reduce' to solve more complex array manipulation tasks?
-For complex tasks, you can chain these methods to perform multiple operations in sequence. For example, you might first use 'filter' to select elements based on certain criteria, then 'map' to transform those elements, and finally 'reduce' to aggregate the results into a single value.
Outlines
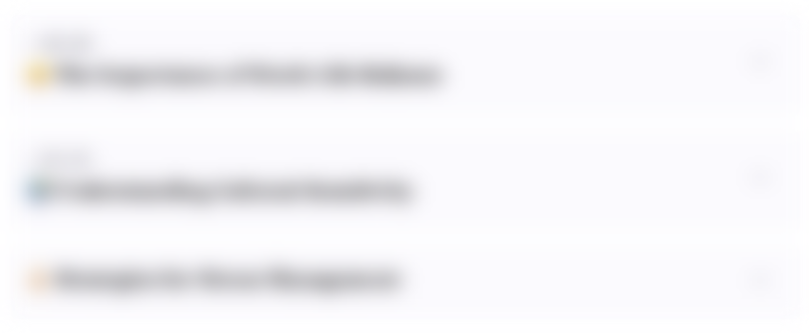
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
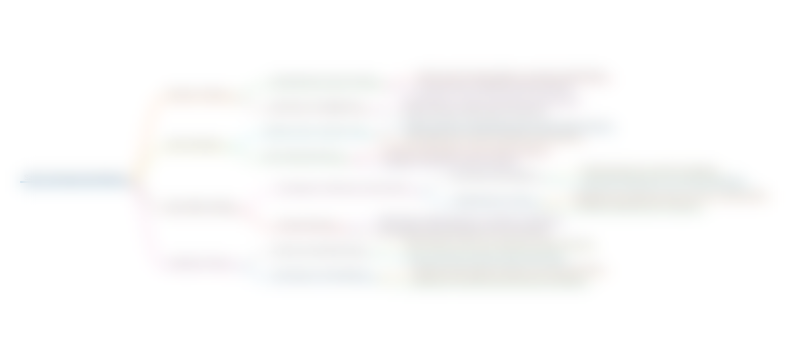
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
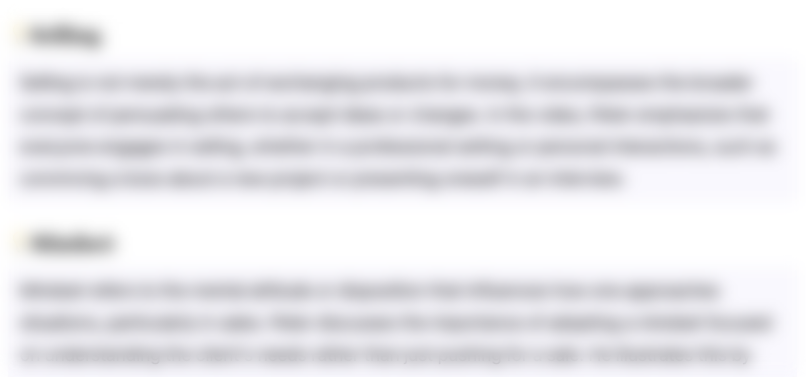
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
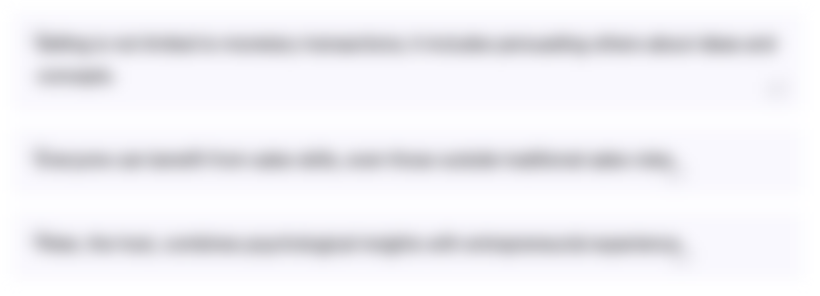
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
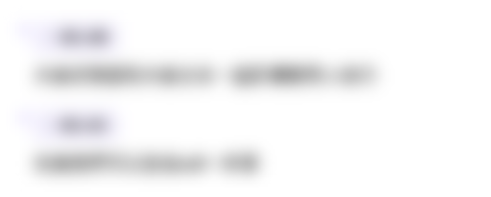
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
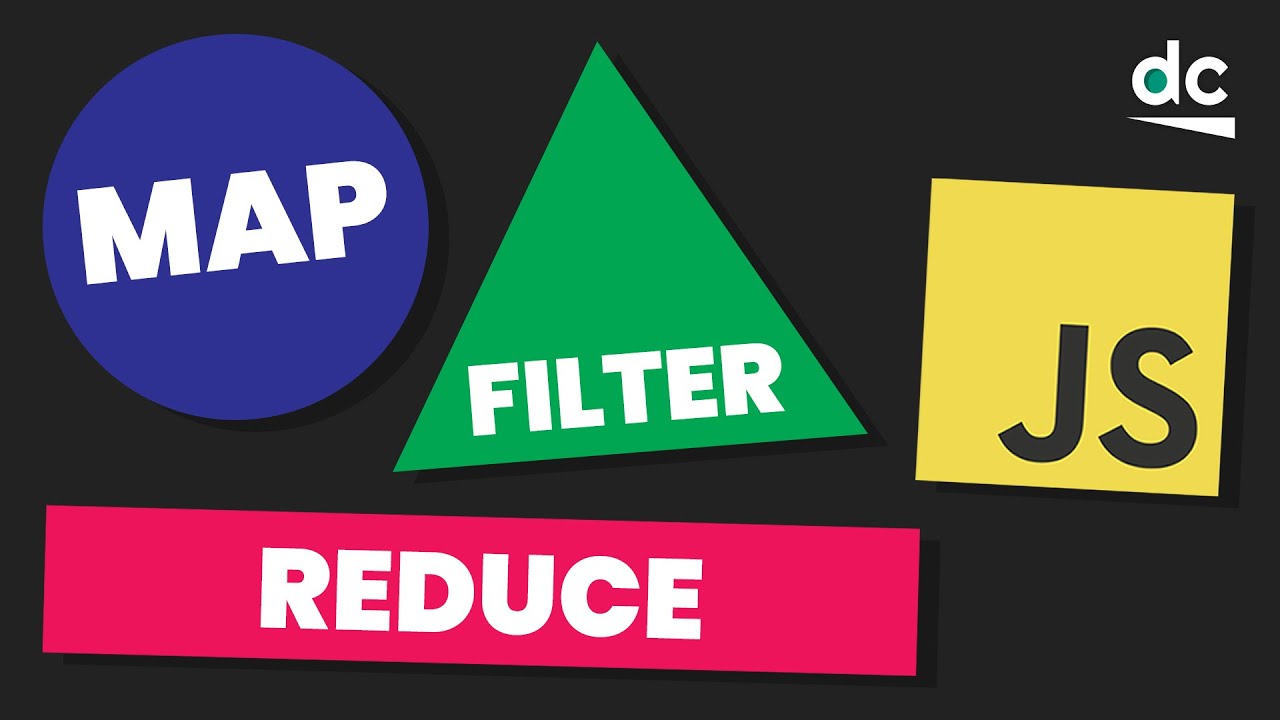
Map, Filter & Reduce EXPLAINED in JavaScript - It's EASY!
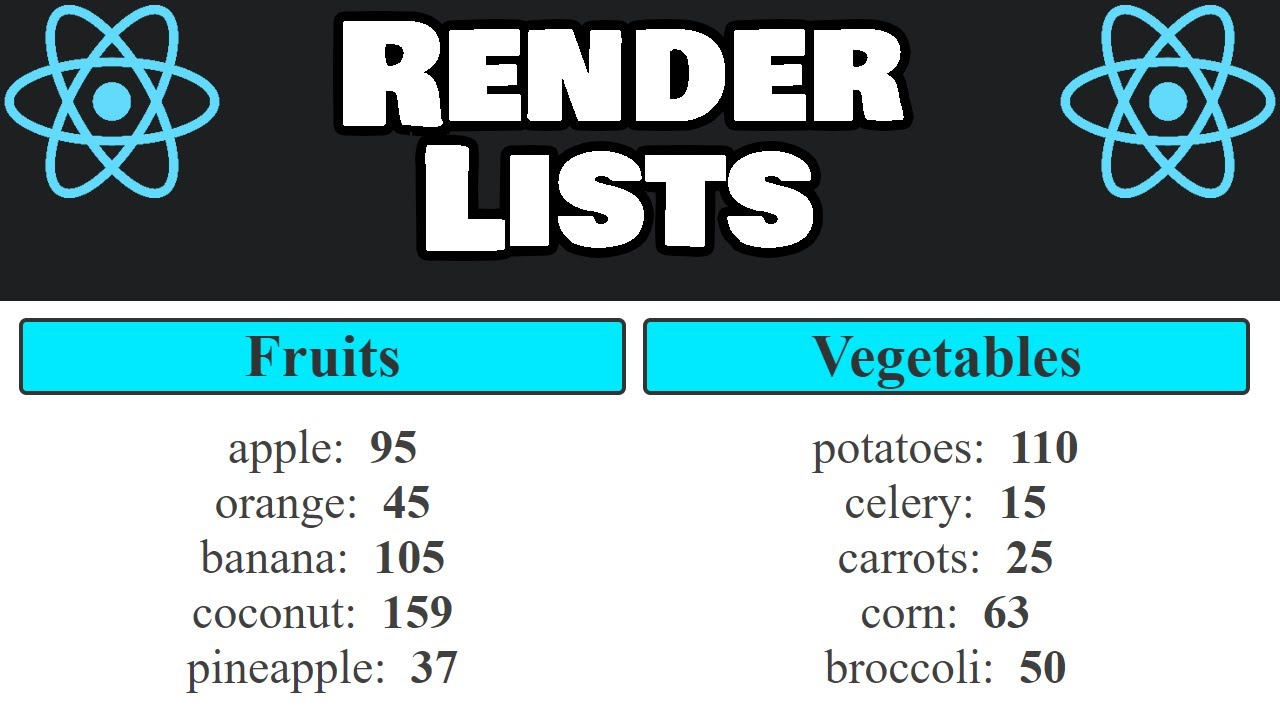
How to render LISTS in React 📃

Crack Your Java Interview With Most-Asked Questions | Java Fundamentals

SUPER() in Python explained! 🔴
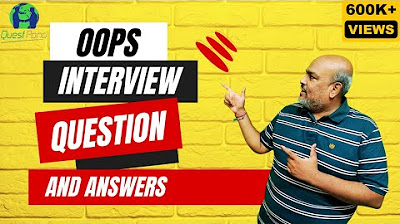
OOPS Interview Questions and Answers | Object Oriented Programming Interview Questions C#
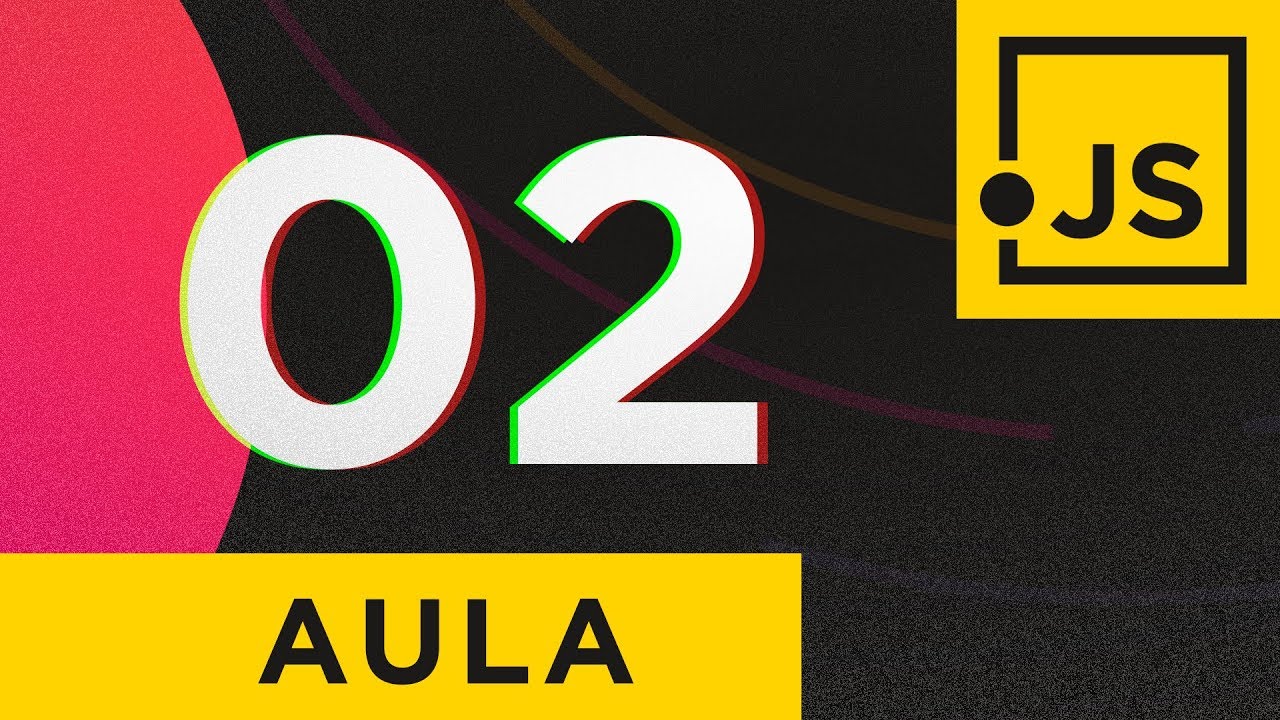
JavaScript: como chegamos até aqui? - Curso JavaScript #02
5.0 / 5 (0 votes)