Understanding the Dangling Pointers
Summary
TLDRThis presentation delves into the concept of dangling pointers in programming, explaining that they are pointers to non-existing memory locations. It demonstrates how a pointer can become dangling after memory has been allocated by 'malloc' and then deallocated with 'free', without the pointer being reinitialized. The solution is to set the pointer to NULL after deallocation. Additionally, it warns against the dangers of returning the address of a local variable from a function, which can also result in a dangling pointer and lead to segmentation faults, emphasizing the importance of best practices to avoid such issues.
Takeaways
- 🔍 A dangling pointer is a pointer that points to a non-existing memory location.
- 💡 malloc is a function used to dynamically allocate memory, returning the address of the first byte of the allocated memory.
- 🔧 After using allocated memory, it should be deallocated using the free function to avoid memory leaks.
- 📌 The pointer should not be left pointing to deallocated memory; it must be reinitialized to avoid becoming a dangling pointer.
- ⚠️ A pointer that still contains the address of deallocated memory is considered dangling and can lead to undefined behavior.
- 👉 The simplest solution to avoid a dangling pointer is to reinitialize the pointer to NULL after freeing the memory.
- 🛠️ Dangling pointers can cause problems such as segmentation faults when the programmer attempts to dereference them.
- 🚫 It is bad practice to return the address of a local variable from a function, as the variable will no longer exist once the function execution is complete.
- 🌐 Understanding and avoiding dangling pointers is crucial for writing robust and error-free code.
- 📝 Initializing pointers to NULL at the start is a good practice to ensure they do not inadvertently point to invalid memory.
- 🔄 Proper memory management, including initializing, allocating, deallocating, and reinitializing pointers, is essential for preventing dangling pointers.
Q & A
What is a dangling pointer?
-A dangling pointer is a pointer that points to a non-existing memory location, typically occurring after the memory it was pointing to has been deallocated or freed.
What is the role of malloc in memory allocation?
-Malloc is a function used to allocate memory dynamically. It returns the address of the first byte of the allocated memory block.
What is the purpose of the free function in memory management?
-The free function is used to deallocate or release memory that was previously allocated by malloc, making the memory available for future allocations.
Why does a pointer become dangling after memory is freed?
-A pointer becomes dangling after memory is freed because it still contains the address of the deallocated memory, which no longer exists.
How can you avoid a pointer from becoming a dangling pointer after freeing memory?
-To avoid a pointer from becoming a dangling pointer, you should reinitialize the pointer, typically setting it to NULL after the memory has been freed.
What is the consequence of dereferencing a dangling pointer?
-Dereferencing a dangling pointer can lead to undefined behavior, including program crashes or accessing illegal memory locations, which can cause a segmentation fault.
Why should you not return the address of a local variable from a function?
-Returning the address of a local variable from a function is problematic because local variables cease to exist once the function execution is complete, leaving the pointer pointing to a non-existing memory location.
What is the best practice for initializing a pointer before its first use?
-The best practice is to initialize a pointer with NULL before its first use, ensuring that it does not point to any object and is safely handled before being assigned an actual memory address.
What does a segmentation fault indicate in the context of dangling pointers?
-A segmentation fault indicates an attempt to read or write into an illegal memory location, which can occur when a dangling pointer is dereferenced.
Can you provide an example of how a dangling pointer might be created in a function?
-A dangling pointer might be created in a function when a pointer is assigned the address of a local variable and then returned from the function. Once the function execution ends, the local variable's scope ends, and the returned address becomes invalid.
Why is it important to handle pointers carefully in programming?
-Handling pointers carefully is important to prevent memory leaks, undefined behavior, and crashes, ensuring the stability and security of the software application.
Outlines
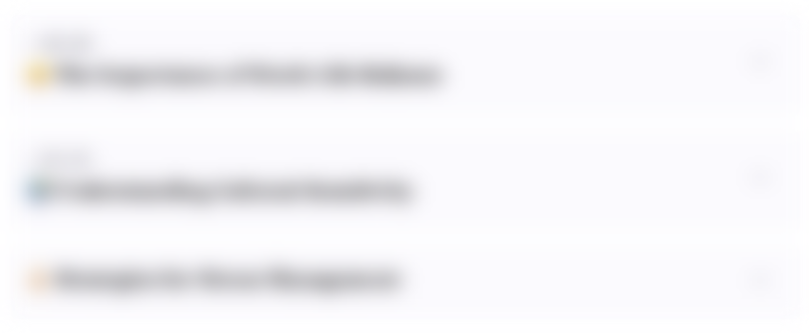
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
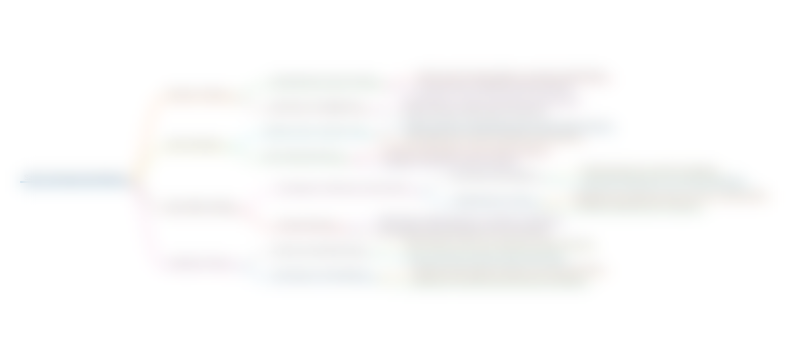
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
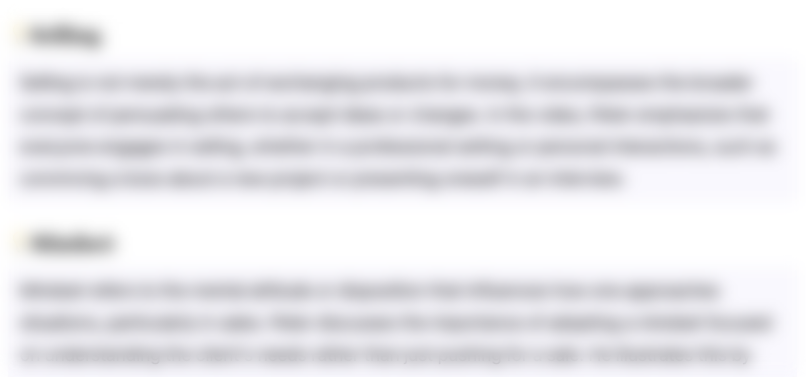
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
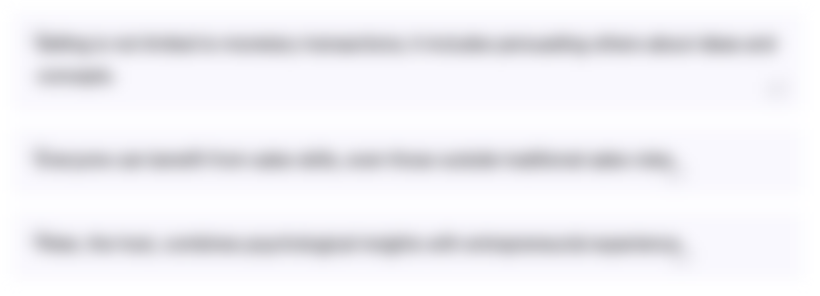
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
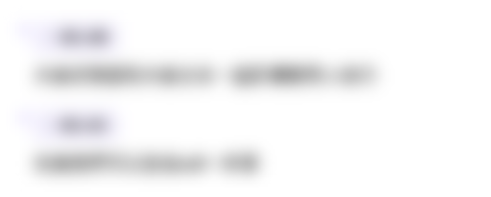
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahora5.0 / 5 (0 votes)