Higher Order Function | javascript interview series
Summary
TLDRIn this video, the host delves into the concept of higher-order functions in JavaScript, emphasizing their importance in interviews. He explains that higher-order functions either take a function as an argument or return a function. The video highlights practical examples, such as setTimeout and array filter methods, to illustrate the concept. Additionally, the host advises candidates to share personal experiences and project implementations to make their answers more engaging and impressive to interviewers. The goal is to transform the interview into a discussion, showcasing not just knowledge, but practical understanding and application of higher-order functions.
Takeaways
- 📚 A higher order function in JavaScript is defined as a function that takes another function as an argument or returns a function.
- 🔍 The candidate's eagerness to answer the definition from MDN is noted, but interviewers seek a deeper understanding and personal experience with higher order functions.
- 💡 The script suggests starting explanations with code examples to illustrate the concept of higher order functions, such as using `setTimeout`.
- 👨🏫 The importance of turning an interview question into a discussion is emphasized, sharing personal stories and experiences with higher order functions.
- 🔑 The script uses `filter` as an example of a higher order function, explaining how it processes arrays based on a provided function.
- 🛠️ The benefits of higher order functions include writing less code, improving readability, and making code more maintainable.
- 📈 The script provides an example of creating an `asyncHandler` to streamline error handling and database interactions in a project.
- 🔄 The `asyncHandler` example demonstrates how functions can be treated as variables, passed around, and composed to create more complex functionality.
- 📝 The candidate is encouraged to share specific use cases from their own projects to showcase their understanding and application of higher order functions.
- 🚀 The script concludes by advising candidates to focus on storytelling and personal experiences with higher order functions to impress interviewers.
- 👋 The video ends with an invitation to subscribe for more interview tips and to stay tuned for the next video in the series.
Q & A
What is the definition of a higher order function according to the video?
-A higher order function is a function that takes another function as an argument or returns a function.
Why is it important to discuss higher order functions in a JavaScript interview?
-Higher order functions are a common practice in JavaScript and discussing them can demonstrate a candidate's understanding and practical experience with functional programming concepts.
What is the first example of a higher order function given in the video?
-The first example given is 'setTimeout', which is a function that takes another function as an argument to execute after a specified delay.
How does the video suggest explaining the concept of higher order functions during an interview?
-The video suggests explaining higher order functions with code examples, starting with basic examples like 'setTimeout', and then moving on to more complex examples like using 'filter' and 'reduce'.
What is the advantage of using higher order functions in coding?
-Higher order functions allow for writing less code that is more readable, maintainable, and can be easily reused and modified.
Can you provide an example of how to use the 'filter' method as a higher order function?
-The 'filter' method can be used to process an array, for example, to filter out salaries above a certain value by providing a function that checks if each element meets the condition.
What is the purpose of creating an asyncHandler in the context of the video?
-The asyncHandler is created to simplify error handling and to avoid repetitive code when interacting with databases, making the code more efficient and less prone to errors.
How does the video suggest using higher order functions to improve the asyncHandler example?
-By treating functions as variables that can be passed and returned, the asyncHandler can wrap any function in a try-catch block and convert it to an async function, making it more versatile and error-resistant.
What is the significance of the 'useHandler' function in the asyncHandler example?
-The 'useHandler' function is significant because it demonstrates how to pass a function as an argument to another function, which then processes it within an async context and error handling, showcasing the power of higher order functions.
How can a candidate impress interviewers with their understanding of higher order functions?
-A candidate can impress interviewers by not only defining higher order functions but also by narrating a story of their first encounter with the concept, explaining how they implemented it in projects, and discussing its advantages.
What is the final advice given in the video for candidates preparing for a JavaScript interview?
-The final advice is to focus on understanding and being able to explain higher order functions with practical examples and use cases, which can demonstrate a well-versed programming skill set.
Outlines
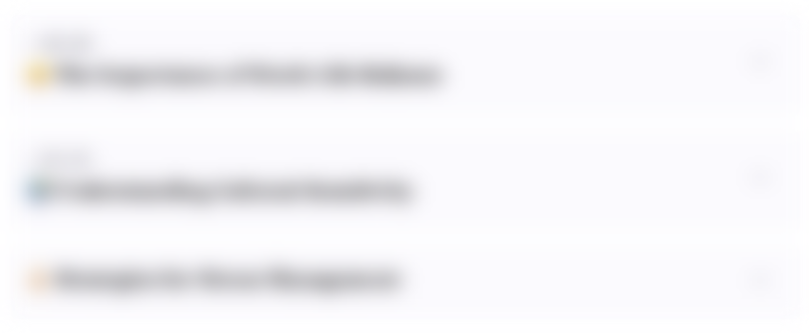
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
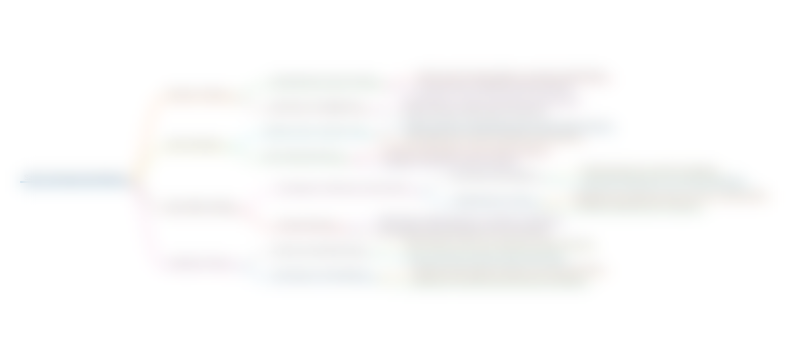
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
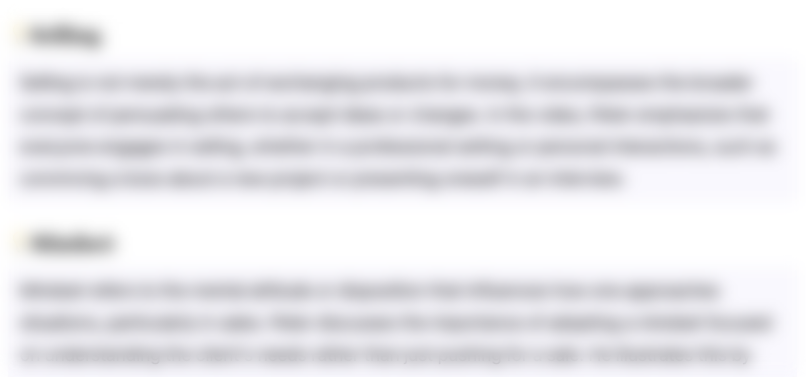
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
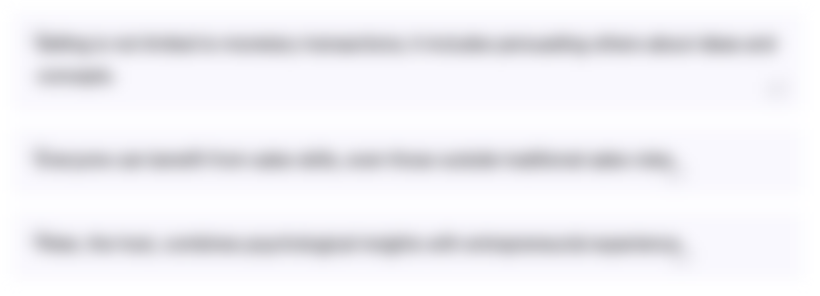
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
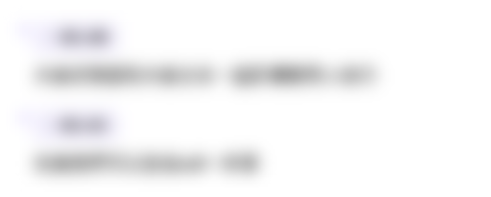
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahora5.0 / 5 (0 votes)